Copyright Agreement
Copyright (c) 2024 Orbit Irrigation Products, LLC.
All rights reserved. This program and the accompanying materials are made available under the terms of the Eclipse Public License v1.0 which accompanies this distribution, and is available at:
http://www.eclipse.org/legal/epl-v10.html
Contributors:
ET Water Systems, Inc. - initial API and implementation
CONTRIBUTION POLICY:
(The following paragraph is not intended to limit the rights granted to you to modify and distribute these APIs under the terms of licenses that may apply to the API.)
Contributions to this API are subject to your understanding and acceptance of the terms and conditions of the ET Water Systems, Inc. Contributor Agreement, which can be found online at:
http://www.etwater.com/legal/contributoragreement/
Introducing the Unity API
ET Unity is a powerful, highly scalable open platform for water management and a wealth of other
environmental services. We have built a sophisticated set of data from many different sources, and used
years of experience to transform and analyze this information. Through a growing set of REST APIs you can directly gain access to the platform's services and insights for easy integration.
Access Environmental Insights:
- Survey class data about land and parcel boundaries
- Profiles and details about soil types and characteristics
- Plant type and growth factors, specifically related to UV, and watering needs and patterns
- Information about climate and weather, including historical, current and forecast events
- Common analysis information like water depletion rates and evapotranspiration (ET) values
We have made the Unity APIs available under an open source access and distribution model. As a result,
it is a simple process to start using the platform services to enable your development efforts, and to start enriching your applications and services.
All you need to do:
- Sign up and immdeiately get an authentication token
- Make sure you understand your licensing and contribution terms and rights
- Use an appropriate development or build environment
- Contact us at developer@etwater.com if you need support or have questions
API Interaction
Any tool for making HTTP requests is suitable to work with the Unity API. Unity API is a REST-like API and following most of the common agreements on HTTP Methods usage. Communication is done in JSON format so any request to the API should be a valid JSON document.
Requests
Any request to the API should be sent via HTTP protocol using corresponding HTTP Method. Requests that contain a request body should have a HTTP header “Content-Type” with a description of content being sent. Also every request should be singed with a personal access token placed in a header.
Header Example:
Authorization: Bearer MWQzMjQ2NjU1NjgxMGQwMzI3MzY0OTE5NmU0ZTQ2NzE3YzYxNmVkZGYxNGE1MWVjOGVkMjZlNjVjMzQ1NDY1NQ
HTTP Methods
Method |
Usage |
GET |
Used for information retrieval. Any request using the GET method is read-only and will not affect any of the objects you are querying |
DELETE |
This method is used to remove any object or collection of objects |
POST |
Used for objects creation. Request should contain all the required fields for an object that being created. Also could be used for performing an actions. For instance, “Force irrigation” action |
PUT |
Used for updating the object. |
PATCH |
Used for bulk operations on multiple objects. |
HTTP Statuses
Each API response contains a corresponding HTTP Status Code.
Codes in a range 200..300 indicate a successful action and means that no error was encountered.
Codes in a range 400..500 indicate that there was an issue with the request that was sent.
Codes in a range 400..500 indicate a server side problem and it means that we are having an issue on our end and cannot fulfill your request currently.
Responses
Typically every response contains a response body as a JSON document. An exception to this are DELETE requests. They always are responded with HTTP status 204 No Content
end empty response body. Most of the responses contain a requested object or a list of object, but in some cases results are wrapped in a structure that included pagination info.
Example of response with pagination:
{
"controllerList": [
{
"id": "example string value",
"serialNumber": "example string value",
"activated": true,
"projectId": "example string value"
}
],
"page": 843,
"size": 213,
"total": 525
}
HTTP Clients
cURL
cURL is installed by default in most popular *nix distributives. It is a widely spread tool and a library for making HTTP requests. cURL is a command line utility and does not have a any UI.
Simple request example:
curl 'https://developer-api.etwater.com/api/v1/hello-unity'
Response:
{
"greetings": "Hello from Unity platform",
"requestMethod: "GET"
}
Request method should be set through -X
console parameter.
PUT request method example:
curl -X PUT 'https://developer-api.etwater.com/api/v1/hello-unity'
Response:
{
"greetings": "Hello from Unity platform",
"requestMethod: "PUT"
}
Request headers should be added should be set through -H
console parameter.
Content-Type and Authorization headers example:
curl -X PUT -H'Content-Type: application/json' -H'Authorization: Bearer $ACCESS_TOKEN' 'https://developer-api.etwater.com/api/v1/hello-unity/auth'
Response:
{
"greetings": "Hello from Unity platform",
"requestMethod: "PUT",
"isAuthenticated": true
}
In last example $ACCESS_TOKEN
placeholder should be replaced by real access token.
POSTMAN
On of the most popular UI application to interact with the HTTP web services is a POSTMAN application (https://www.getpostman.com/). It has an intuitive UI and available on all major platforms as a native application or as a Google’s Chrome extension.
In POSTMAN last cURL request will look like this:
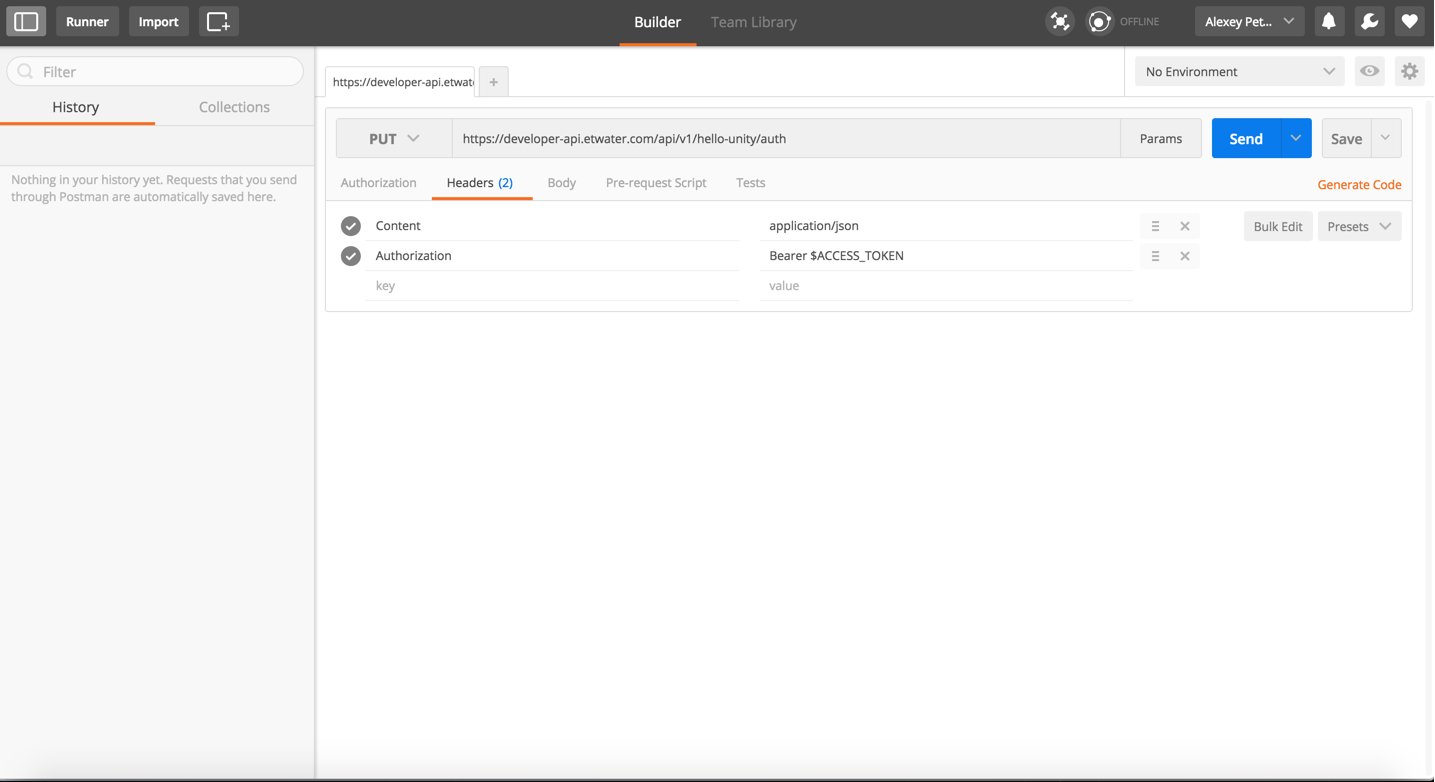
And as a benefit it is possible to generate cURL requests from POSTMAN UI.
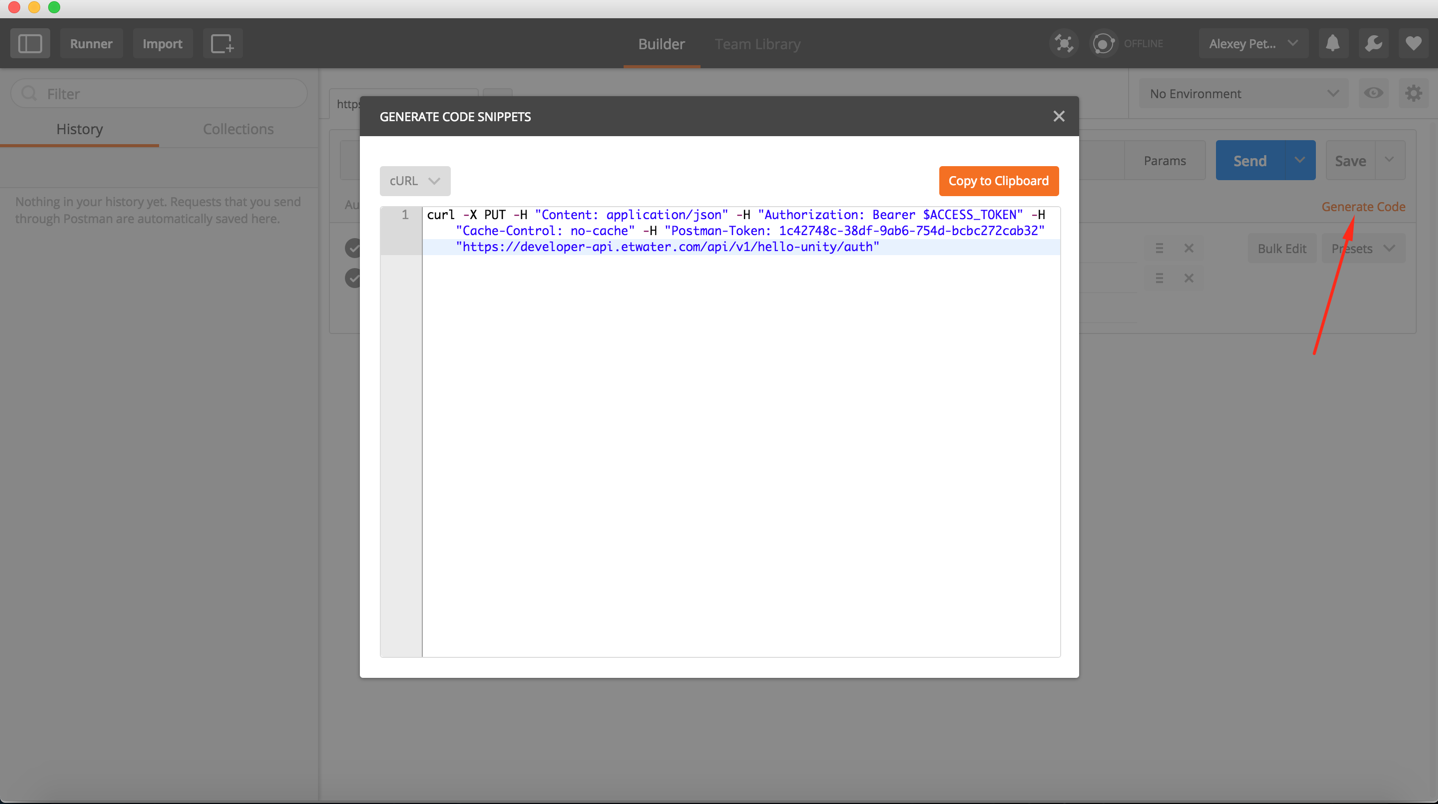
PHPStorm IDE REST Client
PHP as most commonly used language in web development often being used with a PHPStorm IDE. This IDE has a built-in REST Client UI tool that could be used to interact with Unity APIs.
REST client could be found under the Tools menu section:
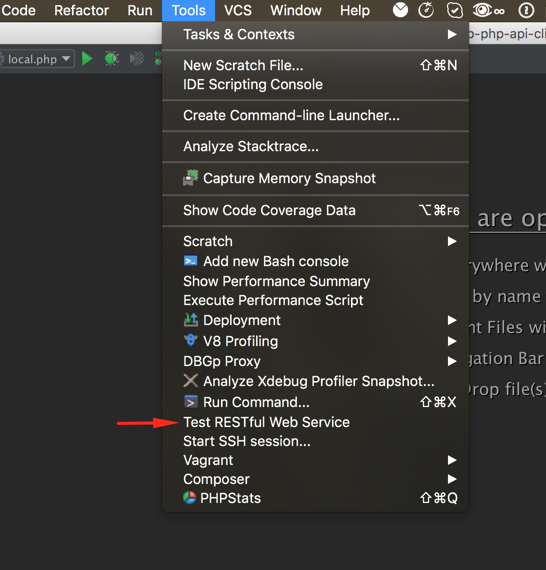
And the last cURL example in this client will look like:
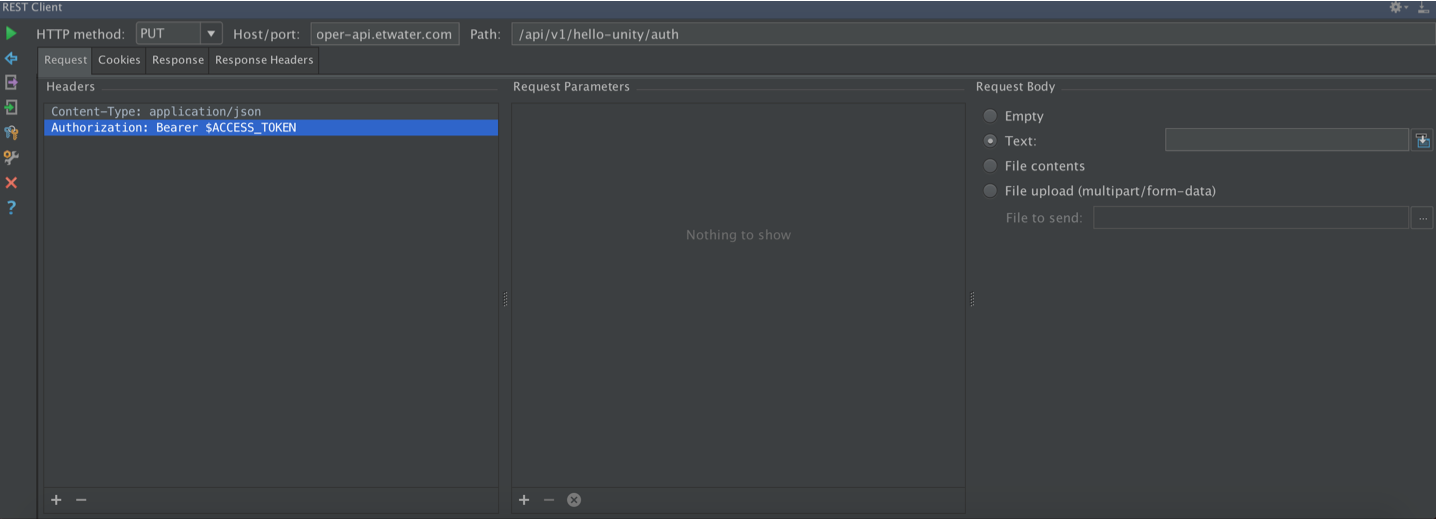
In general, everything remains the same, except an easier HTTP request options setting through IDE UI.
V1 | Communication Statistics | Session Schedule Statistics
Get Session Schedule Statistics
Request
curl "https://developer-api.etwater.com/api/v1/ccu-communication-statistics/session-schedule-statistics?page=0&size=10&sortField=nextSessionDateTime&sortDirection=asc&controllerId=example+string+value&projectId=example+string+value&hasMissedSessions=0&hasMissedSessionsDateFrom=example+string+value&hasMissedSessionsDateTo=example+string+value&unity=1&active=1" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"statistics": [
{
"controllerId": "example string value",
"controllerSerialNumber": "example string value",
"lastSuccessfulSessionDateTime": 316,
"lastScheduledSuccessfulSessionDateTime": 0,
"lastSessionDateTime": 659,
"nextSessionDateTime": 545,
"nextSessionDeadline": 556,
"missedDaysNumber": 979,
"controllerTimezoneOffset": 581,
"userId": "example string value",
"missedSessionsNumber": 690,
"lastSuccessfulSessionId": "example string value",
"lastSessionId": "example string value"
}
],
"page": 349,
"size": 456,
"total": 380
}
HTTP Request
GET /api/v1/ccu-communication-statistics/session-schedule-statistics
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
page |
integer |
query |
false |
|
size |
integer |
query |
false |
|
sortField |
string |
query |
false |
|
sortDirection |
string |
query |
false |
|
controllerId |
string |
query |
false |
|
projectId |
string |
query |
false |
|
hasMissedSessions |
boolean |
query |
false |
|
hasMissedSessionsDateFrom |
string |
query |
false |
|
hasMissedSessionsDateTo |
string |
query |
false |
|
unity |
boolean |
query |
false |
|
active |
boolean |
query |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
statistics |
SessionScheduleStatisticApiResponse[ ] |
false |
|
page |
integer |
false |
|
size |
integer |
false |
|
total |
integer |
false |
|
SessionScheduleStatisticApiResponse
Name |
Type |
Required |
Description |
controllerId |
string |
true |
|
controllerSerialNumber |
string |
true |
|
lastSuccessfulSessionDateTime |
integer |
false |
|
lastScheduledSuccessfulSessionDateTime |
integer |
false |
|
lastSessionDateTime |
integer |
false |
|
nextSessionDateTime |
integer |
false |
|
nextSessionDeadline |
integer |
false |
|
missedDaysNumber |
integer |
false |
|
controllerTimezoneOffset |
integer |
true |
|
userId |
string |
false |
|
missedSessionsNumber |
integer |
false |
|
lastSuccessfulSessionId |
string |
false |
|
lastSessionId |
string |
false |
|
V1 | Controllers | Controller
Request
curl "https://developer-api.etwater.com/api/v1/ccu-management/controllers?page=0&size=10&activated=1&configured=1&suspended=1&projectId=example+string+value&searchTerm=example+string+value&unity=0&controllerStatus%5B0%5D=ACTIVE" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
[
{
"id": "example string value",
"serialNumber": "example string value",
"sim": "example string value",
"imsi": "example string value",
"config": {
"numberOfDials": 614,
"dialScheduleArray": [
{
"secOfDay": 435,
"skipDays": 76,
"retries": 89,
"delayMinutes": 635
}
],
"extraMinutesToFillPipes": 97,
"ip": "example string value",
"secondaryIp": "example string value",
"login": "example string value",
"password": "example string value",
"logString": "example string value",
"pwString": "example string value",
"dialString": "example string value",
"port": 3,
"fertigationValveNumber": 601,
"stationCount": 369,
"flowLimits": {
"overflowGroup0": 748,
"underflowGroup0": 656,
"overflowGroup1": 340,
"underflowGroup1": 602,
"leakLimitPPM": 756
},
"keystrokeTimeout": 33,
"calibrationInfo": {
"inchesOfRainPerTip": 410,
"inchesOfRainStarted": 195,
"inchesOfRainHeavy": 555
},
"shortCircuitThreshold": 202,
"openCircuitThreshold": 110,
"multiValveMode": false,
"disableMSLWReset": true,
"remoteControl": false,
"allowableClockDriftSeconds": 157,
"flowMeasurementEnabled": false,
"requestAverageFlow": true,
"icuTimeZoneOffsetMillis": 895,
"adminSuspendStatus": 498,
"wateringAdjustmentCoefficients": {
"example_property_name": 680.791152492534
},
"maxSimultaneousIrrigationValves": "1",
"enableSMS": true,
"icuModel": "example string value",
"NOT_IN_USE": {
"example_property_name": 731.9446991812181
},
"frequencyAdjustmentsCoefficients": {
"example_property_name": 244
},
"timeZoneIdentifier": "example string value",
"maxStationCount": 38,
"ccuSuspendWatering": false,
"ccuSuspendIsTimed": false
},
"userId": "example string value",
"controllerStatus": "DELETED",
"createdAt": 577,
"updatedAt": 623,
"ccuZones": {
"example_property_name": {
"name": "example string value",
"number": 525,
"projectId": "example string value",
"areaRegionConfigStates": {
"example_property_name": false
},
"configured": true,
"expectedFlowPPM": 199,
"disableFlowSensing": false,
"expectedFlowProcessState": 214,
"startOfUpdatingFlow": 637,
"updatePPM": true,
"gpm": 223,
"customGpm": 390.1341317175581,
"expectedFlowProcessStateUpdatedAt": "example string value",
"stationOverflowGroupNumber": 501
}
},
"flowSensorSettings": {
"flowSensorId": "example string value",
"manufacturer": "example string value",
"type": "example string value",
"factorK": 882,
"offset": 4
},
"configured": false,
"activated": true,
"projectId": "example string value",
"name": "example string value",
"firmwareVersion": "example string value",
"unity": false,
"multiValveSettings": {
"multiValveMode": false,
"maxSimultaneousIrrigationValves": 758,
"masterValve": true,
"boosterPump": false,
"waterAloneStations": [
497
]
},
"connectedAt": 536,
"isPOTS": true,
"modemTypeString": "example string value",
"connectedSuccessfullyAt": 607,
"connectedPlatform": "example string value",
"modemSignalStrength": 566,
"failedCallCount": 983,
"rainSensorType": 328,
"rainSensorEnabled": true,
"icuFamily": "example string value",
"iccid": "example string value",
"nextConnectionAt": 713,
"simNetworkType": "example string value",
"modemTypeNumber": 43
}
]
Dependent on:
- Authorization: Bearer {access_token}
- Content-Type: application/json
where: {access_token} access token value.
Query param description:
- page - number of page;
- size - amount of controllers in single page.
Response body description:
- controllerList - contains all active controllers related to user;
- total - amount of controllers;
- page - number of page;
- size - amount of controllers in single page.
HTTP Request
GET /api/v1/ccu-management/controllers
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
page |
integer |
query |
false |
page number |
size |
integer |
query |
false |
page size |
activated |
boolean |
query |
false |
|
configured |
boolean |
query |
false |
|
suspended |
boolean |
query |
false |
|
projectId |
string |
query |
false |
|
searchTerm |
string |
query |
false |
|
unity |
boolean |
query |
false |
|
controllerStatus |
array |
query |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response is array of
Name |
Type |
Required |
Description |
id |
string |
false |
|
serialNumber |
string |
false |
|
sim |
string |
false |
|
imsi |
string |
false |
|
config |
CcuConfig |
false |
|
userId |
string |
false |
|
controllerStatus |
string |
false |
|
createdAt |
integer |
false |
|
updatedAt |
integer |
false |
|
ccuZones |
Object for field ccuZones |
false |
|
flowSensorSettings |
FlowSensorSettings |
false |
|
configured |
boolean |
false |
|
activated |
boolean |
false |
|
projectId |
string |
false |
|
name |
string |
false |
|
firmwareVersion |
string |
false |
|
unity |
boolean |
false |
|
multiValveSettings |
MultiValveSettings |
false |
|
connectedAt |
integer |
false |
|
isPOTS |
boolean |
false |
|
modemTypeString |
string |
false |
|
connectedSuccessfullyAt |
integer |
false |
|
connectedPlatform |
string |
false |
|
modemSignalStrength |
integer |
false |
|
failedCallCount |
integer |
false |
|
rainSensorType |
integer |
false |
|
rainSensorEnabled |
boolean |
false |
|
icuFamily |
string |
false |
|
iccid |
string |
false |
|
nextConnectionAt |
integer |
false |
|
simNetworkType |
string |
false |
|
modemTypeNumber |
integer |
false |
|
CcuConfig
Name |
Type |
Required |
Description |
numberOfDials |
integer |
false |
|
dialScheduleArray |
DialSchedule[ ] |
false |
|
extraMinutesToFillPipes |
integer |
false |
|
ip |
string |
false |
|
secondaryIp |
string |
false |
|
login |
string |
false |
|
password |
string |
false |
|
logString |
string |
false |
|
pwString |
string |
false |
|
dialString |
string |
false |
|
port |
integer |
false |
|
fertigationValveNumber |
integer |
false |
|
stationCount |
integer |
false |
|
flowLimits |
FlowLimitData |
false |
|
keystrokeTimeout |
integer |
false |
|
calibrationInfo |
TippingBucketCalibration |
false |
|
shortCircuitThreshold |
integer |
false |
|
openCircuitThreshold |
integer |
false |
|
multiValveMode |
boolean |
false |
|
disableMSLWReset |
boolean |
false |
|
remoteControl |
boolean |
false |
|
allowableClockDriftSeconds |
integer |
false |
|
flowMeasurementEnabled |
boolean |
false |
|
requestAverageFlow |
boolean |
false |
|
icuTimeZoneOffsetMillis |
integer |
false |
|
adminSuspendStatus |
integer |
false |
|
wateringAdjustmentCoefficients |
Object for field wateringAdjustmentCoefficients |
false |
|
maxSimultaneousIrrigationValves |
integer |
false |
|
enableSMS |
boolean |
false |
|
icuModel |
string |
false |
|
NOT_IN_USE |
Object for field NOT_IN_USE |
false |
|
frequencyAdjustmentsCoefficients |
Object for field frequencyAdjustmentsCoefficients |
false |
|
timeZoneIdentifier |
string |
false |
|
maxStationCount |
integer |
false |
|
ccuSuspendWatering |
boolean |
false |
|
ccuSuspendIsTimed |
boolean |
false |
|
DialSchedule
Name |
Type |
Required |
Description |
secOfDay |
integer |
true |
|
skipDays |
integer |
true |
|
retries |
integer |
true |
|
delayMinutes |
integer |
true |
|
FlowLimitData
Name |
Type |
Required |
Description |
overflowGroup0 |
integer |
true |
|
underflowGroup0 |
integer |
true |
|
overflowGroup1 |
integer |
true |
|
underflowGroup1 |
integer |
true |
|
leakLimitPPM |
integer |
true |
|
TippingBucketCalibration
Name |
Type |
Required |
Description |
inchesOfRainPerTip |
integer |
false |
|
inchesOfRainStarted |
integer |
false |
|
inchesOfRainHeavy |
integer |
false |
|
Object for field wateringAdjustmentCoefficients
Name |
Type |
Required |
Description |
* |
number |
false |
|
Object for field NOT_IN_USE
Name |
Type |
Required |
Description |
* |
number |
false |
|
Object for field frequencyAdjustmentsCoefficients
Name |
Type |
Required |
Description |
* |
number |
false |
|
Object for field ccuZones
Name |
Type |
Required |
Description |
* |
CCUZone |
false |
|
CCUZone
Name |
Type |
Required |
Description |
name |
string |
false |
|
number |
integer |
false |
|
projectId |
string |
false |
|
areaRegionConfigStates |
Object for field areaRegionConfigStates |
false |
|
configured |
boolean |
false |
|
expectedFlowPPM |
integer |
false |
|
disableFlowSensing |
boolean |
false |
|
expectedFlowProcessState |
integer |
false |
|
startOfUpdatingFlow |
integer |
false |
|
updatePPM |
boolean |
false |
|
gpm |
number |
false |
|
customGpm |
number |
false |
|
expectedFlowProcessStateUpdatedAt |
string |
false |
|
stationOverflowGroupNumber |
integer |
false |
|
Object for field areaRegionConfigStates
Name |
Type |
Required |
Description |
* |
boolean |
false |
|
FlowSensorSettings
Name |
Type |
Required |
Description |
flowSensorId |
string |
false |
|
manufacturer |
string |
false |
|
type |
string |
false |
|
factorK |
number |
false |
|
offset |
number |
false |
|
MultiValveSettings
Name |
Type |
Required |
Description |
multiValveMode |
boolean |
true |
|
maxSimultaneousIrrigationValves |
integer |
true |
|
masterValve |
boolean |
true |
|
boosterPump |
boolean |
true |
|
waterAloneStations |
integer[ ] |
true |
|
Create controller in Unity system.
Request
curl "https://developer-api.etwater.com/api/v1/ccu-management/controllers" \
-X POST \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
--data "{
\"id\": \"example string value\",
\"serialNumber\": \"example string value\",
\"sim\": \"example string value\",
\"imsi\": \"example string value\",
\"config\": {
\"numberOfDials\": 203,
\"dialScheduleArray\": [
{
\"secOfDay\": 416,
\"skipDays\": 637,
\"retries\": 949,
\"delayMinutes\": 328
}
],
\"extraMinutesToFillPipes\": 577,
\"ip\": \"example string value\",
\"secondaryIp\": \"example string value\",
\"login\": \"example string value\",
\"password\": \"example string value\",
\"logString\": \"example string value\",
\"pwString\": \"example string value\",
\"dialString\": \"example string value\",
\"port\": 101,
\"fertigationValveNumber\": 97,
\"stationCount\": 16,
\"flowLimits\": {
\"overflowGroup0\": 810,
\"underflowGroup0\": 74,
\"overflowGroup1\": 911,
\"underflowGroup1\": 278,
\"leakLimitPPM\": 282
},
\"keystrokeTimeout\": 743,
\"calibrationInfo\": {
\"inchesOfRainPerTip\": 73,
\"inchesOfRainStarted\": 80,
\"inchesOfRainHeavy\": 26
},
\"shortCircuitThreshold\": 562,
\"openCircuitThreshold\": 787,
\"multiValveMode\": true,
\"disableMSLWReset\": true,
\"remoteControl\": false,
\"allowableClockDriftSeconds\": 532,
\"flowMeasurementEnabled\": false,
\"requestAverageFlow\": true,
\"icuTimeZoneOffsetMillis\": 64,
\"adminSuspendStatus\": 593,
\"wateringAdjustmentCoefficients\": {
\"example_property_name\": 873.8008937210781
},
\"maxSimultaneousIrrigationValves\": \"1\",
\"enableSMS\": false,
\"icuModel\": \"example string value\",
\"NOT_IN_USE\": {
\"example_property_name\": 155.10872851829453
},
\"frequencyAdjustmentsCoefficients\": {
\"example_property_name\": 772
},
\"timeZoneIdentifier\": \"example string value\",
\"maxStationCount\": 542,
\"ccuSuspendWatering\": true,
\"ccuSuspendIsTimed\": false
},
\"userId\": \"example string value\",
\"controllerStatus\": \"ACTIVE\",
\"createdAt\": 433,
\"updatedAt\": 554,
\"ccuZones\": {
\"example_property_name\": {
\"name\": \"example string value\",
\"number\": 502,
\"projectId\": \"example string value\",
\"areaRegionConfigStates\": {
\"example_property_name\": true
},
\"configured\": false,
\"expectedFlowPPM\": 477,
\"disableFlowSensing\": false,
\"expectedFlowProcessState\": 771,
\"startOfUpdatingFlow\": 758,
\"updatePPM\": true,
\"gpm\": 794,
\"customGpm\": 721.6594464712122,
\"expectedFlowProcessStateUpdatedAt\": \"example string value\",
\"stationOverflowGroupNumber\": 190
}
},
\"flowSensorSettings\": {
\"flowSensorId\": \"example string value\",
\"manufacturer\": \"example string value\",
\"type\": \"example string value\",
\"factorK\": 961,
\"offset\": 165
},
\"configured\": false,
\"activated\": true,
\"projectId\": \"example string value\",
\"name\": \"example string value\",
\"firmwareVersion\": \"example string value\",
\"unity\": false,
\"multiValveSettings\": {
\"multiValveMode\": false,
\"maxSimultaneousIrrigationValves\": 320,
\"masterValve\": false,
\"boosterPump\": false,
\"waterAloneStations\": [
578
]
},
\"connectedAt\": 851,
\"isPOTS\": false,
\"modemTypeString\": \"example string value\",
\"connectedSuccessfullyAt\": 316,
\"connectedPlatform\": \"example string value\",
\"modemSignalStrength\": 701,
\"failedCallCount\": 748,
\"rainSensorType\": 993,
\"rainSensorEnabled\": true,
\"icuFamily\": \"example string value\",
\"iccid\": \"example string value\",
\"nextConnectionAt\": 366,
\"simNetworkType\": \"example string value\",
\"modemTypeNumber\": 791
}"
On success, the above request returns response like
{
"id": "example string value",
"serialNumber": "example string value",
"sim": "example string value",
"imsi": "example string value",
"config": {
"numberOfDials": 870,
"dialScheduleArray": [
{
"secOfDay": 988,
"skipDays": 819,
"retries": 877,
"delayMinutes": 968
}
],
"extraMinutesToFillPipes": 85,
"ip": "example string value",
"secondaryIp": "example string value",
"login": "example string value",
"password": "example string value",
"logString": "example string value",
"pwString": "example string value",
"dialString": "example string value",
"port": 793,
"fertigationValveNumber": 397,
"stationCount": 122,
"flowLimits": {
"overflowGroup0": 527,
"underflowGroup0": 644,
"overflowGroup1": 487,
"underflowGroup1": 137,
"leakLimitPPM": 526
},
"keystrokeTimeout": 546,
"calibrationInfo": {
"inchesOfRainPerTip": 381,
"inchesOfRainStarted": 90,
"inchesOfRainHeavy": 713
},
"shortCircuitThreshold": 512,
"openCircuitThreshold": 254,
"multiValveMode": true,
"disableMSLWReset": true,
"remoteControl": false,
"allowableClockDriftSeconds": 332,
"flowMeasurementEnabled": false,
"requestAverageFlow": false,
"icuTimeZoneOffsetMillis": 911,
"adminSuspendStatus": 558,
"wateringAdjustmentCoefficients": {
"example_property_name": 61.42217855035429
},
"maxSimultaneousIrrigationValves": "1",
"enableSMS": false,
"icuModel": "example string value",
"NOT_IN_USE": {
"example_property_name": 717.3147046553505
},
"frequencyAdjustmentsCoefficients": {
"example_property_name": 70
},
"timeZoneIdentifier": "example string value",
"maxStationCount": 478,
"ccuSuspendWatering": true,
"ccuSuspendIsTimed": false
},
"userId": "example string value",
"controllerStatus": "DELETED",
"createdAt": 478,
"updatedAt": 702,
"ccuZones": {
"example_property_name": {
"name": "example string value",
"number": 284,
"projectId": "example string value",
"areaRegionConfigStates": {
"example_property_name": true
},
"configured": false,
"expectedFlowPPM": 616,
"disableFlowSensing": true,
"expectedFlowProcessState": 756,
"startOfUpdatingFlow": 935,
"updatePPM": false,
"gpm": 892.8356253974306,
"customGpm": 554.7125817065652,
"expectedFlowProcessStateUpdatedAt": "example string value",
"stationOverflowGroupNumber": 780
}
},
"flowSensorSettings": {
"flowSensorId": "example string value",
"manufacturer": "example string value",
"type": "example string value",
"factorK": 682.7301451390284,
"offset": 203
},
"configured": false,
"activated": true,
"projectId": "example string value",
"name": "example string value",
"firmwareVersion": "example string value",
"unity": true,
"multiValveSettings": {
"multiValveMode": true,
"maxSimultaneousIrrigationValves": 137,
"masterValve": true,
"boosterPump": true,
"waterAloneStations": [
83
]
},
"connectedAt": 402,
"isPOTS": false,
"modemTypeString": "example string value",
"connectedSuccessfullyAt": 163,
"connectedPlatform": "example string value",
"modemSignalStrength": 203,
"failedCallCount": 701,
"rainSensorType": 104,
"rainSensorEnabled": false,
"icuFamily": "example string value",
"iccid": "example string value",
"nextConnectionAt": 502,
"simNetworkType": "example string value",
"modemTypeNumber": 670
}
Dependent on:
- Authorization: Bearer {access_token}
- Content-Type: application/json
where: {access_token} access token value.
The simplest way of controller creation is to provide only serialNumber to body request.
Example:
json
{ "serialNumber": <string> }
Request body fields description:
- serialNumber - serial number of controller, it's required and should be unique.
- config - contains configuration of controller, fields:
- icuTimeZoneOffsetMillis - time zone of controller, milliseconds;
Other fields are optional and can be skipped or populated.
In case serial number already exist in system, validation error will be returned.
Response body description:
- id - controller id very important field, it uses in other Unity APIs and methods of CCU Manegement Controllers API;
- serialNumber - serial number of controller.
HTTP Request
POST /api/v1/ccu-management/controllers
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
CcuController |
CcuController |
body |
true |
|
CcuController
Name |
Type |
Required |
Description |
id |
string |
false |
|
serialNumber |
string |
false |
|
sim |
string |
false |
|
imsi |
string |
false |
|
config |
CcuConfig |
false |
|
userId |
string |
false |
|
controllerStatus |
string |
false |
|
createdAt |
integer |
false |
|
updatedAt |
integer |
false |
|
ccuZones |
Object for field ccuZones |
false |
|
flowSensorSettings |
FlowSensorSettings |
false |
|
configured |
boolean |
false |
|
activated |
boolean |
false |
|
projectId |
string |
false |
|
name |
string |
false |
|
firmwareVersion |
string |
false |
|
unity |
boolean |
false |
|
multiValveSettings |
MultiValveSettings |
false |
|
connectedAt |
integer |
false |
|
isPOTS |
boolean |
false |
|
modemTypeString |
string |
false |
|
connectedSuccessfullyAt |
integer |
false |
|
connectedPlatform |
string |
false |
|
modemSignalStrength |
integer |
false |
|
failedCallCount |
integer |
false |
|
rainSensorType |
integer |
false |
|
rainSensorEnabled |
boolean |
false |
|
icuFamily |
string |
false |
|
iccid |
string |
false |
|
nextConnectionAt |
integer |
false |
|
simNetworkType |
string |
false |
|
modemTypeNumber |
integer |
false |
|
CcuConfig
Name |
Type |
Required |
Description |
numberOfDials |
integer |
false |
|
dialScheduleArray |
DialSchedule[ ] |
false |
|
extraMinutesToFillPipes |
integer |
false |
|
ip |
string |
false |
|
secondaryIp |
string |
false |
|
login |
string |
false |
|
password |
string |
false |
|
logString |
string |
false |
|
pwString |
string |
false |
|
dialString |
string |
false |
|
port |
integer |
false |
|
fertigationValveNumber |
integer |
false |
|
stationCount |
integer |
false |
|
flowLimits |
FlowLimitData |
false |
|
keystrokeTimeout |
integer |
false |
|
calibrationInfo |
TippingBucketCalibration |
false |
|
shortCircuitThreshold |
integer |
false |
|
openCircuitThreshold |
integer |
false |
|
multiValveMode |
boolean |
false |
|
disableMSLWReset |
boolean |
false |
|
remoteControl |
boolean |
false |
|
allowableClockDriftSeconds |
integer |
false |
|
flowMeasurementEnabled |
boolean |
false |
|
requestAverageFlow |
boolean |
false |
|
icuTimeZoneOffsetMillis |
integer |
false |
|
adminSuspendStatus |
integer |
false |
|
wateringAdjustmentCoefficients |
Object for field wateringAdjustmentCoefficients |
false |
|
maxSimultaneousIrrigationValves |
integer |
false |
|
enableSMS |
boolean |
false |
|
icuModel |
string |
false |
|
NOT_IN_USE |
Object for field NOT_IN_USE |
false |
|
frequencyAdjustmentsCoefficients |
Object for field frequencyAdjustmentsCoefficients |
false |
|
timeZoneIdentifier |
string |
false |
|
maxStationCount |
integer |
false |
|
ccuSuspendWatering |
boolean |
false |
|
ccuSuspendIsTimed |
boolean |
false |
|
DialSchedule
Name |
Type |
Required |
Description |
secOfDay |
integer |
true |
|
skipDays |
integer |
true |
|
retries |
integer |
true |
|
delayMinutes |
integer |
true |
|
FlowLimitData
Name |
Type |
Required |
Description |
overflowGroup0 |
integer |
true |
|
underflowGroup0 |
integer |
true |
|
overflowGroup1 |
integer |
true |
|
underflowGroup1 |
integer |
true |
|
leakLimitPPM |
integer |
true |
|
TippingBucketCalibration
Name |
Type |
Required |
Description |
inchesOfRainPerTip |
integer |
false |
|
inchesOfRainStarted |
integer |
false |
|
inchesOfRainHeavy |
integer |
false |
|
Object for field wateringAdjustmentCoefficients
Name |
Type |
Required |
Description |
* |
number |
false |
|
Object for field NOT_IN_USE
Name |
Type |
Required |
Description |
* |
number |
false |
|
Object for field frequencyAdjustmentsCoefficients
Name |
Type |
Required |
Description |
* |
number |
false |
|
Object for field ccuZones
Name |
Type |
Required |
Description |
* |
CCUZone |
false |
|
CCUZone
Name |
Type |
Required |
Description |
name |
string |
false |
|
number |
integer |
false |
|
projectId |
string |
false |
|
areaRegionConfigStates |
Object for field areaRegionConfigStates |
false |
|
configured |
boolean |
false |
|
expectedFlowPPM |
integer |
false |
|
disableFlowSensing |
boolean |
false |
|
expectedFlowProcessState |
integer |
false |
|
startOfUpdatingFlow |
integer |
false |
|
updatePPM |
boolean |
false |
|
gpm |
number |
false |
|
customGpm |
number |
false |
|
expectedFlowProcessStateUpdatedAt |
string |
false |
|
stationOverflowGroupNumber |
integer |
false |
|
Object for field areaRegionConfigStates
Name |
Type |
Required |
Description |
* |
boolean |
false |
|
FlowSensorSettings
Name |
Type |
Required |
Description |
flowSensorId |
string |
false |
|
manufacturer |
string |
false |
|
type |
string |
false |
|
factorK |
number |
false |
|
offset |
number |
false |
|
MultiValveSettings
Name |
Type |
Required |
Description |
multiValveMode |
boolean |
true |
|
maxSimultaneousIrrigationValves |
integer |
true |
|
masterValve |
boolean |
true |
|
boosterPump |
boolean |
true |
|
waterAloneStations |
integer[ ] |
true |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
false |
|
serialNumber |
string |
false |
|
sim |
string |
false |
|
imsi |
string |
false |
|
config |
CcuConfig |
false |
|
userId |
string |
false |
|
controllerStatus |
string |
false |
|
createdAt |
integer |
false |
|
updatedAt |
integer |
false |
|
ccuZones |
Object for field ccuZones |
false |
|
flowSensorSettings |
FlowSensorSettings |
false |
|
configured |
boolean |
false |
|
activated |
boolean |
false |
|
projectId |
string |
false |
|
name |
string |
false |
|
firmwareVersion |
string |
false |
|
unity |
boolean |
false |
|
multiValveSettings |
MultiValveSettings |
false |
|
connectedAt |
integer |
false |
|
isPOTS |
boolean |
false |
|
modemTypeString |
string |
false |
|
connectedSuccessfullyAt |
integer |
false |
|
connectedPlatform |
string |
false |
|
modemSignalStrength |
integer |
false |
|
failedCallCount |
integer |
false |
|
rainSensorType |
integer |
false |
|
rainSensorEnabled |
boolean |
false |
|
icuFamily |
string |
false |
|
iccid |
string |
false |
|
nextConnectionAt |
integer |
false |
|
simNetworkType |
string |
false |
|
modemTypeNumber |
integer |
false |
|
CcuConfig
Name |
Type |
Required |
Description |
numberOfDials |
integer |
false |
|
dialScheduleArray |
DialSchedule[ ] |
false |
|
extraMinutesToFillPipes |
integer |
false |
|
ip |
string |
false |
|
secondaryIp |
string |
false |
|
login |
string |
false |
|
password |
string |
false |
|
logString |
string |
false |
|
pwString |
string |
false |
|
dialString |
string |
false |
|
port |
integer |
false |
|
fertigationValveNumber |
integer |
false |
|
stationCount |
integer |
false |
|
flowLimits |
FlowLimitData |
false |
|
keystrokeTimeout |
integer |
false |
|
calibrationInfo |
TippingBucketCalibration |
false |
|
shortCircuitThreshold |
integer |
false |
|
openCircuitThreshold |
integer |
false |
|
multiValveMode |
boolean |
false |
|
disableMSLWReset |
boolean |
false |
|
remoteControl |
boolean |
false |
|
allowableClockDriftSeconds |
integer |
false |
|
flowMeasurementEnabled |
boolean |
false |
|
requestAverageFlow |
boolean |
false |
|
icuTimeZoneOffsetMillis |
integer |
false |
|
adminSuspendStatus |
integer |
false |
|
wateringAdjustmentCoefficients |
Object for field wateringAdjustmentCoefficients |
false |
|
maxSimultaneousIrrigationValves |
integer |
false |
|
enableSMS |
boolean |
false |
|
icuModel |
string |
false |
|
NOT_IN_USE |
Object for field NOT_IN_USE |
false |
|
frequencyAdjustmentsCoefficients |
Object for field frequencyAdjustmentsCoefficients |
false |
|
timeZoneIdentifier |
string |
false |
|
maxStationCount |
integer |
false |
|
ccuSuspendWatering |
boolean |
false |
|
ccuSuspendIsTimed |
boolean |
false |
|
DialSchedule
Name |
Type |
Required |
Description |
secOfDay |
integer |
true |
|
skipDays |
integer |
true |
|
retries |
integer |
true |
|
delayMinutes |
integer |
true |
|
FlowLimitData
Name |
Type |
Required |
Description |
overflowGroup0 |
integer |
true |
|
underflowGroup0 |
integer |
true |
|
overflowGroup1 |
integer |
true |
|
underflowGroup1 |
integer |
true |
|
leakLimitPPM |
integer |
true |
|
TippingBucketCalibration
Name |
Type |
Required |
Description |
inchesOfRainPerTip |
integer |
false |
|
inchesOfRainStarted |
integer |
false |
|
inchesOfRainHeavy |
integer |
false |
|
Object for field wateringAdjustmentCoefficients
Name |
Type |
Required |
Description |
* |
number |
false |
|
Object for field NOT_IN_USE
Name |
Type |
Required |
Description |
* |
number |
false |
|
Object for field frequencyAdjustmentsCoefficients
Name |
Type |
Required |
Description |
* |
number |
false |
|
Object for field ccuZones
Name |
Type |
Required |
Description |
* |
CCUZone |
false |
|
CCUZone
Name |
Type |
Required |
Description |
name |
string |
false |
|
number |
integer |
false |
|
projectId |
string |
false |
|
areaRegionConfigStates |
Object for field areaRegionConfigStates |
false |
|
configured |
boolean |
false |
|
expectedFlowPPM |
integer |
false |
|
disableFlowSensing |
boolean |
false |
|
expectedFlowProcessState |
integer |
false |
|
startOfUpdatingFlow |
integer |
false |
|
updatePPM |
boolean |
false |
|
gpm |
number |
false |
|
customGpm |
number |
false |
|
expectedFlowProcessStateUpdatedAt |
string |
false |
|
stationOverflowGroupNumber |
integer |
false |
|
Object for field areaRegionConfigStates
Name |
Type |
Required |
Description |
* |
boolean |
false |
|
FlowSensorSettings
Name |
Type |
Required |
Description |
flowSensorId |
string |
false |
|
manufacturer |
string |
false |
|
type |
string |
false |
|
factorK |
number |
false |
|
offset |
number |
false |
|
MultiValveSettings
Name |
Type |
Required |
Description |
multiValveMode |
boolean |
true |
|
maxSimultaneousIrrigationValves |
integer |
true |
|
masterValve |
boolean |
true |
|
boosterPump |
boolean |
true |
|
waterAloneStations |
integer[ ] |
true |
|
Create or update controllers
Request
curl "https://developer-api.etwater.com/api/v1/ccu-management/controllers" \
-X PATCH \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
--data "[
{
\"id\": \"example string value\",
\"serialNumber\": \"example string value\",
\"sim\": \"example string value\",
\"imsi\": \"example string value\",
\"config\": {
\"numberOfDials\": 841,
\"dialScheduleArray\": [
{
\"secOfDay\": 138,
\"skipDays\": 508,
\"retries\": 391,
\"delayMinutes\": 58
}
],
\"extraMinutesToFillPipes\": 726,
\"ip\": \"example string value\",
\"secondaryIp\": \"example string value\",
\"login\": \"example string value\",
\"password\": \"example string value\",
\"logString\": \"example string value\",
\"pwString\": \"example string value\",
\"dialString\": \"example string value\",
\"port\": 227,
\"fertigationValveNumber\": 845,
\"stationCount\": 608,
\"flowLimits\": {
\"overflowGroup0\": 422,
\"underflowGroup0\": 815,
\"overflowGroup1\": 380,
\"underflowGroup1\": 74,
\"leakLimitPPM\": 13
},
\"keystrokeTimeout\": 266,
\"calibrationInfo\": {
\"inchesOfRainPerTip\": 248,
\"inchesOfRainStarted\": 914,
\"inchesOfRainHeavy\": 376
},
\"shortCircuitThreshold\": 798,
\"openCircuitThreshold\": 636,
\"multiValveMode\": false,
\"disableMSLWReset\": false,
\"remoteControl\": false,
\"allowableClockDriftSeconds\": 814,
\"flowMeasurementEnabled\": true,
\"requestAverageFlow\": true,
\"icuTimeZoneOffsetMillis\": 423,
\"adminSuspendStatus\": 14,
\"wateringAdjustmentCoefficients\": {
\"example_property_name\": 241.85081303205843
},
\"maxSimultaneousIrrigationValves\": \"1\",
\"enableSMS\": false,
\"icuModel\": \"example string value\",
\"NOT_IN_USE\": {
\"example_property_name\": 764.0293868091095
},
\"frequencyAdjustmentsCoefficients\": {
\"example_property_name\": 479
},
\"timeZoneIdentifier\": \"example string value\",
\"maxStationCount\": 89,
\"ccuSuspendWatering\": false,
\"ccuSuspendIsTimed\": true
},
\"userId\": \"example string value\",
\"controllerStatus\": \"DELETED\",
\"createdAt\": 522,
\"updatedAt\": 469,
\"ccuZones\": {
\"example_property_name\": {
\"name\": \"example string value\",
\"number\": 357,
\"projectId\": \"example string value\",
\"areaRegionConfigStates\": {
\"example_property_name\": false
},
\"configured\": false,
\"expectedFlowPPM\": 199,
\"disableFlowSensing\": false,
\"expectedFlowProcessState\": 953,
\"startOfUpdatingFlow\": 359,
\"updatePPM\": false,
\"gpm\": 858.382681318737,
\"customGpm\": 142,
\"expectedFlowProcessStateUpdatedAt\": \"example string value\",
\"stationOverflowGroupNumber\": 130
}
},
\"flowSensorSettings\": {
\"flowSensorId\": \"example string value\",
\"manufacturer\": \"example string value\",
\"type\": \"example string value\",
\"factorK\": 21,
\"offset\": 696.7007250975356
},
\"configured\": true,
\"activated\": false,
\"projectId\": \"example string value\",
\"name\": \"example string value\",
\"firmwareVersion\": \"example string value\",
\"unity\": false,
\"multiValveSettings\": {
\"multiValveMode\": false,
\"maxSimultaneousIrrigationValves\": 461,
\"masterValve\": false,
\"boosterPump\": false,
\"waterAloneStations\": [
518
]
},
\"connectedAt\": 251,
\"isPOTS\": true,
\"modemTypeString\": \"example string value\",
\"connectedSuccessfullyAt\": 121,
\"connectedPlatform\": \"example string value\",
\"modemSignalStrength\": 241,
\"failedCallCount\": 834,
\"rainSensorType\": 347,
\"rainSensorEnabled\": false,
\"icuFamily\": \"example string value\",
\"iccid\": \"example string value\",
\"nextConnectionAt\": 955,
\"simNetworkType\": \"example string value\",
\"modemTypeNumber\": 502
}
]"
On success, the above request returns response like
[
{
"id": "example string value",
"serialNumber": "example string value",
"sim": "example string value",
"imsi": "example string value",
"config": {
"numberOfDials": 272,
"dialScheduleArray": [
{
"secOfDay": 586,
"skipDays": 125,
"retries": 963,
"delayMinutes": 603
}
],
"extraMinutesToFillPipes": 46,
"ip": "example string value",
"secondaryIp": "example string value",
"login": "example string value",
"password": "example string value",
"logString": "example string value",
"pwString": "example string value",
"dialString": "example string value",
"port": 724,
"fertigationValveNumber": 216,
"stationCount": 165,
"flowLimits": {
"overflowGroup0": 736,
"underflowGroup0": 787,
"overflowGroup1": 558,
"underflowGroup1": 198,
"leakLimitPPM": 651
},
"keystrokeTimeout": 460,
"calibrationInfo": {
"inchesOfRainPerTip": 669,
"inchesOfRainStarted": 502,
"inchesOfRainHeavy": 329
},
"shortCircuitThreshold": 872,
"openCircuitThreshold": 557,
"multiValveMode": true,
"disableMSLWReset": false,
"remoteControl": true,
"allowableClockDriftSeconds": 813,
"flowMeasurementEnabled": true,
"requestAverageFlow": true,
"icuTimeZoneOffsetMillis": 586,
"adminSuspendStatus": 288,
"wateringAdjustmentCoefficients": {
"example_property_name": 848.5608016366888
},
"maxSimultaneousIrrigationValves": "1",
"enableSMS": true,
"icuModel": "example string value",
"NOT_IN_USE": {
"example_property_name": 451
},
"frequencyAdjustmentsCoefficients": {
"example_property_name": 341
},
"timeZoneIdentifier": "example string value",
"maxStationCount": 65,
"ccuSuspendWatering": false,
"ccuSuspendIsTimed": false
},
"userId": "example string value",
"controllerStatus": "DELETED",
"createdAt": 989,
"updatedAt": 299,
"ccuZones": {
"example_property_name": {
"name": "example string value",
"number": 362,
"projectId": "example string value",
"areaRegionConfigStates": {
"example_property_name": false
},
"configured": true,
"expectedFlowPPM": 987,
"disableFlowSensing": false,
"expectedFlowProcessState": 408,
"startOfUpdatingFlow": 814,
"updatePPM": false,
"gpm": 449.74009527347056,
"customGpm": 98,
"expectedFlowProcessStateUpdatedAt": "example string value",
"stationOverflowGroupNumber": 300
}
},
"flowSensorSettings": {
"flowSensorId": "example string value",
"manufacturer": "example string value",
"type": "example string value",
"factorK": 97,
"offset": 118
},
"configured": true,
"activated": false,
"projectId": "example string value",
"name": "example string value",
"firmwareVersion": "example string value",
"unity": false,
"multiValveSettings": {
"multiValveMode": true,
"maxSimultaneousIrrigationValves": 294,
"masterValve": true,
"boosterPump": true,
"waterAloneStations": [
376
]
},
"connectedAt": 274,
"isPOTS": true,
"modemTypeString": "example string value",
"connectedSuccessfullyAt": 93,
"connectedPlatform": "example string value",
"modemSignalStrength": 39,
"failedCallCount": 303,
"rainSensorType": 316,
"rainSensorEnabled": true,
"icuFamily": "example string value",
"iccid": "example string value",
"nextConnectionAt": 231,
"simNetworkType": "example string value",
"modemTypeNumber": 132
}
]
HTTP Request
PATCH /api/v1/ccu-management/controllers
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
CcuControllers |
CcuController[ ] |
body |
true |
|
CcuController
Name |
Type |
Required |
Description |
id |
string |
false |
|
serialNumber |
string |
false |
|
sim |
string |
false |
|
imsi |
string |
false |
|
config |
CcuConfig |
false |
|
userId |
string |
false |
|
controllerStatus |
string |
false |
|
createdAt |
integer |
false |
|
updatedAt |
integer |
false |
|
ccuZones |
Object for field ccuZones |
false |
|
flowSensorSettings |
FlowSensorSettings |
false |
|
configured |
boolean |
false |
|
activated |
boolean |
false |
|
projectId |
string |
false |
|
name |
string |
false |
|
firmwareVersion |
string |
false |
|
unity |
boolean |
false |
|
multiValveSettings |
MultiValveSettings |
false |
|
connectedAt |
integer |
false |
|
isPOTS |
boolean |
false |
|
modemTypeString |
string |
false |
|
connectedSuccessfullyAt |
integer |
false |
|
connectedPlatform |
string |
false |
|
modemSignalStrength |
integer |
false |
|
failedCallCount |
integer |
false |
|
rainSensorType |
integer |
false |
|
rainSensorEnabled |
boolean |
false |
|
icuFamily |
string |
false |
|
iccid |
string |
false |
|
nextConnectionAt |
integer |
false |
|
simNetworkType |
string |
false |
|
modemTypeNumber |
integer |
false |
|
CcuConfig
Name |
Type |
Required |
Description |
numberOfDials |
integer |
false |
|
dialScheduleArray |
DialSchedule[ ] |
false |
|
extraMinutesToFillPipes |
integer |
false |
|
ip |
string |
false |
|
secondaryIp |
string |
false |
|
login |
string |
false |
|
password |
string |
false |
|
logString |
string |
false |
|
pwString |
string |
false |
|
dialString |
string |
false |
|
port |
integer |
false |
|
fertigationValveNumber |
integer |
false |
|
stationCount |
integer |
false |
|
flowLimits |
FlowLimitData |
false |
|
keystrokeTimeout |
integer |
false |
|
calibrationInfo |
TippingBucketCalibration |
false |
|
shortCircuitThreshold |
integer |
false |
|
openCircuitThreshold |
integer |
false |
|
multiValveMode |
boolean |
false |
|
disableMSLWReset |
boolean |
false |
|
remoteControl |
boolean |
false |
|
allowableClockDriftSeconds |
integer |
false |
|
flowMeasurementEnabled |
boolean |
false |
|
requestAverageFlow |
boolean |
false |
|
icuTimeZoneOffsetMillis |
integer |
false |
|
adminSuspendStatus |
integer |
false |
|
wateringAdjustmentCoefficients |
Object for field wateringAdjustmentCoefficients |
false |
|
maxSimultaneousIrrigationValves |
integer |
false |
|
enableSMS |
boolean |
false |
|
icuModel |
string |
false |
|
NOT_IN_USE |
Object for field NOT_IN_USE |
false |
|
frequencyAdjustmentsCoefficients |
Object for field frequencyAdjustmentsCoefficients |
false |
|
timeZoneIdentifier |
string |
false |
|
maxStationCount |
integer |
false |
|
ccuSuspendWatering |
boolean |
false |
|
ccuSuspendIsTimed |
boolean |
false |
|
DialSchedule
Name |
Type |
Required |
Description |
secOfDay |
integer |
true |
|
skipDays |
integer |
true |
|
retries |
integer |
true |
|
delayMinutes |
integer |
true |
|
FlowLimitData
Name |
Type |
Required |
Description |
overflowGroup0 |
integer |
true |
|
underflowGroup0 |
integer |
true |
|
overflowGroup1 |
integer |
true |
|
underflowGroup1 |
integer |
true |
|
leakLimitPPM |
integer |
true |
|
TippingBucketCalibration
Name |
Type |
Required |
Description |
inchesOfRainPerTip |
integer |
false |
|
inchesOfRainStarted |
integer |
false |
|
inchesOfRainHeavy |
integer |
false |
|
Object for field wateringAdjustmentCoefficients
Name |
Type |
Required |
Description |
* |
number |
false |
|
Object for field NOT_IN_USE
Name |
Type |
Required |
Description |
* |
number |
false |
|
Object for field frequencyAdjustmentsCoefficients
Name |
Type |
Required |
Description |
* |
number |
false |
|
Object for field ccuZones
Name |
Type |
Required |
Description |
* |
CCUZone |
false |
|
CCUZone
Name |
Type |
Required |
Description |
name |
string |
false |
|
number |
integer |
false |
|
projectId |
string |
false |
|
areaRegionConfigStates |
Object for field areaRegionConfigStates |
false |
|
configured |
boolean |
false |
|
expectedFlowPPM |
integer |
false |
|
disableFlowSensing |
boolean |
false |
|
expectedFlowProcessState |
integer |
false |
|
startOfUpdatingFlow |
integer |
false |
|
updatePPM |
boolean |
false |
|
gpm |
number |
false |
|
customGpm |
number |
false |
|
expectedFlowProcessStateUpdatedAt |
string |
false |
|
stationOverflowGroupNumber |
integer |
false |
|
Object for field areaRegionConfigStates
Name |
Type |
Required |
Description |
* |
boolean |
false |
|
FlowSensorSettings
Name |
Type |
Required |
Description |
flowSensorId |
string |
false |
|
manufacturer |
string |
false |
|
type |
string |
false |
|
factorK |
number |
false |
|
offset |
number |
false |
|
MultiValveSettings
Name |
Type |
Required |
Description |
multiValveMode |
boolean |
true |
|
maxSimultaneousIrrigationValves |
integer |
true |
|
masterValve |
boolean |
true |
|
boosterPump |
boolean |
true |
|
waterAloneStations |
integer[ ] |
true |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response is array of
Name |
Type |
Required |
Description |
id |
string |
false |
|
serialNumber |
string |
false |
|
sim |
string |
false |
|
imsi |
string |
false |
|
config |
CcuConfig |
false |
|
userId |
string |
false |
|
controllerStatus |
string |
false |
|
createdAt |
integer |
false |
|
updatedAt |
integer |
false |
|
ccuZones |
Object for field ccuZones |
false |
|
flowSensorSettings |
FlowSensorSettings |
false |
|
configured |
boolean |
false |
|
activated |
boolean |
false |
|
projectId |
string |
false |
|
name |
string |
false |
|
firmwareVersion |
string |
false |
|
unity |
boolean |
false |
|
multiValveSettings |
MultiValveSettings |
false |
|
connectedAt |
integer |
false |
|
isPOTS |
boolean |
false |
|
modemTypeString |
string |
false |
|
connectedSuccessfullyAt |
integer |
false |
|
connectedPlatform |
string |
false |
|
modemSignalStrength |
integer |
false |
|
failedCallCount |
integer |
false |
|
rainSensorType |
integer |
false |
|
rainSensorEnabled |
boolean |
false |
|
icuFamily |
string |
false |
|
iccid |
string |
false |
|
nextConnectionAt |
integer |
false |
|
simNetworkType |
string |
false |
|
modemTypeNumber |
integer |
false |
|
CcuConfig
Name |
Type |
Required |
Description |
numberOfDials |
integer |
false |
|
dialScheduleArray |
DialSchedule[ ] |
false |
|
extraMinutesToFillPipes |
integer |
false |
|
ip |
string |
false |
|
secondaryIp |
string |
false |
|
login |
string |
false |
|
password |
string |
false |
|
logString |
string |
false |
|
pwString |
string |
false |
|
dialString |
string |
false |
|
port |
integer |
false |
|
fertigationValveNumber |
integer |
false |
|
stationCount |
integer |
false |
|
flowLimits |
FlowLimitData |
false |
|
keystrokeTimeout |
integer |
false |
|
calibrationInfo |
TippingBucketCalibration |
false |
|
shortCircuitThreshold |
integer |
false |
|
openCircuitThreshold |
integer |
false |
|
multiValveMode |
boolean |
false |
|
disableMSLWReset |
boolean |
false |
|
remoteControl |
boolean |
false |
|
allowableClockDriftSeconds |
integer |
false |
|
flowMeasurementEnabled |
boolean |
false |
|
requestAverageFlow |
boolean |
false |
|
icuTimeZoneOffsetMillis |
integer |
false |
|
adminSuspendStatus |
integer |
false |
|
wateringAdjustmentCoefficients |
Object for field wateringAdjustmentCoefficients |
false |
|
maxSimultaneousIrrigationValves |
integer |
false |
|
enableSMS |
boolean |
false |
|
icuModel |
string |
false |
|
NOT_IN_USE |
Object for field NOT_IN_USE |
false |
|
frequencyAdjustmentsCoefficients |
Object for field frequencyAdjustmentsCoefficients |
false |
|
timeZoneIdentifier |
string |
false |
|
maxStationCount |
integer |
false |
|
ccuSuspendWatering |
boolean |
false |
|
ccuSuspendIsTimed |
boolean |
false |
|
DialSchedule
Name |
Type |
Required |
Description |
secOfDay |
integer |
true |
|
skipDays |
integer |
true |
|
retries |
integer |
true |
|
delayMinutes |
integer |
true |
|
FlowLimitData
Name |
Type |
Required |
Description |
overflowGroup0 |
integer |
true |
|
underflowGroup0 |
integer |
true |
|
overflowGroup1 |
integer |
true |
|
underflowGroup1 |
integer |
true |
|
leakLimitPPM |
integer |
true |
|
TippingBucketCalibration
Name |
Type |
Required |
Description |
inchesOfRainPerTip |
integer |
false |
|
inchesOfRainStarted |
integer |
false |
|
inchesOfRainHeavy |
integer |
false |
|
Object for field wateringAdjustmentCoefficients
Name |
Type |
Required |
Description |
* |
number |
false |
|
Object for field NOT_IN_USE
Name |
Type |
Required |
Description |
* |
number |
false |
|
Object for field frequencyAdjustmentsCoefficients
Name |
Type |
Required |
Description |
* |
number |
false |
|
Object for field ccuZones
Name |
Type |
Required |
Description |
* |
CCUZone |
false |
|
CCUZone
Name |
Type |
Required |
Description |
name |
string |
false |
|
number |
integer |
false |
|
projectId |
string |
false |
|
areaRegionConfigStates |
Object for field areaRegionConfigStates |
false |
|
configured |
boolean |
false |
|
expectedFlowPPM |
integer |
false |
|
disableFlowSensing |
boolean |
false |
|
expectedFlowProcessState |
integer |
false |
|
startOfUpdatingFlow |
integer |
false |
|
updatePPM |
boolean |
false |
|
gpm |
number |
false |
|
customGpm |
number |
false |
|
expectedFlowProcessStateUpdatedAt |
string |
false |
|
stationOverflowGroupNumber |
integer |
false |
|
Object for field areaRegionConfigStates
Name |
Type |
Required |
Description |
* |
boolean |
false |
|
FlowSensorSettings
Name |
Type |
Required |
Description |
flowSensorId |
string |
false |
|
manufacturer |
string |
false |
|
type |
string |
false |
|
factorK |
number |
false |
|
offset |
number |
false |
|
MultiValveSettings
Name |
Type |
Required |
Description |
multiValveMode |
boolean |
true |
|
maxSimultaneousIrrigationValves |
integer |
true |
|
masterValve |
boolean |
true |
|
boosterPump |
boolean |
true |
|
waterAloneStations |
integer[ ] |
true |
|
Retrieves controller by controller id.
Request
curl "https://developer-api.etwater.com/api/v1/ccu-management/controllers/{controllerId}" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"id": "example string value",
"serialNumber": "example string value",
"sim": "example string value",
"imsi": "example string value",
"config": {
"numberOfDials": 936,
"dialScheduleArray": [
{
"secOfDay": 490,
"skipDays": 382,
"retries": 241,
"delayMinutes": 370
}
],
"extraMinutesToFillPipes": 883,
"ip": "example string value",
"secondaryIp": "example string value",
"login": "example string value",
"password": "example string value",
"logString": "example string value",
"pwString": "example string value",
"dialString": "example string value",
"port": 66,
"fertigationValveNumber": 989,
"stationCount": 316,
"flowLimits": {
"overflowGroup0": 764,
"underflowGroup0": 617,
"overflowGroup1": 412,
"underflowGroup1": 687,
"leakLimitPPM": 673
},
"keystrokeTimeout": 381,
"calibrationInfo": {
"inchesOfRainPerTip": 559,
"inchesOfRainStarted": 82,
"inchesOfRainHeavy": 341
},
"shortCircuitThreshold": 865,
"openCircuitThreshold": 911,
"multiValveMode": true,
"disableMSLWReset": true,
"remoteControl": true,
"allowableClockDriftSeconds": 169,
"flowMeasurementEnabled": false,
"requestAverageFlow": true,
"icuTimeZoneOffsetMillis": 226,
"adminSuspendStatus": 945,
"wateringAdjustmentCoefficients": {
"example_property_name": 485
},
"maxSimultaneousIrrigationValves": "1",
"enableSMS": true,
"icuModel": "example string value",
"NOT_IN_USE": {
"example_property_name": 168
},
"frequencyAdjustmentsCoefficients": {
"example_property_name": 257
},
"timeZoneIdentifier": "example string value",
"maxStationCount": 711,
"ccuSuspendWatering": false,
"ccuSuspendIsTimed": false
},
"userId": "example string value",
"controllerStatus": "DEACTIVATED",
"createdAt": 175,
"updatedAt": 475,
"ccuZones": {
"example_property_name": {
"name": "example string value",
"number": 996,
"projectId": "example string value",
"areaRegionConfigStates": {
"example_property_name": true
},
"configured": false,
"expectedFlowPPM": 667,
"disableFlowSensing": true,
"expectedFlowProcessState": 227,
"startOfUpdatingFlow": 71,
"updatePPM": true,
"gpm": 243.88650303887508,
"customGpm": 464.78012412077754,
"expectedFlowProcessStateUpdatedAt": "example string value",
"stationOverflowGroupNumber": 251
}
},
"flowSensorSettings": {
"flowSensorId": "example string value",
"manufacturer": "example string value",
"type": "example string value",
"factorK": 960.8560171727352,
"offset": 889.511228021938
},
"configured": true,
"activated": true,
"projectId": "example string value",
"name": "example string value",
"firmwareVersion": "example string value",
"unity": false,
"multiValveSettings": {
"multiValveMode": true,
"maxSimultaneousIrrigationValves": 317,
"masterValve": false,
"boosterPump": true,
"waterAloneStations": [
814
]
},
"connectedAt": 872,
"isPOTS": true,
"modemTypeString": "example string value",
"connectedSuccessfullyAt": 590,
"connectedPlatform": "example string value",
"modemSignalStrength": 639,
"failedCallCount": 40,
"rainSensorType": 235,
"rainSensorEnabled": false,
"icuFamily": "example string value",
"iccid": "example string value",
"nextConnectionAt": 709,
"simNetworkType": "example string value",
"modemTypeNumber": 168
}
Dependent on:
- Authorization: Bearer {access_token}
- Content-Type: application/json
where: {access_token} access token value.
Path parameter description
{controllerId} - controller id value.
HTTP Request
GET /api/v1/ccu-management/controllers/{controllerId}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
controllerId |
string |
path |
true |
|
HTTP Responses
Code |
Description |
200 |
OK |
404 |
Not Found |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
false |
|
serialNumber |
string |
false |
|
sim |
string |
false |
|
imsi |
string |
false |
|
config |
CcuConfig |
false |
|
userId |
string |
false |
|
controllerStatus |
string |
false |
|
createdAt |
integer |
false |
|
updatedAt |
integer |
false |
|
ccuZones |
Object for field ccuZones |
false |
|
flowSensorSettings |
FlowSensorSettings |
false |
|
configured |
boolean |
false |
|
activated |
boolean |
false |
|
projectId |
string |
false |
|
name |
string |
false |
|
firmwareVersion |
string |
false |
|
unity |
boolean |
false |
|
multiValveSettings |
MultiValveSettings |
false |
|
connectedAt |
integer |
false |
|
isPOTS |
boolean |
false |
|
modemTypeString |
string |
false |
|
connectedSuccessfullyAt |
integer |
false |
|
connectedPlatform |
string |
false |
|
modemSignalStrength |
integer |
false |
|
failedCallCount |
integer |
false |
|
rainSensorType |
integer |
false |
|
rainSensorEnabled |
boolean |
false |
|
icuFamily |
string |
false |
|
iccid |
string |
false |
|
nextConnectionAt |
integer |
false |
|
simNetworkType |
string |
false |
|
modemTypeNumber |
integer |
false |
|
CcuConfig
Name |
Type |
Required |
Description |
numberOfDials |
integer |
false |
|
dialScheduleArray |
DialSchedule[ ] |
false |
|
extraMinutesToFillPipes |
integer |
false |
|
ip |
string |
false |
|
secondaryIp |
string |
false |
|
login |
string |
false |
|
password |
string |
false |
|
logString |
string |
false |
|
pwString |
string |
false |
|
dialString |
string |
false |
|
port |
integer |
false |
|
fertigationValveNumber |
integer |
false |
|
stationCount |
integer |
false |
|
flowLimits |
FlowLimitData |
false |
|
keystrokeTimeout |
integer |
false |
|
calibrationInfo |
TippingBucketCalibration |
false |
|
shortCircuitThreshold |
integer |
false |
|
openCircuitThreshold |
integer |
false |
|
multiValveMode |
boolean |
false |
|
disableMSLWReset |
boolean |
false |
|
remoteControl |
boolean |
false |
|
allowableClockDriftSeconds |
integer |
false |
|
flowMeasurementEnabled |
boolean |
false |
|
requestAverageFlow |
boolean |
false |
|
icuTimeZoneOffsetMillis |
integer |
false |
|
adminSuspendStatus |
integer |
false |
|
wateringAdjustmentCoefficients |
Object for field wateringAdjustmentCoefficients |
false |
|
maxSimultaneousIrrigationValves |
integer |
false |
|
enableSMS |
boolean |
false |
|
icuModel |
string |
false |
|
NOT_IN_USE |
Object for field NOT_IN_USE |
false |
|
frequencyAdjustmentsCoefficients |
Object for field frequencyAdjustmentsCoefficients |
false |
|
timeZoneIdentifier |
string |
false |
|
maxStationCount |
integer |
false |
|
ccuSuspendWatering |
boolean |
false |
|
ccuSuspendIsTimed |
boolean |
false |
|
DialSchedule
Name |
Type |
Required |
Description |
secOfDay |
integer |
true |
|
skipDays |
integer |
true |
|
retries |
integer |
true |
|
delayMinutes |
integer |
true |
|
FlowLimitData
Name |
Type |
Required |
Description |
overflowGroup0 |
integer |
true |
|
underflowGroup0 |
integer |
true |
|
overflowGroup1 |
integer |
true |
|
underflowGroup1 |
integer |
true |
|
leakLimitPPM |
integer |
true |
|
TippingBucketCalibration
Name |
Type |
Required |
Description |
inchesOfRainPerTip |
integer |
false |
|
inchesOfRainStarted |
integer |
false |
|
inchesOfRainHeavy |
integer |
false |
|
Object for field wateringAdjustmentCoefficients
Name |
Type |
Required |
Description |
* |
number |
false |
|
Object for field NOT_IN_USE
Name |
Type |
Required |
Description |
* |
number |
false |
|
Object for field frequencyAdjustmentsCoefficients
Name |
Type |
Required |
Description |
* |
number |
false |
|
Object for field ccuZones
Name |
Type |
Required |
Description |
* |
CCUZone |
false |
|
CCUZone
Name |
Type |
Required |
Description |
name |
string |
false |
|
number |
integer |
false |
|
projectId |
string |
false |
|
areaRegionConfigStates |
Object for field areaRegionConfigStates |
false |
|
configured |
boolean |
false |
|
expectedFlowPPM |
integer |
false |
|
disableFlowSensing |
boolean |
false |
|
expectedFlowProcessState |
integer |
false |
|
startOfUpdatingFlow |
integer |
false |
|
updatePPM |
boolean |
false |
|
gpm |
number |
false |
|
customGpm |
number |
false |
|
expectedFlowProcessStateUpdatedAt |
string |
false |
|
stationOverflowGroupNumber |
integer |
false |
|
Object for field areaRegionConfigStates
Name |
Type |
Required |
Description |
* |
boolean |
false |
|
FlowSensorSettings
Name |
Type |
Required |
Description |
flowSensorId |
string |
false |
|
manufacturer |
string |
false |
|
type |
string |
false |
|
factorK |
number |
false |
|
offset |
number |
false |
|
MultiValveSettings
Name |
Type |
Required |
Description |
multiValveMode |
boolean |
true |
|
maxSimultaneousIrrigationValves |
integer |
true |
|
masterValve |
boolean |
true |
|
boosterPump |
boolean |
true |
|
waterAloneStations |
integer[ ] |
true |
|
Updates controller in Unity system by controller id.
Request
curl "https://developer-api.etwater.com/api/v1/ccu-management/controllers/{controllerId}" \
-X PUT \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
--data "{
\"id\": \"example string value\",
\"serialNumber\": \"example string value\",
\"sim\": \"example string value\",
\"imsi\": \"example string value\",
\"config\": {
\"numberOfDials\": 155,
\"dialScheduleArray\": [
{
\"secOfDay\": 307,
\"skipDays\": 196,
\"retries\": 248,
\"delayMinutes\": 320
}
],
\"extraMinutesToFillPipes\": 216,
\"ip\": \"example string value\",
\"secondaryIp\": \"example string value\",
\"login\": \"example string value\",
\"password\": \"example string value\",
\"logString\": \"example string value\",
\"pwString\": \"example string value\",
\"dialString\": \"example string value\",
\"port\": 684,
\"fertigationValveNumber\": 179,
\"stationCount\": 649,
\"flowLimits\": {
\"overflowGroup0\": 729,
\"underflowGroup0\": 286,
\"overflowGroup1\": 494,
\"underflowGroup1\": 411,
\"leakLimitPPM\": 795
},
\"keystrokeTimeout\": 235,
\"calibrationInfo\": {
\"inchesOfRainPerTip\": 311,
\"inchesOfRainStarted\": 289,
\"inchesOfRainHeavy\": 991
},
\"shortCircuitThreshold\": 838,
\"openCircuitThreshold\": 211,
\"multiValveMode\": true,
\"disableMSLWReset\": true,
\"remoteControl\": false,
\"allowableClockDriftSeconds\": 1,
\"flowMeasurementEnabled\": true,
\"requestAverageFlow\": false,
\"icuTimeZoneOffsetMillis\": 962,
\"adminSuspendStatus\": 106,
\"wateringAdjustmentCoefficients\": {
\"example_property_name\": 289
},
\"maxSimultaneousIrrigationValves\": \"1\",
\"enableSMS\": false,
\"icuModel\": \"example string value\",
\"NOT_IN_USE\": {
\"example_property_name\": 501.2962331535743
},
\"frequencyAdjustmentsCoefficients\": {
\"example_property_name\": 533.2812674032903
},
\"timeZoneIdentifier\": \"example string value\",
\"maxStationCount\": 615,
\"ccuSuspendWatering\": false,
\"ccuSuspendIsTimed\": false
},
\"userId\": \"example string value\",
\"controllerStatus\": \"ACTIVE\",
\"createdAt\": 513,
\"updatedAt\": 67,
\"ccuZones\": {
\"example_property_name\": {
\"name\": \"example string value\",
\"number\": 56,
\"projectId\": \"example string value\",
\"areaRegionConfigStates\": {
\"example_property_name\": true
},
\"configured\": false,
\"expectedFlowPPM\": 803,
\"disableFlowSensing\": true,
\"expectedFlowProcessState\": 506,
\"startOfUpdatingFlow\": 390,
\"updatePPM\": true,
\"gpm\": 724,
\"customGpm\": 697,
\"expectedFlowProcessStateUpdatedAt\": \"example string value\",
\"stationOverflowGroupNumber\": 264
}
},
\"flowSensorSettings\": {
\"flowSensorId\": \"example string value\",
\"manufacturer\": \"example string value\",
\"type\": \"example string value\",
\"factorK\": 376,
\"offset\": 907.0522184050885
},
\"configured\": false,
\"activated\": true,
\"projectId\": \"example string value\",
\"name\": \"example string value\",
\"firmwareVersion\": \"example string value\",
\"unity\": false,
\"multiValveSettings\": {
\"multiValveMode\": true,
\"maxSimultaneousIrrigationValves\": 205,
\"masterValve\": true,
\"boosterPump\": true,
\"waterAloneStations\": [
247
]
},
\"connectedAt\": 499,
\"isPOTS\": false,
\"modemTypeString\": \"example string value\",
\"connectedSuccessfullyAt\": 951,
\"connectedPlatform\": \"example string value\",
\"modemSignalStrength\": 612,
\"failedCallCount\": 137,
\"rainSensorType\": 755,
\"rainSensorEnabled\": false,
\"icuFamily\": \"example string value\",
\"iccid\": \"example string value\",
\"nextConnectionAt\": 748,
\"simNetworkType\": \"example string value\",
\"modemTypeNumber\": 556
}"
On success, the above request returns response like
{
"id": "example string value",
"serialNumber": "example string value",
"sim": "example string value",
"imsi": "example string value",
"config": {
"numberOfDials": 892,
"dialScheduleArray": [
{
"secOfDay": 111,
"skipDays": 782,
"retries": 445,
"delayMinutes": 4
}
],
"extraMinutesToFillPipes": 837,
"ip": "example string value",
"secondaryIp": "example string value",
"login": "example string value",
"password": "example string value",
"logString": "example string value",
"pwString": "example string value",
"dialString": "example string value",
"port": 892,
"fertigationValveNumber": 193,
"stationCount": 882,
"flowLimits": {
"overflowGroup0": 899,
"underflowGroup0": 333,
"overflowGroup1": 743,
"underflowGroup1": 330,
"leakLimitPPM": 45
},
"keystrokeTimeout": 458,
"calibrationInfo": {
"inchesOfRainPerTip": 285,
"inchesOfRainStarted": 217,
"inchesOfRainHeavy": 836
},
"shortCircuitThreshold": 320,
"openCircuitThreshold": 546,
"multiValveMode": true,
"disableMSLWReset": false,
"remoteControl": true,
"allowableClockDriftSeconds": 280,
"flowMeasurementEnabled": true,
"requestAverageFlow": false,
"icuTimeZoneOffsetMillis": 192,
"adminSuspendStatus": 316,
"wateringAdjustmentCoefficients": {
"example_property_name": 820.9776598126524
},
"maxSimultaneousIrrigationValves": "1",
"enableSMS": true,
"icuModel": "example string value",
"NOT_IN_USE": {
"example_property_name": 435.92901455048894
},
"frequencyAdjustmentsCoefficients": {
"example_property_name": 863
},
"timeZoneIdentifier": "example string value",
"maxStationCount": 602,
"ccuSuspendWatering": true,
"ccuSuspendIsTimed": true
},
"userId": "example string value",
"controllerStatus": "DEACTIVATED",
"createdAt": 274,
"updatedAt": 307,
"ccuZones": {
"example_property_name": {
"name": "example string value",
"number": 835,
"projectId": "example string value",
"areaRegionConfigStates": {
"example_property_name": true
},
"configured": true,
"expectedFlowPPM": 375,
"disableFlowSensing": true,
"expectedFlowProcessState": 180,
"startOfUpdatingFlow": 82,
"updatePPM": true,
"gpm": 599.489789269627,
"customGpm": 30,
"expectedFlowProcessStateUpdatedAt": "example string value",
"stationOverflowGroupNumber": 727
}
},
"flowSensorSettings": {
"flowSensorId": "example string value",
"manufacturer": "example string value",
"type": "example string value",
"factorK": 131.5304251068879,
"offset": 25.09345394796387
},
"configured": true,
"activated": true,
"projectId": "example string value",
"name": "example string value",
"firmwareVersion": "example string value",
"unity": true,
"multiValveSettings": {
"multiValveMode": false,
"maxSimultaneousIrrigationValves": 725,
"masterValve": false,
"boosterPump": false,
"waterAloneStations": [
131
]
},
"connectedAt": 665,
"isPOTS": true,
"modemTypeString": "example string value",
"connectedSuccessfullyAt": 193,
"connectedPlatform": "example string value",
"modemSignalStrength": 907,
"failedCallCount": 906,
"rainSensorType": 563,
"rainSensorEnabled": false,
"icuFamily": "example string value",
"iccid": "example string value",
"nextConnectionAt": 346,
"simNetworkType": "example string value",
"modemTypeNumber": 454
}
Note, update operation does not do merge, in case some fields are not set their value will be reset to null.
Dependent on:
- Authorization: Bearer {access_token}
- Content-Type: application/json
where: {access_token} access token value.
Path parameter description
{controllerId} - controller id value.
Request body description:
- serialNumber - serial number of controller, it required and should be unique.
In case serial number already exist in system, validation error will be returned.
HTTP Request
PUT /api/v1/ccu-management/controllers/{controllerId}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
controllerId |
string |
path |
true |
|
CcuController |
CcuController |
body |
true |
|
CcuController
Name |
Type |
Required |
Description |
id |
string |
false |
|
serialNumber |
string |
false |
|
sim |
string |
false |
|
imsi |
string |
false |
|
config |
CcuConfig |
false |
|
userId |
string |
false |
|
controllerStatus |
string |
false |
|
createdAt |
integer |
false |
|
updatedAt |
integer |
false |
|
ccuZones |
Object for field ccuZones |
false |
|
flowSensorSettings |
FlowSensorSettings |
false |
|
configured |
boolean |
false |
|
activated |
boolean |
false |
|
projectId |
string |
false |
|
name |
string |
false |
|
firmwareVersion |
string |
false |
|
unity |
boolean |
false |
|
multiValveSettings |
MultiValveSettings |
false |
|
connectedAt |
integer |
false |
|
isPOTS |
boolean |
false |
|
modemTypeString |
string |
false |
|
connectedSuccessfullyAt |
integer |
false |
|
connectedPlatform |
string |
false |
|
modemSignalStrength |
integer |
false |
|
failedCallCount |
integer |
false |
|
rainSensorType |
integer |
false |
|
rainSensorEnabled |
boolean |
false |
|
icuFamily |
string |
false |
|
iccid |
string |
false |
|
nextConnectionAt |
integer |
false |
|
simNetworkType |
string |
false |
|
modemTypeNumber |
integer |
false |
|
CcuConfig
Name |
Type |
Required |
Description |
numberOfDials |
integer |
false |
|
dialScheduleArray |
DialSchedule[ ] |
false |
|
extraMinutesToFillPipes |
integer |
false |
|
ip |
string |
false |
|
secondaryIp |
string |
false |
|
login |
string |
false |
|
password |
string |
false |
|
logString |
string |
false |
|
pwString |
string |
false |
|
dialString |
string |
false |
|
port |
integer |
false |
|
fertigationValveNumber |
integer |
false |
|
stationCount |
integer |
false |
|
flowLimits |
FlowLimitData |
false |
|
keystrokeTimeout |
integer |
false |
|
calibrationInfo |
TippingBucketCalibration |
false |
|
shortCircuitThreshold |
integer |
false |
|
openCircuitThreshold |
integer |
false |
|
multiValveMode |
boolean |
false |
|
disableMSLWReset |
boolean |
false |
|
remoteControl |
boolean |
false |
|
allowableClockDriftSeconds |
integer |
false |
|
flowMeasurementEnabled |
boolean |
false |
|
requestAverageFlow |
boolean |
false |
|
icuTimeZoneOffsetMillis |
integer |
false |
|
adminSuspendStatus |
integer |
false |
|
wateringAdjustmentCoefficients |
Object for field wateringAdjustmentCoefficients |
false |
|
maxSimultaneousIrrigationValves |
integer |
false |
|
enableSMS |
boolean |
false |
|
icuModel |
string |
false |
|
NOT_IN_USE |
Object for field NOT_IN_USE |
false |
|
frequencyAdjustmentsCoefficients |
Object for field frequencyAdjustmentsCoefficients |
false |
|
timeZoneIdentifier |
string |
false |
|
maxStationCount |
integer |
false |
|
ccuSuspendWatering |
boolean |
false |
|
ccuSuspendIsTimed |
boolean |
false |
|
DialSchedule
Name |
Type |
Required |
Description |
secOfDay |
integer |
true |
|
skipDays |
integer |
true |
|
retries |
integer |
true |
|
delayMinutes |
integer |
true |
|
FlowLimitData
Name |
Type |
Required |
Description |
overflowGroup0 |
integer |
true |
|
underflowGroup0 |
integer |
true |
|
overflowGroup1 |
integer |
true |
|
underflowGroup1 |
integer |
true |
|
leakLimitPPM |
integer |
true |
|
TippingBucketCalibration
Name |
Type |
Required |
Description |
inchesOfRainPerTip |
integer |
false |
|
inchesOfRainStarted |
integer |
false |
|
inchesOfRainHeavy |
integer |
false |
|
Object for field wateringAdjustmentCoefficients
Name |
Type |
Required |
Description |
* |
number |
false |
|
Object for field NOT_IN_USE
Name |
Type |
Required |
Description |
* |
number |
false |
|
Object for field frequencyAdjustmentsCoefficients
Name |
Type |
Required |
Description |
* |
number |
false |
|
Object for field ccuZones
Name |
Type |
Required |
Description |
* |
CCUZone |
false |
|
CCUZone
Name |
Type |
Required |
Description |
name |
string |
false |
|
number |
integer |
false |
|
projectId |
string |
false |
|
areaRegionConfigStates |
Object for field areaRegionConfigStates |
false |
|
configured |
boolean |
false |
|
expectedFlowPPM |
integer |
false |
|
disableFlowSensing |
boolean |
false |
|
expectedFlowProcessState |
integer |
false |
|
startOfUpdatingFlow |
integer |
false |
|
updatePPM |
boolean |
false |
|
gpm |
number |
false |
|
customGpm |
number |
false |
|
expectedFlowProcessStateUpdatedAt |
string |
false |
|
stationOverflowGroupNumber |
integer |
false |
|
Object for field areaRegionConfigStates
Name |
Type |
Required |
Description |
* |
boolean |
false |
|
FlowSensorSettings
Name |
Type |
Required |
Description |
flowSensorId |
string |
false |
|
manufacturer |
string |
false |
|
type |
string |
false |
|
factorK |
number |
false |
|
offset |
number |
false |
|
MultiValveSettings
Name |
Type |
Required |
Description |
multiValveMode |
boolean |
true |
|
maxSimultaneousIrrigationValves |
integer |
true |
|
masterValve |
boolean |
true |
|
boosterPump |
boolean |
true |
|
waterAloneStations |
integer[ ] |
true |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
false |
|
serialNumber |
string |
false |
|
sim |
string |
false |
|
imsi |
string |
false |
|
config |
CcuConfig |
false |
|
userId |
string |
false |
|
controllerStatus |
string |
false |
|
createdAt |
integer |
false |
|
updatedAt |
integer |
false |
|
ccuZones |
Object for field ccuZones |
false |
|
flowSensorSettings |
FlowSensorSettings |
false |
|
configured |
boolean |
false |
|
activated |
boolean |
false |
|
projectId |
string |
false |
|
name |
string |
false |
|
firmwareVersion |
string |
false |
|
unity |
boolean |
false |
|
multiValveSettings |
MultiValveSettings |
false |
|
connectedAt |
integer |
false |
|
isPOTS |
boolean |
false |
|
modemTypeString |
string |
false |
|
connectedSuccessfullyAt |
integer |
false |
|
connectedPlatform |
string |
false |
|
modemSignalStrength |
integer |
false |
|
failedCallCount |
integer |
false |
|
rainSensorType |
integer |
false |
|
rainSensorEnabled |
boolean |
false |
|
icuFamily |
string |
false |
|
iccid |
string |
false |
|
nextConnectionAt |
integer |
false |
|
simNetworkType |
string |
false |
|
modemTypeNumber |
integer |
false |
|
CcuConfig
Name |
Type |
Required |
Description |
numberOfDials |
integer |
false |
|
dialScheduleArray |
DialSchedule[ ] |
false |
|
extraMinutesToFillPipes |
integer |
false |
|
ip |
string |
false |
|
secondaryIp |
string |
false |
|
login |
string |
false |
|
password |
string |
false |
|
logString |
string |
false |
|
pwString |
string |
false |
|
dialString |
string |
false |
|
port |
integer |
false |
|
fertigationValveNumber |
integer |
false |
|
stationCount |
integer |
false |
|
flowLimits |
FlowLimitData |
false |
|
keystrokeTimeout |
integer |
false |
|
calibrationInfo |
TippingBucketCalibration |
false |
|
shortCircuitThreshold |
integer |
false |
|
openCircuitThreshold |
integer |
false |
|
multiValveMode |
boolean |
false |
|
disableMSLWReset |
boolean |
false |
|
remoteControl |
boolean |
false |
|
allowableClockDriftSeconds |
integer |
false |
|
flowMeasurementEnabled |
boolean |
false |
|
requestAverageFlow |
boolean |
false |
|
icuTimeZoneOffsetMillis |
integer |
false |
|
adminSuspendStatus |
integer |
false |
|
wateringAdjustmentCoefficients |
Object for field wateringAdjustmentCoefficients |
false |
|
maxSimultaneousIrrigationValves |
integer |
false |
|
enableSMS |
boolean |
false |
|
icuModel |
string |
false |
|
NOT_IN_USE |
Object for field NOT_IN_USE |
false |
|
frequencyAdjustmentsCoefficients |
Object for field frequencyAdjustmentsCoefficients |
false |
|
timeZoneIdentifier |
string |
false |
|
maxStationCount |
integer |
false |
|
ccuSuspendWatering |
boolean |
false |
|
ccuSuspendIsTimed |
boolean |
false |
|
DialSchedule
Name |
Type |
Required |
Description |
secOfDay |
integer |
true |
|
skipDays |
integer |
true |
|
retries |
integer |
true |
|
delayMinutes |
integer |
true |
|
FlowLimitData
Name |
Type |
Required |
Description |
overflowGroup0 |
integer |
true |
|
underflowGroup0 |
integer |
true |
|
overflowGroup1 |
integer |
true |
|
underflowGroup1 |
integer |
true |
|
leakLimitPPM |
integer |
true |
|
TippingBucketCalibration
Name |
Type |
Required |
Description |
inchesOfRainPerTip |
integer |
false |
|
inchesOfRainStarted |
integer |
false |
|
inchesOfRainHeavy |
integer |
false |
|
Object for field wateringAdjustmentCoefficients
Name |
Type |
Required |
Description |
* |
number |
false |
|
Object for field NOT_IN_USE
Name |
Type |
Required |
Description |
* |
number |
false |
|
Object for field frequencyAdjustmentsCoefficients
Name |
Type |
Required |
Description |
* |
number |
false |
|
Object for field ccuZones
Name |
Type |
Required |
Description |
* |
CCUZone |
false |
|
CCUZone
Name |
Type |
Required |
Description |
name |
string |
false |
|
number |
integer |
false |
|
projectId |
string |
false |
|
areaRegionConfigStates |
Object for field areaRegionConfigStates |
false |
|
configured |
boolean |
false |
|
expectedFlowPPM |
integer |
false |
|
disableFlowSensing |
boolean |
false |
|
expectedFlowProcessState |
integer |
false |
|
startOfUpdatingFlow |
integer |
false |
|
updatePPM |
boolean |
false |
|
gpm |
number |
false |
|
customGpm |
number |
false |
|
expectedFlowProcessStateUpdatedAt |
string |
false |
|
stationOverflowGroupNumber |
integer |
false |
|
Object for field areaRegionConfigStates
Name |
Type |
Required |
Description |
* |
boolean |
false |
|
FlowSensorSettings
Name |
Type |
Required |
Description |
flowSensorId |
string |
false |
|
manufacturer |
string |
false |
|
type |
string |
false |
|
factorK |
number |
false |
|
offset |
number |
false |
|
MultiValveSettings
Name |
Type |
Required |
Description |
multiValveMode |
boolean |
true |
|
maxSimultaneousIrrigationValves |
integer |
true |
|
masterValve |
boolean |
true |
|
boosterPump |
boolean |
true |
|
waterAloneStations |
integer[ ] |
true |
|
V1 | Landscape | Search
The Sites Healthcare Landscape API returns landscape characteristics for a particular location, including elevation, slope, soil type and its percentage of clay, permiability, etc.
Get landscape data for a given location
Request
curl "https://developer-api.etwater.com/api/v1/sites-healthcare/landscape?lat=272&lng=180.73572087136" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"landscapeData": {
"id": "example string value",
"muKey": "example string value",
"elevation": 505,
"slope": 136.32725232109766,
"asa": 855,
"albedoDry": 728.5816430712965,
"createdAt": 765,
"updatedAt": 32,
"soilComponents": [
{
"componentKey": "example string value",
"componentName": "example string value",
"componentPercents": 331,
"layers": [
{
"textureClass": 831,
"paw": 524.8478425316735,
"horizonName": "example string value",
"sandPercents": 45,
"siltPercents": 636.6945210083827,
"clayPercents": 417,
"horizonDepth": 591,
"horizonThickness": 785,
"soilRate": 592
}
]
}
]
},
"landscapeDetails": {
"id": "example string value",
"elevationMeters": 144.98141787246868,
"allowedSurfaceAccumulation": 701.9965726425855,
"plantAvailableWater": 782.598220176342,
"soakRateMmPerMinute": 891,
"slopeDegree": 770
}
}
HTTP Request
GET /api/v1/sites-healthcare/landscape
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
lat |
number |
query |
true |
latitude |
lng |
number |
query |
true |
longtitude |
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
landscapeData |
LandscapeData |
false |
|
landscapeDetails |
LandscapeDetails |
false |
|
LandscapeData
Name |
Type |
Required |
Description |
id |
string |
false |
|
muKey |
string |
false |
|
elevation |
number |
false |
|
slope |
number |
false |
|
asa |
number |
false |
|
albedoDry |
number |
false |
|
createdAt |
integer |
false |
|
updatedAt |
integer |
false |
|
soilComponents |
SoilComponent[ ] |
false |
|
SoilComponent
Name |
Type |
Required |
Description |
componentKey |
string |
false |
|
componentName |
string |
false |
|
componentPercents |
integer |
false |
|
layers |
SoilLayer[ ] |
false |
|
SoilLayer
Name |
Type |
Required |
Description |
textureClass |
integer |
false |
|
paw |
number |
false |
|
horizonName |
string |
false |
|
sandPercents |
number |
false |
|
siltPercents |
number |
false |
|
clayPercents |
number |
false |
|
horizonDepth |
integer |
false |
|
horizonThickness |
integer |
false |
|
soilRate |
number |
false |
|
LandscapeDetails
Name |
Type |
Required |
Description |
id |
string |
false |
|
elevationMeters |
number |
false |
|
allowedSurfaceAccumulation |
number |
false |
|
plantAvailableWater |
number |
false |
|
soakRateMmPerMinute |
number |
false |
|
slopeDegree |
integer |
false |
|
V1 | OAUTH | Authentication
The API Gateway OAuth is used to create, manage and validate logins for your users and give them access to ET Unity services.
Logout
Request
curl "https://developer-api.etwater.com/api/v1/oauth/logout" \
-X POST \
-H "Authorization: example string value"
On success, the above request returns response like
HTTP Request
POST /api/v1/oauth/logout
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
HTTP Responses
Code |
Description |
204 |
Successful Response |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Get Authentication Token
Request
curl "https://developer-api.etwater.com/api/v1/oauth/token" \
-X POST \
-H "Authorization: example string value" \
-H "Content-Type: application/x-www-form-urlencoded" \
--data "grant_type=example+string+value&username=example+string+value&password=example+string+value&scope=example+string+value&is_single_use=example+string+value"
On success, the above request returns response like
{
"access_token": "example string value",
"expires_in": 271,
"token_type": "example string value",
"scope": "example string value",
"refresh_token": "example string value"
}
HTTP Request
POST /api/v1/oauth/token
Request parameters
Name |
Type |
Part |
Required |
Description |
grant_type |
string |
formData |
true |
|
username |
string |
formData |
false |
|
password |
string |
formData |
false |
|
scope |
string |
formData |
false |
|
is_single_use |
string |
formData |
false |
|
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
HTTP Responses
Code |
Description |
200 |
Successful Response |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
access_token |
string |
true |
|
expires_in |
integer |
true |
|
token_type |
string |
true |
|
scope |
string |
false |
|
refresh_token |
string |
false |
|
V1 | OIP | Online Report
The Sites Dashboard OIP Report API creates, or retrieves a previously created, ETwater Optimized Irrigation Plan (OIP). An OIP is a detailed report with projected water use and local soil and climate data, including several graphs and tables. See it in use at www.etwater.com/plan
Create OIP Report
Request
curl "https://developer-api.etwater.com/api/v1/sites-dashboard/oip/projects/{projectId}/reports" \
-X POST \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
--data "{
\"id\": \"example string value\",
\"projectId\": \"example string value\"
}"
On success, the above request returns response like
{
"status": 128,
"errorMessage": "example string value",
"report": {
"id": "example string value",
"projectId": "example string value",
"waterUsageReport": {
"id": "example string value",
"usageGallons": 608,
"avgPerSqFtGallons": 106.42525465526863,
"observedDays": 101,
"monthlyWaterUsageItems": [
{
"timestamp": 877,
"rainIn": 64,
"rainGallons": 211,
"etIn": 940.091890255032,
"usageGallons": 583.1659872006466,
"usageByRegionType": {
"example_property_name": 432
}
}
],
"vegetationCoverageSqFt": {
"example_property_name": 733.4712090592232
},
"dailyTempExtremes": [
{
"timestamp": 821,
"minTemp": 224.73930205439186,
"maxTemp": 819.9921771045738
}
],
"monthlyTempExtremes": [
{
"minTempTimestamp": 808,
"minTemp": 359.82407180584227,
"maxTempTimestamp": 530,
"maxTemp": 967
}
],
"monthlyRainExtremes": [
{
"timestamp": 121,
"rainIn": 731.3773085974983
}
],
"cloudCoverage": [
{
"startTimestamp": 487,
"endTimestamp": 917,
"value": 367.7558788879569
}
],
"tempCoverage": [
{
"startTimestamp": 770,
"endTimestamp": 805,
"value": 531.2199497275147
}
],
"verbalWeather": {
"maxTempTimestamp": 83,
"maxTemp": 867.2833996206911,
"maxTempMonthTempAvg": 253.42354097097348,
"maxTempMonthTempAvgTimestamp": 47,
"minTempTimestamp": 199,
"minTemp": 170.50065480661607,
"minTempMonthTempAvg": 730.6676705976331,
"minTempMonthTempAvgTimestamp": 150,
"frzDays": 555,
"frzSpellDays": 655,
"frzSpellStartTimestamp": 962,
"frzSpellEndTimestamp": 446,
"maxRainIn": 815.6981197258915,
"maxRainTimestamp": 925,
"maxTotalRainMonthIn": 910,
"maxTotalRainMonthInTimestamp": 524
},
"generationTime": 513
}
}
}
HTTP Request
POST /api/v1/sites-dashboard/oip/projects/{projectId}/reports
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
oipReportRequest |
OIPReportRequest |
body |
true |
|
OIPReportRequest
Name |
Type |
Required |
Description |
id |
string |
false |
|
projectId |
string |
false |
|
HTTP Responses
Code |
Description |
200 |
Successful Response |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
status |
integer |
true |
|
errorMessage |
string |
false |
|
report |
OIPReport |
false |
|
OIPReport
Name |
Type |
Required |
Description |
id |
string |
true |
|
projectId |
string |
true |
|
waterUsageReport |
WaterUsageReport |
false |
|
WaterUsageReport
Name |
Type |
Required |
Description |
id |
string |
true |
|
usageGallons |
number |
false |
|
avgPerSqFtGallons |
number |
false |
|
observedDays |
integer |
false |
|
monthlyWaterUsageItems |
MonthlyWaterUsageData[ ] |
false |
|
vegetationCoverageSqFt |
Object for field vegetationCoverageSqFt |
false |
|
dailyTempExtremes |
DailyTempExtreme[ ] |
false |
|
monthlyTempExtremes |
MonthlyTempExtreme[ ] |
false |
|
monthlyRainExtremes |
MontlhyRainExtreme[ ] |
false |
|
cloudCoverage |
CloudCoverageHeatMap[ ] |
false |
|
tempCoverage |
TempCoverageHeatMap[ ] |
false |
|
verbalWeather |
VerbalWeather |
false |
|
generationTime |
integer |
false |
|
MonthlyWaterUsageData
Name |
Type |
Required |
Description |
timestamp |
integer |
true |
|
rainIn |
number |
false |
|
rainGallons |
number |
false |
|
etIn |
number |
false |
|
usageGallons |
number |
false |
|
usageByRegionType |
Object for field usageByRegionType |
false |
|
Object for field usageByRegionType
Name |
Type |
Required |
Description |
* |
number |
false |
|
Object for field vegetationCoverageSqFt
Name |
Type |
Required |
Description |
* |
number |
false |
|
DailyTempExtreme
Name |
Type |
Required |
Description |
timestamp |
integer |
true |
|
minTemp |
number |
false |
|
maxTemp |
number |
false |
|
MonthlyTempExtreme
Name |
Type |
Required |
Description |
minTempTimestamp |
integer |
false |
|
minTemp |
number |
false |
|
maxTempTimestamp |
integer |
false |
|
maxTemp |
number |
false |
|
MontlhyRainExtreme
Name |
Type |
Required |
Description |
timestamp |
integer |
false |
|
rainIn |
number |
false |
|
CloudCoverageHeatMap
Name |
Type |
Required |
Description |
startTimestamp |
integer |
false |
|
endTimestamp |
integer |
false |
|
value |
number |
false |
|
TempCoverageHeatMap
Name |
Type |
Required |
Description |
startTimestamp |
integer |
false |
|
endTimestamp |
integer |
false |
|
value |
number |
false |
|
VerbalWeather
Name |
Type |
Required |
Description |
maxTempTimestamp |
integer |
false |
|
maxTemp |
number |
false |
|
maxTempMonthTempAvg |
number |
false |
|
maxTempMonthTempAvgTimestamp |
integer |
false |
|
minTempTimestamp |
integer |
false |
|
minTemp |
number |
false |
|
minTempMonthTempAvg |
number |
false |
|
minTempMonthTempAvgTimestamp |
integer |
false |
|
frzDays |
integer |
false |
|
frzSpellDays |
integer |
false |
|
frzSpellStartTimestamp |
integer |
false |
|
frzSpellEndTimestamp |
integer |
false |
|
maxRainIn |
number |
false |
|
maxRainTimestamp |
integer |
false |
|
maxTotalRainMonthIn |
number |
false |
|
maxTotalRainMonthInTimestamp |
integer |
false |
|
Get OIP report for project
Request
curl "https://developer-api.etwater.com/api/v1/sites-dashboard/oip/reports/{reportId}" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"status": 16,
"errorMessage": "example string value",
"report": {
"id": "example string value",
"projectId": "example string value",
"waterUsageReport": {
"id": "example string value",
"usageGallons": 363.9130333270473,
"avgPerSqFtGallons": 285.7567566846296,
"observedDays": 297,
"monthlyWaterUsageItems": [
{
"timestamp": 331,
"rainIn": 543,
"rainGallons": 558.5955244296209,
"etIn": 657,
"usageGallons": 683,
"usageByRegionType": {
"example_property_name": 245
}
}
],
"vegetationCoverageSqFt": {
"example_property_name": 483
},
"dailyTempExtremes": [
{
"timestamp": 831,
"minTemp": 648.2893115134394,
"maxTemp": 369.41363120889923
}
],
"monthlyTempExtremes": [
{
"minTempTimestamp": 711,
"minTemp": 238.04795985950528,
"maxTempTimestamp": 982,
"maxTemp": 503.70576069862847
}
],
"monthlyRainExtremes": [
{
"timestamp": 515,
"rainIn": 118.10252262191032
}
],
"cloudCoverage": [
{
"startTimestamp": 231,
"endTimestamp": 347,
"value": 973.3389639171488
}
],
"tempCoverage": [
{
"startTimestamp": 664,
"endTimestamp": 182,
"value": 232.08835592124998
}
],
"verbalWeather": {
"maxTempTimestamp": 926,
"maxTemp": 316.41135565769457,
"maxTempMonthTempAvg": 711,
"maxTempMonthTempAvgTimestamp": 472,
"minTempTimestamp": 242,
"minTemp": 935.0487063336413,
"minTempMonthTempAvg": 357,
"minTempMonthTempAvgTimestamp": 409,
"frzDays": 228,
"frzSpellDays": 992,
"frzSpellStartTimestamp": 98,
"frzSpellEndTimestamp": 219,
"maxRainIn": 443.1163149155287,
"maxRainTimestamp": 537,
"maxTotalRainMonthIn": 682.0023891897882,
"maxTotalRainMonthInTimestamp": 663
},
"generationTime": 234
}
}
}
HTTP Request
GET /api/v1/sites-dashboard/oip/reports/{reportId}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
reportId |
string |
path |
true |
|
HTTP Responses
Code |
Description |
200 |
Successful Response |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
status |
integer |
true |
|
errorMessage |
string |
false |
|
report |
OIPReport |
false |
|
OIPReport
Name |
Type |
Required |
Description |
id |
string |
true |
|
projectId |
string |
true |
|
waterUsageReport |
WaterUsageReport |
false |
|
WaterUsageReport
Name |
Type |
Required |
Description |
id |
string |
true |
|
usageGallons |
number |
false |
|
avgPerSqFtGallons |
number |
false |
|
observedDays |
integer |
false |
|
monthlyWaterUsageItems |
MonthlyWaterUsageData[ ] |
false |
|
vegetationCoverageSqFt |
Object for field vegetationCoverageSqFt |
false |
|
dailyTempExtremes |
DailyTempExtreme[ ] |
false |
|
monthlyTempExtremes |
MonthlyTempExtreme[ ] |
false |
|
monthlyRainExtremes |
MontlhyRainExtreme[ ] |
false |
|
cloudCoverage |
CloudCoverageHeatMap[ ] |
false |
|
tempCoverage |
TempCoverageHeatMap[ ] |
false |
|
verbalWeather |
VerbalWeather |
false |
|
generationTime |
integer |
false |
|
MonthlyWaterUsageData
Name |
Type |
Required |
Description |
timestamp |
integer |
true |
|
rainIn |
number |
false |
|
rainGallons |
number |
false |
|
etIn |
number |
false |
|
usageGallons |
number |
false |
|
usageByRegionType |
Object for field usageByRegionType |
false |
|
Object for field usageByRegionType
Name |
Type |
Required |
Description |
* |
number |
false |
|
Object for field vegetationCoverageSqFt
Name |
Type |
Required |
Description |
* |
number |
false |
|
DailyTempExtreme
Name |
Type |
Required |
Description |
timestamp |
integer |
true |
|
minTemp |
number |
false |
|
maxTemp |
number |
false |
|
MonthlyTempExtreme
Name |
Type |
Required |
Description |
minTempTimestamp |
integer |
false |
|
minTemp |
number |
false |
|
maxTempTimestamp |
integer |
false |
|
maxTemp |
number |
false |
|
MontlhyRainExtreme
Name |
Type |
Required |
Description |
timestamp |
integer |
false |
|
rainIn |
number |
false |
|
CloudCoverageHeatMap
Name |
Type |
Required |
Description |
startTimestamp |
integer |
false |
|
endTimestamp |
integer |
false |
|
value |
number |
false |
|
TempCoverageHeatMap
Name |
Type |
Required |
Description |
startTimestamp |
integer |
false |
|
endTimestamp |
integer |
false |
|
value |
number |
false |
|
VerbalWeather
Name |
Type |
Required |
Description |
maxTempTimestamp |
integer |
false |
|
maxTemp |
number |
false |
|
maxTempMonthTempAvg |
number |
false |
|
maxTempMonthTempAvgTimestamp |
integer |
false |
|
minTempTimestamp |
integer |
false |
|
minTemp |
number |
false |
|
minTempMonthTempAvg |
number |
false |
|
minTempMonthTempAvgTimestamp |
integer |
false |
|
frzDays |
integer |
false |
|
frzSpellDays |
integer |
false |
|
frzSpellStartTimestamp |
integer |
false |
|
frzSpellEndTimestamp |
integer |
false |
|
maxRainIn |
number |
false |
|
maxRainTimestamp |
integer |
false |
|
maxTotalRainMonthIn |
number |
false |
|
maxTotalRainMonthInTimestamp |
integer |
false |
|
V1 | OIP | PDF Report
The Sites Dashboard OIP PDF Report API formats the information available through the Sites Dashboard OIP Report API into a 7-page ETwater Optimized Irrigation Plan PDF. See it in use at www.etwater.com/plan
Create OIP PDF version of report
Request
curl "https://developer-api.etwater.com/api/v1/sites-dashboard/oip/reports/{reportId}/pdf" \
-X POST \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
--data "{
\"id\": \"example string value\",
\"reportId\": \"example string value\",
\"siteName\": \"example string value\",
\"personName\": \"example string value\",
\"personJobTitle\": \"example string value\",
\"personBusinessName\": \"example string value\",
\"personEmail\": \"example string value\",
\"personPhone\": \"example string value\",
\"subscribeToNews\": false,
\"htmlReportUrl\": \"example string value\",
\"emails\": [
{
\"firstName\": \"example string value\",
\"lastName\": \"example string value\",
\"email\": \"example string value\"
}
],
\"onlineReportUrl\": \"example string value\",
\"onlinePdfReportUrl\": \"example string value\"
}"
On success, the above request returns response like
{
"status": 684,
"errorMessage": "example string value",
"report": {
"id": "example string value",
"projectId": "example string value",
"reportId": "example string value",
"reportUrl": "example string value"
}
}
HTTP Request
POST /api/v1/sites-dashboard/oip/reports/{reportId}/pdf
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
reportId |
string |
path |
true |
OIP Report Id |
oipPdfReportRequest |
OIPPdfReportRequest |
body |
true |
|
OIPPdfReportRequest
Name |
Type |
Required |
Description |
id |
string |
false |
|
reportId |
string |
true |
|
siteName |
string |
false |
|
personName |
string |
false |
|
personJobTitle |
string |
false |
|
personBusinessName |
string |
false |
|
personEmail |
string |
false |
|
personPhone |
string |
false |
|
subscribeToNews |
boolean |
false |
|
htmlReportUrl |
string |
false |
|
emails |
OIPPdfEmailInfo[ ] |
false |
|
onlineReportUrl |
string |
false |
|
onlinePdfReportUrl |
string |
false |
|
OIPPdfEmailInfo
Name |
Type |
Required |
Description |
firstName |
string |
false |
|
lastName |
string |
false |
|
email |
string |
false |
|
HTTP Responses
Code |
Description |
200 |
Successful Response |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
status |
integer |
true |
|
errorMessage |
string |
false |
|
report |
OIPPdfReport |
false |
|
OIPPdfReport
Name |
Type |
Required |
Description |
id |
string |
true |
|
projectId |
string |
true |
|
reportId |
string |
true |
|
reportUrl |
string |
false |
|
Create OIP PDF version of report
Request
curl "https://developer-api.etwater.com/api/v1/sites-dashboard/oip/reports/{reportId}/pdf/{id}" \
-X GET \
-H "Authorization: example string value" \
-H "Content-Type: example string value"
On success, the above request returns response like
{
"status": 898,
"errorMessage": "example string value",
"report": {
"id": "example string value",
"projectId": "example string value",
"reportId": "example string value",
"reportUrl": "example string value"
}
}
HTTP Request
GET /api/v1/sites-dashboard/oip/reports/{reportId}/pdf/{id}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
id |
string |
path |
true |
OIP PDF Report Id |
reportId |
string |
path |
true |
OIP Report Id |
HTTP Responses
Code |
Description |
200 |
Successful Response |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
status |
integer |
true |
|
errorMessage |
string |
false |
|
report |
OIPPdfReport |
false |
|
OIPPdfReport
Name |
Type |
Required |
Description |
id |
string |
true |
|
projectId |
string |
true |
|
reportId |
string |
true |
|
reportUrl |
string |
false |
|
V1 | OIP | Weather Summary
The Sites Dashboard Weather Summary API derives an annual aggregate of weather data dynamically computed for a particular location, including annual rain fall (total rain in the past 365 days), annual sunshine hours (total daytime hours without cloud cover in the past 365 days), and longest dry spell period (period with most consecutive days without rain in the past 365 days).
Get weather summary data for a given location
Request
curl "https://developer-api.etwater.com/api/v1/sites-dashboard/reports/weather/summary?lat=800.89490851429&lng=372.74521513504" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"weatherSummary": {
"point": {
"type": "Point",
"coordinates": [
105
],
"properties": {
"example_property_name": "example string value"
}
},
"status": 557,
"annualSunshineHours": 353,
"annualRainMm": 249,
"drySpell": {
"startDateTimestamp": 103,
"endDateTimestamp": 5,
"daysCount": 276
}
}
}
HTTP Request
GET /api/v1/sites-dashboard/reports/weather/summary
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
lat |
number |
query |
true |
latitude |
lng |
number |
query |
true |
longtitude |
HTTP Responses
Code |
Description |
200 |
Successful Response |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
weatherSummary |
WeatherSummary |
false |
|
WeatherSummary
Name |
Type |
Required |
Description |
point |
Point |
true |
|
status |
integer |
true |
|
annualSunshineHours |
number |
false |
|
annualRainMm |
number |
false |
|
drySpell |
DrySpellPeriod |
false |
|
Point
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ] |
true |
|
properties |
Object for field properties |
false |
|
Object for field properties
Name |
Type |
Required |
Description |
* |
string |
false |
|
DrySpellPeriod
Name |
Type |
Required |
Description |
startDateTimestamp |
integer |
true |
|
endDateTimestamp |
integer |
true |
|
daysCount |
integer |
true |
|
V1 | Parcels | Parcels API
The Gardening Parcels API is used to manage property parcels associated with a user. Unity API has a database of over 140 million property parcels, covering more than 96% of the U.S. population, so you'll likely find the parcel boundaries for your project. In case you don't, you can create it. Having a parcel associated with a landscape allows you to verify the address as well as know the landscape size and situation.
Get all Parcels.
Request
curl "https://developer-api.etwater.com/api/v1/gardening/projects/{projectId}/parcels" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
Dependent on:
- Authorization: Bearer {access_token}
where: {access_token} access token value.
Path parameter description
{projectId} - project id value.
Response body fields description:
- id - parcel id;
- county_id - county FIPS identifier;
- county_name - country name;
- muni_name - municipality name;
- state_abbr - state abbreviation;
- addr_number - physical/site house number;
- addr_street_prefix - physical/site street prefix;
- addr_street_name - physical/site street name;
- addr_street_suffix - physical/site street suffix;
- addr_street_type - physical/site Street Type;
- physcity - physical/site city;
- physzip - physical/site zip code;
- census_zip - census zip code;
- owner - owner name;
- mail_name - mailing name;
- mail_address1 - house number street name street type or PO Box
- mail_address2 - suite number, building number, or other mailing information;
- mail_address3 - city, state, and zip;
- trans_date - most recent transfer (Sale) date;
- sale_price - sale price;
- mkt_val_land - land market value;
- mkt_val_bldg - improvement market value;
- mkt_val_tot - total market value;
- bldg_sqft - building / home area in square feet;
- ngh_code - neighborhood code;
- land_use_code - land use code;
- land_use_class - derived land use class ('Residential', 'Agricultural', 'Commercial', 'Tax Exempt', 'Industrial', or 'Mineral');
- story_height - story height;
- muni_id - census municipality id number;
- school_distId - census school district id number;
- acreage_deeded - deeded acreage from source;
- acreage_calc - acreage calculated from area of geometry;
- geom_as_wkt - (MULTI)POLYGON geometry as an OGC Well-Known-Text (WKT) string;
- status - status of parcel, can be: 0 (ACTIVE), 1 (DELETED);
- user_id - id of user, who created this parcel;
- external_id - external id;
- project_id - project id;
- is_custom - is customer flag;
HTTP Request
GET /api/v1/gardening/projects/{projectId}/parcels
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
projectId |
string |
path |
true |
Project id |
HTTP Responses
Code |
Description |
200 |
Successful Response |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response is array of
Create a Parcel.
Request
curl "https://developer-api.etwater.com/api/v1/gardening/projects/{projectId}/parcels" \
-X POST \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
--data "{
\"id\": \"example string value\",
\"county_id\": \"example string value\",
\"county_name\": \"example string value\",
\"muni_name\": \"example string value\",
\"state_abbr\": \"example string value\",
\"addr_number\": \"example string value\",
\"addr_street_prefix\": \"example string value\",
\"addr_street_name\": \"example string value\",
\"addr_street_suffix\": \"example string value\",
\"addr_street_type\": \"example string value\",
\"physcity\": \"example string value\",
\"physzip\": \"example string value\",
\"census_zip\": \"example string value\",
\"owner\": \"example string value\",
\"mail_name\": \"example string value\",
\"mail_address1\": \"example string value\",
\"mail_address2\": \"example string value\",
\"mail_address3\": \"example string value\",
\"trans_date\": \"example string value\",
\"sale_price\": 592,
\"mkt_val_land\": \"example string value\",
\"mkt_val_bldg\": \"example string value\",
\"mkt_val_tot\": 847.2128235023529,
\"bldg_sqft\": 941,
\"ngh_code\": \"example string value\",
\"land_use_code\": \"example string value\",
\"land_use_class\": \"example string value\",
\"story_height\": \"example string value\",
\"muni_id\": \"example string value\",
\"school_distId\": \"example string value\",
\"acreage_deeded\": \"example string value\",
\"acreage_calc\": 925,
\"geom_as_wkt\": \"example string value\",
\"external_id\": \"example string value\",
\"project_id\": \"example string value\",
\"is_custom\": true,
\"centerCoordinates\": [
854
],
\"landscapeId\": \"example string value\",
\"areaM2\": 115.44460296418733,
\"version\": \"-1\"
}"
On success, the above request returns response like
Dependent on:
- Authorization: Bearer {access_token}
- Content-Type: application/json
where: {access_token} access token value.
Path parameter description
{projectId} - project id value.
Request body fields description:
The same as for response body.
Response body fields description:
- id - parcel id;
- county_id - county FIPS identifier;
- county_name - country name;
- muni_name - municipality name;
- state_abbr - state abbreviation;
- addr_number - physical/site house number;
- addr_street_prefix - physical/site street prefix;
- addr_street_name - physical/site street name;
- addr_street_suffix - physical/site street suffix;
- addr_street_type - physical/site Street Type;
- physcity - physical/site city;
- physzip - physical/site zip code;
- census_zip - census zip code;
- owner - owner name;
- mail_name - mailing name;
- mail_address1 - house number street name street type or PO Box
- mail_address2 - suite number, building number, or other mailing information;
- mail_address3 - city, state, and zip;
- trans_date - most recent transfer (Sale) date;
- sale_price - sale price;
- mkt_val_land - land market value;
- mkt_val_bldg - improvement market value;
- mkt_val_tot - total market value;
- bldg_sqft - building / home area in square feet;
- ngh_code - neighborhood code;
- land_use_code - land use code;
- land_use_class - derived land use class ('Residential', 'Agricultural', 'Commercial', 'Tax Exempt', 'Industrial', or 'Mineral');
- story_height - story height;
- muni_id - census municipality id number;
- school_distId - census school district id number;
- acreage_deeded - deeded acreage from source;
- acreage_calc - acreage calculated from area of geometry;
- geom_as_wkt - (MULTI)POLYGON geometry as an OGC Well-Known-Text (WKT) string;
- status - status of parcel, can be: 0 (ACTIVE), 1 (DELETED);
- user_id - id of user, who created this parcel;
- external_id - external id;
- project_id - project id;
- is_custom - is customer flag;
HTTP Request
POST /api/v1/gardening/projects/{projectId}/parcels
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
projectId |
string |
path |
true |
Project id |
parcel |
GardeningParcel |
body |
true |
|
GardeningParcel
Name |
Type |
Required |
Description |
id |
string |
false |
|
county_id |
string |
false |
|
county_name |
string |
false |
|
muni_name |
string |
false |
|
state_abbr |
string |
false |
|
addr_number |
string |
false |
|
addr_street_prefix |
string |
false |
|
addr_street_name |
string |
false |
|
addr_street_suffix |
string |
false |
|
addr_street_type |
string |
false |
|
physcity |
string |
false |
|
physzip |
string |
false |
|
census_zip |
string |
false |
|
owner |
string |
false |
|
mail_name |
string |
false |
|
mail_address1 |
string |
false |
|
mail_address2 |
string |
false |
|
mail_address3 |
string |
false |
|
trans_date |
string |
false |
|
sale_price |
number |
false |
|
mkt_val_land |
string |
false |
|
mkt_val_bldg |
string |
false |
|
mkt_val_tot |
number |
false |
|
bldg_sqft |
integer |
false |
|
ngh_code |
string |
false |
|
land_use_code |
string |
false |
|
land_use_class |
string |
false |
|
story_height |
string |
false |
|
muni_id |
string |
false |
|
school_distId |
string |
false |
|
acreage_deeded |
string |
false |
|
acreage_calc |
number |
false |
|
geom_as_wkt |
string |
false |
|
external_id |
string |
false |
|
project_id |
string |
false |
|
is_custom |
boolean |
false |
|
centerCoordinates |
number[ ] |
false |
|
landscapeId |
string |
false |
|
areaM2 |
number |
false |
|
version |
integer |
false |
|
HTTP Responses
Code |
Description |
201 |
Successful Response |
404 |
Error Response |
409 |
Error Response |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
false |
|
county_id |
string |
false |
|
county_name |
string |
false |
|
muni_name |
string |
false |
|
state_abbr |
string |
false |
|
addr_number |
string |
false |
|
addr_street_prefix |
string |
false |
|
addr_street_name |
string |
false |
|
addr_street_suffix |
string |
false |
|
addr_street_type |
string |
false |
|
physcity |
string |
false |
|
physzip |
string |
false |
|
census_zip |
string |
false |
|
owner |
string |
false |
|
mail_name |
string |
false |
|
mail_address1 |
string |
false |
|
mail_address2 |
string |
false |
|
mail_address3 |
string |
false |
|
trans_date |
string |
false |
|
sale_price |
number |
false |
|
mkt_val_land |
string |
false |
|
mkt_val_bldg |
string |
false |
|
mkt_val_tot |
number |
false |
|
bldg_sqft |
integer |
false |
|
ngh_code |
string |
false |
|
land_use_code |
string |
false |
|
land_use_class |
string |
false |
|
story_height |
string |
false |
|
muni_id |
string |
false |
|
school_distId |
string |
false |
|
acreage_deeded |
string |
false |
|
acreage_calc |
number |
false |
|
geom_as_wkt |
string |
false |
|
external_id |
string |
false |
|
project_id |
string |
false |
|
is_custom |
boolean |
false |
|
centerCoordinates |
number[ ] |
false |
|
landscapeId |
string |
false |
|
areaM2 |
number |
false |
|
version |
integer |
false |
|
Delete all parcels for project
Request
curl "https://developer-api.etwater.com/api/v1/gardening/projects/{projectId}/parcels" \
-X DELETE \
-H "Authorization: example string value"
On success, the above request returns response like
HTTP Request
DELETE /api/v1/gardening/projects/{projectId}/parcels
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
projectId |
string |
path |
true |
Project id |
HTTP Responses
Code |
Description |
204 |
Successful Response |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Get Parcel by id
Request
curl "https://developer-api.etwater.com/api/v1/gardening/projects/{projectId}/parcels/{parcelId}" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"id": "example string value",
"county_id": "example string value",
"county_name": "example string value",
"muni_name": "example string value",
"state_abbr": "example string value",
"addr_number": "example string value",
"addr_street_prefix": "example string value",
"addr_street_name": "example string value",
"addr_street_suffix": "example string value",
"addr_street_type": "example string value",
"physcity": "example string value",
"physzip": "example string value",
"census_zip": "example string value",
"owner": "example string value",
"mail_name": "example string value",
"mail_address1": "example string value",
"mail_address2": "example string value",
"mail_address3": "example string value",
"trans_date": "example string value",
"sale_price": 663,
"mkt_val_land": "example string value",
"mkt_val_bldg": "example string value",
"mkt_val_tot": 932,
"bldg_sqft": 825,
"ngh_code": "example string value",
"land_use_code": "example string value",
"land_use_class": "example string value",
"story_height": "example string value",
"muni_id": "example string value",
"school_distId": "example string value",
"acreage_deeded": "example string value",
"acreage_calc": 84,
"geom_as_wkt": "example string value",
"external_id": "example string value",
"project_id": "example string value",
"is_custom": true,
"centerCoordinates": [
392
],
"landscapeId": "example string value",
"areaM2": 215,
"version": "-1"
}
Dependent on:
- Authorization: Bearer {access_token}
where: {access_token} access token value.
Path parameter description
- {projectId} - project id value.
- {parcelId} - id of parcel.
Response body fields description:
- id - parcel id;
- county_id - county FIPS identifier;
- county_name - country name;
- muni_name - municipality name;
- state_abbr - state abbreviation;
- addr_number - physical/site house number;
- addr_street_prefix - physical/site street prefix;
- addr_street_name - physical/site street name;
- addr_street_suffix - physical/site street suffix;
- addr_street_type - physical/site Street Type;
- physcity - physical/site city;
- physzip - physical/site zip code;
- census_zip - census zip code;
- owner - owner name;
- mail_name - mailing name;
- mail_address1 - house number street name street type or PO Box
- mail_address2 - suite number, building number, or other mailing information;
- mail_address3 - city, state, and zip;
- trans_date - most recent transfer (Sale) date;
- sale_price - sale price;
- mkt_val_land - land market value;
- mkt_val_bldg - improvement market value;
- mkt_val_tot - total market value;
- bldg_sqft - building / home area in square feet;
- ngh_code - neighborhood code;
- land_use_code - land use code;
- land_use_class - derived land use class ('Residential', 'Agricultural', 'Commercial', 'Tax Exempt', 'Industrial', or 'Mineral');
- story_height - story height;
- muni_id - census municipality id number;
- school_distId - census school district id number;
- acreage_deeded - deeded acreage from source;
- acreage_calc - acreage calculated from area of geometry;
- geom_as_wkt - (MULTI)POLYGON geometry as an OGC Well-Known-Text (WKT) string;
- status - status of parcel, can be: 0 (ACTIVE), 1 (DELETED);
- user_id - id of user, who created this parcel;
- external_id - external id;
- project_id - project id;
- is_custom - is customer flag;
HTTP Request
GET /api/v1/gardening/projects/{projectId}/parcels/{parcelId}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
projectId |
string |
path |
true |
Project id |
parcelId |
string |
path |
true |
Parcel id |
HTTP Responses
Code |
Description |
200 |
Successful Response |
404 |
Error Response |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
false |
|
county_id |
string |
false |
|
county_name |
string |
false |
|
muni_name |
string |
false |
|
state_abbr |
string |
false |
|
addr_number |
string |
false |
|
addr_street_prefix |
string |
false |
|
addr_street_name |
string |
false |
|
addr_street_suffix |
string |
false |
|
addr_street_type |
string |
false |
|
physcity |
string |
false |
|
physzip |
string |
false |
|
census_zip |
string |
false |
|
owner |
string |
false |
|
mail_name |
string |
false |
|
mail_address1 |
string |
false |
|
mail_address2 |
string |
false |
|
mail_address3 |
string |
false |
|
trans_date |
string |
false |
|
sale_price |
number |
false |
|
mkt_val_land |
string |
false |
|
mkt_val_bldg |
string |
false |
|
mkt_val_tot |
number |
false |
|
bldg_sqft |
integer |
false |
|
ngh_code |
string |
false |
|
land_use_code |
string |
false |
|
land_use_class |
string |
false |
|
story_height |
string |
false |
|
muni_id |
string |
false |
|
school_distId |
string |
false |
|
acreage_deeded |
string |
false |
|
acreage_calc |
number |
false |
|
geom_as_wkt |
string |
false |
|
external_id |
string |
false |
|
project_id |
string |
false |
|
is_custom |
boolean |
false |
|
centerCoordinates |
number[ ] |
false |
|
landscapeId |
string |
false |
|
areaM2 |
number |
false |
|
version |
integer |
false |
|
Delete Parcel by id.
Request
curl "https://developer-api.etwater.com/api/v1/gardening/projects/{projectId}/parcels/{parcelId}" \
-X DELETE \
-H "Authorization: example string value"
On success, the above request returns response like
Dependent on:
- Authorization: Bearer {access_token}
where: {access_token} access token value.
Path parameter description
- {projectId} - project id value.
- {parcelId} - id of parcel.
HTTP Request
DELETE /api/v1/gardening/projects/{projectId}/parcels/{parcelId}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
projectId |
string |
path |
true |
Project id |
parcelId |
string |
path |
true |
id of Parcel |
HTTP Responses
Code |
Description |
204 |
Successful Response |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Update Parcel by id.
Request
curl "https://developer-api.etwater.com/api/v1/gardening/projects/{projectId}/parcels/{parcelId}" \
-X PUT \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
--data "{
\"id\": \"example string value\",
\"county_id\": \"example string value\",
\"county_name\": \"example string value\",
\"muni_name\": \"example string value\",
\"state_abbr\": \"example string value\",
\"addr_number\": \"example string value\",
\"addr_street_prefix\": \"example string value\",
\"addr_street_name\": \"example string value\",
\"addr_street_suffix\": \"example string value\",
\"addr_street_type\": \"example string value\",
\"physcity\": \"example string value\",
\"physzip\": \"example string value\",
\"census_zip\": \"example string value\",
\"owner\": \"example string value\",
\"mail_name\": \"example string value\",
\"mail_address1\": \"example string value\",
\"mail_address2\": \"example string value\",
\"mail_address3\": \"example string value\",
\"trans_date\": \"example string value\",
\"sale_price\": 158,
\"mkt_val_land\": \"example string value\",
\"mkt_val_bldg\": \"example string value\",
\"mkt_val_tot\": 202.87670577078904,
\"bldg_sqft\": 310,
\"ngh_code\": \"example string value\",
\"land_use_code\": \"example string value\",
\"land_use_class\": \"example string value\",
\"story_height\": \"example string value\",
\"muni_id\": \"example string value\",
\"school_distId\": \"example string value\",
\"acreage_deeded\": \"example string value\",
\"acreage_calc\": 471.49637037492187,
\"geom_as_wkt\": \"example string value\",
\"external_id\": \"example string value\",
\"project_id\": \"example string value\",
\"is_custom\": false,
\"centerCoordinates\": [
378
],
\"landscapeId\": \"example string value\",
\"areaM2\": 509,
\"version\": \"-1\"
}"
On success, the above request returns response like
{
"id": "example string value",
"county_id": "example string value",
"county_name": "example string value",
"muni_name": "example string value",
"state_abbr": "example string value",
"addr_number": "example string value",
"addr_street_prefix": "example string value",
"addr_street_name": "example string value",
"addr_street_suffix": "example string value",
"addr_street_type": "example string value",
"physcity": "example string value",
"physzip": "example string value",
"census_zip": "example string value",
"owner": "example string value",
"mail_name": "example string value",
"mail_address1": "example string value",
"mail_address2": "example string value",
"mail_address3": "example string value",
"trans_date": "example string value",
"sale_price": 94,
"mkt_val_land": "example string value",
"mkt_val_bldg": "example string value",
"mkt_val_tot": 847,
"bldg_sqft": 853,
"ngh_code": "example string value",
"land_use_code": "example string value",
"land_use_class": "example string value",
"story_height": "example string value",
"muni_id": "example string value",
"school_distId": "example string value",
"acreage_deeded": "example string value",
"acreage_calc": 634.1000020662788,
"geom_as_wkt": "example string value",
"external_id": "example string value",
"project_id": "example string value",
"is_custom": false,
"centerCoordinates": [
261
],
"landscapeId": "example string value",
"areaM2": 670,
"version": "-1"
}
Dependent on:
- Authorization: Bearer {access_token}
- Content-Type: application/json
where: {access_token} access token value.
Path parameter description
- {projectId} - project id value.
- {parcelId} - id of parcel.
Request body fields description:
The same as for response body.
Response body fields description:
- id - parcel id;
- county_id - county FIPS identifier;
- county_name - country name;
- muni_name - municipality name;
- state_abbr - state abbreviation;
- addr_number - physical/site house number;
- addr_street_prefix - physical/site street prefix;
- addr_street_name - physical/site street name;
- addr_street_suffix - physical/site street suffix;
- addr_street_type - physical/site Street Type;
- physcity - physical/site city;
- physzip - physical/site zip code;
- census_zip - census zip code;
- owner - owner name;
- mail_name - mailing name;
- mail_address1 - house number street name street type or PO Box
- mail_address2 - suite number, building number, or other mailing information;
- mail_address3 - city, state, and zip;
- trans_date - most recent transfer (Sale) date;
- sale_price - sale price;
- mkt_val_land - land market value;
- mkt_val_bldg - improvement market value;
- mkt_val_tot - total market value;
- bldg_sqft - building / home area in square feet;
- ngh_code - neighborhood code;
- land_use_code - land use code;
- land_use_class - derived land use class ('Residential', 'Agricultural', 'Commercial', 'Tax Exempt', 'Industrial', or 'Mineral');
- story_height - story height;
- muni_id - census municipality id number;
- school_distId - census school district id number;
- acreage_deeded - deeded acreage from source;
- acreage_calc - acreage calculated from area of geometry;
- geom_as_wkt - (MULTI)POLYGON geometry as an OGC Well-Known-Text (WKT) string;
- status - status of parcel, can be: 0 (ACTIVE), 1 (DELETED);
- user_id - id of user, who created this parcel;
- external_id - external id;
- project_id - project id;
- is_custom - is customer flag;
HTTP Request
PUT /api/v1/gardening/projects/{projectId}/parcels/{parcelId}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
projectId |
string |
path |
true |
Project id |
parcelId |
string |
path |
true |
id of Parcel |
parcel |
GardeningParcel |
body |
true |
|
GardeningParcel
Name |
Type |
Required |
Description |
id |
string |
false |
|
county_id |
string |
false |
|
county_name |
string |
false |
|
muni_name |
string |
false |
|
state_abbr |
string |
false |
|
addr_number |
string |
false |
|
addr_street_prefix |
string |
false |
|
addr_street_name |
string |
false |
|
addr_street_suffix |
string |
false |
|
addr_street_type |
string |
false |
|
physcity |
string |
false |
|
physzip |
string |
false |
|
census_zip |
string |
false |
|
owner |
string |
false |
|
mail_name |
string |
false |
|
mail_address1 |
string |
false |
|
mail_address2 |
string |
false |
|
mail_address3 |
string |
false |
|
trans_date |
string |
false |
|
sale_price |
number |
false |
|
mkt_val_land |
string |
false |
|
mkt_val_bldg |
string |
false |
|
mkt_val_tot |
number |
false |
|
bldg_sqft |
integer |
false |
|
ngh_code |
string |
false |
|
land_use_code |
string |
false |
|
land_use_class |
string |
false |
|
story_height |
string |
false |
|
muni_id |
string |
false |
|
school_distId |
string |
false |
|
acreage_deeded |
string |
false |
|
acreage_calc |
number |
false |
|
geom_as_wkt |
string |
false |
|
external_id |
string |
false |
|
project_id |
string |
false |
|
is_custom |
boolean |
false |
|
centerCoordinates |
number[ ] |
false |
|
landscapeId |
string |
false |
|
areaM2 |
number |
false |
|
version |
integer |
false |
|
HTTP Responses
Code |
Description |
200 |
Successful Response |
404 |
Error Response |
409 |
Error Response |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
false |
|
county_id |
string |
false |
|
county_name |
string |
false |
|
muni_name |
string |
false |
|
state_abbr |
string |
false |
|
addr_number |
string |
false |
|
addr_street_prefix |
string |
false |
|
addr_street_name |
string |
false |
|
addr_street_suffix |
string |
false |
|
addr_street_type |
string |
false |
|
physcity |
string |
false |
|
physzip |
string |
false |
|
census_zip |
string |
false |
|
owner |
string |
false |
|
mail_name |
string |
false |
|
mail_address1 |
string |
false |
|
mail_address2 |
string |
false |
|
mail_address3 |
string |
false |
|
trans_date |
string |
false |
|
sale_price |
number |
false |
|
mkt_val_land |
string |
false |
|
mkt_val_bldg |
string |
false |
|
mkt_val_tot |
number |
false |
|
bldg_sqft |
integer |
false |
|
ngh_code |
string |
false |
|
land_use_code |
string |
false |
|
land_use_class |
string |
false |
|
story_height |
string |
false |
|
muni_id |
string |
false |
|
school_distId |
string |
false |
|
acreage_deeded |
string |
false |
|
acreage_calc |
number |
false |
|
geom_as_wkt |
string |
false |
|
external_id |
string |
false |
|
project_id |
string |
false |
|
is_custom |
boolean |
false |
|
centerCoordinates |
number[ ] |
false |
|
landscapeId |
string |
false |
|
areaM2 |
number |
false |
|
version |
integer |
false |
|
V1 | Projects | Projects
API is dedicated for managing Projects. Project is usually one physical location with one or several CCU installed and binds together all the vegetation item being irrigated.
Get all projects
Request
curl "https://developer-api.etwater.com/api/v1/gardening/projects?page=0&size=10&unity=0" \
-X GET \
-H "Authorization: example string value" \
-H "Unity-Api-Gateway-Replace-UserId: example string value"
On success, the above request returns response like
HTTP Request
GET /api/v1/gardening/projects
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
OAuth2 authorization header. Should be in the following format: Authorization: Bearer $TOKEN |
page |
integer |
query |
false |
|
size |
integer |
query |
false |
|
unity |
boolean |
query |
false |
|
Unity-Api-Gateway-Replace-UserId |
string |
header |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response is array of
Create Project action.
Request
curl "https://developer-api.etwater.com/api/v1/gardening/projects?version=771" \
-X POST \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
-H "Unity-Api-Gateway-Replace-UserId: example string value" \
--data "{
\"id\": \"example string value\",
\"name\": \"example string value\",
\"user_id\": \"example string value\",
\"address\": \"example string value\",
\"location\": {
\"type\": \"Point\",
\"coordinates\": [
316.81427514032197
],
\"properties\": {
\"example_property_name\": \"example string value\"
}
},
\"created_at\": 888,
\"updated_at\": 856,
\"version\": \"-1\",
\"postal_code\": \"example string value\",
\"billingAddress\": {
\"firstName\": \"example string value\",
\"lastName\": \"example string value\",
\"company\": \"example string value\",
\"address1\": \"example string value\",
\"address2\": \"example string value\",
\"city\": \"example string value\",
\"state\": \"example string value\",
\"postcode\": \"example string value\",
\"country\": \"example string value\",
\"email\": \"example string value\",
\"phone\": \"example string value\"
},
\"projectAddress\": {
\"address1\": \"example string value\",
\"address2\": \"example string value\",
\"city\": \"example string value\",
\"state\": \"example string value\",
\"postcode\": \"example string value\",
\"country\": \"example string value\"
},
\"unity\": true
}"
On success, the above request returns response like
HTTP Request
POST /api/v1/gardening/projects
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
Unity-Api-Gateway-Replace-UserId |
string |
header |
false |
|
Project |
GardeningProject |
body |
true |
|
version |
integer |
query |
false |
version of Project |
GardeningProject
Name |
Type |
Required |
Description |
id |
string |
false |
A unique string that can be used to identify and reference a specific project. |
name |
string |
false |
A human-readable name for the project. |
user_id |
string |
false |
|
address |
string |
false |
A human-readable name for the project. |
location |
Point |
false |
Location information |
created_at |
integer |
false |
Unix timestamp of a project creation |
updated_at |
integer |
false |
Unix timestamp of a project last update |
version |
integer |
false |
Version of a project |
postal_code |
string |
false |
Project’s postal code. |
billingAddress |
BillingAddress |
false |
|
projectAddress |
ProjectAddress |
false |
|
unity |
boolean |
false |
|
Point
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ] |
true |
|
properties |
Object for field properties |
false |
|
Object for field properties
Name |
Type |
Required |
Description |
* |
string |
false |
|
BillingAddress
Name |
Type |
Required |
Description |
firstName |
string |
false |
|
lastName |
string |
false |
|
company |
string |
false |
|
address1 |
string |
false |
|
address2 |
string |
false |
|
city |
string |
false |
|
state |
string |
false |
|
postcode |
string |
false |
|
country |
string |
false |
|
email |
string |
false |
|
phone |
string |
false |
|
ProjectAddress
Name |
Type |
Required |
Description |
address1 |
string |
false |
|
address2 |
string |
false |
|
city |
string |
false |
|
state |
string |
false |
|
postcode |
string |
false |
|
country |
string |
false |
|
HTTP Responses
Code |
Description |
201 |
Created |
409 |
Conflict |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
false |
A unique string that can be used to identify and reference a specific project. |
name |
string |
false |
A human-readable name for the project. |
user_id |
string |
false |
|
address |
string |
false |
A human-readable name for the project. |
location |
Point |
false |
Location information |
created_at |
integer |
false |
Unix timestamp of a project creation |
updated_at |
integer |
false |
Unix timestamp of a project last update |
version |
integer |
false |
Version of a project |
postal_code |
string |
false |
Project’s postal code. |
billingAddress |
BillingAddress |
false |
|
projectAddress |
ProjectAddress |
false |
|
unity |
boolean |
false |
|
Point
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ] |
true |
|
properties |
Object for field properties |
false |
|
Object for field properties
Name |
Type |
Required |
Description |
* |
string |
false |
|
BillingAddress
Name |
Type |
Required |
Description |
firstName |
string |
false |
|
lastName |
string |
false |
|
company |
string |
false |
|
address1 |
string |
false |
|
address2 |
string |
false |
|
city |
string |
false |
|
state |
string |
false |
|
postcode |
string |
false |
|
country |
string |
false |
|
email |
string |
false |
|
phone |
string |
false |
|
ProjectAddress
Name |
Type |
Required |
Description |
address1 |
string |
false |
|
address2 |
string |
false |
|
city |
string |
false |
|
state |
string |
false |
|
postcode |
string |
false |
|
country |
string |
false |
|
Create or update projects
Request
curl "https://developer-api.etwater.com/api/v1/gardening/projects" \
-X PATCH \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
--data "[
{
\"id\": \"example string value\",
\"name\": \"example string value\",
\"user_id\": \"example string value\",
\"address\": \"example string value\",
\"location\": {
\"type\": \"Point\",
\"coordinates\": [
414.0013225441805
],
\"properties\": {
\"example_property_name\": \"example string value\"
}
},
\"created_at\": 337,
\"updated_at\": 970,
\"version\": \"-1\",
\"postal_code\": \"example string value\",
\"billingAddress\": {
\"firstName\": \"example string value\",
\"lastName\": \"example string value\",
\"company\": \"example string value\",
\"address1\": \"example string value\",
\"address2\": \"example string value\",
\"city\": \"example string value\",
\"state\": \"example string value\",
\"postcode\": \"example string value\",
\"country\": \"example string value\",
\"email\": \"example string value\",
\"phone\": \"example string value\"
},
\"projectAddress\": {
\"address1\": \"example string value\",
\"address2\": \"example string value\",
\"city\": \"example string value\",
\"state\": \"example string value\",
\"postcode\": \"example string value\",
\"country\": \"example string value\"
},
\"unity\": false
}
]"
On success, the above request returns response like
[
{
"id": "example string value",
"name": "example string value",
"user_id": "example string value",
"address": "example string value",
"location": {
"type": "Point",
"coordinates": [
605
],
"properties": {
"example_property_name": "example string value"
}
},
"created_at": 6,
"updated_at": 260,
"version": "-1",
"postal_code": "example string value",
"billingAddress": {
"firstName": "example string value",
"lastName": "example string value",
"company": "example string value",
"address1": "example string value",
"address2": "example string value",
"city": "example string value",
"state": "example string value",
"postcode": "example string value",
"country": "example string value",
"email": "example string value",
"phone": "example string value"
},
"projectAddress": {
"address1": "example string value",
"address2": "example string value",
"city": "example string value",
"state": "example string value",
"postcode": "example string value",
"country": "example string value"
},
"unity": false
}
]
HTTP Request
PATCH /api/v1/gardening/projects
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
Projects |
GardeningProject[ ] |
body |
true |
|
GardeningProject
Name |
Type |
Required |
Description |
id |
string |
false |
A unique string that can be used to identify and reference a specific project. |
name |
string |
false |
A human-readable name for the project. |
user_id |
string |
false |
|
address |
string |
false |
A human-readable name for the project. |
location |
Point |
false |
Location information |
created_at |
integer |
false |
Unix timestamp of a project creation |
updated_at |
integer |
false |
Unix timestamp of a project last update |
version |
integer |
false |
Version of a project |
postal_code |
string |
false |
Project’s postal code. |
billingAddress |
BillingAddress |
false |
|
projectAddress |
ProjectAddress |
false |
|
unity |
boolean |
false |
|
Point
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ] |
true |
|
properties |
Object for field properties |
false |
|
Object for field properties
Name |
Type |
Required |
Description |
* |
string |
false |
|
BillingAddress
Name |
Type |
Required |
Description |
firstName |
string |
false |
|
lastName |
string |
false |
|
company |
string |
false |
|
address1 |
string |
false |
|
address2 |
string |
false |
|
city |
string |
false |
|
state |
string |
false |
|
postcode |
string |
false |
|
country |
string |
false |
|
email |
string |
false |
|
phone |
string |
false |
|
ProjectAddress
Name |
Type |
Required |
Description |
address1 |
string |
false |
|
address2 |
string |
false |
|
city |
string |
false |
|
state |
string |
false |
|
postcode |
string |
false |
|
country |
string |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
404 |
Not Found |
409 |
Conflict |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response is array of
Name |
Type |
Required |
Description |
id |
string |
false |
A unique string that can be used to identify and reference a specific project. |
name |
string |
false |
A human-readable name for the project. |
user_id |
string |
false |
|
address |
string |
false |
A human-readable name for the project. |
location |
Point |
false |
Location information |
created_at |
integer |
false |
Unix timestamp of a project creation |
updated_at |
integer |
false |
Unix timestamp of a project last update |
version |
integer |
false |
Version of a project |
postal_code |
string |
false |
Project’s postal code. |
billingAddress |
BillingAddress |
false |
|
projectAddress |
ProjectAddress |
false |
|
unity |
boolean |
false |
|
Point
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ] |
true |
|
properties |
Object for field properties |
false |
|
Object for field properties
Name |
Type |
Required |
Description |
* |
string |
false |
|
BillingAddress
Name |
Type |
Required |
Description |
firstName |
string |
false |
|
lastName |
string |
false |
|
company |
string |
false |
|
address1 |
string |
false |
|
address2 |
string |
false |
|
city |
string |
false |
|
state |
string |
false |
|
postcode |
string |
false |
|
country |
string |
false |
|
email |
string |
false |
|
phone |
string |
false |
|
ProjectAddress
Name |
Type |
Required |
Description |
address1 |
string |
false |
|
address2 |
string |
false |
|
city |
string |
false |
|
state |
string |
false |
|
postcode |
string |
false |
|
country |
string |
false |
|
Search project by term
Request
curl "https://developer-api.etwater.com/api/v1/gardening/projects/search?page=0&size=10&unity=1&searchTerm=example+string+value" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
[
{
"projects": [
{
"id": "example string value",
"name": "example string value",
"user_id": "example string value",
"address": "example string value",
"location": {
"type": "Point",
"coordinates": [
735
],
"properties": {
"example_property_name": "example string value"
}
},
"created_at": 604,
"updated_at": 743,
"version": "-1",
"postal_code": "example string value",
"billingAddress": {
"firstName": "example string value",
"lastName": "example string value",
"company": "example string value",
"address1": "example string value",
"address2": "example string value",
"city": "example string value",
"state": "example string value",
"postcode": "example string value",
"country": "example string value",
"email": "example string value",
"phone": "example string value"
},
"projectAddress": {
"address1": "example string value",
"address2": "example string value",
"city": "example string value",
"state": "example string value",
"postcode": "example string value",
"country": "example string value"
},
"unity": false
}
],
"page": 569,
"size": 409,
"total": 597
}
]
HTTP Request
GET /api/v1/gardening/projects/search
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
page |
integer |
query |
false |
|
size |
integer |
query |
false |
|
unity |
boolean |
query |
false |
|
searchTerm |
string |
query |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response is array of
Name |
Type |
Required |
Description |
projects |
Project[ ] |
false |
|
page |
integer |
false |
|
size |
integer |
false |
|
total |
integer |
false |
|
Project
Name |
Type |
Required |
Description |
id |
string |
false |
A unique string that can be used to identify and reference a specific project. |
name |
string |
false |
A human-readable name for the project. |
user_id |
string |
false |
|
address |
string |
false |
A human-readable name for the project. |
location |
Point |
false |
Location information |
created_at |
integer |
false |
Unix timestamp of a project creation |
updated_at |
integer |
false |
Unix timestamp of a project last update |
version |
integer |
false |
Version of a project |
postal_code |
string |
false |
Project’s postal code. |
billingAddress |
BillingAddress |
false |
|
projectAddress |
ProjectAddress |
false |
|
unity |
boolean |
false |
|
Point
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ] |
true |
|
properties |
Object for field properties |
false |
|
Object for field properties
Name |
Type |
Required |
Description |
* |
string |
false |
|
BillingAddress
Name |
Type |
Required |
Description |
firstName |
string |
false |
|
lastName |
string |
false |
|
company |
string |
false |
|
address1 |
string |
false |
|
address2 |
string |
false |
|
city |
string |
false |
|
state |
string |
false |
|
postcode |
string |
false |
|
country |
string |
false |
|
email |
string |
false |
|
phone |
string |
false |
|
ProjectAddress
Name |
Type |
Required |
Description |
address1 |
string |
false |
|
address2 |
string |
false |
|
city |
string |
false |
|
state |
string |
false |
|
postcode |
string |
false |
|
country |
string |
false |
|
Get Project by it's id.
Request
curl "https://developer-api.etwater.com/api/v1/gardening/projects/{projectId}" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"id": "example string value",
"name": "example string value",
"user_id": "example string value",
"address": "example string value",
"location": {
"type": "Point",
"coordinates": [
976
],
"properties": {
"example_property_name": "example string value"
}
},
"created_at": 471,
"updated_at": 900,
"version": "-1",
"postal_code": "example string value",
"billingAddress": {
"firstName": "example string value",
"lastName": "example string value",
"company": "example string value",
"address1": "example string value",
"address2": "example string value",
"city": "example string value",
"state": "example string value",
"postcode": "example string value",
"country": "example string value",
"email": "example string value",
"phone": "example string value"
},
"projectAddress": {
"address1": "example string value",
"address2": "example string value",
"city": "example string value",
"state": "example string value",
"postcode": "example string value",
"country": "example string value"
},
"unity": true
}
HTTP Request
GET /api/v1/gardening/projects/{projectId}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
projectId |
string |
path |
true |
Project id |
HTTP Responses
Code |
Description |
200 |
OK |
404 |
Not Found |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
false |
A unique string that can be used to identify and reference a specific project. |
name |
string |
false |
A human-readable name for the project. |
user_id |
string |
false |
|
address |
string |
false |
A human-readable name for the project. |
location |
Point |
false |
Location information |
created_at |
integer |
false |
Unix timestamp of a project creation |
updated_at |
integer |
false |
Unix timestamp of a project last update |
version |
integer |
false |
Version of a project |
postal_code |
string |
false |
Project’s postal code. |
billingAddress |
BillingAddress |
false |
|
projectAddress |
ProjectAddress |
false |
|
unity |
boolean |
false |
|
Point
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ] |
true |
|
properties |
Object for field properties |
false |
|
Object for field properties
Name |
Type |
Required |
Description |
* |
string |
false |
|
BillingAddress
Name |
Type |
Required |
Description |
firstName |
string |
false |
|
lastName |
string |
false |
|
company |
string |
false |
|
address1 |
string |
false |
|
address2 |
string |
false |
|
city |
string |
false |
|
state |
string |
false |
|
postcode |
string |
false |
|
country |
string |
false |
|
email |
string |
false |
|
phone |
string |
false |
|
ProjectAddress
Name |
Type |
Required |
Description |
address1 |
string |
false |
|
address2 |
string |
false |
|
city |
string |
false |
|
state |
string |
false |
|
postcode |
string |
false |
|
country |
string |
false |
|
Delete Project by it's id.
Request
curl "https://developer-api.etwater.com/api/v1/gardening/projects/{projectId}?version=103" \
-X DELETE \
-H "Authorization: example string value" \
-H "Unity-Api-Gateway-Replace-UserId: example string value"
On success, the above request returns response like
HTTP Request
DELETE /api/v1/gardening/projects/{projectId}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Unity-Api-Gateway-Replace-UserId |
string |
header |
false |
|
projectId |
string |
path |
true |
id of Project |
version |
integer |
query |
false |
version of Project |
HTTP Responses
Code |
Description |
204 |
No Content |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Update Project by it's id.
Request
curl "https://developer-api.etwater.com/api/v1/gardening/projects/{projectId}?version=385" \
-X PUT \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
-H "Unity-Api-Gateway-Replace-UserId: example string value" \
--data "{
\"id\": \"example string value\",
\"name\": \"example string value\",
\"user_id\": \"example string value\",
\"address\": \"example string value\",
\"location\": {
\"type\": \"Point\",
\"coordinates\": [
763.1263429127291
],
\"properties\": {
\"example_property_name\": \"example string value\"
}
},
\"created_at\": 141,
\"updated_at\": 895,
\"version\": \"-1\",
\"postal_code\": \"example string value\",
\"billingAddress\": {
\"firstName\": \"example string value\",
\"lastName\": \"example string value\",
\"company\": \"example string value\",
\"address1\": \"example string value\",
\"address2\": \"example string value\",
\"city\": \"example string value\",
\"state\": \"example string value\",
\"postcode\": \"example string value\",
\"country\": \"example string value\",
\"email\": \"example string value\",
\"phone\": \"example string value\"
},
\"projectAddress\": {
\"address1\": \"example string value\",
\"address2\": \"example string value\",
\"city\": \"example string value\",
\"state\": \"example string value\",
\"postcode\": \"example string value\",
\"country\": \"example string value\"
},
\"unity\": true
}"
On success, the above request returns response like
{
"id": "example string value",
"name": "example string value",
"user_id": "example string value",
"address": "example string value",
"location": {
"type": "Point",
"coordinates": [
569.5050757236336
],
"properties": {
"example_property_name": "example string value"
}
},
"created_at": 172,
"updated_at": 907,
"version": "-1",
"postal_code": "example string value",
"billingAddress": {
"firstName": "example string value",
"lastName": "example string value",
"company": "example string value",
"address1": "example string value",
"address2": "example string value",
"city": "example string value",
"state": "example string value",
"postcode": "example string value",
"country": "example string value",
"email": "example string value",
"phone": "example string value"
},
"projectAddress": {
"address1": "example string value",
"address2": "example string value",
"city": "example string value",
"state": "example string value",
"postcode": "example string value",
"country": "example string value"
},
"unity": false
}
HTTP Request
PUT /api/v1/gardening/projects/{projectId}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
Unity-Api-Gateway-Replace-UserId |
string |
header |
false |
|
projectId |
string |
path |
true |
id of Project |
Project |
GardeningProject |
body |
true |
|
version |
integer |
query |
false |
version of Project |
GardeningProject
Name |
Type |
Required |
Description |
id |
string |
false |
A unique string that can be used to identify and reference a specific project. |
name |
string |
false |
A human-readable name for the project. |
user_id |
string |
false |
|
address |
string |
false |
A human-readable name for the project. |
location |
Point |
false |
Location information |
created_at |
integer |
false |
Unix timestamp of a project creation |
updated_at |
integer |
false |
Unix timestamp of a project last update |
version |
integer |
false |
Version of a project |
postal_code |
string |
false |
Project’s postal code. |
billingAddress |
BillingAddress |
false |
|
projectAddress |
ProjectAddress |
false |
|
unity |
boolean |
false |
|
Point
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ] |
true |
|
properties |
Object for field properties |
false |
|
Object for field properties
Name |
Type |
Required |
Description |
* |
string |
false |
|
BillingAddress
Name |
Type |
Required |
Description |
firstName |
string |
false |
|
lastName |
string |
false |
|
company |
string |
false |
|
address1 |
string |
false |
|
address2 |
string |
false |
|
city |
string |
false |
|
state |
string |
false |
|
postcode |
string |
false |
|
country |
string |
false |
|
email |
string |
false |
|
phone |
string |
false |
|
ProjectAddress
Name |
Type |
Required |
Description |
address1 |
string |
false |
|
address2 |
string |
false |
|
city |
string |
false |
|
state |
string |
false |
|
postcode |
string |
false |
|
country |
string |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
404 |
Not Found |
409 |
Conflict |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
false |
A unique string that can be used to identify and reference a specific project. |
name |
string |
false |
A human-readable name for the project. |
user_id |
string |
false |
|
address |
string |
false |
A human-readable name for the project. |
location |
Point |
false |
Location information |
created_at |
integer |
false |
Unix timestamp of a project creation |
updated_at |
integer |
false |
Unix timestamp of a project last update |
version |
integer |
false |
Version of a project |
postal_code |
string |
false |
Project’s postal code. |
billingAddress |
BillingAddress |
false |
|
projectAddress |
ProjectAddress |
false |
|
unity |
boolean |
false |
|
Point
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ] |
true |
|
properties |
Object for field properties |
false |
|
Object for field properties
Name |
Type |
Required |
Description |
* |
string |
false |
|
BillingAddress
Name |
Type |
Required |
Description |
firstName |
string |
false |
|
lastName |
string |
false |
|
company |
string |
false |
|
address1 |
string |
false |
|
address2 |
string |
false |
|
city |
string |
false |
|
state |
string |
false |
|
postcode |
string |
false |
|
country |
string |
false |
|
email |
string |
false |
|
phone |
string |
false |
|
ProjectAddress
Name |
Type |
Required |
Description |
address1 |
string |
false |
|
address2 |
string |
false |
|
city |
string |
false |
|
state |
string |
false |
|
postcode |
string |
false |
|
country |
string |
false |
|
V1 | Regions | Region Types
The Gardening Region Types API provides a list of possible choices for types of vegetation, used to provide the available options when mapping areas.
Get Region Types Tree
Request
curl "https://developer-api.etwater.com/api/v1/gardening/region-types" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
HTTP Request
GET /api/v1/gardening/region-types
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
HTTP Responses
Code |
Description |
200 |
Successful Response |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response is array of
V1 | Regions | Regions
Request
curl "https://developer-api.etwater.com/api/v1/gardening/projects/{projectId}/regions?controllerId=example+string+value" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
[
{
"type": "Feature",
"geometry": {
"type": "Point",
"coordinates": [
609
],
"properties": {
"example_property_name": "example string value"
}
},
"properties": {
"region_name": "example string value",
"vegetation_type": 346,
"sprinkler_type": 570,
"shade_level": 2,
"newly_planted": true,
"local_region_id": 127,
"remote_region_id": "example string value",
"images": [
{
"image_id": "example string value",
"imageFormat": "example string value",
"width": 320,
"height": 943,
"thumbnails": [
{
"image_id": "example string value",
"imageFormat": "example string value",
"width": 478,
"height": 579,
"thumbnails": [],
"updatedAt": 519,
"imageType": 878
}
],
"updatedAt": 587,
"imageType": 572
}
],
"controller_serial": "example string value",
"emitter_count": 37,
"plant_area": 965,
"zone_id": "example string value",
"project_id": "example string value",
"created_at": 426,
"updated_at": 731,
"plant_center_coordinates": [
212.43868824673757
],
"status": "DELETED",
"parcel_id": "example string value",
"parcelExists": false,
"elevation": 641.417461746101,
"plantFactor": [
346
],
"onlyVegetation": "1",
"extendAreaRegionType": "1",
"version": "-1",
"controllerId": "example string value",
"areaId": "example string value"
}
}
]
Dependent on:
- Authorization: Bearer {access_token}
- Content-Type: application/json
where: {access_token} access token value.
Path parameter description
{projectId} - project id value.
Response body field description:
geolocation pair of longitude/latitude. Example:[[[76.37695312499999, 15.749962572748768], [ 76.37695312499999, 18.396230138028827], [79.27734374999999, 18.396230138028827]]]
* properties subentity - contains infomation about the region, subfields:
* region_name - name of region;
* (required) vegetation_type - type of vegetation, which
growth
on region, possible values: 0 (TURF), 1 (SHRUBS), 2 (TREES), 3 (FLOWER_AND_VEGETABLES), 4 (CACTI_AND_SUCCULENTS);
* (required) sprinkler_type - type of emitter, which watering
the region, possible values: 0 (SPRAY), 1 (STREAM), 2 (BUBBLER), 3 (DRIP);
* shade_level - describes the shaded level of region, value
can
be 0 - 100%;
* newly_planted - describe if plant is newly planted or not;
* user_id - id of owner user;
* local_region_id - id uses in mobile app;
* remote_region_id - id of region (regionId), uses for
get,
update, delete methods;
* images - contains information about images on S3 Amazon
Service;
* controller_serial - serial number of controller;
* emitter_count - amount of emitters on region;
* plant_area - area of the region, square meters;
* zone_id - id of zone to which region related;
* project_id - id of project to which region related;
* created_at - date and time when region was created, epoch
millis in UTC;
* updated_at - date and time when region was updated, epoch
millis in UTC;
* plant_center_coordinates - array of geolocation pair of
longitude/latitude, which describe the center of region, example: [ 76.37695312499999, 18.396230138028827];
* status - status of region, can be: ACTIVE, DELETED;
* parcel_id - id of the parcel;
* parcelExists - describes if parcel exist for this region;
* elevation - elevation of the region;
* plantFactor - plant factor values per month.
HTTP Request
GET /api/v1/gardening/projects/{projectId}/regions
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
projectId |
string |
path |
true |
Project id |
controllerId |
string |
query |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
404 |
Not Found |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response is array of
Name |
Type |
Required |
Description |
type |
string |
true |
|
geometry |
Geometry |
false |
|
properties |
RegionProperties |
false |
|
Geometry is any of: Point, MultiPoint, LineString, MultiLineString, Polygon, MultiPolygon, GeometryCollection
Point
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ] |
true |
|
properties |
Object for field properties |
false |
|
Object for field properties
Name |
Type |
Required |
Description |
* |
string |
false |
|
MultiPoint
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
LineString
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
MultiLineString
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
Polygon
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
MultiPolygon
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ][ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
GeometryCollection
Name |
Type |
Required |
Description |
type |
string |
true |
|
geometries |
Geometry[ ] |
true |
|
RegionProperties
Name |
Type |
Required |
Description |
region_name |
string |
false |
|
vegetation_type |
integer |
false |
|
sprinkler_type |
integer |
false |
|
shade_level |
integer |
false |
|
newly_planted |
boolean |
false |
|
local_region_id |
integer |
false |
|
remote_region_id |
string |
false |
|
images |
ImageInfo[ ] |
false |
|
controller_serial |
string |
false |
|
emitter_count |
integer |
false |
|
plant_area |
number |
false |
|
zone_id |
string |
false |
|
project_id |
string |
true |
|
created_at |
integer |
false |
|
updated_at |
integer |
false |
|
plant_center_coordinates |
number[ ] |
false |
|
status |
string |
false |
|
parcel_id |
string |
false |
|
parcelExists |
boolean |
false |
|
elevation |
number |
false |
|
plantFactor |
number[ ] |
false |
|
onlyVegetation |
boolean |
false |
|
extendAreaRegionType |
boolean |
false |
|
version |
integer |
false |
|
controllerId |
string |
false |
|
areaId |
string |
false |
|
ImageInfo
Name |
Type |
Required |
Description |
image_id |
string |
true |
|
imageFormat |
string |
false |
|
width |
integer |
false |
|
height |
integer |
false |
|
thumbnails |
ImageInfo[ ] |
false |
|
updatedAt |
integer |
false |
|
imageType |
integer |
false |
|
Create a Region.
Request
curl "https://developer-api.etwater.com/api/v1/gardening/projects/{projectId}/regions?version=899" \
-X POST \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
--data "{
\"type\": \"Feature\",
\"geometry\": {
\"type\": \"MultiLineString\",
\"coordinates\": [
[
[
56
]
]
],
\"properties\": {
\"example_property_name\": \"example string value\"
}
},
\"properties\": {
\"region_name\": \"example string value\",
\"vegetation_type\": 479,
\"sprinkler_type\": 251,
\"shade_level\": 755,
\"newly_planted\": false,
\"local_region_id\": 387,
\"remote_region_id\": \"example string value\",
\"images\": [
{
\"image_id\": \"example string value\",
\"imageFormat\": \"example string value\",
\"width\": 401,
\"height\": 762,
\"thumbnails\": [
{
\"image_id\": \"example string value\",
\"imageFormat\": \"example string value\",
\"width\": 330,
\"height\": 503,
\"thumbnails\": [],
\"updatedAt\": 402,
\"imageType\": 809
}
],
\"updatedAt\": 840,
\"imageType\": 543
}
],
\"controller_serial\": \"example string value\",
\"emitter_count\": 400,
\"plant_area\": 364.5760046153217,
\"zone_id\": \"example string value\",
\"project_id\": \"example string value\",
\"created_at\": 791,
\"updated_at\": 767,
\"plant_center_coordinates\": [
554.4469391715
],
\"status\": \"DELETED\",
\"parcel_id\": \"example string value\",
\"parcelExists\": true,
\"elevation\": 607.4394116212798,
\"plantFactor\": [
563
],
\"onlyVegetation\": \"1\",
\"extendAreaRegionType\": \"1\",
\"version\": \"-1\",
\"controllerId\": \"example string value\",
\"areaId\": \"example string value\"
}
}"
On success, the above request returns response like
Dependent on:
- Authorization: Bearer {access_token}
- Content-Type: application/json
where: {access_token} access token value.
Path parameter description
{projectId} - project id value.
Request body fields description:
Request body fields description the same as for response body.
Note, if you want correct ET calculation and watering schedule generation, following fields should be populated in request body:
* controller_serial - serial number of controller. It requires for watering schedule generation. If region does not linked to controller, schedule for this region won't be generated. Schedule is generated for controller;
* zone_id - id of zone. It requires for watering schedule generation;
* plant_center_coordinates - coordinates of region. Coordinates are used to gather weather and solar radiation information (used for ET calculation) and for getting soil information (used for watering schedule generation);
* plant_area - cron size of the plant in case BUBBLER or DRIP emitter type is used otherwase 1 square meter value can be used.
Response body field description:
- (required) type - should be "Feature";
- geometry subentity - describe the geometry (shape) of the area by coordinates, subfields:
- type - type of geometry, should be "Polygon".
- coordinates - three-dimensional array which contains the geolocation pair of longitude/latitude. Example:[[ [76.37695312499999, 15.749962572748768], [ 76.37695312499999, 18.396230138028827], [79.27734374999999, 18.396230138028827]]]
- properties subentity - contains infomation about the region, subfields:
- region_name - name of region;
- (required) vegetation_type - type of vegetation, which growth on region, possible values: 0 (TURF), 1 (SHRUBS), 2 (TREES), 3 (FLOWER_AND_VEGETABLES), 4 (CACTI_AND_SUCCULENTS);
- (required) sprinkler_type - type of emitter, which watering the region, possible values: 0 (SPRAY), 1 (STREAM), 2 (BUBBLER), 3 (DRIP);
- shade_level - describes the shaded level of region, value can be 0 - 100%;
- newly_planted - describe if plant is newly planted or not;
- user_id - id of owner user;
- local_region_id - id uses in mobile app;
- remote_region_id - id of region (regionId), uses for get, update, delete methods;
- images - contains information about images on S3 Amazon Service;
- controller_serial - serial number of controller;
- emitter_count - amount of emitters on region;
- plant_area - area of the region, square meters;
- zone_id - id of zone to which region related;
- project_id - id of project to which region related;
- created_at - date and time when region was created, epoch millis in UTC;
- updated_at - date and time when region was updated, epoch millis in UTC;
- plant_center_coordinates - array of geolocation pair of longitude/latitude, which describe the center of region, example: [ 76.37695312499999, 18.396230138028827];
- status - status of region, can be: ACTIVE, DELETED;
- parcel_id - id of the parcel;
- parcelExists - describes if parcel exist for this region;
- elevation - elevation of the region;
- plantFactor - plant factor values per month.
HTTP Request
POST /api/v1/gardening/projects/{projectId}/regions
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
projectId |
string |
path |
true |
Project id |
region |
GardeningRegion |
body |
true |
|
version |
integer |
query |
false |
version of Project |
GardeningRegion
Name |
Type |
Required |
Description |
type |
string |
true |
|
geometry |
Geometry |
false |
|
properties |
RegionProperties |
false |
|
Geometry is any of: Point, MultiPoint, LineString, MultiLineString, Polygon, MultiPolygon, GeometryCollection
Point
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ] |
true |
|
properties |
Object for field properties |
false |
|
Object for field properties
Name |
Type |
Required |
Description |
* |
string |
false |
|
MultiPoint
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
LineString
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
MultiLineString
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
Polygon
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
MultiPolygon
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ][ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
GeometryCollection
Name |
Type |
Required |
Description |
type |
string |
true |
|
geometries |
Geometry[ ] |
true |
|
RegionProperties
Name |
Type |
Required |
Description |
region_name |
string |
false |
|
vegetation_type |
integer |
false |
|
sprinkler_type |
integer |
false |
|
shade_level |
integer |
false |
|
newly_planted |
boolean |
false |
|
local_region_id |
integer |
false |
|
remote_region_id |
string |
false |
|
images |
ImageInfo[ ] |
false |
|
controller_serial |
string |
false |
|
emitter_count |
integer |
false |
|
plant_area |
number |
false |
|
zone_id |
string |
false |
|
project_id |
string |
true |
|
created_at |
integer |
false |
|
updated_at |
integer |
false |
|
plant_center_coordinates |
number[ ] |
false |
|
status |
string |
false |
|
parcel_id |
string |
false |
|
parcelExists |
boolean |
false |
|
elevation |
number |
false |
|
plantFactor |
number[ ] |
false |
|
onlyVegetation |
boolean |
false |
|
extendAreaRegionType |
boolean |
false |
|
version |
integer |
false |
|
controllerId |
string |
false |
|
areaId |
string |
false |
|
ImageInfo
Name |
Type |
Required |
Description |
image_id |
string |
true |
|
imageFormat |
string |
false |
|
width |
integer |
false |
|
height |
integer |
false |
|
thumbnails |
ImageInfo[ ] |
false |
|
updatedAt |
integer |
false |
|
imageType |
integer |
false |
|
HTTP Responses
Code |
Description |
201 |
Created |
404 |
Not Found |
409 |
Conflict |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
type |
string |
true |
|
geometry |
Geometry |
false |
|
properties |
RegionProperties |
false |
|
Geometry is any of: Point, MultiPoint, LineString, MultiLineString, Polygon, MultiPolygon, GeometryCollection
Point
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ] |
true |
|
properties |
Object for field properties |
false |
|
Object for field properties
Name |
Type |
Required |
Description |
* |
string |
false |
|
MultiPoint
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
LineString
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
MultiLineString
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
Polygon
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
MultiPolygon
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ][ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
GeometryCollection
Name |
Type |
Required |
Description |
type |
string |
true |
|
geometries |
Geometry[ ] |
true |
|
RegionProperties
Name |
Type |
Required |
Description |
region_name |
string |
false |
|
vegetation_type |
integer |
false |
|
sprinkler_type |
integer |
false |
|
shade_level |
integer |
false |
|
newly_planted |
boolean |
false |
|
local_region_id |
integer |
false |
|
remote_region_id |
string |
false |
|
images |
ImageInfo[ ] |
false |
|
controller_serial |
string |
false |
|
emitter_count |
integer |
false |
|
plant_area |
number |
false |
|
zone_id |
string |
false |
|
project_id |
string |
true |
|
created_at |
integer |
false |
|
updated_at |
integer |
false |
|
plant_center_coordinates |
number[ ] |
false |
|
status |
string |
false |
|
parcel_id |
string |
false |
|
parcelExists |
boolean |
false |
|
elevation |
number |
false |
|
plantFactor |
number[ ] |
false |
|
onlyVegetation |
boolean |
false |
|
extendAreaRegionType |
boolean |
false |
|
version |
integer |
false |
|
controllerId |
string |
false |
|
areaId |
string |
false |
|
ImageInfo
Name |
Type |
Required |
Description |
image_id |
string |
true |
|
imageFormat |
string |
false |
|
width |
integer |
false |
|
height |
integer |
false |
|
thumbnails |
ImageInfo[ ] |
false |
|
updatedAt |
integer |
false |
|
imageType |
integer |
false |
|
Create or update regions for project
Request
curl "https://developer-api.etwater.com/api/v1/gardening/projects/{projectId}/regions?version=289" \
-X PATCH \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
--data "[
{
\"type\": \"Feature\",
\"geometry\": {
\"type\": \"MultiLineString\",
\"coordinates\": [
[
[
245.86794723098538
]
]
],
\"properties\": {
\"example_property_name\": \"example string value\"
}
},
\"properties\": {
\"region_name\": \"example string value\",
\"vegetation_type\": 819,
\"sprinkler_type\": 142,
\"shade_level\": 249,
\"newly_planted\": true,
\"local_region_id\": 594,
\"remote_region_id\": \"example string value\",
\"images\": [
{
\"image_id\": \"example string value\",
\"imageFormat\": \"example string value\",
\"width\": 763,
\"height\": 76,
\"thumbnails\": [
{
\"image_id\": \"example string value\",
\"imageFormat\": \"example string value\",
\"width\": 633,
\"height\": 763,
\"thumbnails\": [],
\"updatedAt\": 427,
\"imageType\": 827
}
],
\"updatedAt\": 111,
\"imageType\": 543
}
],
\"controller_serial\": \"example string value\",
\"emitter_count\": 15,
\"plant_area\": 97,
\"zone_id\": \"example string value\",
\"project_id\": \"example string value\",
\"created_at\": 839,
\"updated_at\": 570,
\"plant_center_coordinates\": [
472.75565866043587
],
\"status\": \"DELETED\",
\"parcel_id\": \"example string value\",
\"parcelExists\": false,
\"elevation\": 255,
\"plantFactor\": [
446
],
\"onlyVegetation\": \"1\",
\"extendAreaRegionType\": \"1\",
\"version\": \"-1\",
\"controllerId\": \"example string value\",
\"areaId\": \"example string value\"
}
}
]"
On success, the above request returns response like
[
{
"type": "Feature",
"geometry": {
"type": "GeometryCollection",
"geometries": []
},
"properties": {
"region_name": "example string value",
"vegetation_type": 647,
"sprinkler_type": 192,
"shade_level": 683,
"newly_planted": false,
"local_region_id": 609,
"remote_region_id": "example string value",
"images": [
{
"image_id": "example string value",
"imageFormat": "example string value",
"width": 192,
"height": 291,
"thumbnails": [
{
"image_id": "example string value",
"imageFormat": "example string value",
"width": 820,
"height": 799,
"thumbnails": [],
"updatedAt": 875,
"imageType": 818
}
],
"updatedAt": 698,
"imageType": 848
}
],
"controller_serial": "example string value",
"emitter_count": 297,
"plant_area": 118.77341853397593,
"zone_id": "example string value",
"project_id": "example string value",
"created_at": 398,
"updated_at": 312,
"plant_center_coordinates": [
887
],
"status": "DELETED",
"parcel_id": "example string value",
"parcelExists": false,
"elevation": 366.0664853481885,
"plantFactor": [
980
],
"onlyVegetation": "1",
"extendAreaRegionType": "1",
"version": "-1",
"controllerId": "example string value",
"areaId": "example string value"
}
}
]
HTTP Request
PATCH /api/v1/gardening/projects/{projectId}/regions
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
projectId |
string |
path |
true |
Project id |
Regions |
GardeningRegion[ ] |
body |
true |
|
version |
integer |
query |
false |
version of Project |
GardeningRegion
Name |
Type |
Required |
Description |
type |
string |
true |
|
geometry |
Geometry |
false |
|
properties |
RegionProperties |
false |
|
Geometry is any of: Point, MultiPoint, LineString, MultiLineString, Polygon, MultiPolygon, GeometryCollection
Point
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ] |
true |
|
properties |
Object for field properties |
false |
|
Object for field properties
Name |
Type |
Required |
Description |
* |
string |
false |
|
MultiPoint
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
LineString
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
MultiLineString
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
Polygon
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
MultiPolygon
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ][ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
GeometryCollection
Name |
Type |
Required |
Description |
type |
string |
true |
|
geometries |
Geometry[ ] |
true |
|
RegionProperties
Name |
Type |
Required |
Description |
region_name |
string |
false |
|
vegetation_type |
integer |
false |
|
sprinkler_type |
integer |
false |
|
shade_level |
integer |
false |
|
newly_planted |
boolean |
false |
|
local_region_id |
integer |
false |
|
remote_region_id |
string |
false |
|
images |
ImageInfo[ ] |
false |
|
controller_serial |
string |
false |
|
emitter_count |
integer |
false |
|
plant_area |
number |
false |
|
zone_id |
string |
false |
|
project_id |
string |
true |
|
created_at |
integer |
false |
|
updated_at |
integer |
false |
|
plant_center_coordinates |
number[ ] |
false |
|
status |
string |
false |
|
parcel_id |
string |
false |
|
parcelExists |
boolean |
false |
|
elevation |
number |
false |
|
plantFactor |
number[ ] |
false |
|
onlyVegetation |
boolean |
false |
|
extendAreaRegionType |
boolean |
false |
|
version |
integer |
false |
|
controllerId |
string |
false |
|
areaId |
string |
false |
|
ImageInfo
Name |
Type |
Required |
Description |
image_id |
string |
true |
|
imageFormat |
string |
false |
|
width |
integer |
false |
|
height |
integer |
false |
|
thumbnails |
ImageInfo[ ] |
false |
|
updatedAt |
integer |
false |
|
imageType |
integer |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
404 |
Not Found |
409 |
Conflict |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response is array of
Name |
Type |
Required |
Description |
type |
string |
true |
|
geometry |
Geometry |
false |
|
properties |
RegionProperties |
false |
|
Geometry is any of: Point, MultiPoint, LineString, MultiLineString, Polygon, MultiPolygon, GeometryCollection
Point
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ] |
true |
|
properties |
Object for field properties |
false |
|
Object for field properties
Name |
Type |
Required |
Description |
* |
string |
false |
|
MultiPoint
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
LineString
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
MultiLineString
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
Polygon
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
MultiPolygon
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ][ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
GeometryCollection
Name |
Type |
Required |
Description |
type |
string |
true |
|
geometries |
Geometry[ ] |
true |
|
RegionProperties
Name |
Type |
Required |
Description |
region_name |
string |
false |
|
vegetation_type |
integer |
false |
|
sprinkler_type |
integer |
false |
|
shade_level |
integer |
false |
|
newly_planted |
boolean |
false |
|
local_region_id |
integer |
false |
|
remote_region_id |
string |
false |
|
images |
ImageInfo[ ] |
false |
|
controller_serial |
string |
false |
|
emitter_count |
integer |
false |
|
plant_area |
number |
false |
|
zone_id |
string |
false |
|
project_id |
string |
true |
|
created_at |
integer |
false |
|
updated_at |
integer |
false |
|
plant_center_coordinates |
number[ ] |
false |
|
status |
string |
false |
|
parcel_id |
string |
false |
|
parcelExists |
boolean |
false |
|
elevation |
number |
false |
|
plantFactor |
number[ ] |
false |
|
onlyVegetation |
boolean |
false |
|
extendAreaRegionType |
boolean |
false |
|
version |
integer |
false |
|
controllerId |
string |
false |
|
areaId |
string |
false |
|
ImageInfo
Name |
Type |
Required |
Description |
image_id |
string |
true |
|
imageFormat |
string |
false |
|
width |
integer |
false |
|
height |
integer |
false |
|
thumbnails |
ImageInfo[ ] |
false |
|
updatedAt |
integer |
false |
|
imageType |
integer |
false |
|
Delete all regions for project
Request
curl "https://developer-api.etwater.com/api/v1/gardening/projects/{projectId}/regions?version=231" \
-X DELETE \
-H "Authorization: example string value"
On success, the above request returns response like
HTTP Request
DELETE /api/v1/gardening/projects/{projectId}/regions
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
projectId |
string |
path |
true |
Project id |
version |
integer |
query |
false |
version of Project |
HTTP Responses
Code |
Description |
204 |
No Content |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Get Region by id.
Request
curl "https://developer-api.etwater.com/api/v1/gardening/projects/{projectId}/regions/{regionId}" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"type": "Feature",
"geometry": {
"type": "LineString",
"coordinates": [
[
60
]
],
"properties": {
"example_property_name": "example string value"
}
},
"properties": {
"region_name": "example string value",
"vegetation_type": 222,
"sprinkler_type": 74,
"shade_level": 357,
"newly_planted": false,
"local_region_id": 74,
"remote_region_id": "example string value",
"images": [
{
"image_id": "example string value",
"imageFormat": "example string value",
"width": 222,
"height": 653,
"thumbnails": [
{
"image_id": "example string value",
"imageFormat": "example string value",
"width": 7,
"height": 869,
"thumbnails": [],
"updatedAt": 964,
"imageType": 11
}
],
"updatedAt": 116,
"imageType": 809
}
],
"controller_serial": "example string value",
"emitter_count": 468,
"plant_area": 544,
"zone_id": "example string value",
"project_id": "example string value",
"created_at": 962,
"updated_at": 159,
"plant_center_coordinates": [
640
],
"status": "DELETED",
"parcel_id": "example string value",
"parcelExists": true,
"elevation": 727,
"plantFactor": [
7
],
"onlyVegetation": "1",
"extendAreaRegionType": "1",
"version": "-1",
"controllerId": "example string value",
"areaId": "example string value"
}
}
Dependent on:
- Authorization: Bearer {access_token}
- Content-Type: application/json
where: {access_token} access token value.
Path parameter description
- {projectId} - project id value.
- {regionId} - region id value.
Response body field description:
geolocation pair of longitude/latitude. Example:[ [76.37695312499999, 15.749962572748768], [ 76.37695312499999, 18.396230138028827], [79.27734374999999, 18.396230138028827]]
* properties subentity - contains infomation about the region, subfields:
* region_name - name of region;
* (required) vegetation_type - type of vegetation, which
growth
on region, possible values: 0 (TURF), 1 (SHRUBS), 2 (TREES), 3 (FLOWER_AND_VEGETABLES), 4 (CACTI_AND_SUCCULENTS);
* (required) sprinkler_type - type of emitter, which watering
the region, possible values: 0 (SPRAY), 1 (STREAM), 2 (BUBBLER), 3 (DRIP);
* shade_level - describes the shaded level of region, value
can
be 0 - 100%;
* newly_planted - describe if plant is newly planted or not;
* user_id - id of owner user;
* local_region_id - id uses in mobile app;
* remote_region_id - id of region (regionId), uses for
get,
update, delete methods;
* images - contains information about images on S3 Amazon
Service;
* controller_serial - serial number of controller;
* emitter_count - amount of emitters on region;
* plant_area - area of the region, square meters;
* zone_id - id of zone to which region related;
* project_id - id of project to which region related;
* created_at - date and time when region was created, epoch
millis in UTC;
* updated_at - date and time when region was updated, epoch
millis in UTC;
* plant_center_coordinates - array of geolocation pair of
longitude/latitude, which describe the center of region, example: [ 76.37695312499999, 18.396230138028827];
* status - status of region, can be: ACTIVE, DELETED;
* parcel_id - id of the parcel;
* parcelExists - describes if parcel exist for this region;
* elevation - elevation of the region;
* plantFactor - plant factor values per month.
HTTP Request
GET /api/v1/gardening/projects/{projectId}/regions/{regionId}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
projectId |
string |
path |
true |
Project id |
regionId |
string |
path |
true |
Region id |
HTTP Responses
Code |
Description |
200 |
OK |
404 |
Not Found |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
type |
string |
true |
|
geometry |
Geometry |
false |
|
properties |
RegionProperties |
false |
|
Geometry is any of: Point, MultiPoint, LineString, MultiLineString, Polygon, MultiPolygon, GeometryCollection
Point
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ] |
true |
|
properties |
Object for field properties |
false |
|
Object for field properties
Name |
Type |
Required |
Description |
* |
string |
false |
|
MultiPoint
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
LineString
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
MultiLineString
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
Polygon
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
MultiPolygon
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ][ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
GeometryCollection
Name |
Type |
Required |
Description |
type |
string |
true |
|
geometries |
Geometry[ ] |
true |
|
RegionProperties
Name |
Type |
Required |
Description |
region_name |
string |
false |
|
vegetation_type |
integer |
false |
|
sprinkler_type |
integer |
false |
|
shade_level |
integer |
false |
|
newly_planted |
boolean |
false |
|
local_region_id |
integer |
false |
|
remote_region_id |
string |
false |
|
images |
ImageInfo[ ] |
false |
|
controller_serial |
string |
false |
|
emitter_count |
integer |
false |
|
plant_area |
number |
false |
|
zone_id |
string |
false |
|
project_id |
string |
true |
|
created_at |
integer |
false |
|
updated_at |
integer |
false |
|
plant_center_coordinates |
number[ ] |
false |
|
status |
string |
false |
|
parcel_id |
string |
false |
|
parcelExists |
boolean |
false |
|
elevation |
number |
false |
|
plantFactor |
number[ ] |
false |
|
onlyVegetation |
boolean |
false |
|
extendAreaRegionType |
boolean |
false |
|
version |
integer |
false |
|
controllerId |
string |
false |
|
areaId |
string |
false |
|
ImageInfo
Name |
Type |
Required |
Description |
image_id |
string |
true |
|
imageFormat |
string |
false |
|
width |
integer |
false |
|
height |
integer |
false |
|
thumbnails |
ImageInfo[ ] |
false |
|
updatedAt |
integer |
false |
|
imageType |
integer |
false |
|
Delete Region by id.
Request
curl "https://developer-api.etwater.com/api/v1/gardening/projects/{projectId}/regions/{regionId}?version=966" \
-X DELETE \
-H "Authorization: example string value"
On success, the above request returns response like
Dependent on:
- Authorization: Bearer {access_token}
- Content-Type: application/json
where: {access_token} access token value.
Path parameter description
- {projectId} - project id value.
- {regionId} - region id value.
HTTP Request
DELETE /api/v1/gardening/projects/{projectId}/regions/{regionId}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
projectId |
string |
path |
true |
Project id |
regionId |
string |
path |
true |
id of Region |
version |
integer |
query |
false |
version of Project |
HTTP Responses
Code |
Description |
204 |
No Content |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Update Area Region by id.
Request
curl "https://developer-api.etwater.com/api/v1/gardening/projects/{projectId}/regions/{regionId}?version=576" \
-X PUT \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
--data "{
\"type\": \"Feature\",
\"geometry\": {
\"type\": \"MultiLineString\",
\"coordinates\": [
[
[
535.8188345729461
]
]
],
\"properties\": {
\"example_property_name\": \"example string value\"
}
},
\"properties\": {
\"region_name\": \"example string value\",
\"vegetation_type\": 374,
\"sprinkler_type\": 968,
\"shade_level\": 438,
\"newly_planted\": false,
\"local_region_id\": 648,
\"remote_region_id\": \"example string value\",
\"images\": [
{
\"image_id\": \"example string value\",
\"imageFormat\": \"example string value\",
\"width\": 552,
\"height\": 519,
\"thumbnails\": [
{
\"image_id\": \"example string value\",
\"imageFormat\": \"example string value\",
\"width\": 62,
\"height\": 619,
\"thumbnails\": [],
\"updatedAt\": 370,
\"imageType\": 659
}
],
\"updatedAt\": 524,
\"imageType\": 595
}
],
\"controller_serial\": \"example string value\",
\"emitter_count\": 66,
\"plant_area\": 280,
\"zone_id\": \"example string value\",
\"project_id\": \"example string value\",
\"created_at\": 994,
\"updated_at\": 892,
\"plant_center_coordinates\": [
530
],
\"status\": \"ACTIVE\",
\"parcel_id\": \"example string value\",
\"parcelExists\": false,
\"elevation\": 782.135110712673,
\"plantFactor\": [
926
],
\"onlyVegetation\": \"1\",
\"extendAreaRegionType\": \"1\",
\"version\": \"-1\",
\"controllerId\": \"example string value\",
\"areaId\": \"example string value\"
}
}"
On success, the above request returns response like
{
"type": "Feature",
"geometry": {
"type": "MultiLineString",
"coordinates": [
[
[
727.427539288731
]
]
],
"properties": {
"example_property_name": "example string value"
}
},
"properties": {
"region_name": "example string value",
"vegetation_type": 722,
"sprinkler_type": 874,
"shade_level": 617,
"newly_planted": true,
"local_region_id": 515,
"remote_region_id": "example string value",
"images": [
{
"image_id": "example string value",
"imageFormat": "example string value",
"width": 86,
"height": 5,
"thumbnails": [
{
"image_id": "example string value",
"imageFormat": "example string value",
"width": 458,
"height": 41,
"thumbnails": [],
"updatedAt": 916,
"imageType": 835
}
],
"updatedAt": 112,
"imageType": 125
}
],
"controller_serial": "example string value",
"emitter_count": 976,
"plant_area": 362.77075128758827,
"zone_id": "example string value",
"project_id": "example string value",
"created_at": 69,
"updated_at": 857,
"plant_center_coordinates": [
331
],
"status": "ACTIVE",
"parcel_id": "example string value",
"parcelExists": false,
"elevation": 79.02533285274419,
"plantFactor": [
513.15552113259
],
"onlyVegetation": "1",
"extendAreaRegionType": "1",
"version": "-1",
"controllerId": "example string value",
"areaId": "example string value"
}
}
Dependent on:
- Authorization: Bearer {access_token}
- Content-Type: application/json
where: {access_token} access token value.
Path parameter description
- {projectId} - project id value.
- {regionId} - region id value.
Request body fields description:
The same as for response body.
Response body field description:
geolocation pair of longitude/latitude. Example:[ [76.37695312499999, 15.749962572748768], [ 76.37695312499999, 18.396230138028827], [79.27734374999999, 18.396230138028827]]
* properties subentity - contains infomation about the region, subfields:
* region_name - name of region;
* (required) vegetation_type - type of vegetation, which
growth
on region, possible values: 0 (TURF), 1 (SHRUBS), 2 (TREES), 3 (FLOWER_AND_VEGETABLES), 4 (CACTI_AND_SUCCULENTS);
* (required) sprinkler_type - type of emitter, which watering
the region, possible values: 0 (SPRAY), 1 (STREAM), 2 (BUBBLER), 3 (DRIP);
* shade_level - describes the shaded level of region, value
can
be 0 - 100%;
* newly_planted - describe if plant is newly planted or not;
* user_id - id of owner user;
* local_region_id - id uses in mobile app;
* remote_region_id - id of region (regionId), uses for
get,
update, delete methods;
* images - contains information about images on S3 Amazon
Service;
* controller_serial - serial number of controller;
* emitter_count - amount of emitters on region;
* plant_area - area of the region, square meters;
* zone_id - id of zone to which region related;
* project_id - id of project to which region related;
* created_at - date and time when region was created, epoch
millis in UTC;
* updated_at - date and time when region was updated, epoch
millis in UTC;
* plant_center_coordinates - array of geolocation pair of
longitude/latitude, which describe the center of region, example: [ 76.37695312499999, 18.396230138028827];
* status - status of region, can be: ACTIVE, DELETED;
* parcel_id - id of the parcel;
* parcelExists - describes if parcel exist for this region;
* elevation - elevation of the region;
* plantFactor - plant factor values per month.
HTTP Request
PUT /api/v1/gardening/projects/{projectId}/regions/{regionId}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
projectId |
string |
path |
true |
Project id |
regionId |
string |
path |
true |
id of Region |
region |
GardeningRegion |
body |
true |
|
version |
integer |
query |
false |
version of Project |
GardeningRegion
Name |
Type |
Required |
Description |
type |
string |
true |
|
geometry |
Geometry |
false |
|
properties |
RegionProperties |
false |
|
Geometry is any of: Point, MultiPoint, LineString, MultiLineString, Polygon, MultiPolygon, GeometryCollection
Point
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ] |
true |
|
properties |
Object for field properties |
false |
|
Object for field properties
Name |
Type |
Required |
Description |
* |
string |
false |
|
MultiPoint
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
LineString
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
MultiLineString
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
Polygon
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
MultiPolygon
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ][ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
GeometryCollection
Name |
Type |
Required |
Description |
type |
string |
true |
|
geometries |
Geometry[ ] |
true |
|
RegionProperties
Name |
Type |
Required |
Description |
region_name |
string |
false |
|
vegetation_type |
integer |
false |
|
sprinkler_type |
integer |
false |
|
shade_level |
integer |
false |
|
newly_planted |
boolean |
false |
|
local_region_id |
integer |
false |
|
remote_region_id |
string |
false |
|
images |
ImageInfo[ ] |
false |
|
controller_serial |
string |
false |
|
emitter_count |
integer |
false |
|
plant_area |
number |
false |
|
zone_id |
string |
false |
|
project_id |
string |
true |
|
created_at |
integer |
false |
|
updated_at |
integer |
false |
|
plant_center_coordinates |
number[ ] |
false |
|
status |
string |
false |
|
parcel_id |
string |
false |
|
parcelExists |
boolean |
false |
|
elevation |
number |
false |
|
plantFactor |
number[ ] |
false |
|
onlyVegetation |
boolean |
false |
|
extendAreaRegionType |
boolean |
false |
|
version |
integer |
false |
|
controllerId |
string |
false |
|
areaId |
string |
false |
|
ImageInfo
Name |
Type |
Required |
Description |
image_id |
string |
true |
|
imageFormat |
string |
false |
|
width |
integer |
false |
|
height |
integer |
false |
|
thumbnails |
ImageInfo[ ] |
false |
|
updatedAt |
integer |
false |
|
imageType |
integer |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
404 |
Not Found |
409 |
Conflict |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
type |
string |
true |
|
geometry |
Geometry |
false |
|
properties |
RegionProperties |
false |
|
Geometry is any of: Point, MultiPoint, LineString, MultiLineString, Polygon, MultiPolygon, GeometryCollection
Point
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ] |
true |
|
properties |
Object for field properties |
false |
|
Object for field properties
Name |
Type |
Required |
Description |
* |
string |
false |
|
MultiPoint
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
LineString
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
MultiLineString
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
Polygon
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
MultiPolygon
Name |
Type |
Required |
Description |
type |
string |
true |
|
coordinates |
number[ ][ ][ ][ ] |
true |
|
properties |
Object for field properties |
false |
|
GeometryCollection
Name |
Type |
Required |
Description |
type |
string |
true |
|
geometries |
Geometry[ ] |
true |
|
RegionProperties
Name |
Type |
Required |
Description |
region_name |
string |
false |
|
vegetation_type |
integer |
false |
|
sprinkler_type |
integer |
false |
|
shade_level |
integer |
false |
|
newly_planted |
boolean |
false |
|
local_region_id |
integer |
false |
|
remote_region_id |
string |
false |
|
images |
ImageInfo[ ] |
false |
|
controller_serial |
string |
false |
|
emitter_count |
integer |
false |
|
plant_area |
number |
false |
|
zone_id |
string |
false |
|
project_id |
string |
true |
|
created_at |
integer |
false |
|
updated_at |
integer |
false |
|
plant_center_coordinates |
number[ ] |
false |
|
status |
string |
false |
|
parcel_id |
string |
false |
|
parcelExists |
boolean |
false |
|
elevation |
number |
false |
|
plantFactor |
number[ ] |
false |
|
onlyVegetation |
boolean |
false |
|
extendAreaRegionType |
boolean |
false |
|
version |
integer |
false |
|
controllerId |
string |
false |
|
areaId |
string |
false |
|
ImageInfo
Name |
Type |
Required |
Description |
image_id |
string |
true |
|
imageFormat |
string |
false |
|
width |
integer |
false |
|
height |
integer |
false |
|
thumbnails |
ImageInfo[ ] |
false |
|
updatedAt |
integer |
false |
|
imageType |
integer |
false |
|
V1 | Reports | CCU Alerts Report
Create CCU alerts report
Request
curl "https://developer-api.etwater.com/api/v1/reports/ccu-alerts-report?startDate=example+string+value&endDate=example+string+value&interval=example+string+value" \
-X POST \
-H "Authorization: example string value" \
--data "{
\"filters\": {
\"projectControllers\": {
\"projectControllers\": [
{
\"projectId\": \"example string value\",
\"controllerIdList\": [
\"example string value\"
]
}
]
},
\"alertCodes\": {
\"codes\": [
641
]
},
\"period\": {
\"startDate\": \"example string value\",
\"endDate\": \"example string value\",
\"interval\": \"MONTH\"
}
},
\"meta\": {
\"example_property_name\": \"example string value\"
}
}"
On success, the above request returns response like
{
"id": "example string value",
"userId": "example string value",
"data": {
"total": {
"totalAlertsCount": 364,
"affectedProjectsCount": 422,
"affectedControllersCount": 90,
"projects": [
{
"projectId": "example string value",
"name": "example string value",
"ccuAlertsStats": [
{
"alertCode": 310,
"alertCodeName": "example string value",
"totalAlertsCount": 798
}
],
"controllers": [
{
"controllerId": "example string value",
"name": "example string value",
"ccuAlertsStats": [
{
"alertCode": 693,
"alertCodeName": "example string value",
"totalAlertsCount": 944
}
],
"zones": [
{
"zoneId": "example string value",
"zoneNumber": 848,
"name": "example string value",
"ccuAlertsStats": [
{
"alertCode": 833,
"alertCodeName": "example string value",
"totalAlertsCount": 439
}
],
"ccuAlerts": [
{
"userId": "example string value",
"userName": "example string value",
"projectId": "example string value",
"projectName": "example string value",
"controllerId": "example string value",
"controllerName": "example string value",
"zoneId": "example string value",
"zoneName": "example string value",
"zoneNumber": 0,
"alertCode": 10,
"alertCodeName": "example string value",
"date": "example string value"
}
]
}
]
}
]
}
]
},
"buckets": [
{
"dateStart": "example string value",
"dateEnd": "example string value",
"totalAlertsCount": 469,
"projects": [
{
"projectId": "example string value",
"name": "example string value",
"ccuAlertsStats": [
{
"alertCode": 815,
"alertCodeName": "example string value",
"totalAlertsCount": 361
}
],
"controllers": [
{
"controllerId": "example string value",
"name": "example string value",
"ccuAlertsStats": [
{
"alertCode": 158,
"alertCodeName": "example string value",
"totalAlertsCount": 986
}
],
"zones": [
{
"zoneId": "example string value",
"zoneNumber": 78,
"name": "example string value",
"ccuAlertsStats": [
{
"alertCode": 113,
"alertCodeName": "example string value",
"totalAlertsCount": 614
}
],
"ccuAlerts": [
{
"userId": "example string value",
"userName": "example string value",
"projectId": "example string value",
"projectName": "example string value",
"controllerId": "example string value",
"controllerName": "example string value",
"zoneId": "example string value",
"zoneName": "example string value",
"zoneNumber": 953,
"alertCode": 937,
"alertCodeName": "example string value",
"date": "example string value"
}
]
}
]
}
]
}
]
}
],
"bucketsTotal": [
{
"dateStart": "example string value",
"dateEnd": "example string value",
"totalAlertsCount": 620,
"affectedProjectsCount": 877,
"affectedControllersCount": 29
}
]
},
"filters": {
"projectControllers": {
"projectControllers": [
{
"projectId": "example string value",
"controllerIdList": [
"example string value"
]
}
]
},
"alertCodes": {
"codes": [
62
]
},
"period": {
"startDate": "example string value",
"endDate": "example string value",
"interval": "BILLING_PERIOD"
}
},
"meta": {
"example_property_name": "example string value"
},
"storedDataUrl": "example string value"
}
HTTP Request
POST /api/v1/reports/ccu-alerts-report
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
startDate |
string |
query |
true |
|
endDate |
string |
query |
true |
|
interval |
string |
query |
false |
|
CcuAlertsReportCreateRequest |
CcuAlertsReportCreateRequest |
body |
true |
|
CcuAlertsReportCreateRequest
Name |
Type |
Required |
Description |
filters |
ReportFilters |
true |
|
meta |
Object for field meta |
false |
|
ReportFilters
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersFilter |
false |
|
alertCodes |
AlertCodesFilter |
false |
|
period |
PeriodFilter |
true |
|
ProjectControllersFilter
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersValue[ ] |
true |
|
ProjectControllersValue
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
controllerIdList |
string[ ] |
false |
|
AlertCodesFilter
Name |
Type |
Required |
Description |
codes |
integer[ ] |
true |
|
PeriodFilter
Name |
Type |
Required |
Description |
startDate |
string |
true |
|
endDate |
string |
true |
|
interval |
string |
true |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
true |
|
userId |
string |
true |
|
data |
ReportData |
false |
|
filters |
ReportFilters |
true |
|
meta |
Object for field meta |
false |
|
storedDataUrl |
string |
false |
|
ReportData
Name |
Type |
Required |
Description |
total |
Total |
true |
|
buckets |
Bucket[ ] |
true |
|
bucketsTotal |
BucketTotal[ ] |
true |
|
Total
Name |
Type |
Required |
Description |
totalAlertsCount |
integer |
true |
|
affectedProjectsCount |
integer |
true |
|
affectedControllersCount |
integer |
true |
|
projects |
Project[ ] |
true |
|
Project
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
name |
string |
true |
|
ccuAlertsStats |
CcuAlertsStatsItem[ ] |
true |
|
controllers |
Controller[ ] |
true |
|
CcuAlertsStatsItem
Name |
Type |
Required |
Description |
alertCode |
integer |
true |
|
alertCodeName |
string |
true |
|
totalAlertsCount |
integer |
true |
|
Controller
Name |
Type |
Required |
Description |
controllerId |
string |
true |
|
name |
string |
true |
|
ccuAlertsStats |
CcuAlertsStatsItem[ ] |
true |
|
zones |
Zone[ ] |
true |
|
Zone
Name |
Type |
Required |
Description |
zoneId |
string |
true |
|
zoneNumber |
integer |
true |
|
name |
string |
true |
|
ccuAlertsStats |
CcuAlertsStatsItem[ ] |
true |
|
ccuAlerts |
CcuAlert[ ] |
true |
|
CcuAlert
Name |
Type |
Required |
Description |
userId |
string |
true |
|
userName |
string |
true |
|
projectId |
string |
true |
|
projectName |
string |
true |
|
controllerId |
string |
true |
|
controllerName |
string |
true |
|
zoneId |
string |
true |
|
zoneName |
string |
true |
|
zoneNumber |
integer |
true |
|
alertCode |
integer |
true |
|
alertCodeName |
string |
true |
|
date |
string |
true |
|
Bucket
Name |
Type |
Required |
Description |
dateStart |
string |
true |
|
dateEnd |
string |
true |
|
totalAlertsCount |
integer |
true |
|
projects |
Project[ ] |
true |
|
BucketTotal
Name |
Type |
Required |
Description |
dateStart |
string |
true |
|
dateEnd |
string |
true |
|
totalAlertsCount |
integer |
true |
|
affectedProjectsCount |
integer |
true |
|
affectedControllersCount |
integer |
true |
|
ReportFilters
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersFilter |
false |
|
alertCodes |
AlertCodesFilter |
false |
|
period |
PeriodFilter |
true |
|
ProjectControllersFilter
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersValue[ ] |
true |
|
ProjectControllersValue
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
controllerIdList |
string[ ] |
false |
|
AlertCodesFilter
Name |
Type |
Required |
Description |
codes |
integer[ ] |
true |
|
PeriodFilter
Name |
Type |
Required |
Description |
startDate |
string |
true |
|
endDate |
string |
true |
|
interval |
string |
true |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
Get CCU alerts report
Request
curl "https://developer-api.etwater.com/api/v1/reports/ccu-alerts-report/{id}" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"id": "example string value",
"userId": "example string value",
"filters": {
"projectControllers": {
"projectControllers": [
{
"projectId": "example string value",
"controllerIdList": [
"example string value"
]
}
]
},
"alertCodes": {
"codes": [
46
]
},
"period": {
"startDate": "example string value",
"endDate": "example string value",
"interval": "BILLING_PERIOD"
}
},
"data": {
"total": {
"totalAlertsCount": 981,
"affectedProjectsCount": 35,
"affectedControllersCount": 430,
"projects": [
{
"projectId": "example string value",
"name": "example string value",
"ccuAlertsStats": [
{
"alertCode": 319,
"alertCodeName": "example string value",
"totalAlertsCount": 106
}
],
"controllers": [
{
"controllerId": "example string value",
"name": "example string value",
"ccuAlertsStats": [
{
"alertCode": 440,
"alertCodeName": "example string value",
"totalAlertsCount": 469
}
],
"zones": [
{
"zoneId": "example string value",
"zoneNumber": 111,
"name": "example string value",
"ccuAlertsStats": [
{
"alertCode": 498,
"alertCodeName": "example string value",
"totalAlertsCount": 216
}
],
"ccuAlerts": [
{
"userId": "example string value",
"userName": "example string value",
"projectId": "example string value",
"projectName": "example string value",
"controllerId": "example string value",
"controllerName": "example string value",
"zoneId": "example string value",
"zoneName": "example string value",
"zoneNumber": 649,
"alertCode": 354,
"alertCodeName": "example string value",
"date": "example string value"
}
]
}
]
}
]
}
]
},
"buckets": [
{
"dateStart": "example string value",
"dateEnd": "example string value",
"totalAlertsCount": 907,
"projects": [
{
"projectId": "example string value",
"name": "example string value",
"ccuAlertsStats": [
{
"alertCode": 340,
"alertCodeName": "example string value",
"totalAlertsCount": 182
}
],
"controllers": [
{
"controllerId": "example string value",
"name": "example string value",
"ccuAlertsStats": [
{
"alertCode": 655,
"alertCodeName": "example string value",
"totalAlertsCount": 374
}
],
"zones": [
{
"zoneId": "example string value",
"zoneNumber": 117,
"name": "example string value",
"ccuAlertsStats": [
{
"alertCode": 472,
"alertCodeName": "example string value",
"totalAlertsCount": 827
}
],
"ccuAlerts": [
{
"userId": "example string value",
"userName": "example string value",
"projectId": "example string value",
"projectName": "example string value",
"controllerId": "example string value",
"controllerName": "example string value",
"zoneId": "example string value",
"zoneName": "example string value",
"zoneNumber": 130,
"alertCode": 280,
"alertCodeName": "example string value",
"date": "example string value"
}
]
}
]
}
]
}
]
}
],
"bucketsTotal": [
{
"dateStart": "example string value",
"dateEnd": "example string value",
"totalAlertsCount": 190,
"affectedProjectsCount": 153,
"affectedControllersCount": 331
}
]
},
"meta": {
"example_property_name": "example string value"
},
"storedDataUrl": "example string value"
}
HTTP Request
GET /api/v1/reports/ccu-alerts-report/{id}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
id |
string |
path |
true |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
true |
|
userId |
string |
true |
|
filters |
ReportFilters |
true |
|
data |
ReportData |
false |
|
meta |
Object for field meta |
false |
|
storedDataUrl |
string |
false |
|
ReportFilters
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersFilter |
false |
|
alertCodes |
AlertCodesFilter |
false |
|
period |
PeriodFilter |
true |
|
ProjectControllersFilter
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersValue[ ] |
true |
|
ProjectControllersValue
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
controllerIdList |
string[ ] |
false |
|
AlertCodesFilter
Name |
Type |
Required |
Description |
codes |
integer[ ] |
true |
|
PeriodFilter
Name |
Type |
Required |
Description |
startDate |
string |
true |
|
endDate |
string |
true |
|
interval |
string |
true |
|
ReportData
Name |
Type |
Required |
Description |
total |
Total |
true |
|
buckets |
Bucket[ ] |
true |
|
bucketsTotal |
BucketTotal[ ] |
true |
|
Total
Name |
Type |
Required |
Description |
totalAlertsCount |
integer |
true |
|
affectedProjectsCount |
integer |
true |
|
affectedControllersCount |
integer |
true |
|
projects |
Project[ ] |
true |
|
Project
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
name |
string |
true |
|
ccuAlertsStats |
CcuAlertsStatsItem[ ] |
true |
|
controllers |
Controller[ ] |
true |
|
CcuAlertsStatsItem
Name |
Type |
Required |
Description |
alertCode |
integer |
true |
|
alertCodeName |
string |
true |
|
totalAlertsCount |
integer |
true |
|
Controller
Name |
Type |
Required |
Description |
controllerId |
string |
true |
|
name |
string |
true |
|
ccuAlertsStats |
CcuAlertsStatsItem[ ] |
true |
|
zones |
Zone[ ] |
true |
|
Zone
Name |
Type |
Required |
Description |
zoneId |
string |
true |
|
zoneNumber |
integer |
true |
|
name |
string |
true |
|
ccuAlertsStats |
CcuAlertsStatsItem[ ] |
true |
|
ccuAlerts |
CcuAlert[ ] |
true |
|
CcuAlert
Name |
Type |
Required |
Description |
userId |
string |
true |
|
userName |
string |
true |
|
projectId |
string |
true |
|
projectName |
string |
true |
|
controllerId |
string |
true |
|
controllerName |
string |
true |
|
zoneId |
string |
true |
|
zoneName |
string |
true |
|
zoneNumber |
integer |
true |
|
alertCode |
integer |
true |
|
alertCodeName |
string |
true |
|
date |
string |
true |
|
Bucket
Name |
Type |
Required |
Description |
dateStart |
string |
true |
|
dateEnd |
string |
true |
|
totalAlertsCount |
integer |
true |
|
projects |
Project[ ] |
true |
|
BucketTotal
Name |
Type |
Required |
Description |
dateStart |
string |
true |
|
dateEnd |
string |
true |
|
totalAlertsCount |
integer |
true |
|
affectedProjectsCount |
integer |
true |
|
affectedControllersCount |
integer |
true |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
V1 | Reports | Estimated Water Cost Report
Validate estimated water cost report
Request
curl "https://developer-api.etwater.com/api/v1/reports/estimated-water-cost-report/validate" \
-X POST \
-H "Authorization: example string value" \
--data "{
\"projectControllers\": {
\"projectControllers\": [
{
\"projectId\": \"example string value\",
\"controllerIdList\": [
\"example string value\"
]
}
]
}
}"
On success, the above request returns response like
{
"projectsFlowRates": [
{
"projectId": "example string value",
"projectName": "example string value",
"controllers": [
{
"controllerId": "example string value",
"name": "example string value",
"zones": [
{
"zoneId": "example string value",
"number": 498,
"name": "example string value",
"flowRateGpm": 950.8971171224943
}
]
}
]
}
]
}
HTTP Request
POST /api/v1/reports/estimated-water-cost-report/validate
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
EstimatedWaterCostReportValidateRequest |
EstimatedWaterCostReportValidateRequest |
body |
true |
|
EstimatedWaterCostReportValidateRequest
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersFilter |
true |
|
ProjectControllersFilter
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersValue[ ] |
true |
|
ProjectControllersValue
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
controllerIdList |
string[ ] |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
projectsFlowRates |
ProjectFlowRate[ ] |
true |
|
ProjectFlowRate
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
projectName |
string |
true |
|
controllers |
Controller[ ] |
true |
|
Controller
Name |
Type |
Required |
Description |
controllerId |
string |
true |
|
name |
string |
true |
|
zones |
Zone[ ] |
true |
|
Zone
Name |
Type |
Required |
Description |
zoneId |
string |
true |
|
number |
integer |
true |
|
name |
string |
true |
|
flowRateGpm |
number |
false |
|
Create estimated water cost report
Request
curl "https://developer-api.etwater.com/api/v1/reports/estimated-water-cost-report?startDate=example+string+value&endDate=example+string+value&interval=DAY&measureUnits=example+string+value" \
-X POST \
-H "Authorization: example string value" \
--data "{
\"filters\": {
\"projectControllers\": {
\"projectControllers\": [
{
\"projectId\": \"example string value\",
\"controllerIdList\": [
\"example string value\"
]
}
]
},
\"measureUnits\": {
\"units\": \"GALLONS\"
},
\"period\": {
\"startDate\": \"example string value\",
\"endDate\": \"example string value\",
\"interval\": \"BILLING_PERIOD\"
}
},
\"projectsFlowRates\": [
{
\"projectId\": \"example string value\",
\"projectName\": \"example string value\",
\"controllers\": [
{
\"controllerId\": \"example string value\",
\"name\": \"example string value\",
\"zones\": [
{
\"zoneId\": \"example string value\",
\"number\": 583,
\"name\": \"example string value\",
\"flowRateGpm\": 304
}
]
}
]
}
],
\"meta\": {
\"example_property_name\": \"example string value\"
}
}"
On success, the above request returns response like
{
"id": "example string value",
"status": "DONE",
"userId": "example string value",
"data": {
"total": {
"dateStart": "example string value",
"dateEnd": "example string value",
"waterUsage": 312.37615845742454,
"waterUsageCost": 170,
"projects": [
{
"projectId": "example string value",
"name": "example string value",
"waterUsage": 711,
"waterUsageCost": 73.4982779591802,
"controllers": [
{
"controllerId": "example string value",
"name": "example string value",
"waterUsage": 895,
"waterUsageCost": 226,
"zones": [
{
"zoneNumber": 590,
"name": "example string value",
"waterUsage": 684.6271016097753,
"waterUsageCost": 697,
"scheduledWaterUsage": 883,
"scheduledWaterUsageCost": 799,
"manualWaterUsage": 236,
"manualWaterUsageCost": 596.705418357954
}
],
"scheduledWaterUsage": 724,
"scheduledWaterUsageCost": 337,
"manualWaterUsage": 676,
"manualWaterUsageCost": 739
}
],
"scheduledWaterUsage": 202,
"scheduledWaterUsageCost": 398,
"manualWaterUsage": 238,
"manualWaterUsageCost": 846
}
],
"scheduledWaterUsage": 31,
"scheduledWaterUsageCost": 25.955021393464424,
"manualWaterUsage": 877,
"manualWaterUsageCost": 311.0744987200361
},
"buckets": [
{
"dateStart": "example string value",
"dateEnd": "example string value",
"waterUsage": 770.4268567126369,
"waterUsageCost": 653,
"projects": [
{
"projectId": "example string value",
"name": "example string value",
"waterUsage": 63,
"waterUsageCost": 217.30632857294117,
"controllers": [
{
"controllerId": "example string value",
"name": "example string value",
"waterUsage": 91,
"waterUsageCost": 237,
"zones": [
{
"zoneNumber": 679,
"name": "example string value",
"waterUsage": 340,
"waterUsageCost": 527.5832687167373,
"scheduledWaterUsage": 686.8446696022733,
"scheduledWaterUsageCost": 308,
"manualWaterUsage": 757.3752742062208,
"manualWaterUsageCost": 227.97423798030906
}
],
"scheduledWaterUsage": 936,
"scheduledWaterUsageCost": 636,
"manualWaterUsage": 453.0053993002537,
"manualWaterUsageCost": 80.7200656648353
}
],
"scheduledWaterUsage": 128.02755186708066,
"scheduledWaterUsageCost": 451,
"manualWaterUsage": 813.5753412794206,
"manualWaterUsageCost": 152
}
],
"scheduledWaterUsage": 73,
"scheduledWaterUsageCost": 153.28951326817716,
"manualWaterUsage": 416,
"manualWaterUsageCost": 791.3905534853184
}
],
"bucketsTotal": [
{
"dateStart": "example string value",
"dateEnd": "example string value",
"waterUsage": 783.7765304296169,
"waterUsageCost": 32.06751636791393,
"scheduledWaterUsage": 540.1921014022977,
"scheduledWaterUsageCost": 243,
"manualWaterUsage": 337,
"manualWaterUsageCost": 397.7750928130816
}
],
"projectsFlowRates": [
{
"projectId": "example string value",
"projectName": "example string value",
"controllers": [
{
"controllerId": "example string value",
"name": "example string value",
"zones": [
{
"zoneId": "example string value",
"number": 220,
"name": "example string value",
"flowRateGpm": 529
}
]
}
]
}
]
},
"filters": {
"projectControllers": {
"projectControllers": [
{
"projectId": "example string value",
"controllerIdList": [
"example string value"
]
}
]
},
"measureUnits": {
"units": "GALLONS"
},
"period": {
"startDate": "example string value",
"endDate": "example string value",
"interval": "MONTH"
}
},
"meta": {
"example_property_name": "example string value"
},
"storedDataUrl": "example string value"
}
HTTP Request
POST /api/v1/reports/estimated-water-cost-report
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
startDate |
string |
query |
true |
|
endDate |
string |
query |
true |
|
interval |
string |
query |
false |
|
measureUnits |
string |
query |
false |
|
EstimatedWaterCostReportCreateRequest |
EstimatedWaterCostReportCreateRequest |
body |
true |
|
EstimatedWaterCostReportCreateRequest
Name |
Type |
Required |
Description |
filters |
ReportFilters |
true |
|
projectsFlowRates |
ProjectFlowRate[ ] |
false |
|
meta |
Object for field meta |
false |
|
ReportFilters
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersFilter |
false |
|
measureUnits |
WateringMeasureUnitsFilter |
true |
|
period |
PeriodFilter |
true |
|
ProjectControllersFilter
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersValue[ ] |
true |
|
ProjectControllersValue
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
controllerIdList |
string[ ] |
false |
|
WateringMeasureUnitsFilter
Name |
Type |
Required |
Description |
units |
string |
true |
|
PeriodFilter
Name |
Type |
Required |
Description |
startDate |
string |
true |
|
endDate |
string |
true |
|
interval |
string |
true |
|
ProjectFlowRate
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
projectName |
string |
true |
|
controllers |
Controller[ ] |
true |
|
Controller
Name |
Type |
Required |
Description |
controllerId |
string |
true |
|
name |
string |
true |
|
zones |
Zone[ ] |
true |
|
Zone
Name |
Type |
Required |
Description |
zoneId |
string |
true |
|
number |
integer |
true |
|
name |
string |
true |
|
flowRateGpm |
number |
false |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
false |
|
status |
string |
true |
|
userId |
string |
true |
|
data |
ReportData |
false |
|
filters |
ReportFilters |
true |
|
meta |
Object for field meta |
false |
|
storedDataUrl |
string |
false |
|
ReportData
Name |
Type |
Required |
Description |
total |
Bucket |
true |
|
buckets |
Bucket[ ] |
true |
|
bucketsTotal |
BucketTotal[ ] |
true |
|
projectsFlowRates |
ProjectFlowRate[ ] |
true |
|
Bucket
Name |
Type |
Required |
Description |
dateStart |
string |
true |
|
dateEnd |
string |
true |
|
waterUsage |
number |
true |
|
waterUsageCost |
number |
true |
|
projects |
ReportProject[ ] |
true |
|
scheduledWaterUsage |
number |
false |
|
scheduledWaterUsageCost |
number |
false |
|
manualWaterUsage |
number |
false |
|
manualWaterUsageCost |
number |
false |
|
ReportProject
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
name |
string |
true |
|
waterUsage |
number |
true |
|
waterUsageCost |
number |
true |
|
controllers |
ReportController[ ] |
true |
|
scheduledWaterUsage |
number |
false |
|
scheduledWaterUsageCost |
number |
false |
|
manualWaterUsage |
number |
false |
|
manualWaterUsageCost |
number |
false |
|
ReportController
Name |
Type |
Required |
Description |
controllerId |
string |
true |
|
name |
string |
true |
|
waterUsage |
number |
true |
|
waterUsageCost |
number |
true |
|
zones |
ReportZone[ ] |
true |
|
scheduledWaterUsage |
number |
false |
|
scheduledWaterUsageCost |
number |
false |
|
manualWaterUsage |
number |
false |
|
manualWaterUsageCost |
number |
false |
|
ReportZone
Name |
Type |
Required |
Description |
zoneNumber |
integer |
true |
|
name |
string |
true |
|
waterUsage |
number |
true |
|
waterUsageCost |
number |
true |
|
scheduledWaterUsage |
number |
false |
|
scheduledWaterUsageCost |
number |
false |
|
manualWaterUsage |
number |
false |
|
manualWaterUsageCost |
number |
false |
|
BucketTotal
Name |
Type |
Required |
Description |
dateStart |
string |
true |
|
dateEnd |
string |
true |
|
waterUsage |
number |
true |
|
waterUsageCost |
number |
true |
|
scheduledWaterUsage |
number |
false |
|
scheduledWaterUsageCost |
number |
false |
|
manualWaterUsage |
number |
false |
|
manualWaterUsageCost |
number |
false |
|
ProjectFlowRate
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
projectName |
string |
true |
|
controllers |
Controller[ ] |
true |
|
Controller
Name |
Type |
Required |
Description |
controllerId |
string |
true |
|
name |
string |
true |
|
zones |
Zone[ ] |
true |
|
Zone
Name |
Type |
Required |
Description |
zoneId |
string |
true |
|
number |
integer |
true |
|
name |
string |
true |
|
flowRateGpm |
number |
false |
|
ReportFilters
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersFilter |
false |
|
measureUnits |
WateringMeasureUnitsFilter |
true |
|
period |
PeriodFilter |
true |
|
ProjectControllersFilter
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersValue[ ] |
true |
|
ProjectControllersValue
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
controllerIdList |
string[ ] |
false |
|
WateringMeasureUnitsFilter
Name |
Type |
Required |
Description |
units |
string |
true |
|
PeriodFilter
Name |
Type |
Required |
Description |
startDate |
string |
true |
|
endDate |
string |
true |
|
interval |
string |
true |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
Get estimated water cost report
Request
curl "https://developer-api.etwater.com/api/v1/reports/estimated-water-cost-report/{id}" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"id": "example string value",
"status": "UNPROCESSABLE",
"userId": "example string value",
"data": {
"total": {
"dateStart": "example string value",
"dateEnd": "example string value",
"waterUsage": 139.96882044708767,
"waterUsageCost": 569,
"projects": [
{
"projectId": "example string value",
"name": "example string value",
"waterUsage": 127,
"waterUsageCost": 703.9593629091789,
"controllers": [
{
"controllerId": "example string value",
"name": "example string value",
"waterUsage": 833,
"waterUsageCost": 847.3431057517153,
"zones": [
{
"zoneNumber": 80,
"name": "example string value",
"waterUsage": 135.3042293970027,
"waterUsageCost": 755.89426781791,
"scheduledWaterUsage": 493,
"scheduledWaterUsageCost": 646.6016949371442,
"manualWaterUsage": 162.37209791428043,
"manualWaterUsageCost": 695.2547797445463
}
],
"scheduledWaterUsage": 930.7485613649471,
"scheduledWaterUsageCost": 527.0319276149534,
"manualWaterUsage": 960,
"manualWaterUsageCost": 250
}
],
"scheduledWaterUsage": 214.36984521074677,
"scheduledWaterUsageCost": 307,
"manualWaterUsage": 541,
"manualWaterUsageCost": 346.5626367118967
}
],
"scheduledWaterUsage": 557,
"scheduledWaterUsageCost": 925.191876443658,
"manualWaterUsage": 799.844865593987,
"manualWaterUsageCost": 293
},
"buckets": [
{
"dateStart": "example string value",
"dateEnd": "example string value",
"waterUsage": 24.122951097843682,
"waterUsageCost": 309,
"projects": [
{
"projectId": "example string value",
"name": "example string value",
"waterUsage": 156,
"waterUsageCost": 653,
"controllers": [
{
"controllerId": "example string value",
"name": "example string value",
"waterUsage": 152.5059696997078,
"waterUsageCost": 433.2002594290302,
"zones": [
{
"zoneNumber": 829,
"name": "example string value",
"waterUsage": 245.0100789987529,
"waterUsageCost": 532,
"scheduledWaterUsage": 677.769903874849,
"scheduledWaterUsageCost": 162,
"manualWaterUsage": 870.7537110293068,
"manualWaterUsageCost": 244.9985571415157
}
],
"scheduledWaterUsage": 668,
"scheduledWaterUsageCost": 860,
"manualWaterUsage": 458,
"manualWaterUsageCost": 435.8293220567653
}
],
"scheduledWaterUsage": 765,
"scheduledWaterUsageCost": 951,
"manualWaterUsage": 682,
"manualWaterUsageCost": 730.798487891815
}
],
"scheduledWaterUsage": 91,
"scheduledWaterUsageCost": 87,
"manualWaterUsage": 576.0086381696204,
"manualWaterUsageCost": 577
}
],
"bucketsTotal": [
{
"dateStart": "example string value",
"dateEnd": "example string value",
"waterUsage": 156,
"waterUsageCost": 324,
"scheduledWaterUsage": 920,
"scheduledWaterUsageCost": 117.68363142278214,
"manualWaterUsage": 853.431160493489,
"manualWaterUsageCost": 290
}
],
"projectsFlowRates": [
{
"projectId": "example string value",
"projectName": "example string value",
"controllers": [
{
"controllerId": "example string value",
"name": "example string value",
"zones": [
{
"zoneId": "example string value",
"number": 215,
"name": "example string value",
"flowRateGpm": 181.74249780445476
}
]
}
]
}
]
},
"filters": {
"projectControllers": {
"projectControllers": [
{
"projectId": "example string value",
"controllerIdList": [
"example string value"
]
}
]
},
"measureUnits": {
"units": "GALLONS"
},
"period": {
"startDate": "example string value",
"endDate": "example string value",
"interval": "BILLING_PERIOD"
}
},
"meta": {
"example_property_name": "example string value"
},
"storedDataUrl": "example string value"
}
HTTP Request
GET /api/v1/reports/estimated-water-cost-report/{id}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
id |
string |
path |
true |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
true |
|
status |
string |
true |
|
userId |
string |
true |
|
data |
ReportData |
false |
|
filters |
ReportFilters |
true |
|
meta |
Object for field meta |
false |
|
storedDataUrl |
string |
false |
|
ReportData
Name |
Type |
Required |
Description |
total |
Bucket |
true |
|
buckets |
Bucket[ ] |
true |
|
bucketsTotal |
BucketTotal[ ] |
true |
|
projectsFlowRates |
ProjectFlowRate[ ] |
true |
|
Bucket
Name |
Type |
Required |
Description |
dateStart |
string |
true |
|
dateEnd |
string |
true |
|
waterUsage |
number |
true |
|
waterUsageCost |
number |
true |
|
projects |
ReportProject[ ] |
true |
|
scheduledWaterUsage |
number |
false |
|
scheduledWaterUsageCost |
number |
false |
|
manualWaterUsage |
number |
false |
|
manualWaterUsageCost |
number |
false |
|
ReportProject
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
name |
string |
true |
|
waterUsage |
number |
true |
|
waterUsageCost |
number |
true |
|
controllers |
ReportController[ ] |
true |
|
scheduledWaterUsage |
number |
false |
|
scheduledWaterUsageCost |
number |
false |
|
manualWaterUsage |
number |
false |
|
manualWaterUsageCost |
number |
false |
|
ReportController
Name |
Type |
Required |
Description |
controllerId |
string |
true |
|
name |
string |
true |
|
waterUsage |
number |
true |
|
waterUsageCost |
number |
true |
|
zones |
ReportZone[ ] |
true |
|
scheduledWaterUsage |
number |
false |
|
scheduledWaterUsageCost |
number |
false |
|
manualWaterUsage |
number |
false |
|
manualWaterUsageCost |
number |
false |
|
ReportZone
Name |
Type |
Required |
Description |
zoneNumber |
integer |
true |
|
name |
string |
true |
|
waterUsage |
number |
true |
|
waterUsageCost |
number |
true |
|
scheduledWaterUsage |
number |
false |
|
scheduledWaterUsageCost |
number |
false |
|
manualWaterUsage |
number |
false |
|
manualWaterUsageCost |
number |
false |
|
BucketTotal
Name |
Type |
Required |
Description |
dateStart |
string |
true |
|
dateEnd |
string |
true |
|
waterUsage |
number |
true |
|
waterUsageCost |
number |
true |
|
scheduledWaterUsage |
number |
false |
|
scheduledWaterUsageCost |
number |
false |
|
manualWaterUsage |
number |
false |
|
manualWaterUsageCost |
number |
false |
|
ProjectFlowRate
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
projectName |
string |
true |
|
controllers |
Controller[ ] |
true |
|
Controller
Name |
Type |
Required |
Description |
controllerId |
string |
true |
|
name |
string |
true |
|
zones |
Zone[ ] |
true |
|
Zone
Name |
Type |
Required |
Description |
zoneId |
string |
true |
|
number |
integer |
true |
|
name |
string |
true |
|
flowRateGpm |
number |
false |
|
ReportFilters
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersFilter |
false |
|
measureUnits |
WateringMeasureUnitsFilter |
true |
|
period |
PeriodFilter |
true |
|
ProjectControllersFilter
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersValue[ ] |
true |
|
ProjectControllersValue
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
controllerIdList |
string[ ] |
false |
|
WateringMeasureUnitsFilter
Name |
Type |
Required |
Description |
units |
string |
true |
|
PeriodFilter
Name |
Type |
Required |
Description |
startDate |
string |
true |
|
endDate |
string |
true |
|
interval |
string |
true |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
V1 | Reports | Estimated Water Usage Report
Validate estimated water usage report
Request
curl "https://developer-api.etwater.com/api/v1/reports/estimated-water-usage-report/validate" \
-X POST \
-H "Authorization: example string value" \
--data "{
\"projectControllers\": {
\"projectControllers\": [
{
\"projectId\": \"example string value\",
\"controllerIdList\": [
\"example string value\"
]
}
]
}
}"
On success, the above request returns response like
{
"projectsFlowRates": [
{
"projectId": "example string value",
"projectName": "example string value",
"controllers": [
{
"controllerId": "example string value",
"name": "example string value",
"zones": [
{
"zoneId": "example string value",
"number": 111,
"name": "example string value",
"flowRateGpm": 504.24136291455545
}
]
}
]
}
]
}
HTTP Request
POST /api/v1/reports/estimated-water-usage-report/validate
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
EstimatedWaterUsageReportValidateRequest |
EstimatedWaterUsageReportValidateRequest |
body |
true |
|
EstimatedWaterUsageReportValidateRequest
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersFilter |
true |
|
ProjectControllersFilter
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersValue[ ] |
true |
|
ProjectControllersValue
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
controllerIdList |
string[ ] |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
projectsFlowRates |
ProjectFlowRate[ ] |
true |
|
ProjectFlowRate
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
projectName |
string |
true |
|
controllers |
Controller[ ] |
true |
|
Controller
Name |
Type |
Required |
Description |
controllerId |
string |
true |
|
name |
string |
true |
|
zones |
Zone[ ] |
true |
|
Zone
Name |
Type |
Required |
Description |
zoneId |
string |
true |
|
number |
integer |
true |
|
name |
string |
true |
|
flowRateGpm |
number |
false |
|
Create estimated water usage report
Request
curl "https://developer-api.etwater.com/api/v1/reports/estimated-water-usage-report?startDate=example+string+value&endDate=example+string+value&interval=MONTH&measureUnits=example+string+value" \
-X POST \
-H "Authorization: example string value" \
--data "{
\"filters\": {
\"projectControllers\": {
\"projectControllers\": [
{
\"projectId\": \"example string value\",
\"controllerIdList\": [
\"example string value\"
]
}
]
},
\"measureUnits\": {
\"units\": \"HCF\"
},
\"period\": {
\"startDate\": \"example string value\",
\"endDate\": \"example string value\",
\"interval\": \"DAY\"
}
},
\"projectsFlowRates\": [
{
\"projectId\": \"example string value\",
\"projectName\": \"example string value\",
\"controllers\": [
{
\"controllerId\": \"example string value\",
\"name\": \"example string value\",
\"zones\": [
{
\"zoneId\": \"example string value\",
\"number\": 656,
\"name\": \"example string value\",
\"flowRateGpm\": 20
}
]
}
]
}
],
\"meta\": {
\"example_property_name\": \"example string value\"
}
}"
On success, the above request returns response like
{
"id": "example string value",
"status": "IN_PROGRESS",
"filters": {
"projectControllers": {
"projectControllers": [
{
"projectId": "example string value",
"controllerIdList": [
"example string value"
]
}
]
},
"measureUnits": {
"units": "HCF"
},
"period": {
"startDate": "example string value",
"endDate": "example string value",
"interval": "BILLING_PERIOD"
}
},
"userId": "example string value",
"meta": {
"example_property_name": "example string value"
}
}
HTTP Request
POST /api/v1/reports/estimated-water-usage-report
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
startDate |
string |
query |
true |
|
endDate |
string |
query |
true |
|
interval |
string |
query |
false |
|
measureUnits |
string |
query |
false |
|
EstimatedWaterUsageReportCreateRequest |
EstimatedWaterUsageReportCreateRequest |
body |
true |
|
EstimatedWaterUsageReportCreateRequest
Name |
Type |
Required |
Description |
filters |
ReportFilters |
true |
|
projectsFlowRates |
ProjectFlowRate[ ] |
false |
|
meta |
Object for field meta |
false |
|
ReportFilters
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersFilter |
false |
|
measureUnits |
WateringMeasureUnitsFilter |
true |
|
period |
PeriodFilter |
true |
|
ProjectControllersFilter
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersValue[ ] |
true |
|
ProjectControllersValue
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
controllerIdList |
string[ ] |
false |
|
WateringMeasureUnitsFilter
Name |
Type |
Required |
Description |
units |
string |
true |
|
PeriodFilter
Name |
Type |
Required |
Description |
startDate |
string |
true |
|
endDate |
string |
true |
|
interval |
string |
true |
|
ProjectFlowRate
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
projectName |
string |
true |
|
controllers |
Controller[ ] |
true |
|
Controller
Name |
Type |
Required |
Description |
controllerId |
string |
true |
|
name |
string |
true |
|
zones |
Zone[ ] |
true |
|
Zone
Name |
Type |
Required |
Description |
zoneId |
string |
true |
|
number |
integer |
true |
|
name |
string |
true |
|
flowRateGpm |
number |
false |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
false |
|
status |
string |
true |
|
filters |
ReportFilters |
true |
|
userId |
string |
true |
|
meta |
Object for field meta |
false |
|
ReportFilters
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersFilter |
false |
|
measureUnits |
WateringMeasureUnitsFilter |
true |
|
period |
PeriodFilter |
true |
|
ProjectControllersFilter
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersValue[ ] |
true |
|
ProjectControllersValue
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
controllerIdList |
string[ ] |
false |
|
WateringMeasureUnitsFilter
Name |
Type |
Required |
Description |
units |
string |
true |
|
PeriodFilter
Name |
Type |
Required |
Description |
startDate |
string |
true |
|
endDate |
string |
true |
|
interval |
string |
true |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
Get estimated water usage report
Request
curl "https://developer-api.etwater.com/api/v1/reports/estimated-water-usage-report/{id}" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"id": "example string value",
"status": "UNPROCESSABLE",
"data": {
"total": {
"dateStart": "example string value",
"dateEnd": "example string value",
"waterUsage": 471,
"projects": [
{
"projectId": "example string value",
"name": "example string value",
"waterUsage": 236.41081258487458,
"controllers": [
{
"controllerId": "example string value",
"name": "example string value",
"waterUsage": 704,
"zones": [
{
"zoneId": "example string value",
"zoneNumber": 177,
"name": "example string value",
"waterUsage": 507.5218670570859,
"scheduledWaterUsage": 422,
"manualWaterUsage": 154
}
],
"scheduledWaterUsage": 891,
"manualWaterUsage": 156.87226511392382
}
],
"scheduledWaterUsage": 95,
"manualWaterUsage": 431
}
],
"scheduledWaterUsage": 159,
"manualWaterUsage": 66.70828446126929
},
"buckets": [
{
"dateStart": "example string value",
"dateEnd": "example string value",
"waterUsage": 867,
"projects": [
{
"projectId": "example string value",
"name": "example string value",
"waterUsage": 161,
"controllers": [
{
"controllerId": "example string value",
"name": "example string value",
"waterUsage": 226.46784094463467,
"zones": [
{
"zoneId": "example string value",
"zoneNumber": 913,
"name": "example string value",
"waterUsage": 999.4863737372152,
"scheduledWaterUsage": 627,
"manualWaterUsage": 445.3445796134624
}
],
"scheduledWaterUsage": 294,
"manualWaterUsage": 878
}
],
"scheduledWaterUsage": 190,
"manualWaterUsage": 686.3771661586952
}
],
"scheduledWaterUsage": 934.2398764259367,
"manualWaterUsage": 433.97974103408853
}
],
"bucketsTotal": [
{
"dateStart": "example string value",
"dateEnd": "example string value",
"waterUsage": 683,
"scheduledWaterUsage": 965.2744820179765,
"manualWaterUsage": 401
}
],
"projectsFlowRates": [
{
"projectId": "example string value",
"projectName": "example string value",
"controllers": [
{
"controllerId": "example string value",
"name": "example string value",
"zones": [
{
"zoneId": "example string value",
"number": 548,
"name": "example string value",
"flowRateGpm": 14
}
]
}
]
}
]
},
"filters": {
"projectControllers": {
"projectControllers": [
{
"projectId": "example string value",
"controllerIdList": [
"example string value"
]
}
]
},
"measureUnits": {
"units": "HCF"
},
"period": {
"startDate": "example string value",
"endDate": "example string value",
"interval": "DAY"
}
},
"userId": "example string value",
"meta": {
"example_property_name": "example string value"
},
"storedDataUrl": "example string value"
}
HTTP Request
GET /api/v1/reports/estimated-water-usage-report/{id}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
id |
string |
path |
true |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
true |
|
status |
string |
true |
|
data |
ReportData |
false |
|
filters |
ReportFilters |
true |
|
userId |
string |
true |
|
meta |
Object for field meta |
false |
|
storedDataUrl |
string |
false |
|
ReportData
Name |
Type |
Required |
Description |
total |
Bucket |
true |
|
buckets |
Bucket[ ] |
true |
|
bucketsTotal |
BucketTotal[ ] |
true |
|
projectsFlowRates |
ProjectFlowRate[ ] |
true |
|
Bucket
Name |
Type |
Required |
Description |
dateStart |
string |
true |
|
dateEnd |
string |
true |
|
waterUsage |
number |
true |
|
projects |
ReportProject[ ] |
true |
|
scheduledWaterUsage |
number |
false |
|
manualWaterUsage |
number |
false |
|
ReportProject
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
name |
string |
true |
|
waterUsage |
number |
true |
|
controllers |
ReportController[ ] |
true |
|
scheduledWaterUsage |
number |
false |
|
manualWaterUsage |
number |
false |
|
ReportController
Name |
Type |
Required |
Description |
controllerId |
string |
true |
|
name |
string |
true |
|
waterUsage |
number |
true |
|
zones |
ReportZone[ ] |
true |
|
scheduledWaterUsage |
number |
false |
|
manualWaterUsage |
number |
false |
|
ReportZone
Name |
Type |
Required |
Description |
zoneId |
string |
true |
|
zoneNumber |
integer |
true |
|
name |
string |
true |
|
waterUsage |
number |
true |
|
scheduledWaterUsage |
number |
false |
|
manualWaterUsage |
number |
false |
|
BucketTotal
Name |
Type |
Required |
Description |
dateStart |
string |
true |
|
dateEnd |
string |
true |
|
waterUsage |
number |
true |
|
scheduledWaterUsage |
number |
false |
|
manualWaterUsage |
number |
false |
|
ProjectFlowRate
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
projectName |
string |
true |
|
controllers |
Controller[ ] |
true |
|
Controller
Name |
Type |
Required |
Description |
controllerId |
string |
true |
|
name |
string |
true |
|
zones |
Zone[ ] |
true |
|
Zone
Name |
Type |
Required |
Description |
zoneId |
string |
true |
|
number |
integer |
true |
|
name |
string |
true |
|
flowRateGpm |
number |
false |
|
ReportFilters
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersFilter |
false |
|
measureUnits |
WateringMeasureUnitsFilter |
true |
|
period |
PeriodFilter |
true |
|
ProjectControllersFilter
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersValue[ ] |
true |
|
ProjectControllersValue
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
controllerIdList |
string[ ] |
false |
|
WateringMeasureUnitsFilter
Name |
Type |
Required |
Description |
units |
string |
true |
|
PeriodFilter
Name |
Type |
Required |
Description |
startDate |
string |
true |
|
endDate |
string |
true |
|
interval |
string |
true |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
V1 | Reports | Landscape Report
Create Landscape report
Request
curl "https://developer-api.etwater.com/api/v1/reports/landscape-report" \
-X POST \
-H "Authorization: example string value" \
--data "{
\"filters\": {
\"projectControllers\": {
\"projectControllers\": [
{
\"projectId\": \"example string value\",
\"controllerIdList\": [
\"example string value\"
]
}
]
},
\"scheduleTypes\": [
\"FULLY_AUTOMATED\"
]
},
\"meta\": {
\"example_property_name\": \"example string value\"
}
}"
On success, the above request returns response like
{
"id": "example string value",
"status": "UNPROCESSABLE",
"userId": "example string value",
"data": {
"projects": [
{
"id": "example string value",
"name": "example string value",
"controllers": [
{
"id": "example string value",
"name": "example string value",
"zones": [
{
"id": "example string value",
"number": 815,
"name": "example string value",
"vegetationTypes": [
"example string value"
],
"emitterTypes": [
"example string value"
],
"scheduleType": "ESTABLISHMENT",
"rootDepthMeters": 991.275490723213,
"intervalAdjustmentCoefficient": 327.3163779300248,
"waterBudgetAdjustmentCoefficient": 586,
"triggerDepletionPercent": 966,
"soilType": "example string value",
"slopeTypeId": 332,
"shadeLevel": 136
}
]
}
]
}
]
},
"filters": {
"projectControllers": {
"projectControllers": [
{
"projectId": "example string value",
"controllerIdList": [
"example string value"
]
}
]
},
"scheduleTypes": [
"ONE_TIME"
]
},
"meta": {
"example_property_name": "example string value"
},
"storedDataUrl": "example string value"
}
HTTP Request
POST /api/v1/reports/landscape-report
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
GetLandscapeReportRequest |
LandscapeReportCreateRequest |
body |
false |
|
LandscapeReportCreateRequest
Name |
Type |
Required |
Description |
filters |
ReportFilters |
true |
|
meta |
Object for field meta |
false |
|
ReportFilters
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersFilter |
true |
|
scheduleTypes |
string[ ] |
false |
|
ProjectControllersFilter
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersValue[ ] |
true |
|
ProjectControllersValue
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
controllerIdList |
string[ ] |
false |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
false |
|
status |
string |
true |
|
userId |
string |
true |
|
data |
ReportData |
false |
|
filters |
ReportFilters |
true |
|
meta |
Object for field meta |
false |
|
storedDataUrl |
string |
false |
|
ReportData
Name |
Type |
Required |
Description |
projects |
Project[ ] |
true |
|
Project
Name |
Type |
Required |
Description |
id |
string |
true |
|
name |
string |
true |
|
controllers |
Controller[ ] |
true |
|
Controller
Name |
Type |
Required |
Description |
id |
string |
true |
|
name |
string |
true |
|
zones |
Zone[ ] |
true |
|
Zone
Name |
Type |
Required |
Description |
id |
string |
true |
|
number |
integer |
true |
|
name |
string |
true |
|
vegetationTypes |
string[ ] |
true |
|
emitterTypes |
string[ ] |
true |
|
scheduleType |
string |
true |
|
rootDepthMeters |
number |
true |
|
intervalAdjustmentCoefficient |
number |
true |
|
waterBudgetAdjustmentCoefficient |
number |
true |
|
triggerDepletionPercent |
integer |
true |
|
soilType |
string |
true |
|
slopeTypeId |
integer |
true |
|
shadeLevel |
integer |
true |
|
ReportFilters
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersFilter |
true |
|
scheduleTypes |
string[ ] |
false |
|
ProjectControllersFilter
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersValue[ ] |
true |
|
ProjectControllersValue
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
controllerIdList |
string[ ] |
false |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
Get Landscape report
Request
curl "https://developer-api.etwater.com/api/v1/reports/landscape-report/{id}" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"id": "example string value",
"status": "UNPROCESSABLE",
"userId": "example string value",
"data": {
"projects": [
{
"id": "example string value",
"name": "example string value",
"controllers": [
{
"id": "example string value",
"name": "example string value",
"zones": [
{
"id": "example string value",
"number": 275,
"name": "example string value",
"vegetationTypes": [
"example string value"
],
"emitterTypes": [
"example string value"
],
"scheduleType": "ESTABLISHMENT",
"rootDepthMeters": 129.61841101274752,
"intervalAdjustmentCoefficient": 678.0169390505258,
"waterBudgetAdjustmentCoefficient": 868,
"triggerDepletionPercent": 282,
"soilType": "example string value",
"slopeTypeId": 788,
"shadeLevel": 925
}
]
}
]
}
]
},
"filters": {
"projectControllers": {
"projectControllers": [
{
"projectId": "example string value",
"controllerIdList": [
"example string value"
]
}
]
},
"scheduleTypes": [
"FULLY_AUTOMATED"
]
},
"meta": {
"example_property_name": "example string value"
},
"storedDataUrl": "example string value"
}
HTTP Request
GET /api/v1/reports/landscape-report/{id}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
id |
string |
path |
true |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
true |
|
status |
string |
true |
|
userId |
string |
true |
|
data |
ReportData |
false |
|
filters |
ReportFilters |
true |
|
meta |
Object for field meta |
false |
|
storedDataUrl |
string |
false |
|
ReportData
Name |
Type |
Required |
Description |
projects |
Project[ ] |
true |
|
Project
Name |
Type |
Required |
Description |
id |
string |
true |
|
name |
string |
true |
|
controllers |
Controller[ ] |
true |
|
Controller
Name |
Type |
Required |
Description |
id |
string |
true |
|
name |
string |
true |
|
zones |
Zone[ ] |
true |
|
Zone
Name |
Type |
Required |
Description |
id |
string |
true |
|
number |
integer |
true |
|
name |
string |
true |
|
vegetationTypes |
string[ ] |
true |
|
emitterTypes |
string[ ] |
true |
|
scheduleType |
string |
true |
|
rootDepthMeters |
number |
true |
|
intervalAdjustmentCoefficient |
number |
true |
|
waterBudgetAdjustmentCoefficient |
number |
true |
|
triggerDepletionPercent |
integer |
true |
|
soilType |
string |
true |
|
slopeTypeId |
integer |
true |
|
shadeLevel |
integer |
true |
|
ReportFilters
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersFilter |
true |
|
scheduleTypes |
string[ ] |
false |
|
ProjectControllersFilter
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersValue[ ] |
true |
|
ProjectControllersValue
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
controllerIdList |
string[ ] |
false |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
V1 | Reports | Measured Water Cost Report
Create measured water cost report
Request
curl "https://developer-api.etwater.com/api/v1/reports/measured-water-cost-report?startDate=example+string+value&endDate=example+string+value&interval=MONTH&measureUnits=example+string+value" \
-X POST \
-H "Authorization: example string value" \
--data "{
\"filters\": {
\"projectControllers\": {
\"projectControllers\": [
{
\"projectId\": \"example string value\",
\"controllerIdList\": [
\"example string value\"
]
}
]
},
\"measureUnits\": {
\"units\": \"HCF\"
},
\"period\": {
\"startDate\": \"example string value\",
\"endDate\": \"example string value\",
\"interval\": \"BILLING_PERIOD\"
}
},
\"meta\": {
\"example_property_name\": \"example string value\"
}
}"
On success, the above request returns response like
{
"id": "example string value",
"status": "UNPROCESSABLE",
"userId": "example string value",
"data": {
"total": {
"dateStart": "example string value",
"dateEnd": "example string value",
"manualUsage": 634,
"manualUsageCost": 944,
"scheduledUsage": 492.43562924323396,
"scheduledUsageCost": 786,
"otherUsage": 891,
"otherUsageCost": 575,
"projects": [
{
"projectId": "example string value",
"name": "example string value",
"manualUsage": 177.76008750207725,
"manualUsageCost": 347,
"scheduledUsage": 833.8059526094264,
"scheduledUsageCost": 782,
"otherUsage": 295,
"otherUsageCost": 445,
"controllers": [
{
"controllerId": "example string value",
"name": "example string value",
"manualUsage": 726.7972942100826,
"manualUsageCost": 539,
"scheduledUsage": 133.7148720089881,
"scheduledUsageCost": 188,
"otherUsage": 187,
"otherUsageCost": 434.9782105698149,
"zones": [
{
"zoneNumber": 465,
"name": "example string value",
"manualUsage": 767,
"manualUsageCost": 891.6523637676855,
"scheduledUsage": 319,
"scheduledUsageCost": 398,
"totalWaterUsage": 523.7457028235057,
"totalWaterUsageCost": 205
}
],
"totalWaterUsage": 405,
"totalWaterUsageCost": 654
}
],
"totalWaterUsage": 839.9893426522564,
"totalWaterUsageCost": 256
}
],
"totalWaterUsage": 316,
"totalWaterUsageCost": 600.3772176803916
},
"buckets": [
{
"dateStart": "example string value",
"dateEnd": "example string value",
"manualUsage": 279,
"manualUsageCost": 405,
"scheduledUsage": 182.17299002323907,
"scheduledUsageCost": 901,
"otherUsage": 632.9456091080539,
"otherUsageCost": 984,
"projects": [
{
"projectId": "example string value",
"name": "example string value",
"manualUsage": 729,
"manualUsageCost": 544,
"scheduledUsage": 898.4388722565207,
"scheduledUsageCost": 276,
"otherUsage": 401,
"otherUsageCost": 520.6105040947956,
"controllers": [
{
"controllerId": "example string value",
"name": "example string value",
"manualUsage": 145.21136746984504,
"manualUsageCost": 16,
"scheduledUsage": 454.59601304242204,
"scheduledUsageCost": 188.17257703662506,
"otherUsage": 424.8796456609292,
"otherUsageCost": 554.4647716705058,
"zones": [
{
"zoneNumber": 58,
"name": "example string value",
"manualUsage": 277.5534285593561,
"manualUsageCost": 558,
"scheduledUsage": 123,
"scheduledUsageCost": 315.7391228320725,
"totalWaterUsage": 775,
"totalWaterUsageCost": 435.4783037842616
}
],
"totalWaterUsage": 48,
"totalWaterUsageCost": 134
}
],
"totalWaterUsage": 83,
"totalWaterUsageCost": 673
}
],
"totalWaterUsage": 333.56329907363437,
"totalWaterUsageCost": 742.2739587455401
}
],
"bucketsTotal": [
{
"dateStart": "example string value",
"dateEnd": "example string value",
"manualUsage": 710.4427999399802,
"manualUsageCost": 705,
"scheduledUsage": 860,
"scheduledUsageCost": 317,
"otherUsage": 227.91809506151736,
"otherUsageCost": 651,
"totalWaterUsage": 833,
"totalWaterUsageCost": 119
}
]
},
"filters": {
"projectControllers": {
"projectControllers": [
{
"projectId": "example string value",
"controllerIdList": [
"example string value"
]
}
]
},
"measureUnits": {
"units": "HCF"
},
"period": {
"startDate": "example string value",
"endDate": "example string value",
"interval": "MONTH"
}
},
"meta": {
"example_property_name": "example string value"
},
"storedDataUrl": "example string value"
}
HTTP Request
POST /api/v1/reports/measured-water-cost-report
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
startDate |
string |
query |
true |
|
endDate |
string |
query |
true |
|
interval |
string |
query |
false |
|
measureUnits |
string |
query |
false |
|
MeasuredWaterCostReportCreateRequest |
MeasuredWaterCostReportCreateRequest |
body |
true |
|
MeasuredWaterCostReportCreateRequest
Name |
Type |
Required |
Description |
filters |
ReportFilters |
true |
|
meta |
Object for field meta |
false |
|
ReportFilters
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersFilter |
false |
|
measureUnits |
WateringMeasureUnitsFilter |
true |
|
period |
PeriodFilter |
true |
|
ProjectControllersFilter
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersValue[ ] |
true |
|
ProjectControllersValue
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
controllerIdList |
string[ ] |
false |
|
WateringMeasureUnitsFilter
Name |
Type |
Required |
Description |
units |
string |
true |
|
PeriodFilter
Name |
Type |
Required |
Description |
startDate |
string |
true |
|
endDate |
string |
true |
|
interval |
string |
true |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
false |
|
status |
string |
true |
|
userId |
string |
true |
|
data |
ReportData |
false |
|
filters |
ReportFilters |
true |
|
meta |
Object for field meta |
false |
|
storedDataUrl |
string |
false |
|
ReportData
Name |
Type |
Required |
Description |
total |
Bucket |
true |
|
buckets |
Bucket[ ] |
true |
|
bucketsTotal |
BucketTotal[ ] |
true |
|
Bucket
Name |
Type |
Required |
Description |
dateStart |
string |
true |
|
dateEnd |
string |
true |
|
manualUsage |
number |
true |
|
manualUsageCost |
number |
true |
|
scheduledUsage |
number |
true |
|
scheduledUsageCost |
number |
true |
|
otherUsage |
number |
true |
|
otherUsageCost |
number |
true |
|
projects |
Project[ ] |
true |
|
totalWaterUsage |
number |
true |
|
totalWaterUsageCost |
number |
true |
|
Project
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
name |
string |
true |
|
manualUsage |
number |
true |
|
manualUsageCost |
number |
true |
|
scheduledUsage |
number |
true |
|
scheduledUsageCost |
number |
true |
|
otherUsage |
number |
true |
|
otherUsageCost |
number |
true |
|
controllers |
Controller[ ] |
true |
|
totalWaterUsage |
number |
true |
|
totalWaterUsageCost |
number |
true |
|
Controller
Name |
Type |
Required |
Description |
controllerId |
string |
true |
|
name |
string |
true |
|
manualUsage |
number |
true |
|
manualUsageCost |
number |
true |
|
scheduledUsage |
number |
true |
|
scheduledUsageCost |
number |
true |
|
otherUsage |
number |
true |
|
otherUsageCost |
number |
true |
|
zones |
Zone[ ] |
true |
|
totalWaterUsage |
number |
true |
|
totalWaterUsageCost |
number |
true |
|
Zone
Name |
Type |
Required |
Description |
zoneNumber |
integer |
true |
|
name |
string |
true |
|
manualUsage |
number |
true |
|
manualUsageCost |
number |
true |
|
scheduledUsage |
number |
true |
|
scheduledUsageCost |
number |
true |
|
totalWaterUsage |
number |
true |
|
totalWaterUsageCost |
number |
true |
|
BucketTotal
Name |
Type |
Required |
Description |
dateStart |
string |
true |
|
dateEnd |
string |
true |
|
manualUsage |
number |
true |
|
manualUsageCost |
number |
true |
|
scheduledUsage |
number |
true |
|
scheduledUsageCost |
number |
true |
|
otherUsage |
number |
true |
|
otherUsageCost |
number |
true |
|
totalWaterUsage |
number |
true |
|
totalWaterUsageCost |
number |
true |
|
ReportFilters
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersFilter |
false |
|
measureUnits |
WateringMeasureUnitsFilter |
true |
|
period |
PeriodFilter |
true |
|
ProjectControllersFilter
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersValue[ ] |
true |
|
ProjectControllersValue
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
controllerIdList |
string[ ] |
false |
|
WateringMeasureUnitsFilter
Name |
Type |
Required |
Description |
units |
string |
true |
|
PeriodFilter
Name |
Type |
Required |
Description |
startDate |
string |
true |
|
endDate |
string |
true |
|
interval |
string |
true |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
Get measured water cost report
Request
curl "https://developer-api.etwater.com/api/v1/reports/measured-water-cost-report/{id}" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"id": "example string value",
"status": "IN_PROGRESS",
"userId": "example string value",
"data": {
"total": {
"dateStart": "example string value",
"dateEnd": "example string value",
"manualUsage": 73.21329138857,
"manualUsageCost": 578.0666976133672,
"scheduledUsage": 516.1931945552086,
"scheduledUsageCost": 214,
"otherUsage": 659,
"otherUsageCost": 82,
"projects": [
{
"projectId": "example string value",
"name": "example string value",
"manualUsage": 795,
"manualUsageCost": 393.5505209460624,
"scheduledUsage": 938.6997348343486,
"scheduledUsageCost": 68,
"otherUsage": 131.699375403905,
"otherUsageCost": 969.8356492304409,
"controllers": [
{
"controllerId": "example string value",
"name": "example string value",
"manualUsage": 803.9088872279548,
"manualUsageCost": 454,
"scheduledUsage": 22,
"scheduledUsageCost": 872.9824791070923,
"otherUsage": 828.8984074391883,
"otherUsageCost": 754,
"zones": [
{
"zoneNumber": 703,
"name": "example string value",
"manualUsage": 308,
"manualUsageCost": 96,
"scheduledUsage": 976,
"scheduledUsageCost": 380,
"totalWaterUsage": 753,
"totalWaterUsageCost": 533
}
],
"totalWaterUsage": 43,
"totalWaterUsageCost": 968.3137060926825
}
],
"totalWaterUsage": 328,
"totalWaterUsageCost": 304
}
],
"totalWaterUsage": 873,
"totalWaterUsageCost": 843
},
"buckets": [
{
"dateStart": "example string value",
"dateEnd": "example string value",
"manualUsage": 137.7217984468312,
"manualUsageCost": 963.2056699894395,
"scheduledUsage": 784.8534010280173,
"scheduledUsageCost": 928.2959983350225,
"otherUsage": 483,
"otherUsageCost": 859.2269177824385,
"projects": [
{
"projectId": "example string value",
"name": "example string value",
"manualUsage": 561.4721484302879,
"manualUsageCost": 835,
"scheduledUsage": 49.717873358036336,
"scheduledUsageCost": 319,
"otherUsage": 352.77855412698284,
"otherUsageCost": 578.8982834568704,
"controllers": [
{
"controllerId": "example string value",
"name": "example string value",
"manualUsage": 309,
"manualUsageCost": 588.4711470401246,
"scheduledUsage": 316,
"scheduledUsageCost": 624.2268032507164,
"otherUsage": 996.0791566390913,
"otherUsageCost": 944,
"zones": [
{
"zoneNumber": 218,
"name": "example string value",
"manualUsage": 387,
"manualUsageCost": 676.0852740500519,
"scheduledUsage": 2.747751773683239,
"scheduledUsageCost": 563,
"totalWaterUsage": 874,
"totalWaterUsageCost": 947
}
],
"totalWaterUsage": 28.70335431243449,
"totalWaterUsageCost": 769.0822369275066
}
],
"totalWaterUsage": 614.8098249057354,
"totalWaterUsageCost": 707.1003474793864
}
],
"totalWaterUsage": 370.8619099906003,
"totalWaterUsageCost": 595.7042787204051
}
],
"bucketsTotal": [
{
"dateStart": "example string value",
"dateEnd": "example string value",
"manualUsage": 99,
"manualUsageCost": 38.98417159867667,
"scheduledUsage": 646.3975662581612,
"scheduledUsageCost": 238.36531966848545,
"otherUsage": 201,
"otherUsageCost": 31.65202449618467,
"totalWaterUsage": 63.34230073883305,
"totalWaterUsageCost": 865
}
]
},
"filters": {
"projectControllers": {
"projectControllers": [
{
"projectId": "example string value",
"controllerIdList": [
"example string value"
]
}
]
},
"measureUnits": {
"units": "HCF"
},
"period": {
"startDate": "example string value",
"endDate": "example string value",
"interval": "DAY"
}
},
"meta": {
"example_property_name": "example string value"
},
"storedDataUrl": "example string value"
}
HTTP Request
GET /api/v1/reports/measured-water-cost-report/{id}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
id |
string |
path |
true |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
true |
|
status |
string |
true |
|
userId |
string |
true |
|
data |
ReportData |
false |
|
filters |
ReportFilters |
true |
|
meta |
Object for field meta |
false |
|
storedDataUrl |
string |
false |
|
ReportData
Name |
Type |
Required |
Description |
total |
Bucket |
true |
|
buckets |
Bucket[ ] |
true |
|
bucketsTotal |
BucketTotal[ ] |
true |
|
Bucket
Name |
Type |
Required |
Description |
dateStart |
string |
true |
|
dateEnd |
string |
true |
|
manualUsage |
number |
true |
|
manualUsageCost |
number |
true |
|
scheduledUsage |
number |
true |
|
scheduledUsageCost |
number |
true |
|
otherUsage |
number |
true |
|
otherUsageCost |
number |
true |
|
projects |
Project[ ] |
true |
|
totalWaterUsage |
number |
true |
|
totalWaterUsageCost |
number |
true |
|
Project
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
name |
string |
true |
|
manualUsage |
number |
true |
|
manualUsageCost |
number |
true |
|
scheduledUsage |
number |
true |
|
scheduledUsageCost |
number |
true |
|
otherUsage |
number |
true |
|
otherUsageCost |
number |
true |
|
controllers |
Controller[ ] |
true |
|
totalWaterUsage |
number |
true |
|
totalWaterUsageCost |
number |
true |
|
Controller
Name |
Type |
Required |
Description |
controllerId |
string |
true |
|
name |
string |
true |
|
manualUsage |
number |
true |
|
manualUsageCost |
number |
true |
|
scheduledUsage |
number |
true |
|
scheduledUsageCost |
number |
true |
|
otherUsage |
number |
true |
|
otherUsageCost |
number |
true |
|
zones |
Zone[ ] |
true |
|
totalWaterUsage |
number |
true |
|
totalWaterUsageCost |
number |
true |
|
Zone
Name |
Type |
Required |
Description |
zoneNumber |
integer |
true |
|
name |
string |
true |
|
manualUsage |
number |
true |
|
manualUsageCost |
number |
true |
|
scheduledUsage |
number |
true |
|
scheduledUsageCost |
number |
true |
|
totalWaterUsage |
number |
true |
|
totalWaterUsageCost |
number |
true |
|
BucketTotal
Name |
Type |
Required |
Description |
dateStart |
string |
true |
|
dateEnd |
string |
true |
|
manualUsage |
number |
true |
|
manualUsageCost |
number |
true |
|
scheduledUsage |
number |
true |
|
scheduledUsageCost |
number |
true |
|
otherUsage |
number |
true |
|
otherUsageCost |
number |
true |
|
totalWaterUsage |
number |
true |
|
totalWaterUsageCost |
number |
true |
|
ReportFilters
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersFilter |
false |
|
measureUnits |
WateringMeasureUnitsFilter |
true |
|
period |
PeriodFilter |
true |
|
ProjectControllersFilter
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersValue[ ] |
true |
|
ProjectControllersValue
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
controllerIdList |
string[ ] |
false |
|
WateringMeasureUnitsFilter
Name |
Type |
Required |
Description |
units |
string |
true |
|
PeriodFilter
Name |
Type |
Required |
Description |
startDate |
string |
true |
|
endDate |
string |
true |
|
interval |
string |
true |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
V1 | Reports | Measured Water Usage Report
Create measured water usage report
Request
curl "https://developer-api.etwater.com/api/v1/reports/measured-water-usage-report?startDate=example+string+value&endDate=example+string+value&interval=BILLING_PERIOD&measureUnits=example+string+value" \
-X POST \
-H "Authorization: example string value" \
--data "{
\"filters\": {
\"projectControllers\": {
\"projectControllers\": [
{
\"projectId\": \"example string value\",
\"controllerIdList\": [
\"example string value\"
]
}
]
},
\"measureUnits\": {
\"units\": \"HCF\"
},
\"period\": {
\"startDate\": \"example string value\",
\"endDate\": \"example string value\",
\"interval\": \"DAY\"
}
},
\"meta\": {
\"example_property_name\": \"example string value\"
}
}"
On success, the above request returns response like
{
"id": "example string value",
"status": "IN_PROGRESS",
"userId": "example string value",
"data": {
"total": {
"dateStart": "example string value",
"dateEnd": "example string value",
"manualUsage": 673,
"scheduledUsage": 486,
"otherUsage": 322,
"projects": [
{
"projectId": "example string value",
"name": "example string value",
"manualUsage": 563,
"scheduledUsage": 649.6952519052173,
"otherUsage": 916.7192657090347,
"controllers": [
{
"controllerId": "example string value",
"name": "example string value",
"manualUsage": 560,
"scheduledUsage": 990.2627528599755,
"otherUsage": 163,
"zones": [
{
"zoneNumber": 693,
"name": "example string value",
"manualUsage": 460,
"scheduledUsage": 127,
"totalWaterUsage": 750
}
],
"totalWaterUsage": 200
}
],
"totalWaterUsage": 662.5726137601642
}
],
"totalWaterUsage": 325
},
"buckets": [
{
"dateStart": "example string value",
"dateEnd": "example string value",
"manualUsage": 631,
"scheduledUsage": 328,
"otherUsage": 10,
"projects": [
{
"projectId": "example string value",
"name": "example string value",
"manualUsage": 665,
"scheduledUsage": 267,
"otherUsage": 346,
"controllers": [
{
"controllerId": "example string value",
"name": "example string value",
"manualUsage": 637,
"scheduledUsage": 406.2583089835282,
"otherUsage": 724,
"zones": [
{
"zoneNumber": 122,
"name": "example string value",
"manualUsage": 26,
"scheduledUsage": 21,
"totalWaterUsage": 18
}
],
"totalWaterUsage": 398
}
],
"totalWaterUsage": 278
}
],
"totalWaterUsage": 51.66941138527795
}
],
"bucketsTotal": [
{
"dateStart": "example string value",
"dateEnd": "example string value",
"manualUsage": 863.877047255578,
"scheduledUsage": 403.828498164112,
"otherUsage": 201,
"totalWaterUsage": 982.4379593983468
}
]
},
"filters": {
"projectControllers": {
"projectControllers": [
{
"projectId": "example string value",
"controllerIdList": [
"example string value"
]
}
]
},
"measureUnits": {
"units": "GALLONS"
},
"period": {
"startDate": "example string value",
"endDate": "example string value",
"interval": "BILLING_PERIOD"
}
},
"meta": {
"example_property_name": "example string value"
},
"storedDataUrl": "example string value"
}
HTTP Request
POST /api/v1/reports/measured-water-usage-report
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
startDate |
string |
query |
true |
|
endDate |
string |
query |
true |
|
interval |
string |
query |
false |
|
measureUnits |
string |
query |
false |
|
MeasuredWaterUsageReportCreateRequest |
MeasuredWaterUsageReportCreateRequest |
body |
true |
|
MeasuredWaterUsageReportCreateRequest
Name |
Type |
Required |
Description |
filters |
ReportFilters |
true |
|
meta |
Object for field meta |
false |
|
ReportFilters
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersFilter |
false |
|
measureUnits |
WateringMeasureUnitsFilter |
true |
|
period |
PeriodFilter |
true |
|
ProjectControllersFilter
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersValue[ ] |
true |
|
ProjectControllersValue
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
controllerIdList |
string[ ] |
false |
|
WateringMeasureUnitsFilter
Name |
Type |
Required |
Description |
units |
string |
true |
|
PeriodFilter
Name |
Type |
Required |
Description |
startDate |
string |
true |
|
endDate |
string |
true |
|
interval |
string |
true |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
false |
|
status |
string |
true |
|
userId |
string |
true |
|
data |
ReportData |
false |
|
filters |
ReportFilters |
true |
|
meta |
Object for field meta |
false |
|
storedDataUrl |
string |
false |
|
ReportData
Name |
Type |
Required |
Description |
total |
Bucket |
true |
|
buckets |
Bucket[ ] |
true |
|
bucketsTotal |
BucketTotal[ ] |
true |
|
Bucket
Name |
Type |
Required |
Description |
dateStart |
string |
true |
|
dateEnd |
string |
true |
|
manualUsage |
number |
true |
|
scheduledUsage |
number |
true |
|
otherUsage |
number |
true |
|
projects |
Project[ ] |
true |
|
totalWaterUsage |
number |
true |
|
Project
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
name |
string |
true |
|
manualUsage |
number |
true |
|
scheduledUsage |
number |
true |
|
otherUsage |
number |
true |
|
controllers |
Controller[ ] |
true |
|
totalWaterUsage |
number |
true |
|
Controller
Name |
Type |
Required |
Description |
controllerId |
string |
true |
|
name |
string |
true |
|
manualUsage |
number |
true |
|
scheduledUsage |
number |
true |
|
otherUsage |
number |
true |
|
zones |
Zone[ ] |
true |
|
totalWaterUsage |
number |
true |
|
Zone
Name |
Type |
Required |
Description |
zoneNumber |
integer |
true |
|
name |
string |
true |
|
manualUsage |
number |
true |
|
scheduledUsage |
number |
true |
|
totalWaterUsage |
number |
true |
|
BucketTotal
Name |
Type |
Required |
Description |
dateStart |
string |
true |
|
dateEnd |
string |
true |
|
manualUsage |
number |
true |
|
scheduledUsage |
number |
true |
|
otherUsage |
number |
true |
|
totalWaterUsage |
number |
true |
|
ReportFilters
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersFilter |
false |
|
measureUnits |
WateringMeasureUnitsFilter |
true |
|
period |
PeriodFilter |
true |
|
ProjectControllersFilter
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersValue[ ] |
true |
|
ProjectControllersValue
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
controllerIdList |
string[ ] |
false |
|
WateringMeasureUnitsFilter
Name |
Type |
Required |
Description |
units |
string |
true |
|
PeriodFilter
Name |
Type |
Required |
Description |
startDate |
string |
true |
|
endDate |
string |
true |
|
interval |
string |
true |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
Get measured water usage report
Request
curl "https://developer-api.etwater.com/api/v1/reports/measured-water-usage-report/{id}" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"id": "example string value",
"status": "IN_PROGRESS",
"userId": "example string value",
"data": {
"total": {
"dateStart": "example string value",
"dateEnd": "example string value",
"manualUsage": 892.4322556203381,
"scheduledUsage": 451.90189147922297,
"otherUsage": 610.8645925348926,
"projects": [
{
"projectId": "example string value",
"name": "example string value",
"manualUsage": 738,
"scheduledUsage": 388,
"otherUsage": 522,
"controllers": [
{
"controllerId": "example string value",
"name": "example string value",
"manualUsage": 583.5992882883173,
"scheduledUsage": 66,
"otherUsage": 315.51075368910597,
"zones": [
{
"zoneNumber": 140,
"name": "example string value",
"manualUsage": 819.5439986044281,
"scheduledUsage": 744,
"totalWaterUsage": 107
}
],
"totalWaterUsage": 999
}
],
"totalWaterUsage": 589.6376015570189
}
],
"totalWaterUsage": 104.1615237966932
},
"buckets": [
{
"dateStart": "example string value",
"dateEnd": "example string value",
"manualUsage": 739.1687690928434,
"scheduledUsage": 219,
"otherUsage": 461.69274554666725,
"projects": [
{
"projectId": "example string value",
"name": "example string value",
"manualUsage": 320.1352908835445,
"scheduledUsage": 204,
"otherUsage": 695.7108027747418,
"controllers": [
{
"controllerId": "example string value",
"name": "example string value",
"manualUsage": 338,
"scheduledUsage": 57.35064347151231,
"otherUsage": 887,
"zones": [
{
"zoneNumber": 986,
"name": "example string value",
"manualUsage": 816.1577292793233,
"scheduledUsage": 411.8768313954942,
"totalWaterUsage": 622.113357122109
}
],
"totalWaterUsage": 490
}
],
"totalWaterUsage": 106.12030797922999
}
],
"totalWaterUsage": 324.9705174588461
}
],
"bucketsTotal": [
{
"dateStart": "example string value",
"dateEnd": "example string value",
"manualUsage": 21,
"scheduledUsage": 186,
"otherUsage": 24.022032517950066,
"totalWaterUsage": 376.8191078569829
}
]
},
"filters": {
"projectControllers": {
"projectControllers": [
{
"projectId": "example string value",
"controllerIdList": [
"example string value"
]
}
]
},
"measureUnits": {
"units": "HCF"
},
"period": {
"startDate": "example string value",
"endDate": "example string value",
"interval": "BILLING_PERIOD"
}
},
"meta": {
"example_property_name": "example string value"
},
"storedDataUrl": "example string value"
}
HTTP Request
GET /api/v1/reports/measured-water-usage-report/{id}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
id |
string |
path |
true |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
true |
|
status |
string |
true |
|
userId |
string |
true |
|
data |
ReportData |
false |
|
filters |
ReportFilters |
true |
|
meta |
Object for field meta |
false |
|
storedDataUrl |
string |
false |
|
ReportData
Name |
Type |
Required |
Description |
total |
Bucket |
true |
|
buckets |
Bucket[ ] |
true |
|
bucketsTotal |
BucketTotal[ ] |
true |
|
Bucket
Name |
Type |
Required |
Description |
dateStart |
string |
true |
|
dateEnd |
string |
true |
|
manualUsage |
number |
true |
|
scheduledUsage |
number |
true |
|
otherUsage |
number |
true |
|
projects |
Project[ ] |
true |
|
totalWaterUsage |
number |
true |
|
Project
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
name |
string |
true |
|
manualUsage |
number |
true |
|
scheduledUsage |
number |
true |
|
otherUsage |
number |
true |
|
controllers |
Controller[ ] |
true |
|
totalWaterUsage |
number |
true |
|
Controller
Name |
Type |
Required |
Description |
controllerId |
string |
true |
|
name |
string |
true |
|
manualUsage |
number |
true |
|
scheduledUsage |
number |
true |
|
otherUsage |
number |
true |
|
zones |
Zone[ ] |
true |
|
totalWaterUsage |
number |
true |
|
Zone
Name |
Type |
Required |
Description |
zoneNumber |
integer |
true |
|
name |
string |
true |
|
manualUsage |
number |
true |
|
scheduledUsage |
number |
true |
|
totalWaterUsage |
number |
true |
|
BucketTotal
Name |
Type |
Required |
Description |
dateStart |
string |
true |
|
dateEnd |
string |
true |
|
manualUsage |
number |
true |
|
scheduledUsage |
number |
true |
|
otherUsage |
number |
true |
|
totalWaterUsage |
number |
true |
|
ReportFilters
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersFilter |
false |
|
measureUnits |
WateringMeasureUnitsFilter |
true |
|
period |
PeriodFilter |
true |
|
ProjectControllersFilter
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersValue[ ] |
true |
|
ProjectControllersValue
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
controllerIdList |
string[ ] |
false |
|
WateringMeasureUnitsFilter
Name |
Type |
Required |
Description |
units |
string |
true |
|
PeriodFilter
Name |
Type |
Required |
Description |
startDate |
string |
true |
|
endDate |
string |
true |
|
interval |
string |
true |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
V1 | Reports | Over/Under Report
Create Over/Under report
Request
curl "https://developer-api.etwater.com/api/v1/reports/over-under-report?startDate=example+string+value&endDate=example+string+value&interval=example+string+value" \
-X POST \
-H "Authorization: example string value" \
--data "{
\"filters\": {
\"projectControllers\": {
\"projectControllers\": [
{
\"projectId\": \"example string value\",
\"controllerIdList\": [
\"example string value\"
]
}
]
},
\"period\": {
\"startDate\": \"example string value\",
\"endDate\": \"example string value\",
\"interval\": \"DAY\"
}
},
\"meta\": {
\"example_property_name\": \"example string value\"
}
}"
On success, the above request returns response like
{
"id": "example string value",
"status": "IN_PROGRESS",
"userId": "example string value",
"filters": {
"projectControllers": {
"projectControllers": [
{
"projectId": "example string value",
"controllerIdList": [
"example string value"
]
}
]
},
"period": {
"startDate": "example string value",
"endDate": "example string value",
"interval": "DAY"
}
},
"meta": {
"example_property_name": "example string value"
}
}
HTTP Request
POST /api/v1/reports/over-under-report
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
startDate |
string |
query |
true |
|
endDate |
string |
query |
true |
|
interval |
string |
query |
false |
|
OverUnderReportCreateRequest |
OverUnderReportCreateRequest |
body |
true |
|
OverUnderReportCreateRequest
Name |
Type |
Required |
Description |
filters |
ReportFilters |
true |
|
meta |
Object for field meta |
false |
|
ReportFilters
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersFilter |
false |
|
period |
PeriodFilter |
true |
|
ProjectControllersFilter
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersValue[ ] |
true |
|
ProjectControllersValue
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
controllerIdList |
string[ ] |
false |
|
PeriodFilter
Name |
Type |
Required |
Description |
startDate |
string |
true |
|
endDate |
string |
true |
|
interval |
string |
true |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
false |
|
status |
string |
true |
|
userId |
string |
true |
|
filters |
ReportFilters |
true |
|
meta |
Object for field meta |
false |
|
ReportFilters
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersFilter |
false |
|
period |
PeriodFilter |
true |
|
ProjectControllersFilter
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersValue[ ] |
true |
|
ProjectControllersValue
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
controllerIdList |
string[ ] |
false |
|
PeriodFilter
Name |
Type |
Required |
Description |
startDate |
string |
true |
|
endDate |
string |
true |
|
interval |
string |
true |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
Get Over/Under report
Request
curl "https://developer-api.etwater.com/api/v1/reports/over-under-report/{id}" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"id": "example string value",
"status": "IN_PROGRESS",
"userId": "example string value",
"data": {
"total": {
"dateStart": "example string value",
"dateEnd": "example string value",
"rainMm": 831.4348337386897,
"etcMm": 873.7008515157275,
"etoMm": 156.8857520617944,
"realEffectiveWateringMm": 594,
"projects": [
{
"id": "example string value",
"name": "example string value",
"controllers": [
{
"id": "example string value",
"name": "example string value",
"zones": [
{
"id": "example string value",
"number": 571,
"name": "example string value",
"rainMm": 613,
"etcMm": 889.5991290405389,
"etoMm": 638.6600996547659,
"realEffectiveWateringMm": 217.85584288549418
}
],
"rainMm": 590.241344920472,
"etcMm": 293,
"etoMm": 269,
"realEffectiveWateringMm": 678.513931426459
}
],
"rainMm": 889,
"etcMm": 614.7590007701698,
"etoMm": 521,
"realEffectiveWateringMm": 361
}
],
"avgRealEffectiveWateringMm": 93,
"avgEtcMmByStation": 47,
"avgRealEffectiveWateringMmByStation": 670
},
"buckets": [
{
"dateStart": "example string value",
"dateEnd": "example string value",
"rainMm": 758.8893537218167,
"etcMm": 195,
"etoMm": 379,
"realEffectiveWateringMm": 919.6275365164631,
"projects": [
{
"id": "example string value",
"name": "example string value",
"controllers": [
{
"id": "example string value",
"name": "example string value",
"zones": [
{
"id": "example string value",
"number": 828,
"name": "example string value",
"rainMm": 459,
"etcMm": 396.73373354446784,
"etoMm": 330,
"realEffectiveWateringMm": 834.497263112337
}
],
"rainMm": 798.8867516624214,
"etcMm": 607.6169286890034,
"etoMm": 218,
"realEffectiveWateringMm": 76
}
],
"rainMm": 876,
"etcMm": 215.2797482978924,
"etoMm": 490,
"realEffectiveWateringMm": 843.3314104766265
}
],
"avgRealEffectiveWateringMm": 471,
"avgEtcMmByStation": 647.7163246123662,
"avgRealEffectiveWateringMmByStation": 505.4170375249428
}
],
"bucketsTotal": [
{
"dateStart": "example string value",
"dateEnd": "example string value",
"rainMm": 956,
"etcMm": 655.438425790257,
"etoMm": 802,
"realEffectiveWateringMm": 847.5451627036301,
"avgRealEffectiveWateringMm": 653.612104548892,
"avgEtcMmByStation": 398,
"avgRealEffectiveWateringMmByStation": 57
}
],
"landscapeReport": [
{
"id": "example string value",
"name": "example string value",
"controllers": [
{
"id": "example string value",
"name": "example string value",
"zones": [
{
"id": "example string value",
"number": 915,
"name": "example string value",
"vegetationTypes": [
"example string value"
],
"emitterTypes": [
"example string value"
],
"scheduleType": "MANUAL",
"rootDepthMeters": 551,
"intervalAdjustmentCoefficient": 608,
"waterBudgetAdjustmentCoefficient": 329.55751443726825,
"triggerDepletionPercent": 200,
"soilType": "example string value",
"slopeTypeId": 964,
"shadeLevel": 716
}
]
}
]
}
]
},
"filters": {
"projectControllers": {
"projectControllers": [
{
"projectId": "example string value",
"controllerIdList": [
"example string value"
]
}
]
},
"period": {
"startDate": "example string value",
"endDate": "example string value",
"interval": "BILLING_PERIOD"
}
},
"meta": {
"example_property_name": "example string value"
},
"storedDataUrl": "example string value"
}
HTTP Request
GET /api/v1/reports/over-under-report/{id}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
id |
string |
path |
true |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
true |
|
status |
string |
true |
|
userId |
string |
true |
|
data |
ReportData |
false |
|
filters |
ReportFilters |
true |
|
meta |
Object for field meta |
false |
|
storedDataUrl |
string |
false |
|
ReportData
Name |
Type |
Required |
Description |
total |
Bucket |
true |
|
buckets |
Bucket[ ] |
true |
|
bucketsTotal |
BucketTotal[ ] |
true |
|
landscapeReport |
Project[ ] |
true |
|
Bucket
Name |
Type |
Required |
Description |
dateStart |
string |
true |
|
dateEnd |
string |
true |
|
rainMm |
number |
true |
|
etcMm |
number |
true |
|
etoMm |
number |
true |
|
realEffectiveWateringMm |
number |
true |
|
projects |
Project[ ] |
true |
|
avgRealEffectiveWateringMm |
number |
true |
|
avgEtcMmByStation |
number |
false |
|
avgRealEffectiveWateringMmByStation |
number |
false |
|
Project
Name |
Type |
Required |
Description |
id |
string |
true |
|
name |
string |
true |
|
controllers |
Controller[ ] |
true |
|
rainMm |
number |
true |
|
etcMm |
number |
true |
|
etoMm |
number |
true |
|
realEffectiveWateringMm |
number |
true |
|
Controller
Name |
Type |
Required |
Description |
id |
string |
true |
|
name |
string |
true |
|
zones |
Zone[ ] |
true |
|
rainMm |
number |
true |
|
etcMm |
number |
true |
|
etoMm |
number |
true |
|
realEffectiveWateringMm |
number |
true |
|
Zone
Name |
Type |
Required |
Description |
id |
string |
true |
|
number |
integer |
true |
|
name |
string |
true |
|
rainMm |
number |
true |
|
etcMm |
number |
true |
|
etoMm |
number |
true |
|
realEffectiveWateringMm |
number |
true |
|
BucketTotal
Name |
Type |
Required |
Description |
dateStart |
string |
true |
|
dateEnd |
string |
true |
|
rainMm |
number |
true |
|
etcMm |
number |
true |
|
etoMm |
number |
true |
|
realEffectiveWateringMm |
number |
true |
|
avgRealEffectiveWateringMm |
number |
true |
|
avgEtcMmByStation |
number |
false |
|
avgRealEffectiveWateringMmByStation |
number |
false |
|
ReportFilters
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersFilter |
false |
|
period |
PeriodFilter |
true |
|
ProjectControllersFilter
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersValue[ ] |
true |
|
ProjectControllersValue
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
controllerIdList |
string[ ] |
false |
|
PeriodFilter
Name |
Type |
Required |
Description |
startDate |
string |
true |
|
endDate |
string |
true |
|
interval |
string |
true |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
V1 | Reports | Runtime Report
Create runtime report
Request
curl "https://developer-api.etwater.com/api/v1/reports/runtime-report?startDate=example+string+value&endDate=example+string+value&interval=example+string+value" \
-X POST \
-H "Authorization: example string value" \
--data "{
\"filters\": {
\"projectControllers\": {
\"projectControllers\": [
{
\"projectId\": \"example string value\",
\"controllerIdList\": [
\"example string value\"
]
}
]
},
\"period\": {
\"startDate\": \"example string value\",
\"endDate\": \"example string value\",
\"interval\": \"DAY\"
}
},
\"meta\": {
\"example_property_name\": \"example string value\"
}
}"
On success, the above request returns response like
{
"id": "example string value",
"status": "DONE",
"userId": "example string value",
"filters": {
"projectControllers": {
"projectControllers": [
{
"projectId": "example string value",
"controllerIdList": [
"example string value"
]
}
]
},
"period": {
"startDate": "example string value",
"endDate": "example string value",
"interval": "BILLING_PERIOD"
}
},
"meta": {
"example_property_name": "example string value"
}
}
HTTP Request
POST /api/v1/reports/runtime-report
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
startDate |
string |
query |
true |
|
endDate |
string |
query |
true |
|
interval |
string |
query |
false |
|
RuntimeReportCreateRequest |
RuntimeReportCreateRequest |
body |
true |
|
RuntimeReportCreateRequest
Name |
Type |
Required |
Description |
filters |
ReportFilters |
true |
|
meta |
Object for field meta |
false |
|
ReportFilters
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersFilter |
false |
|
period |
PeriodFilter |
true |
|
ProjectControllersFilter
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersValue[ ] |
true |
|
ProjectControllersValue
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
controllerIdList |
string[ ] |
false |
|
PeriodFilter
Name |
Type |
Required |
Description |
startDate |
string |
true |
|
endDate |
string |
true |
|
interval |
string |
true |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
true |
|
status |
string |
true |
|
userId |
string |
true |
|
filters |
ReportFilters |
true |
|
meta |
Object for field meta |
false |
|
ReportFilters
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersFilter |
false |
|
period |
PeriodFilter |
true |
|
ProjectControllersFilter
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersValue[ ] |
true |
|
ProjectControllersValue
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
controllerIdList |
string[ ] |
false |
|
PeriodFilter
Name |
Type |
Required |
Description |
startDate |
string |
true |
|
endDate |
string |
true |
|
interval |
string |
true |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
Get runtime report
Request
curl "https://developer-api.etwater.com/api/v1/reports/runtime-report/{id}" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"id": "example string value",
"status": "IN_PROGRESS",
"userId": "example string value",
"data": {
"total": {
"dateStart": "example string value",
"dateEnd": "example string value",
"runtimeMinutes": 335,
"avgRainMm": 526,
"avgEtoMm": 559.8914677090437,
"projects": [
{
"projectId": "example string value",
"name": "example string value",
"runtimeMinutes": 670,
"avgRainMm": 759.561675954406,
"etoMm": 727.2458615374034,
"controllers": [
{
"controllerId": "example string value",
"name": "example string value",
"runtimeMinutes": 841,
"zones": [
{
"zoneNumber": 300,
"name": "example string value",
"runtimeMinutes": 344,
"emitterType": "example string value",
"emitterPrecipitationRate": 506,
"plantTypes": [
"example string value"
],
"wateringMm": 32,
"emitterCount": 960,
"emitterTypeId": 562,
"emitterPrecipitationRateMm": 407,
"scheduledRuntimeMinutes": 108,
"scheduledWateringMm": 898.0281487563756,
"manualRuntimeMinutes": 52,
"manualWateringMm": 755
}
],
"wateringMm": 447,
"scheduledRuntimeMinutes": 547,
"scheduledWateringMm": 254,
"manualRuntimeMinutes": 125,
"manualWateringMm": 539.3103759453215
}
],
"wateringMm": 318.5943576128196,
"scheduledRuntimeMinutes": 279,
"scheduledWateringMm": 994,
"manualRuntimeMinutes": 496,
"manualWateringMm": 398
}
],
"minEtoMm": 715.7255763726894,
"maxEtoMm": 763.7092414143073,
"wateringMm": 402.0344528379079,
"scheduledRuntimeMinutes": 154,
"scheduledWateringMm": 366,
"manualRuntimeMinutes": 916,
"manualWateringMm": 189.39279168350288
},
"buckets": [
{
"dateStart": "example string value",
"dateEnd": "example string value",
"runtimeMinutes": 780,
"avgRainMm": 448,
"avgEtoMm": 446,
"projects": [
{
"projectId": "example string value",
"name": "example string value",
"runtimeMinutes": 526,
"avgRainMm": 988.8692516781712,
"etoMm": 277,
"controllers": [
{
"controllerId": "example string value",
"name": "example string value",
"runtimeMinutes": 675,
"zones": [
{
"zoneNumber": 157,
"name": "example string value",
"runtimeMinutes": 818,
"emitterType": "example string value",
"emitterPrecipitationRate": 699.7285767922776,
"plantTypes": [
"example string value"
],
"wateringMm": 145,
"emitterCount": 751,
"emitterTypeId": 522,
"emitterPrecipitationRateMm": 826,
"scheduledRuntimeMinutes": 271,
"scheduledWateringMm": 792.0987707525952,
"manualRuntimeMinutes": 214,
"manualWateringMm": 144.8689108457737
}
],
"wateringMm": 500,
"scheduledRuntimeMinutes": 44,
"scheduledWateringMm": 462.7855687694557,
"manualRuntimeMinutes": 689,
"manualWateringMm": 611.4874918067303
}
],
"wateringMm": 260.5368505513933,
"scheduledRuntimeMinutes": 621,
"scheduledWateringMm": 209,
"manualRuntimeMinutes": 500,
"manualWateringMm": 710.5060143910841
}
],
"minEtoMm": 395,
"maxEtoMm": 37.407073675378726,
"wateringMm": 957,
"scheduledRuntimeMinutes": 612,
"scheduledWateringMm": 421,
"manualRuntimeMinutes": 182,
"manualWateringMm": 10.969661646974115
}
],
"bucketsTotal": [
{
"dateStart": "example string value",
"dateEnd": "example string value",
"runtimeMinutes": 800,
"avgRainMm": 935.009355626539,
"etoMm": 995.5494739094514,
"minEtoMm": 612.9725894951133,
"maxEtoMm": 805.2024449246015,
"wateringMm": 713,
"scheduledRuntimeMinutes": 786,
"scheduledWateringMm": 311,
"manualRuntimeMinutes": 262,
"manualWateringMm": 894
}
]
},
"filters": {
"projectControllers": {
"projectControllers": [
{
"projectId": "example string value",
"controllerIdList": [
"example string value"
]
}
]
},
"period": {
"startDate": "example string value",
"endDate": "example string value",
"interval": "BILLING_PERIOD"
}
},
"meta": {
"example_property_name": "example string value"
},
"storedDataUrl": "example string value"
}
HTTP Request
GET /api/v1/reports/runtime-report/{id}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
id |
string |
path |
true |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
true |
|
status |
string |
true |
|
userId |
string |
true |
|
data |
ReportData |
false |
|
filters |
ReportFilters |
true |
|
meta |
Object for field meta |
false |
|
storedDataUrl |
string |
false |
|
ReportData
Name |
Type |
Required |
Description |
total |
Bucket |
true |
|
buckets |
Bucket[ ] |
true |
|
bucketsTotal |
BucketTotal[ ] |
true |
|
Bucket
Name |
Type |
Required |
Description |
dateStart |
string |
true |
|
dateEnd |
string |
true |
|
runtimeMinutes |
integer |
true |
|
avgRainMm |
number |
true |
|
avgEtoMm |
number |
true |
|
projects |
Project[ ] |
true |
|
minEtoMm |
number |
true |
|
maxEtoMm |
number |
true |
|
wateringMm |
number |
false |
|
scheduledRuntimeMinutes |
integer |
false |
|
scheduledWateringMm |
number |
false |
|
manualRuntimeMinutes |
integer |
false |
|
manualWateringMm |
number |
false |
|
Project
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
name |
string |
true |
|
runtimeMinutes |
integer |
true |
|
avgRainMm |
number |
true |
|
etoMm |
number |
true |
|
controllers |
Controller[ ] |
true |
|
wateringMm |
number |
false |
|
scheduledRuntimeMinutes |
integer |
false |
|
scheduledWateringMm |
number |
false |
|
manualRuntimeMinutes |
integer |
false |
|
manualWateringMm |
number |
false |
|
Controller
Name |
Type |
Required |
Description |
controllerId |
string |
true |
|
name |
string |
true |
|
runtimeMinutes |
integer |
true |
|
zones |
Zone[ ] |
true |
|
wateringMm |
number |
false |
|
scheduledRuntimeMinutes |
integer |
false |
|
scheduledWateringMm |
number |
false |
|
manualRuntimeMinutes |
integer |
false |
|
manualWateringMm |
number |
false |
|
Zone
Name |
Type |
Required |
Description |
zoneNumber |
integer |
true |
|
name |
string |
true |
|
runtimeMinutes |
integer |
true |
|
emitterType |
string |
false |
|
emitterPrecipitationRate |
number |
false |
|
plantTypes |
string[ ] |
false |
|
wateringMm |
number |
false |
|
emitterCount |
integer |
false |
|
emitterTypeId |
integer |
false |
|
emitterPrecipitationRateMm |
number |
false |
|
scheduledRuntimeMinutes |
integer |
false |
|
scheduledWateringMm |
number |
false |
|
manualRuntimeMinutes |
integer |
false |
|
manualWateringMm |
number |
false |
|
BucketTotal
Name |
Type |
Required |
Description |
dateStart |
string |
true |
|
dateEnd |
string |
true |
|
runtimeMinutes |
integer |
true |
|
avgRainMm |
number |
true |
|
etoMm |
number |
true |
|
minEtoMm |
number |
true |
|
maxEtoMm |
number |
true |
|
wateringMm |
number |
false |
|
scheduledRuntimeMinutes |
integer |
false |
|
scheduledWateringMm |
number |
false |
|
manualRuntimeMinutes |
integer |
false |
|
manualWateringMm |
number |
false |
|
ReportFilters
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersFilter |
false |
|
period |
PeriodFilter |
true |
|
ProjectControllersFilter
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersValue[ ] |
true |
|
ProjectControllersValue
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
controllerIdList |
string[ ] |
false |
|
PeriodFilter
Name |
Type |
Required |
Description |
startDate |
string |
true |
|
endDate |
string |
true |
|
interval |
string |
true |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
V1 | Reports | Time Restrictions Report
Create Time restrictions report
Request
curl "https://developer-api.etwater.com/api/v1/reports/time-restrictions-report" \
-X POST \
-H "Authorization: example string value" \
--data "{
\"filters\": {
\"projectControllers\": {
\"projectControllers\": [
{
\"projectId\": \"example string value\",
\"controllerIdList\": [
\"example string value\"
]
}
]
}
},
\"meta\": {
\"example_property_name\": \"example string value\"
}
}"
On success, the above request returns response like
{
"id": "example string value",
"userId": "example string value",
"data": {
"projects": [
{
"id": "example string value",
"name": "example string value",
"controllers": [
{
"id": "example string value",
"name": "example string value",
"timeRestrictions": [
{
"id": "example string value",
"name": "example string value",
"repeatPattern": {
"dailyRepeatPattern": {
"repeatCount": 199,
"applyUntilDateTime": "example string value",
"interval": 104
},
"weeklyRepeatPattern": {
"repeatCount": 294,
"applyUntilDateTime": "example string value",
"interval": 46,
"daysOfWeek": [
"example string value"
]
},
"monthlyRepeatPattern": {
"repeatCount": 759,
"applyUntilDateTime": "example string value",
"interval": 894,
"daysOfMonth": [
497
],
"positionInMonth": 530,
"daysOfWeek": [
"example string value"
]
},
"yearlyRepeatPattern": {
"repeatCount": 85,
"applyUntilDateTime": "example string value",
"interval": 833,
"daysOfWeek": [
"example string value"
],
"daysOfYear": [
319
],
"numbersOfWeek": [
385
]
},
"oddDaysRepeatPattern": {
"repeatCount": 418,
"applyUntilDateTime": "example string value"
},
"evenDaysRepeatPattern": {
"repeatCount": 254,
"applyUntilDateTime": "example string value"
},
"weeklySameDayRepeatPattern": {
"repeatCount": 409,
"applyUntilDateTime": "example string value"
},
"weeklyWorkdaysRepeatPattern": {
"repeatCount": 781,
"applyUntilDateTime": "example string value"
},
"monthlySameDateRepeatPattern": {
"repeatCount": 833,
"applyUntilDateTime": "example string value"
},
"monthlySameDayRepeatPattern": {
"repeatCount": 65,
"applyUntilDateTime": "example string value"
},
"annuallySameDateRepeatPattern": {
"repeatCount": 419,
"applyUntilDateTime": "example string value"
}
},
"startDateTime": "example string value",
"endDateTime": "example string value",
"areaRegionTypeIds": [
1000
],
"emitterTypeIds": [
116
],
"stationNumbers": [
236
],
"allStations": false,
"effect": "example string value"
}
]
}
]
}
]
},
"filters": {
"projectControllers": {
"projectControllers": [
{
"projectId": "example string value",
"controllerIdList": [
"example string value"
]
}
]
}
},
"meta": {
"example_property_name": "example string value"
},
"storedDataUrl": "example string value"
}
HTTP Request
POST /api/v1/reports/time-restrictions-report
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
TimeRestrictionsReportCreateRequest |
TimeRestrictionsReportCreateRequest |
body |
true |
|
TimeRestrictionsReportCreateRequest
Name |
Type |
Required |
Description |
filters |
ReportFilters |
true |
|
meta |
Object for field meta |
false |
|
ReportFilters
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersFilter |
true |
|
ProjectControllersFilter
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersValue[ ] |
true |
|
ProjectControllersValue
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
controllerIdList |
string[ ] |
false |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
true |
|
userId |
string |
true |
|
data |
ReportData |
false |
|
filters |
ReportFilters |
true |
|
meta |
Object for field meta |
false |
|
storedDataUrl |
string |
false |
|
ReportData
Name |
Type |
Required |
Description |
projects |
Project[ ] |
true |
|
Project
Name |
Type |
Required |
Description |
id |
string |
true |
|
name |
string |
true |
|
controllers |
Controller[ ] |
true |
|
Controller
Name |
Type |
Required |
Description |
id |
string |
true |
|
name |
string |
true |
|
timeRestrictions |
TimeRestriction[ ] |
true |
|
TimeRestriction
Name |
Type |
Required |
Description |
id |
string |
true |
|
name |
string |
true |
|
repeatPattern |
RepeatPattern |
false |
|
startDateTime |
string |
true |
|
endDateTime |
string |
true |
|
areaRegionTypeIds |
integer[ ] |
false |
|
emitterTypeIds |
integer[ ] |
false |
|
stationNumbers |
integer[ ] |
false |
|
allStations |
boolean |
false |
|
effect |
string |
true |
|
RepeatPattern
Name |
Type |
Required |
Description |
dailyRepeatPattern |
DailyRepeatPattern |
false |
|
weeklyRepeatPattern |
WeeklyRepeatPattern |
false |
|
monthlyRepeatPattern |
MonthlyRepeatPattern |
false |
|
yearlyRepeatPattern |
YearlyRepeatPattern |
false |
|
oddDaysRepeatPattern |
OddDaysRepeatPattern |
false |
|
evenDaysRepeatPattern |
EvenDaysRepeatPattern |
false |
|
weeklySameDayRepeatPattern |
WeeklySameDayRepeatPattern |
false |
|
weeklyWorkdaysRepeatPattern |
WeeklyWorkdaysRepeatPattern |
false |
|
monthlySameDateRepeatPattern |
MonthlySameDateRepeatPattern |
false |
|
monthlySameDayRepeatPattern |
MonthlySameDayRepeatPattern |
false |
|
annuallySameDateRepeatPattern |
AnnuallySameDateRepeatPattern |
false |
|
DailyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
WeeklyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
daysOfWeek |
string[ ] |
false |
|
MonthlyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
daysOfMonth |
integer[ ] |
false |
|
positionInMonth |
integer |
false |
|
daysOfWeek |
string[ ] |
false |
|
YearlyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
daysOfWeek |
string[ ] |
false |
|
daysOfYear |
integer[ ] |
false |
|
numbersOfWeek |
integer[ ] |
false |
|
OddDaysRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
EvenDaysRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
WeeklySameDayRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
WeeklyWorkdaysRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
MonthlySameDateRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
MonthlySameDayRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
AnnuallySameDateRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
ReportFilters
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersFilter |
true |
|
ProjectControllersFilter
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersValue[ ] |
true |
|
ProjectControllersValue
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
controllerIdList |
string[ ] |
false |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
Get Time restrictions report
Request
curl "https://developer-api.etwater.com/api/v1/reports/time-restrictions-report/{id}" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"id": "example string value",
"userId": "example string value",
"data": {
"projects": [
{
"id": "example string value",
"name": "example string value",
"controllers": [
{
"id": "example string value",
"name": "example string value",
"timeRestrictions": [
{
"id": "example string value",
"name": "example string value",
"repeatPattern": {
"dailyRepeatPattern": {
"repeatCount": 272,
"applyUntilDateTime": "example string value",
"interval": 155
},
"weeklyRepeatPattern": {
"repeatCount": 678,
"applyUntilDateTime": "example string value",
"interval": 783,
"daysOfWeek": [
"example string value"
]
},
"monthlyRepeatPattern": {
"repeatCount": 484,
"applyUntilDateTime": "example string value",
"interval": 215,
"daysOfMonth": [
457
],
"positionInMonth": 342,
"daysOfWeek": [
"example string value"
]
},
"yearlyRepeatPattern": {
"repeatCount": 540,
"applyUntilDateTime": "example string value",
"interval": 162,
"daysOfWeek": [
"example string value"
],
"daysOfYear": [
692
],
"numbersOfWeek": [
686
]
},
"oddDaysRepeatPattern": {
"repeatCount": 555,
"applyUntilDateTime": "example string value"
},
"evenDaysRepeatPattern": {
"repeatCount": 454,
"applyUntilDateTime": "example string value"
},
"weeklySameDayRepeatPattern": {
"repeatCount": 764,
"applyUntilDateTime": "example string value"
},
"weeklyWorkdaysRepeatPattern": {
"repeatCount": 613,
"applyUntilDateTime": "example string value"
},
"monthlySameDateRepeatPattern": {
"repeatCount": 473,
"applyUntilDateTime": "example string value"
},
"monthlySameDayRepeatPattern": {
"repeatCount": 321,
"applyUntilDateTime": "example string value"
},
"annuallySameDateRepeatPattern": {
"repeatCount": 575,
"applyUntilDateTime": "example string value"
}
},
"startDateTime": "example string value",
"endDateTime": "example string value",
"areaRegionTypeIds": [
780
],
"emitterTypeIds": [
392
],
"stationNumbers": [
502
],
"allStations": true,
"effect": "example string value"
}
]
}
]
}
]
},
"filters": {
"projectControllers": {
"projectControllers": [
{
"projectId": "example string value",
"controllerIdList": [
"example string value"
]
}
]
}
},
"meta": {
"example_property_name": "example string value"
},
"storedDataUrl": "example string value"
}
HTTP Request
GET /api/v1/reports/time-restrictions-report/{id}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
id |
string |
path |
true |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
true |
|
userId |
string |
true |
|
data |
ReportData |
false |
|
filters |
ReportFilters |
true |
|
meta |
Object for field meta |
false |
|
storedDataUrl |
string |
false |
|
ReportData
Name |
Type |
Required |
Description |
projects |
Project[ ] |
true |
|
Project
Name |
Type |
Required |
Description |
id |
string |
true |
|
name |
string |
true |
|
controllers |
Controller[ ] |
true |
|
Controller
Name |
Type |
Required |
Description |
id |
string |
true |
|
name |
string |
true |
|
timeRestrictions |
TimeRestriction[ ] |
true |
|
TimeRestriction
Name |
Type |
Required |
Description |
id |
string |
true |
|
name |
string |
true |
|
repeatPattern |
RepeatPattern |
false |
|
startDateTime |
string |
true |
|
endDateTime |
string |
true |
|
areaRegionTypeIds |
integer[ ] |
false |
|
emitterTypeIds |
integer[ ] |
false |
|
stationNumbers |
integer[ ] |
false |
|
allStations |
boolean |
false |
|
effect |
string |
true |
|
RepeatPattern
Name |
Type |
Required |
Description |
dailyRepeatPattern |
DailyRepeatPattern |
false |
|
weeklyRepeatPattern |
WeeklyRepeatPattern |
false |
|
monthlyRepeatPattern |
MonthlyRepeatPattern |
false |
|
yearlyRepeatPattern |
YearlyRepeatPattern |
false |
|
oddDaysRepeatPattern |
OddDaysRepeatPattern |
false |
|
evenDaysRepeatPattern |
EvenDaysRepeatPattern |
false |
|
weeklySameDayRepeatPattern |
WeeklySameDayRepeatPattern |
false |
|
weeklyWorkdaysRepeatPattern |
WeeklyWorkdaysRepeatPattern |
false |
|
monthlySameDateRepeatPattern |
MonthlySameDateRepeatPattern |
false |
|
monthlySameDayRepeatPattern |
MonthlySameDayRepeatPattern |
false |
|
annuallySameDateRepeatPattern |
AnnuallySameDateRepeatPattern |
false |
|
DailyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
WeeklyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
daysOfWeek |
string[ ] |
false |
|
MonthlyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
daysOfMonth |
integer[ ] |
false |
|
positionInMonth |
integer |
false |
|
daysOfWeek |
string[ ] |
false |
|
YearlyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
daysOfWeek |
string[ ] |
false |
|
daysOfYear |
integer[ ] |
false |
|
numbersOfWeek |
integer[ ] |
false |
|
OddDaysRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
EvenDaysRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
WeeklySameDayRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
WeeklyWorkdaysRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
MonthlySameDateRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
MonthlySameDayRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
AnnuallySameDateRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
ReportFilters
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersFilter |
true |
|
ProjectControllersFilter
Name |
Type |
Required |
Description |
projectControllers |
ProjectControllersValue[ ] |
true |
|
ProjectControllersValue
Name |
Type |
Required |
Description |
projectId |
string |
true |
|
controllerIdList |
string[ ] |
false |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
V1 | Restrictions | Time Restrictions
Get all Time Restrictions
Request
curl "https://developer-api.etwater.com/api/v1/restrictions/time?controllerId=example+string+value&active=1&stationGroupId=example+string+value&projectId=example+string+value" \
-X GET \
-H "Authorization: example string value" \
-H "Unity-Api-Gateway-Replace-UserId: example string value"
On success, the above request returns response like
[
{
"id": "example string value",
"controller": {
"id": "example string value",
"name": "example string value"
},
"project": {
"id": "example string value",
"name": "example string value"
},
"zoneNumbers": [
55
],
"startTime": 610,
"endTime": 974,
"count": 441,
"applyUntil": 865,
"dailyRecurrence": {
"interval": "1"
},
"weeklyRecurrence": {
"interval": "1",
"dayOfWeekList": [
2
]
},
"monthlyRecurrence": {
"interval": "1",
"daysOfMonth": null,
"positionInMonth": 563,
"daysOfWeek": [
1
]
},
"yearlyRecurrence": {
"interval": "1",
"dayOfWeekList": [
2
],
"daysOfYear": null,
"numberOfWeekList": null
},
"wateringManuallyAllowed": "1",
"metadata": {
"example_property_name": "example string value"
},
"areaRegionTypeIds": [
724
],
"emitterTypeIds": [
623
],
"municipalRestrictionId": "example string value",
"modified": true,
"basicMunicipalRestriction": {
"id": "example string value",
"controllerId": "example string value",
"zoneNumbers": [
272
],
"startTime": 439,
"endTime": 745,
"count": 670,
"applyUntil": 386,
"dailyRecurrence": {
"interval": "1"
},
"weeklyRecurrence": {
"interval": "1",
"dayOfWeekList": [
5
]
},
"monthlyRecurrence": {
"interval": "1",
"daysOfMonth": null,
"positionInMonth": 123,
"daysOfWeek": [
4
]
},
"yearlyRecurrence": {
"interval": "1",
"dayOfWeekList": [
1
],
"daysOfYear": null,
"numberOfWeekList": null
},
"wateringManuallyAllowed": "1",
"metadata": {
"example_property_name": "example string value"
},
"areaRegionTypeIds": [
780
],
"emitterTypeIds": [
845
],
"municipalRestrictionId": "example string value",
"modified": true,
"basicMunicipalRestriction": {
"id": "example string value",
"controllerId": "example string value",
"zoneNumbers": [
628
],
"startTime": 618,
"endTime": 215,
"count": 163,
"applyUntil": 256,
"dailyRecurrence": {
"interval": "1"
},
"weeklyRecurrence": {
"interval": "1",
"dayOfWeekList": [
3
]
},
"monthlyRecurrence": {
"interval": "1",
"daysOfMonth": null,
"positionInMonth": 568,
"daysOfWeek": [
5
]
},
"yearlyRecurrence": {
"interval": "1",
"dayOfWeekList": [
6
],
"daysOfYear": null,
"numberOfWeekList": null
},
"wateringManuallyAllowed": "1",
"metadata": {
"example_property_name": "example string value"
},
"areaRegionTypeIds": [
584
],
"emitterTypeIds": [
410
],
"municipalRestrictionId": "example string value",
"modified": false,
"basicMunicipalRestriction": null,
"name": "example string value",
"weatherRestrictionId": "example string value",
"userId": "example string value",
"allZones": false
},
"name": "example string value",
"weatherRestrictionId": "example string value",
"userId": "example string value",
"allZones": true
},
"name": "example string value",
"weatherRestrictionId": "example string value",
"userId": "example string value",
"allZones": false
}
]
HTTP Request
GET /api/v1/restrictions/time
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Unity-Api-Gateway-Replace-UserId |
string |
header |
false |
|
controllerId |
string |
query |
false |
|
active |
boolean |
query |
false |
|
stationGroupId |
string |
query |
false |
|
projectId |
string |
query |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response is array of
Name |
Type |
Required |
Description |
id |
string |
false |
|
controller |
Controller |
false |
|
project |
Project |
false |
|
zoneNumbers |
integer[ ] |
false |
|
startTime |
integer |
false |
|
endTime |
integer |
false |
|
count |
integer |
false |
|
applyUntil |
integer |
false |
|
dailyRecurrence |
DailyRecurrence |
false |
|
weeklyRecurrence |
WeeklyRecurrence |
false |
|
monthlyRecurrence |
MonthlyRecurrence |
false |
|
yearlyRecurrence |
YearlyRecurrence |
false |
|
wateringManuallyAllowed |
boolean |
false |
|
metadata |
Object for field metadata |
false |
|
areaRegionTypeIds |
integer[ ] |
false |
|
emitterTypeIds |
integer[ ] |
false |
|
municipalRestrictionId |
string |
false |
|
modified |
boolean |
false |
|
basicMunicipalRestriction |
TimeRestriction |
false |
|
name |
string |
false |
|
weatherRestrictionId |
string |
false |
|
userId |
string |
false |
|
allZones |
boolean |
false |
|
Controller
Name |
Type |
Required |
Description |
id |
string |
true |
|
name |
string |
true |
|
Project
Name |
Type |
Required |
Description |
id |
string |
true |
|
name |
string |
true |
|
DailyRecurrence
Name |
Type |
Required |
Description |
interval |
integer |
false |
|
WeeklyRecurrence
Name |
Type |
Required |
Description |
interval |
integer |
false |
|
dayOfWeekList |
integer[ ] |
false |
|
MonthlyRecurrence
Name |
Type |
Required |
Description |
interval |
integer |
false |
|
daysOfMonth |
integer[ ] |
false |
|
positionInMonth |
integer |
false |
|
daysOfWeek |
integer[ ] |
false |
|
YearlyRecurrence
Name |
Type |
Required |
Description |
interval |
integer |
false |
|
dayOfWeekList |
integer[ ] |
false |
|
daysOfYear |
integer[ ] |
false |
|
numberOfWeekList |
integer[ ] |
false |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
TimeRestriction
Name |
Type |
Required |
Description |
id |
string |
false |
|
controllerId |
string |
false |
|
zoneNumbers |
integer[ ] |
false |
|
startTime |
integer |
false |
|
endTime |
integer |
false |
|
count |
integer |
false |
|
applyUntil |
integer |
false |
|
dailyRecurrence |
DailyRecurrence |
false |
|
weeklyRecurrence |
WeeklyRecurrence |
false |
|
monthlyRecurrence |
MonthlyRecurrence |
false |
|
yearlyRecurrence |
YearlyRecurrence |
false |
|
wateringManuallyAllowed |
boolean |
false |
|
metadata |
Object for field metadata |
false |
|
areaRegionTypeIds |
integer[ ] |
false |
|
emitterTypeIds |
integer[ ] |
false |
|
municipalRestrictionId |
string |
false |
|
modified |
boolean |
false |
|
basicMunicipalRestriction |
TimeRestriction |
false |
|
name |
string |
false |
|
weatherRestrictionId |
string |
false |
|
userId |
string |
false |
|
allZones |
boolean |
false |
|
Create a Time Restriction
Request
curl "https://developer-api.etwater.com/api/v1/restrictions/time" \
-X POST \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
-H "Unity-Api-Gateway-Replace-UserId: example string value" \
--data "{
\"id\": \"example string value\",
\"controllerId\": \"example string value\",
\"zoneNumbers\": [
101
],
\"startTime\": 93,
\"endTime\": 218,
\"count\": 527,
\"applyUntil\": 858,
\"dailyRecurrence\": {
\"interval\": \"1\"
},
\"weeklyRecurrence\": {
\"interval\": \"1\",
\"dayOfWeekList\": [
0
]
},
\"monthlyRecurrence\": {
\"interval\": \"1\",
\"daysOfMonth\": null,
\"positionInMonth\": 381,
\"daysOfWeek\": [
4
]
},
\"yearlyRecurrence\": {
\"interval\": \"1\",
\"dayOfWeekList\": [
3
],
\"daysOfYear\": null,
\"numberOfWeekList\": null
},
\"wateringManuallyAllowed\": \"1\",
\"metadata\": {
\"example_property_name\": \"example string value\"
},
\"areaRegionTypeIds\": [
725
],
\"emitterTypeIds\": [
276
],
\"municipalRestrictionId\": \"example string value\",
\"modified\": false,
\"basicMunicipalRestriction\": {
\"id\": \"example string value\",
\"controllerId\": \"example string value\",
\"zoneNumbers\": [
924
],
\"startTime\": 436,
\"endTime\": 173,
\"count\": 738,
\"applyUntil\": 717,
\"dailyRecurrence\": {
\"interval\": \"1\"
},
\"weeklyRecurrence\": {
\"interval\": \"1\",
\"dayOfWeekList\": [
2
]
},
\"monthlyRecurrence\": {
\"interval\": \"1\",
\"daysOfMonth\": null,
\"positionInMonth\": 663,
\"daysOfWeek\": [
0
]
},
\"yearlyRecurrence\": {
\"interval\": \"1\",
\"dayOfWeekList\": [
2
],
\"daysOfYear\": null,
\"numberOfWeekList\": null
},
\"wateringManuallyAllowed\": \"1\",
\"metadata\": {
\"example_property_name\": \"example string value\"
},
\"areaRegionTypeIds\": [
287
],
\"emitterTypeIds\": [
61
],
\"municipalRestrictionId\": \"example string value\",
\"modified\": false,
\"basicMunicipalRestriction\": null,
\"name\": \"example string value\",
\"weatherRestrictionId\": \"example string value\",
\"userId\": \"example string value\",
\"allZones\": true
},
\"name\": \"example string value\",
\"weatherRestrictionId\": \"example string value\",
\"userId\": \"example string value\",
\"allZones\": true
}"
On success, the above request returns response like
HTTP Request
POST /api/v1/restrictions/time
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
Unity-Api-Gateway-Replace-UserId |
string |
header |
false |
|
TimeRestriction |
TimeRestriction |
body |
true |
|
TimeRestriction
Name |
Type |
Required |
Description |
id |
string |
false |
|
controllerId |
string |
false |
|
zoneNumbers |
integer[ ] |
false |
|
startTime |
integer |
false |
|
endTime |
integer |
false |
|
count |
integer |
false |
|
applyUntil |
integer |
false |
|
dailyRecurrence |
DailyRecurrence |
false |
|
weeklyRecurrence |
WeeklyRecurrence |
false |
|
monthlyRecurrence |
MonthlyRecurrence |
false |
|
yearlyRecurrence |
YearlyRecurrence |
false |
|
wateringManuallyAllowed |
boolean |
false |
|
metadata |
Object for field metadata |
false |
|
areaRegionTypeIds |
integer[ ] |
false |
|
emitterTypeIds |
integer[ ] |
false |
|
municipalRestrictionId |
string |
false |
|
modified |
boolean |
false |
|
basicMunicipalRestriction |
TimeRestriction |
false |
|
name |
string |
false |
|
weatherRestrictionId |
string |
false |
|
userId |
string |
false |
|
allZones |
boolean |
false |
|
DailyRecurrence
Name |
Type |
Required |
Description |
interval |
integer |
false |
|
WeeklyRecurrence
Name |
Type |
Required |
Description |
interval |
integer |
false |
|
dayOfWeekList |
integer[ ] |
false |
|
MonthlyRecurrence
Name |
Type |
Required |
Description |
interval |
integer |
false |
|
daysOfMonth |
integer[ ] |
false |
|
positionInMonth |
integer |
false |
|
daysOfWeek |
integer[ ] |
false |
|
YearlyRecurrence
Name |
Type |
Required |
Description |
interval |
integer |
false |
|
dayOfWeekList |
integer[ ] |
false |
|
daysOfYear |
integer[ ] |
false |
|
numberOfWeekList |
integer[ ] |
false |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
HTTP Responses
Code |
Description |
201 |
Created |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
false |
|
controllerId |
string |
false |
|
zoneNumbers |
integer[ ] |
false |
|
startTime |
integer |
false |
|
endTime |
integer |
false |
|
count |
integer |
false |
|
applyUntil |
integer |
false |
|
dailyRecurrence |
DailyRecurrence |
false |
|
weeklyRecurrence |
WeeklyRecurrence |
false |
|
monthlyRecurrence |
MonthlyRecurrence |
false |
|
yearlyRecurrence |
YearlyRecurrence |
false |
|
wateringManuallyAllowed |
boolean |
false |
|
metadata |
Object for field metadata |
false |
|
areaRegionTypeIds |
integer[ ] |
false |
|
emitterTypeIds |
integer[ ] |
false |
|
municipalRestrictionId |
string |
false |
|
modified |
boolean |
false |
|
basicMunicipalRestriction |
TimeRestriction |
false |
|
name |
string |
false |
|
weatherRestrictionId |
string |
false |
|
userId |
string |
false |
|
allZones |
boolean |
false |
|
DailyRecurrence
Name |
Type |
Required |
Description |
interval |
integer |
false |
|
WeeklyRecurrence
Name |
Type |
Required |
Description |
interval |
integer |
false |
|
dayOfWeekList |
integer[ ] |
false |
|
MonthlyRecurrence
Name |
Type |
Required |
Description |
interval |
integer |
false |
|
daysOfMonth |
integer[ ] |
false |
|
positionInMonth |
integer |
false |
|
daysOfWeek |
integer[ ] |
false |
|
YearlyRecurrence
Name |
Type |
Required |
Description |
interval |
integer |
false |
|
dayOfWeekList |
integer[ ] |
false |
|
daysOfYear |
integer[ ] |
false |
|
numberOfWeekList |
integer[ ] |
false |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
TimeRestriction
Name |
Type |
Required |
Description |
id |
string |
false |
|
controllerId |
string |
false |
|
zoneNumbers |
integer[ ] |
false |
|
startTime |
integer |
false |
|
endTime |
integer |
false |
|
count |
integer |
false |
|
applyUntil |
integer |
false |
|
dailyRecurrence |
DailyRecurrence |
false |
|
weeklyRecurrence |
WeeklyRecurrence |
false |
|
monthlyRecurrence |
MonthlyRecurrence |
false |
|
yearlyRecurrence |
YearlyRecurrence |
false |
|
wateringManuallyAllowed |
boolean |
false |
|
metadata |
Object for field metadata |
false |
|
areaRegionTypeIds |
integer[ ] |
false |
|
emitterTypeIds |
integer[ ] |
false |
|
municipalRestrictionId |
string |
false |
|
modified |
boolean |
false |
|
basicMunicipalRestriction |
TimeRestriction |
false |
|
name |
string |
false |
|
weatherRestrictionId |
string |
false |
|
userId |
string |
false |
|
allZones |
boolean |
false |
|
Get a Time Restriction by id
Request
curl "https://developer-api.etwater.com/api/v1/restrictions/time/{id}" \
-X GET \
-H "Authorization: example string value" \
-H "Unity-Api-Gateway-Replace-UserId: example string value"
On success, the above request returns response like
{
"id": "example string value",
"controllerId": "example string value",
"zoneNumbers": [
498
],
"startTime": 922,
"endTime": 265,
"count": 597,
"applyUntil": 938,
"dailyRecurrence": {
"interval": "1"
},
"weeklyRecurrence": {
"interval": "1",
"dayOfWeekList": [
0
]
},
"monthlyRecurrence": {
"interval": "1",
"daysOfMonth": null,
"positionInMonth": 971,
"daysOfWeek": [
3
]
},
"yearlyRecurrence": {
"interval": "1",
"dayOfWeekList": [
3
],
"daysOfYear": null,
"numberOfWeekList": null
},
"wateringManuallyAllowed": "1",
"metadata": {
"example_property_name": "example string value"
},
"areaRegionTypeIds": [
27
],
"emitterTypeIds": [
280
],
"municipalRestrictionId": "example string value",
"modified": true,
"basicMunicipalRestriction": {
"id": "example string value",
"controllerId": "example string value",
"zoneNumbers": [
254
],
"startTime": 878,
"endTime": 300,
"count": 729,
"applyUntil": 632,
"dailyRecurrence": {
"interval": "1"
},
"weeklyRecurrence": {
"interval": "1",
"dayOfWeekList": [
3
]
},
"monthlyRecurrence": {
"interval": "1",
"daysOfMonth": null,
"positionInMonth": 89,
"daysOfWeek": [
2
]
},
"yearlyRecurrence": {
"interval": "1",
"dayOfWeekList": [
4
],
"daysOfYear": null,
"numberOfWeekList": null
},
"wateringManuallyAllowed": "1",
"metadata": {
"example_property_name": "example string value"
},
"areaRegionTypeIds": [
83
],
"emitterTypeIds": [
829
],
"municipalRestrictionId": "example string value",
"modified": false,
"basicMunicipalRestriction": null,
"name": "example string value",
"weatherRestrictionId": "example string value",
"userId": "example string value",
"allZones": false
},
"name": "example string value",
"weatherRestrictionId": "example string value",
"userId": "example string value",
"allZones": false
}
HTTP Request
GET /api/v1/restrictions/time/{id}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Unity-Api-Gateway-Replace-UserId |
string |
header |
false |
|
id |
string |
path |
true |
|
HTTP Responses
Code |
Description |
200 |
OK |
404 |
Not Found |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
false |
|
controllerId |
string |
false |
|
zoneNumbers |
integer[ ] |
false |
|
startTime |
integer |
false |
|
endTime |
integer |
false |
|
count |
integer |
false |
|
applyUntil |
integer |
false |
|
dailyRecurrence |
DailyRecurrence |
false |
|
weeklyRecurrence |
WeeklyRecurrence |
false |
|
monthlyRecurrence |
MonthlyRecurrence |
false |
|
yearlyRecurrence |
YearlyRecurrence |
false |
|
wateringManuallyAllowed |
boolean |
false |
|
metadata |
Object for field metadata |
false |
|
areaRegionTypeIds |
integer[ ] |
false |
|
emitterTypeIds |
integer[ ] |
false |
|
municipalRestrictionId |
string |
false |
|
modified |
boolean |
false |
|
basicMunicipalRestriction |
TimeRestriction |
false |
|
name |
string |
false |
|
weatherRestrictionId |
string |
false |
|
userId |
string |
false |
|
allZones |
boolean |
false |
|
DailyRecurrence
Name |
Type |
Required |
Description |
interval |
integer |
false |
|
WeeklyRecurrence
Name |
Type |
Required |
Description |
interval |
integer |
false |
|
dayOfWeekList |
integer[ ] |
false |
|
MonthlyRecurrence
Name |
Type |
Required |
Description |
interval |
integer |
false |
|
daysOfMonth |
integer[ ] |
false |
|
positionInMonth |
integer |
false |
|
daysOfWeek |
integer[ ] |
false |
|
YearlyRecurrence
Name |
Type |
Required |
Description |
interval |
integer |
false |
|
dayOfWeekList |
integer[ ] |
false |
|
daysOfYear |
integer[ ] |
false |
|
numberOfWeekList |
integer[ ] |
false |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
TimeRestriction
Name |
Type |
Required |
Description |
id |
string |
false |
|
controllerId |
string |
false |
|
zoneNumbers |
integer[ ] |
false |
|
startTime |
integer |
false |
|
endTime |
integer |
false |
|
count |
integer |
false |
|
applyUntil |
integer |
false |
|
dailyRecurrence |
DailyRecurrence |
false |
|
weeklyRecurrence |
WeeklyRecurrence |
false |
|
monthlyRecurrence |
MonthlyRecurrence |
false |
|
yearlyRecurrence |
YearlyRecurrence |
false |
|
wateringManuallyAllowed |
boolean |
false |
|
metadata |
Object for field metadata |
false |
|
areaRegionTypeIds |
integer[ ] |
false |
|
emitterTypeIds |
integer[ ] |
false |
|
municipalRestrictionId |
string |
false |
|
modified |
boolean |
false |
|
basicMunicipalRestriction |
TimeRestriction |
false |
|
name |
string |
false |
|
weatherRestrictionId |
string |
false |
|
userId |
string |
false |
|
allZones |
boolean |
false |
|
Delete a Time Restriction by id
Request
curl "https://developer-api.etwater.com/api/v1/restrictions/time/{id}" \
-X DELETE \
-H "Authorization: example string value" \
-H "Unity-Api-Gateway-Replace-UserId: example string value"
On success, the above request returns response like
HTTP Request
DELETE /api/v1/restrictions/time/{id}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Unity-Api-Gateway-Replace-UserId |
string |
header |
false |
|
id |
string |
path |
true |
|
HTTP Responses
Code |
Description |
204 |
No Content |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Update a Schedule Restriction by id
Request
curl "https://developer-api.etwater.com/api/v1/restrictions/time/{id}" \
-X PUT \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
-H "Unity-Api-Gateway-Replace-UserId: example string value" \
--data "{
\"id\": \"example string value\",
\"controllerId\": \"example string value\",
\"zoneNumbers\": [
789
],
\"startTime\": 561,
\"endTime\": 789,
\"count\": 140,
\"applyUntil\": 371,
\"dailyRecurrence\": {
\"interval\": \"1\"
},
\"weeklyRecurrence\": {
\"interval\": \"1\",
\"dayOfWeekList\": [
6
]
},
\"monthlyRecurrence\": {
\"interval\": \"1\",
\"daysOfMonth\": null,
\"positionInMonth\": 600,
\"daysOfWeek\": [
4
]
},
\"yearlyRecurrence\": {
\"interval\": \"1\",
\"dayOfWeekList\": [
4
],
\"daysOfYear\": null,
\"numberOfWeekList\": null
},
\"wateringManuallyAllowed\": \"1\",
\"metadata\": {
\"example_property_name\": \"example string value\"
},
\"areaRegionTypeIds\": [
316
],
\"emitterTypeIds\": [
336
],
\"municipalRestrictionId\": \"example string value\",
\"modified\": true,
\"basicMunicipalRestriction\": {
\"id\": \"example string value\",
\"controllerId\": \"example string value\",
\"zoneNumbers\": [
650
],
\"startTime\": 62,
\"endTime\": 345,
\"count\": 122,
\"applyUntil\": 1000,
\"dailyRecurrence\": {
\"interval\": \"1\"
},
\"weeklyRecurrence\": {
\"interval\": \"1\",
\"dayOfWeekList\": [
1
]
},
\"monthlyRecurrence\": {
\"interval\": \"1\",
\"daysOfMonth\": null,
\"positionInMonth\": 994,
\"daysOfWeek\": [
2
]
},
\"yearlyRecurrence\": {
\"interval\": \"1\",
\"dayOfWeekList\": [
3
],
\"daysOfYear\": null,
\"numberOfWeekList\": null
},
\"wateringManuallyAllowed\": \"1\",
\"metadata\": {
\"example_property_name\": \"example string value\"
},
\"areaRegionTypeIds\": [
94
],
\"emitterTypeIds\": [
627
],
\"municipalRestrictionId\": \"example string value\",
\"modified\": true,
\"basicMunicipalRestriction\": null,
\"name\": \"example string value\",
\"weatherRestrictionId\": \"example string value\",
\"userId\": \"example string value\",
\"allZones\": false
},
\"name\": \"example string value\",
\"weatherRestrictionId\": \"example string value\",
\"userId\": \"example string value\",
\"allZones\": true
}"
On success, the above request returns response like
{
"id": "example string value",
"controllerId": "example string value",
"zoneNumbers": [
693
],
"startTime": 71,
"endTime": 645,
"count": 801,
"applyUntil": 498,
"dailyRecurrence": {
"interval": "1"
},
"weeklyRecurrence": {
"interval": "1",
"dayOfWeekList": [
6
]
},
"monthlyRecurrence": {
"interval": "1",
"daysOfMonth": null,
"positionInMonth": 977,
"daysOfWeek": [
1
]
},
"yearlyRecurrence": {
"interval": "1",
"dayOfWeekList": [
2
],
"daysOfYear": null,
"numberOfWeekList": null
},
"wateringManuallyAllowed": "1",
"metadata": {
"example_property_name": "example string value"
},
"areaRegionTypeIds": [
241
],
"emitterTypeIds": [
367
],
"municipalRestrictionId": "example string value",
"modified": false,
"basicMunicipalRestriction": {
"id": "example string value",
"controllerId": "example string value",
"zoneNumbers": [
488
],
"startTime": 82,
"endTime": 277,
"count": 874,
"applyUntil": 245,
"dailyRecurrence": {
"interval": "1"
},
"weeklyRecurrence": {
"interval": "1",
"dayOfWeekList": [
5
]
},
"monthlyRecurrence": {
"interval": "1",
"daysOfMonth": null,
"positionInMonth": 227,
"daysOfWeek": [
3
]
},
"yearlyRecurrence": {
"interval": "1",
"dayOfWeekList": [
4
],
"daysOfYear": null,
"numberOfWeekList": null
},
"wateringManuallyAllowed": "1",
"metadata": {
"example_property_name": "example string value"
},
"areaRegionTypeIds": [
687
],
"emitterTypeIds": [
306
],
"municipalRestrictionId": "example string value",
"modified": true,
"basicMunicipalRestriction": null,
"name": "example string value",
"weatherRestrictionId": "example string value",
"userId": "example string value",
"allZones": true
},
"name": "example string value",
"weatherRestrictionId": "example string value",
"userId": "example string value",
"allZones": true
}
HTTP Request
PUT /api/v1/restrictions/time/{id}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
Unity-Api-Gateway-Replace-UserId |
string |
header |
false |
|
id |
string |
path |
true |
|
TimeRestriction |
TimeRestriction |
body |
true |
|
TimeRestriction
Name |
Type |
Required |
Description |
id |
string |
false |
|
controllerId |
string |
false |
|
zoneNumbers |
integer[ ] |
false |
|
startTime |
integer |
false |
|
endTime |
integer |
false |
|
count |
integer |
false |
|
applyUntil |
integer |
false |
|
dailyRecurrence |
DailyRecurrence |
false |
|
weeklyRecurrence |
WeeklyRecurrence |
false |
|
monthlyRecurrence |
MonthlyRecurrence |
false |
|
yearlyRecurrence |
YearlyRecurrence |
false |
|
wateringManuallyAllowed |
boolean |
false |
|
metadata |
Object for field metadata |
false |
|
areaRegionTypeIds |
integer[ ] |
false |
|
emitterTypeIds |
integer[ ] |
false |
|
municipalRestrictionId |
string |
false |
|
modified |
boolean |
false |
|
basicMunicipalRestriction |
TimeRestriction |
false |
|
name |
string |
false |
|
weatherRestrictionId |
string |
false |
|
userId |
string |
false |
|
allZones |
boolean |
false |
|
DailyRecurrence
Name |
Type |
Required |
Description |
interval |
integer |
false |
|
WeeklyRecurrence
Name |
Type |
Required |
Description |
interval |
integer |
false |
|
dayOfWeekList |
integer[ ] |
false |
|
MonthlyRecurrence
Name |
Type |
Required |
Description |
interval |
integer |
false |
|
daysOfMonth |
integer[ ] |
false |
|
positionInMonth |
integer |
false |
|
daysOfWeek |
integer[ ] |
false |
|
YearlyRecurrence
Name |
Type |
Required |
Description |
interval |
integer |
false |
|
dayOfWeekList |
integer[ ] |
false |
|
daysOfYear |
integer[ ] |
false |
|
numberOfWeekList |
integer[ ] |
false |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
false |
|
controllerId |
string |
false |
|
zoneNumbers |
integer[ ] |
false |
|
startTime |
integer |
false |
|
endTime |
integer |
false |
|
count |
integer |
false |
|
applyUntil |
integer |
false |
|
dailyRecurrence |
DailyRecurrence |
false |
|
weeklyRecurrence |
WeeklyRecurrence |
false |
|
monthlyRecurrence |
MonthlyRecurrence |
false |
|
yearlyRecurrence |
YearlyRecurrence |
false |
|
wateringManuallyAllowed |
boolean |
false |
|
metadata |
Object for field metadata |
false |
|
areaRegionTypeIds |
integer[ ] |
false |
|
emitterTypeIds |
integer[ ] |
false |
|
municipalRestrictionId |
string |
false |
|
modified |
boolean |
false |
|
basicMunicipalRestriction |
TimeRestriction |
false |
|
name |
string |
false |
|
weatherRestrictionId |
string |
false |
|
userId |
string |
false |
|
allZones |
boolean |
false |
|
DailyRecurrence
Name |
Type |
Required |
Description |
interval |
integer |
false |
|
WeeklyRecurrence
Name |
Type |
Required |
Description |
interval |
integer |
false |
|
dayOfWeekList |
integer[ ] |
false |
|
MonthlyRecurrence
Name |
Type |
Required |
Description |
interval |
integer |
false |
|
daysOfMonth |
integer[ ] |
false |
|
positionInMonth |
integer |
false |
|
daysOfWeek |
integer[ ] |
false |
|
YearlyRecurrence
Name |
Type |
Required |
Description |
interval |
integer |
false |
|
dayOfWeekList |
integer[ ] |
false |
|
daysOfYear |
integer[ ] |
false |
|
numberOfWeekList |
integer[ ] |
false |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
TimeRestriction
Name |
Type |
Required |
Description |
id |
string |
false |
|
controllerId |
string |
false |
|
zoneNumbers |
integer[ ] |
false |
|
startTime |
integer |
false |
|
endTime |
integer |
false |
|
count |
integer |
false |
|
applyUntil |
integer |
false |
|
dailyRecurrence |
DailyRecurrence |
false |
|
weeklyRecurrence |
WeeklyRecurrence |
false |
|
monthlyRecurrence |
MonthlyRecurrence |
false |
|
yearlyRecurrence |
YearlyRecurrence |
false |
|
wateringManuallyAllowed |
boolean |
false |
|
metadata |
Object for field metadata |
false |
|
areaRegionTypeIds |
integer[ ] |
false |
|
emitterTypeIds |
integer[ ] |
false |
|
municipalRestrictionId |
string |
false |
|
modified |
boolean |
false |
|
basicMunicipalRestriction |
TimeRestriction |
false |
|
name |
string |
false |
|
weatherRestrictionId |
string |
false |
|
userId |
string |
false |
|
allZones |
boolean |
false |
|
Copy Time Restriction
Request
curl "https://developer-api.etwater.com/api/v1/restrictions/time/{id}/rpc-copy" \
-X POST \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
--data "{
\"sourceId\": \"example string value\",
\"destinationName\": \"example string value\",
\"destinationControllerId\": \"example string value\"
}"
On success, the above request returns response like
HTTP Request
POST /api/v1/restrictions/time/{id}/rpc-copy
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
id |
string |
path |
true |
|
TimeRestriction |
CopyTimeRestrictionRequest |
body |
true |
|
CopyTimeRestrictionRequest
Name |
Type |
Required |
Description |
sourceId |
string |
true |
|
destinationName |
string |
false |
|
destinationControllerId |
string |
true |
|
HTTP Responses
Code |
Description |
201 |
Created |
404 |
Not Found |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
false |
|
controllerId |
string |
false |
|
zoneNumbers |
integer[ ] |
false |
|
startTime |
integer |
false |
|
endTime |
integer |
false |
|
count |
integer |
false |
|
applyUntil |
integer |
false |
|
dailyRecurrence |
DailyRecurrence |
false |
|
weeklyRecurrence |
WeeklyRecurrence |
false |
|
monthlyRecurrence |
MonthlyRecurrence |
false |
|
yearlyRecurrence |
YearlyRecurrence |
false |
|
wateringManuallyAllowed |
boolean |
false |
|
metadata |
Object for field metadata |
false |
|
areaRegionTypeIds |
integer[ ] |
false |
|
emitterTypeIds |
integer[ ] |
false |
|
municipalRestrictionId |
string |
false |
|
modified |
boolean |
false |
|
basicMunicipalRestriction |
TimeRestriction |
false |
|
name |
string |
false |
|
weatherRestrictionId |
string |
false |
|
userId |
string |
false |
|
allZones |
boolean |
false |
|
DailyRecurrence
Name |
Type |
Required |
Description |
interval |
integer |
false |
|
WeeklyRecurrence
Name |
Type |
Required |
Description |
interval |
integer |
false |
|
dayOfWeekList |
integer[ ] |
false |
|
MonthlyRecurrence
Name |
Type |
Required |
Description |
interval |
integer |
false |
|
daysOfMonth |
integer[ ] |
false |
|
positionInMonth |
integer |
false |
|
daysOfWeek |
integer[ ] |
false |
|
YearlyRecurrence
Name |
Type |
Required |
Description |
interval |
integer |
false |
|
dayOfWeekList |
integer[ ] |
false |
|
daysOfYear |
integer[ ] |
false |
|
numberOfWeekList |
integer[ ] |
false |
|
Name |
Type |
Required |
Description |
* |
string |
false |
|
TimeRestriction
Name |
Type |
Required |
Description |
id |
string |
false |
|
controllerId |
string |
false |
|
zoneNumbers |
integer[ ] |
false |
|
startTime |
integer |
false |
|
endTime |
integer |
false |
|
count |
integer |
false |
|
applyUntil |
integer |
false |
|
dailyRecurrence |
DailyRecurrence |
false |
|
weeklyRecurrence |
WeeklyRecurrence |
false |
|
monthlyRecurrence |
MonthlyRecurrence |
false |
|
yearlyRecurrence |
YearlyRecurrence |
false |
|
wateringManuallyAllowed |
boolean |
false |
|
metadata |
Object for field metadata |
false |
|
areaRegionTypeIds |
integer[ ] |
false |
|
emitterTypeIds |
integer[ ] |
false |
|
municipalRestrictionId |
string |
false |
|
modified |
boolean |
false |
|
basicMunicipalRestriction |
TimeRestriction |
false |
|
name |
string |
false |
|
weatherRestrictionId |
string |
false |
|
userId |
string |
false |
|
allZones |
boolean |
false |
|
V1 | Restrictions | Weather Restrictions
The Unity API will automatically pick the best time during the day or night when to irrigate based on the weather, the soil and the plant needs. Use the Restrictions Weather API to set and manage when to disallow irrigation based on weather conditions, such as with high winds, low temperatures, after it rained or when rain is forecast. Each can be set per zone to its own threshold.
Get all Weather Restrictions
Request
curl "https://developer-api.etwater.com/api/v1/restrictions/weather?controllerId=example+string+value" \
-X GET \
-H "Authorization: example string value" \
-H "Unity-Api-Gateway-Replace-UserId: example string value"
On success, the above request returns response like
[
{
"id": "example string value",
"userId": "example string value",
"controllerId": "example string value",
"zoneNumbers": [
346
],
"wateringInRain": false,
"rainProbability": 472,
"minWateringTemperature": 837,
"wateringAfterRain": 172,
"highWind": false,
"createdAt": 848,
"updatedAt": 655,
"municipalRestrictionId": "example string value",
"modified": true,
"basicMunicipalRestriction": {
"id": "example string value",
"userId": "example string value",
"controllerId": "example string value",
"zoneNumbers": [
395
],
"wateringInRain": true,
"rainProbability": 425,
"minWateringTemperature": 232,
"wateringAfterRain": 364,
"highWind": false,
"createdAt": 485,
"updatedAt": 555,
"municipalRestrictionId": "example string value",
"modified": true,
"basicMunicipalRestriction": null,
"name": "example string value",
"areaId": "example string value",
"denyWateringRainAmount": 767.6380107959909,
"allZones": false,
"forbiddenWateringBeforeRainHours": 409,
"rainSensitivityLevel": "example string value"
},
"name": "example string value",
"areaId": "example string value",
"denyWateringRainAmount": 626,
"allZones": true,
"forbiddenWateringBeforeRainHours": 645,
"rainSensitivityLevel": "example string value"
}
]
HTTP Request
GET /api/v1/restrictions/weather
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Unity-Api-Gateway-Replace-UserId |
string |
header |
false |
|
controllerId |
string |
query |
true |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response is array of
Name |
Type |
Required |
Description |
id |
string |
false |
|
userId |
string |
false |
|
controllerId |
string |
true |
|
zoneNumbers |
integer[ ] |
false |
|
wateringInRain |
boolean |
false |
|
rainProbability |
integer |
false |
|
minWateringTemperature |
number |
false |
|
wateringAfterRain |
integer |
false |
|
highWind |
boolean |
false |
|
createdAt |
integer |
false |
|
updatedAt |
integer |
false |
|
municipalRestrictionId |
string |
false |
|
modified |
boolean |
false |
|
basicMunicipalRestriction |
WeatherConstraint |
false |
|
name |
string |
false |
|
areaId |
string |
false |
|
denyWateringRainAmount |
number |
false |
|
allZones |
boolean |
false |
|
forbiddenWateringBeforeRainHours |
integer |
false |
|
rainSensitivityLevel |
string |
false |
|
WeatherConstraint
Name |
Type |
Required |
Description |
id |
string |
false |
|
userId |
string |
false |
|
controllerId |
string |
true |
|
zoneNumbers |
integer[ ] |
false |
|
wateringInRain |
boolean |
false |
|
rainProbability |
integer |
false |
|
minWateringTemperature |
number |
false |
|
wateringAfterRain |
integer |
false |
|
highWind |
boolean |
false |
|
createdAt |
integer |
false |
|
updatedAt |
integer |
false |
|
municipalRestrictionId |
string |
false |
|
modified |
boolean |
false |
|
basicMunicipalRestriction |
WeatherConstraint |
false |
|
name |
string |
false |
|
areaId |
string |
false |
|
denyWateringRainAmount |
number |
false |
|
allZones |
boolean |
false |
|
forbiddenWateringBeforeRainHours |
integer |
false |
|
rainSensitivityLevel |
string |
false |
|
Create a Time Restriction
Request
curl "https://developer-api.etwater.com/api/v1/restrictions/weather" \
-X POST \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
-H "Unity-Api-Gateway-Replace-UserId: example string value" \
--data "{
\"id\": \"example string value\",
\"userId\": \"example string value\",
\"controllerId\": \"example string value\",
\"zoneNumbers\": [
854
],
\"wateringInRain\": false,
\"rainProbability\": 225,
\"minWateringTemperature\": 83.25523747282811,
\"wateringAfterRain\": 420,
\"highWind\": true,
\"createdAt\": 214,
\"updatedAt\": 217,
\"municipalRestrictionId\": \"example string value\",
\"modified\": false,
\"basicMunicipalRestriction\": {
\"id\": \"example string value\",
\"userId\": \"example string value\",
\"controllerId\": \"example string value\",
\"zoneNumbers\": [
822
],
\"wateringInRain\": true,
\"rainProbability\": 407,
\"minWateringTemperature\": 380.6093807241923,
\"wateringAfterRain\": 502,
\"highWind\": false,
\"createdAt\": 303,
\"updatedAt\": 696,
\"municipalRestrictionId\": \"example string value\",
\"modified\": true,
\"basicMunicipalRestriction\": null,
\"name\": \"example string value\",
\"areaId\": \"example string value\",
\"denyWateringRainAmount\": 80,
\"allZones\": true,
\"forbiddenWateringBeforeRainHours\": 300,
\"rainSensitivityLevel\": \"example string value\"
},
\"name\": \"example string value\",
\"areaId\": \"example string value\",
\"denyWateringRainAmount\": 778,
\"allZones\": false,
\"forbiddenWateringBeforeRainHours\": 431,
\"rainSensitivityLevel\": \"example string value\"
}"
On success, the above request returns response like
HTTP Request
POST /api/v1/restrictions/weather
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
Unity-Api-Gateway-Replace-UserId |
string |
header |
false |
|
WeatherConstraint |
WeatherConstraint |
body |
true |
|
WeatherConstraint
Name |
Type |
Required |
Description |
id |
string |
false |
|
userId |
string |
false |
|
controllerId |
string |
true |
|
zoneNumbers |
integer[ ] |
false |
|
wateringInRain |
boolean |
false |
|
rainProbability |
integer |
false |
|
minWateringTemperature |
number |
false |
|
wateringAfterRain |
integer |
false |
|
highWind |
boolean |
false |
|
createdAt |
integer |
false |
|
updatedAt |
integer |
false |
|
municipalRestrictionId |
string |
false |
|
modified |
boolean |
false |
|
basicMunicipalRestriction |
WeatherConstraint |
false |
|
name |
string |
false |
|
areaId |
string |
false |
|
denyWateringRainAmount |
number |
false |
|
allZones |
boolean |
false |
|
forbiddenWateringBeforeRainHours |
integer |
false |
|
rainSensitivityLevel |
string |
false |
|
HTTP Responses
Code |
Description |
201 |
Created |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
false |
|
userId |
string |
false |
|
controllerId |
string |
true |
|
zoneNumbers |
integer[ ] |
false |
|
wateringInRain |
boolean |
false |
|
rainProbability |
integer |
false |
|
minWateringTemperature |
number |
false |
|
wateringAfterRain |
integer |
false |
|
highWind |
boolean |
false |
|
createdAt |
integer |
false |
|
updatedAt |
integer |
false |
|
municipalRestrictionId |
string |
false |
|
modified |
boolean |
false |
|
basicMunicipalRestriction |
WeatherConstraint |
false |
|
name |
string |
false |
|
areaId |
string |
false |
|
denyWateringRainAmount |
number |
false |
|
allZones |
boolean |
false |
|
forbiddenWateringBeforeRainHours |
integer |
false |
|
rainSensitivityLevel |
string |
false |
|
WeatherConstraint
Name |
Type |
Required |
Description |
id |
string |
false |
|
userId |
string |
false |
|
controllerId |
string |
true |
|
zoneNumbers |
integer[ ] |
false |
|
wateringInRain |
boolean |
false |
|
rainProbability |
integer |
false |
|
minWateringTemperature |
number |
false |
|
wateringAfterRain |
integer |
false |
|
highWind |
boolean |
false |
|
createdAt |
integer |
false |
|
updatedAt |
integer |
false |
|
municipalRestrictionId |
string |
false |
|
modified |
boolean |
false |
|
basicMunicipalRestriction |
WeatherConstraint |
false |
|
name |
string |
false |
|
areaId |
string |
false |
|
denyWateringRainAmount |
number |
false |
|
allZones |
boolean |
false |
|
forbiddenWateringBeforeRainHours |
integer |
false |
|
rainSensitivityLevel |
string |
false |
|
Get a Time Restriction by id
Request
curl "https://developer-api.etwater.com/api/v1/restrictions/weather/{id}" \
-X GET \
-H "Authorization: example string value" \
-H "Unity-Api-Gateway-Replace-UserId: example string value"
On success, the above request returns response like
{
"id": "example string value",
"userId": "example string value",
"controllerId": "example string value",
"zoneNumbers": [
365
],
"wateringInRain": false,
"rainProbability": 860,
"minWateringTemperature": 690.984267597545,
"wateringAfterRain": 772,
"highWind": false,
"createdAt": 556,
"updatedAt": 658,
"municipalRestrictionId": "example string value",
"modified": true,
"basicMunicipalRestriction": {
"id": "example string value",
"userId": "example string value",
"controllerId": "example string value",
"zoneNumbers": [
427
],
"wateringInRain": true,
"rainProbability": 391,
"minWateringTemperature": 62.204813613651694,
"wateringAfterRain": 660,
"highWind": true,
"createdAt": 713,
"updatedAt": 473,
"municipalRestrictionId": "example string value",
"modified": true,
"basicMunicipalRestriction": null,
"name": "example string value",
"areaId": "example string value",
"denyWateringRainAmount": 122,
"allZones": true,
"forbiddenWateringBeforeRainHours": 783,
"rainSensitivityLevel": "example string value"
},
"name": "example string value",
"areaId": "example string value",
"denyWateringRainAmount": 571,
"allZones": true,
"forbiddenWateringBeforeRainHours": 969,
"rainSensitivityLevel": "example string value"
}
HTTP Request
GET /api/v1/restrictions/weather/{id}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Unity-Api-Gateway-Replace-UserId |
string |
header |
false |
|
id |
string |
path |
true |
|
HTTP Responses
Code |
Description |
200 |
OK |
404 |
Not Found |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
false |
|
userId |
string |
false |
|
controllerId |
string |
true |
|
zoneNumbers |
integer[ ] |
false |
|
wateringInRain |
boolean |
false |
|
rainProbability |
integer |
false |
|
minWateringTemperature |
number |
false |
|
wateringAfterRain |
integer |
false |
|
highWind |
boolean |
false |
|
createdAt |
integer |
false |
|
updatedAt |
integer |
false |
|
municipalRestrictionId |
string |
false |
|
modified |
boolean |
false |
|
basicMunicipalRestriction |
WeatherConstraint |
false |
|
name |
string |
false |
|
areaId |
string |
false |
|
denyWateringRainAmount |
number |
false |
|
allZones |
boolean |
false |
|
forbiddenWateringBeforeRainHours |
integer |
false |
|
rainSensitivityLevel |
string |
false |
|
WeatherConstraint
Name |
Type |
Required |
Description |
id |
string |
false |
|
userId |
string |
false |
|
controllerId |
string |
true |
|
zoneNumbers |
integer[ ] |
false |
|
wateringInRain |
boolean |
false |
|
rainProbability |
integer |
false |
|
minWateringTemperature |
number |
false |
|
wateringAfterRain |
integer |
false |
|
highWind |
boolean |
false |
|
createdAt |
integer |
false |
|
updatedAt |
integer |
false |
|
municipalRestrictionId |
string |
false |
|
modified |
boolean |
false |
|
basicMunicipalRestriction |
WeatherConstraint |
false |
|
name |
string |
false |
|
areaId |
string |
false |
|
denyWateringRainAmount |
number |
false |
|
allZones |
boolean |
false |
|
forbiddenWateringBeforeRainHours |
integer |
false |
|
rainSensitivityLevel |
string |
false |
|
Delete a Time Restriction by id
Request
curl "https://developer-api.etwater.com/api/v1/restrictions/weather/{id}" \
-X DELETE \
-H "Authorization: example string value" \
-H "Unity-Api-Gateway-Replace-UserId: example string value"
On success, the above request returns response like
HTTP Request
DELETE /api/v1/restrictions/weather/{id}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Unity-Api-Gateway-Replace-UserId |
string |
header |
false |
|
id |
string |
path |
true |
|
HTTP Responses
Code |
Description |
204 |
No Content |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Update a Schedule Restriction by id
Request
curl "https://developer-api.etwater.com/api/v1/restrictions/weather/{id}" \
-X PUT \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
-H "Unity-Api-Gateway-Replace-UserId: example string value" \
--data "{
\"id\": \"example string value\",
\"userId\": \"example string value\",
\"controllerId\": \"example string value\",
\"zoneNumbers\": [
576
],
\"wateringInRain\": true,
\"rainProbability\": 888,
\"minWateringTemperature\": 280,
\"wateringAfterRain\": 277,
\"highWind\": true,
\"createdAt\": 698,
\"updatedAt\": 805,
\"municipalRestrictionId\": \"example string value\",
\"modified\": true,
\"basicMunicipalRestriction\": {
\"id\": \"example string value\",
\"userId\": \"example string value\",
\"controllerId\": \"example string value\",
\"zoneNumbers\": [
685
],
\"wateringInRain\": false,
\"rainProbability\": 555,
\"minWateringTemperature\": 532,
\"wateringAfterRain\": 869,
\"highWind\": false,
\"createdAt\": 601,
\"updatedAt\": 401,
\"municipalRestrictionId\": \"example string value\",
\"modified\": true,
\"basicMunicipalRestriction\": null,
\"name\": \"example string value\",
\"areaId\": \"example string value\",
\"denyWateringRainAmount\": 305.148008421598,
\"allZones\": false,
\"forbiddenWateringBeforeRainHours\": 407,
\"rainSensitivityLevel\": \"example string value\"
},
\"name\": \"example string value\",
\"areaId\": \"example string value\",
\"denyWateringRainAmount\": 654.2636480435094,
\"allZones\": false,
\"forbiddenWateringBeforeRainHours\": 894,
\"rainSensitivityLevel\": \"example string value\"
}"
On success, the above request returns response like
{
"id": "example string value",
"userId": "example string value",
"controllerId": "example string value",
"zoneNumbers": [
93
],
"wateringInRain": true,
"rainProbability": 349,
"minWateringTemperature": 493.4745349425238,
"wateringAfterRain": 776,
"highWind": false,
"createdAt": 424,
"updatedAt": 524,
"municipalRestrictionId": "example string value",
"modified": true,
"basicMunicipalRestriction": {
"id": "example string value",
"userId": "example string value",
"controllerId": "example string value",
"zoneNumbers": [
107
],
"wateringInRain": false,
"rainProbability": 470,
"minWateringTemperature": 386,
"wateringAfterRain": 61,
"highWind": true,
"createdAt": 745,
"updatedAt": 192,
"municipalRestrictionId": "example string value",
"modified": true,
"basicMunicipalRestriction": null,
"name": "example string value",
"areaId": "example string value",
"denyWateringRainAmount": 758.122631701698,
"allZones": true,
"forbiddenWateringBeforeRainHours": 132,
"rainSensitivityLevel": "example string value"
},
"name": "example string value",
"areaId": "example string value",
"denyWateringRainAmount": 534.5762150988803,
"allZones": true,
"forbiddenWateringBeforeRainHours": 197,
"rainSensitivityLevel": "example string value"
}
HTTP Request
PUT /api/v1/restrictions/weather/{id}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
Unity-Api-Gateway-Replace-UserId |
string |
header |
false |
|
id |
string |
path |
true |
|
WeatherConstraint |
WeatherConstraint |
body |
true |
|
WeatherConstraint
Name |
Type |
Required |
Description |
id |
string |
false |
|
userId |
string |
false |
|
controllerId |
string |
true |
|
zoneNumbers |
integer[ ] |
false |
|
wateringInRain |
boolean |
false |
|
rainProbability |
integer |
false |
|
minWateringTemperature |
number |
false |
|
wateringAfterRain |
integer |
false |
|
highWind |
boolean |
false |
|
createdAt |
integer |
false |
|
updatedAt |
integer |
false |
|
municipalRestrictionId |
string |
false |
|
modified |
boolean |
false |
|
basicMunicipalRestriction |
WeatherConstraint |
false |
|
name |
string |
false |
|
areaId |
string |
false |
|
denyWateringRainAmount |
number |
false |
|
allZones |
boolean |
false |
|
forbiddenWateringBeforeRainHours |
integer |
false |
|
rainSensitivityLevel |
string |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
false |
|
userId |
string |
false |
|
controllerId |
string |
true |
|
zoneNumbers |
integer[ ] |
false |
|
wateringInRain |
boolean |
false |
|
rainProbability |
integer |
false |
|
minWateringTemperature |
number |
false |
|
wateringAfterRain |
integer |
false |
|
highWind |
boolean |
false |
|
createdAt |
integer |
false |
|
updatedAt |
integer |
false |
|
municipalRestrictionId |
string |
false |
|
modified |
boolean |
false |
|
basicMunicipalRestriction |
WeatherConstraint |
false |
|
name |
string |
false |
|
areaId |
string |
false |
|
denyWateringRainAmount |
number |
false |
|
allZones |
boolean |
false |
|
forbiddenWateringBeforeRainHours |
integer |
false |
|
rainSensitivityLevel |
string |
false |
|
WeatherConstraint
Name |
Type |
Required |
Description |
id |
string |
false |
|
userId |
string |
false |
|
controllerId |
string |
true |
|
zoneNumbers |
integer[ ] |
false |
|
wateringInRain |
boolean |
false |
|
rainProbability |
integer |
false |
|
minWateringTemperature |
number |
false |
|
wateringAfterRain |
integer |
false |
|
highWind |
boolean |
false |
|
createdAt |
integer |
false |
|
updatedAt |
integer |
false |
|
municipalRestrictionId |
string |
false |
|
modified |
boolean |
false |
|
basicMunicipalRestriction |
WeatherConstraint |
false |
|
name |
string |
false |
|
areaId |
string |
false |
|
denyWateringRainAmount |
number |
false |
|
allZones |
boolean |
false |
|
forbiddenWateringBeforeRainHours |
integer |
false |
|
rainSensitivityLevel |
string |
false |
|
V1 | Stations | Zones
Get all Zones.
Request
curl "https://developer-api.etwater.com/api/v1/gardening/projects/{projectId}/zones?controllerId=example+string+value&flagged=0" \
-X GET \
-H "Authorization: example string value" \
-H "Unity-Api-Gateway-Replace-UserId: example string value"
On success, the above request returns response like
Dependent on:
- Authorization: Bearer {access_token}
- Content-Type: application/json
where: {access_token} access token value.
Path parameter description
{projectId} - project id value.
Response body fields description:
- id - id of zone;
- number - number of the zone;
- status - status of zone, can be 0 or 1 (ACTIVE, DELETED);
- name - name of the zone;
- created_at - time when zone was created, epoch millis in UTC;
- updated_at - time when zone was updated, epoch millis in UTC;
- weight - sorting weight number;
- project_id - project id;
- local_id - mobile internal local id;
HTTP Request
GET /api/v1/gardening/projects/{projectId}/zones
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Unity-Api-Gateway-Replace-UserId |
string |
header |
false |
|
projectId |
string |
path |
true |
Project id |
controllerId |
string |
query |
false |
|
flagged |
boolean |
query |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
404 |
Not Found |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response is array of
Create a Zone.
Request
curl "https://developer-api.etwater.com/api/v1/gardening/projects/{projectId}/zones?version=332" \
-X POST \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
--data "{
\"id\": \"example string value\",
\"number\": 334,
\"status\": 597,
\"name\": \"example string value\",
\"created_at\": 417,
\"updated_at\": 75,
\"weight\": 264,
\"project_id\": \"example string value\",
\"local_id\": \"example string value\",
\"version\": \"-1\",
\"controllerId\": \"example string value\",
\"configured\": false,
\"areaRegionStates\": {
\"example_property_name\": false
},
\"ignoreRain\": false,
\"flagged\": true,
\"description\": \"example string value\"
}"
On success, the above request returns response like
Zone has "Many to One" relation with Project, so for Zone creation project id is required.
Dependent on:
- Authorization: Bearer {access_token}
- Content-Type: application/json
where: {access_token} access token value.
Path parameter description
{projectId} - project id value.
Request body fields description:
The same as for response body.
Response body fields description:
- id - id of zone;
- number - number of the zone;
- status - status of zone, can be 0 or 1 (ACTIVE, DELETED);
- name - name of the zone;
- created_at - time when zone was created, epoch millis in UTC;
- updated_at - time when zone was updated, epoch millis in UTC;
- weight - sorting weight number;
- project_id - project id;
- local_id - mobile internal local id;
HTTP Request
POST /api/v1/gardening/projects/{projectId}/zones
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
projectId |
string |
path |
true |
Project id |
Zone |
GardeningZone |
body |
true |
|
version |
integer |
query |
false |
version of Project |
GardeningZone
Name |
Type |
Required |
Description |
id |
string |
false |
|
number |
integer |
false |
|
status |
integer |
false |
|
name |
string |
false |
|
created_at |
integer |
false |
|
updated_at |
integer |
false |
|
weight |
integer |
false |
|
project_id |
string |
false |
|
local_id |
string |
false |
|
version |
integer |
false |
|
controllerId |
string |
false |
|
configured |
boolean |
false |
|
areaRegionStates |
Object for field areaRegionStates |
false |
|
ignoreRain |
boolean |
false |
|
flagged |
boolean |
false |
|
description |
string |
false |
|
Object for field areaRegionStates
Name |
Type |
Required |
Description |
* |
boolean |
false |
|
HTTP Responses
Code |
Description |
201 |
Created |
404 |
Not Found |
409 |
Conflict |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
false |
|
number |
integer |
false |
|
status |
integer |
false |
|
name |
string |
false |
|
created_at |
integer |
false |
|
updated_at |
integer |
false |
|
weight |
integer |
false |
|
project_id |
string |
false |
|
local_id |
string |
false |
|
version |
integer |
false |
|
controllerId |
string |
false |
|
configured |
boolean |
false |
|
areaRegionStates |
Object for field areaRegionStates |
false |
|
ignoreRain |
boolean |
false |
|
flagged |
boolean |
false |
|
description |
string |
false |
|
Object for field areaRegionStates
Name |
Type |
Required |
Description |
* |
boolean |
false |
|
Create or update zones for project
Request
curl "https://developer-api.etwater.com/api/v1/gardening/projects/{projectId}/zones?version=804" \
-X PATCH \
-H "Authorization: example string value" \
--data "[
{
\"id\": \"example string value\",
\"number\": 329,
\"status\": 834,
\"name\": \"example string value\",
\"created_at\": 503,
\"updated_at\": 722,
\"weight\": 871,
\"project_id\": \"example string value\",
\"local_id\": \"example string value\",
\"version\": \"-1\",
\"controllerId\": \"example string value\",
\"configured\": false,
\"areaRegionStates\": {
\"example_property_name\": true
},
\"ignoreRain\": false,
\"flagged\": true,
\"description\": \"example string value\"
}
]"
On success, the above request returns response like
[
{
"id": "example string value",
"number": 219,
"status": 805,
"name": "example string value",
"created_at": 861,
"updated_at": 983,
"weight": 620,
"project_id": "example string value",
"local_id": "example string value",
"version": "-1",
"controllerId": "example string value",
"configured": true,
"areaRegionStates": {
"example_property_name": false
},
"ignoreRain": true,
"flagged": true,
"description": "example string value"
}
]
HTTP Request
PATCH /api/v1/gardening/projects/{projectId}/zones
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
projectId |
string |
path |
true |
Project id |
Zones |
GardeningZone[ ] |
body |
true |
|
version |
integer |
query |
false |
version of Project |
GardeningZone
Name |
Type |
Required |
Description |
id |
string |
false |
|
number |
integer |
false |
|
status |
integer |
false |
|
name |
string |
false |
|
created_at |
integer |
false |
|
updated_at |
integer |
false |
|
weight |
integer |
false |
|
project_id |
string |
false |
|
local_id |
string |
false |
|
version |
integer |
false |
|
controllerId |
string |
false |
|
configured |
boolean |
false |
|
areaRegionStates |
Object for field areaRegionStates |
false |
|
ignoreRain |
boolean |
false |
|
flagged |
boolean |
false |
|
description |
string |
false |
|
Object for field areaRegionStates
Name |
Type |
Required |
Description |
* |
boolean |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
404 |
Not Found |
409 |
Conflict |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response is array of
Name |
Type |
Required |
Description |
id |
string |
false |
|
number |
integer |
false |
|
status |
integer |
false |
|
name |
string |
false |
|
created_at |
integer |
false |
|
updated_at |
integer |
false |
|
weight |
integer |
false |
|
project_id |
string |
false |
|
local_id |
string |
false |
|
version |
integer |
false |
|
controllerId |
string |
false |
|
configured |
boolean |
false |
|
areaRegionStates |
Object for field areaRegionStates |
false |
|
ignoreRain |
boolean |
false |
|
flagged |
boolean |
false |
|
description |
string |
false |
|
Object for field areaRegionStates
Name |
Type |
Required |
Description |
* |
boolean |
false |
|
Get Zone by id.
Request
curl "https://developer-api.etwater.com/api/v1/gardening/projects/{projectId}/zones/{zoneId}" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"id": "example string value",
"number": 345,
"status": 851,
"name": "example string value",
"created_at": 768,
"updated_at": 250,
"weight": 191,
"project_id": "example string value",
"local_id": "example string value",
"version": "-1",
"controllerId": "example string value",
"configured": true,
"areaRegionStates": {
"example_property_name": false
},
"ignoreRain": true,
"flagged": true,
"description": "example string value"
}
Dependent on:
- Authorization: Bearer {access_token}
- Content-Type: application/json
where: {access_token} access token value.
Path parameter description
- {projectId} - project id value.
- {zoneId} - zone id value.
Response body fields description:
- id - id of zone;
- number - number of the zone;
- status - status of zone, can be 0 or 1 (ACTIVE, DELETED);
- name - name of the zone;
- created_at - time when zone was created, epoch millis in UTC;
- updated_at - time when zone was updated, epoch millis in UTC;
- weight - sorting weight number;
- project_id - project id;
- local_id - mobile internal local id;
HTTP Request
GET /api/v1/gardening/projects/{projectId}/zones/{zoneId}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
projectId |
string |
path |
true |
Project id |
zoneId |
string |
path |
true |
Zone id |
HTTP Responses
Code |
Description |
200 |
OK |
404 |
Not Found |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
false |
|
number |
integer |
false |
|
status |
integer |
false |
|
name |
string |
false |
|
created_at |
integer |
false |
|
updated_at |
integer |
false |
|
weight |
integer |
false |
|
project_id |
string |
false |
|
local_id |
string |
false |
|
version |
integer |
false |
|
controllerId |
string |
false |
|
configured |
boolean |
false |
|
areaRegionStates |
Object for field areaRegionStates |
false |
|
ignoreRain |
boolean |
false |
|
flagged |
boolean |
false |
|
description |
string |
false |
|
Object for field areaRegionStates
Name |
Type |
Required |
Description |
* |
boolean |
false |
|
Delete Zone by id.
Request
curl "https://developer-api.etwater.com/api/v1/gardening/projects/{projectId}/zones/{zoneId}?version=714" \
-X DELETE \
-H "Authorization: example string value"
On success, the above request returns response like
Dependent on:
- Authorization: Bearer {access_token}
- Content-Type: application/json
where: {access_token} access token value.
Path parameter description
- {projectId} - project id value.
- {zoneId} - zone id value.
HTTP Request
DELETE /api/v1/gardening/projects/{projectId}/zones/{zoneId}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
projectId |
string |
path |
true |
Project id |
zoneId |
string |
path |
true |
id of Zone |
version |
integer |
query |
false |
version of Project |
HTTP Responses
Code |
Description |
204 |
No Content |
404 |
Not Found |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Update/Merge Zone by id.
Request
curl "https://developer-api.etwater.com/api/v1/gardening/projects/{projectId}/zones/{zoneId}?version=799" \
-X PUT \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
--data "{
\"id\": \"example string value\",
\"number\": 485,
\"status\": 21,
\"name\": \"example string value\",
\"created_at\": 531,
\"updated_at\": 978,
\"weight\": 887,
\"project_id\": \"example string value\",
\"local_id\": \"example string value\",
\"version\": \"-1\",
\"controllerId\": \"example string value\",
\"configured\": true,
\"areaRegionStates\": {
\"example_property_name\": false
},
\"ignoreRain\": true,
\"flagged\": true,
\"description\": \"example string value\"
}"
On success, the above request returns response like
{
"id": "example string value",
"number": 212,
"status": 602,
"name": "example string value",
"created_at": 661,
"updated_at": 754,
"weight": 4,
"project_id": "example string value",
"local_id": "example string value",
"version": "-1",
"controllerId": "example string value",
"configured": false,
"areaRegionStates": {
"example_property_name": true
},
"ignoreRain": false,
"flagged": false,
"description": "example string value"
}
Dependent on:
- Authorization: Bearer {access_token}
- Content-Type: application/json
where: {access_token} access token value.
Path parameter description
- {projectId} - project id value.
- {zoneId} - zone id value.
Request body fields description:
The same as for response body.
Response body fields description:
- id - id of zone;
- number - number of the zone;
- status - status of zone, can be 0 or 1 (ACTIVE, DELETED);
- name - name of the zone;
- created_at - time when zone was created, epoch millis in UTC;
- updated_at - time when zone was updated, epoch millis in UTC;
- weight - sorting weight number;
- project_id - project id;
- local_id - mobile internal local id;
HTTP Request
PUT /api/v1/gardening/projects/{projectId}/zones/{zoneId}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
projectId |
string |
path |
true |
Project id |
zoneId |
string |
path |
true |
id of Zone |
Zone |
GardeningZone |
body |
true |
|
version |
integer |
query |
false |
version of Project |
GardeningZone
Name |
Type |
Required |
Description |
id |
string |
false |
|
number |
integer |
false |
|
status |
integer |
false |
|
name |
string |
false |
|
created_at |
integer |
false |
|
updated_at |
integer |
false |
|
weight |
integer |
false |
|
project_id |
string |
false |
|
local_id |
string |
false |
|
version |
integer |
false |
|
controllerId |
string |
false |
|
configured |
boolean |
false |
|
areaRegionStates |
Object for field areaRegionStates |
false |
|
ignoreRain |
boolean |
false |
|
flagged |
boolean |
false |
|
description |
string |
false |
|
Object for field areaRegionStates
Name |
Type |
Required |
Description |
* |
boolean |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
404 |
Not Found |
409 |
Conflict |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
false |
|
number |
integer |
false |
|
status |
integer |
false |
|
name |
string |
false |
|
created_at |
integer |
false |
|
updated_at |
integer |
false |
|
weight |
integer |
false |
|
project_id |
string |
false |
|
local_id |
string |
false |
|
version |
integer |
false |
|
controllerId |
string |
false |
|
configured |
boolean |
false |
|
areaRegionStates |
Object for field areaRegionStates |
false |
|
ignoreRain |
boolean |
false |
|
flagged |
boolean |
false |
|
description |
string |
false |
|
Object for field areaRegionStates
Name |
Type |
Required |
Description |
* |
boolean |
false |
|
V2 | Atmosphere API extended
Get daily atmosphere data
Request
curl "https://developer-api.etwater.com/api/v2/atmosphere/extended/daily?longitude=705.48386113042&latitude=693&startTimestamp=585&endTimestamp=285&keys%5B0%5D=ET" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"cluster": {
"id": "example string value",
"location": {
"type": "example string value",
"coordinates": [
501.092786202716
]
}
},
"hourly": [
{
"time": 383,
"etMm": 479,
"rainMm": 301,
"ghWm2": 278,
"temperatureC": 920,
"dewPointC": 249.0707734828213,
"windKmH": 440,
"relativeHumidityPercent": 884.0964822490217,
"precipitationProbabilityPercent": 554.1624462018547,
"cloudCoverPercent": 410,
"pressureKilopascals": 905,
"windGustKmH": 878.0323620317655,
"timeEpoch": 555
}
],
"daily": [
{
"time": 766,
"sunrise": 952,
"sunset": 535,
"etMm": 424.73941688646534,
"rainMm": 936,
"ghWm2": 373,
"dewPointC": 508.24514660436904,
"minTemperatureC": 474.87263869255435,
"maxTemperatureC": 504.5923434684949,
"minWindKmH": 267.8684439826144,
"maxWindKmH": 614,
"avgPressureKilopascals": 861.4522213402447,
"avgCloudCoverPercent": 939.5929029861431,
"avgRelativeHumidityPercent": 361.3111802196648,
"maxPrecipitationProbabilityPercent": 531,
"avgTemperatureC": 829.1307109543731,
"minRelativeHumidityPercent": 273,
"maxRelativeHumidityPercent": 322.9694544910311
}
],
"monthly": [
{
"time": 257,
"etMm": 908.7764196604381,
"rainMm": 747.5314236933046,
"ghWm2": 182,
"minTemperatureC": 398,
"maxTemperatureC": 620.5151521696314,
"minWindKmH": 546,
"maxWindKmH": 783.9214172139398,
"avgPressureKilopascals": 363.5458184236408,
"avgCloudCoverPercent": 890.927198292188,
"avgRelativeHumidityPercent": 343.30146868866933,
"maxPrecipitationProbabilityPercent": 195.24835571425425,
"avgWindKmH": 706,
"avgTemperatureC": 147.42056846032878,
"avgDewPointC": 279,
"minRelativeHumidityPercent": 435,
"maxRelativeHumidityPercent": 542
}
],
"status": 760
}
HTTP Request
GET /api/v2/atmosphere/extended/daily
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
longitude |
number |
query |
false |
|
latitude |
number |
query |
false |
|
startTimestamp |
integer |
query |
false |
|
endTimestamp |
integer |
query |
false |
|
keys |
array |
query |
false |
names of sections to show |
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
cluster |
WeatherCluster |
true |
|
hourly |
WeatherHourly[ ] |
false |
|
daily |
WeatherDaily[ ] |
false |
|
monthly |
WeatherMonthly[ ] |
false |
|
status |
integer |
true |
|
WeatherCluster
Name |
Type |
Required |
Description |
id |
string |
false |
|
location |
Location |
false |
|
Location
Name |
Type |
Required |
Description |
type |
string |
false |
|
coordinates |
number[ ] |
false |
|
WeatherHourly
Name |
Type |
Required |
Description |
time |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
temperatureC |
number |
false |
|
dewPointC |
number |
false |
|
windKmH |
number |
false |
|
relativeHumidityPercent |
number |
false |
|
precipitationProbabilityPercent |
number |
false |
|
cloudCoverPercent |
number |
false |
|
pressureKilopascals |
number |
false |
|
windGustKmH |
number |
false |
|
timeEpoch |
integer |
false |
|
WeatherDaily
Name |
Type |
Required |
Description |
time |
integer |
false |
|
sunrise |
integer |
false |
|
sunset |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
dewPointC |
number |
false |
|
minTemperatureC |
number |
false |
|
maxTemperatureC |
number |
false |
|
minWindKmH |
number |
false |
|
maxWindKmH |
number |
false |
|
avgPressureKilopascals |
number |
false |
|
avgCloudCoverPercent |
number |
false |
|
avgRelativeHumidityPercent |
number |
false |
|
maxPrecipitationProbabilityPercent |
number |
false |
|
avgTemperatureC |
number |
false |
|
minRelativeHumidityPercent |
number |
false |
|
maxRelativeHumidityPercent |
number |
false |
|
WeatherMonthly
Name |
Type |
Required |
Description |
time |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
minTemperatureC |
number |
false |
|
maxTemperatureC |
number |
false |
|
minWindKmH |
number |
false |
|
maxWindKmH |
number |
false |
|
avgPressureKilopascals |
number |
false |
|
avgCloudCoverPercent |
number |
false |
|
avgRelativeHumidityPercent |
number |
false |
|
maxPrecipitationProbabilityPercent |
number |
false |
|
avgWindKmH |
number |
false |
|
avgTemperatureC |
number |
false |
|
avgDewPointC |
number |
false |
|
minRelativeHumidityPercent |
number |
false |
|
maxRelativeHumidityPercent |
number |
false |
|
Get hourly atmosphere data
Request
curl "https://developer-api.etwater.com/api/v2/atmosphere/extended/hourly?longitude=462&latitude=490&startTimestamp=283&endTimestamp=593&keys%5B0%5D=SUNSET" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"cluster": {
"id": "example string value",
"location": {
"type": "example string value",
"coordinates": [
645
]
}
},
"hourly": [
{
"time": 470,
"etMm": 32,
"rainMm": 664.1462257430638,
"ghWm2": 548,
"temperatureC": 327,
"dewPointC": 786.5076897603029,
"windKmH": 474,
"relativeHumidityPercent": 576.3758973108493,
"precipitationProbabilityPercent": 394,
"cloudCoverPercent": 52.15591613769341,
"pressureKilopascals": 614.502402308631,
"windGustKmH": 98.65340222541867,
"timeEpoch": 375
}
],
"daily": [
{
"time": 416,
"sunrise": 774,
"sunset": 677,
"etMm": 357.87296637793673,
"rainMm": 329,
"ghWm2": 559,
"dewPointC": 708.5119517094046,
"minTemperatureC": 385,
"maxTemperatureC": 93,
"minWindKmH": 964,
"maxWindKmH": 400.61385761975026,
"avgPressureKilopascals": 226,
"avgCloudCoverPercent": 631,
"avgRelativeHumidityPercent": 288.58396796909346,
"maxPrecipitationProbabilityPercent": 543,
"avgTemperatureC": 540,
"minRelativeHumidityPercent": 531.9211094323178,
"maxRelativeHumidityPercent": 982.4184998788025
}
],
"monthly": [
{
"time": 230,
"etMm": 997.9923311611601,
"rainMm": 98.23376550256916,
"ghWm2": 528,
"minTemperatureC": 389,
"maxTemperatureC": 583.7726344278886,
"minWindKmH": 673,
"maxWindKmH": 303.01956334291935,
"avgPressureKilopascals": 44.27439674934111,
"avgCloudCoverPercent": 816,
"avgRelativeHumidityPercent": 648,
"maxPrecipitationProbabilityPercent": 981.108965809042,
"avgWindKmH": 256.0284413658215,
"avgTemperatureC": 65,
"avgDewPointC": 682.2262172038788,
"minRelativeHumidityPercent": 335,
"maxRelativeHumidityPercent": 37
}
],
"status": 167
}
HTTP Request
GET /api/v2/atmosphere/extended/hourly
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
longitude |
number |
query |
false |
|
latitude |
number |
query |
false |
|
startTimestamp |
integer |
query |
false |
|
endTimestamp |
integer |
query |
false |
|
keys |
array |
query |
false |
names of sections to show |
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
cluster |
WeatherCluster |
true |
|
hourly |
WeatherHourly[ ] |
false |
|
daily |
WeatherDaily[ ] |
false |
|
monthly |
WeatherMonthly[ ] |
false |
|
status |
integer |
true |
|
WeatherCluster
Name |
Type |
Required |
Description |
id |
string |
false |
|
location |
Location |
false |
|
Location
Name |
Type |
Required |
Description |
type |
string |
false |
|
coordinates |
number[ ] |
false |
|
WeatherHourly
Name |
Type |
Required |
Description |
time |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
temperatureC |
number |
false |
|
dewPointC |
number |
false |
|
windKmH |
number |
false |
|
relativeHumidityPercent |
number |
false |
|
precipitationProbabilityPercent |
number |
false |
|
cloudCoverPercent |
number |
false |
|
pressureKilopascals |
number |
false |
|
windGustKmH |
number |
false |
|
timeEpoch |
integer |
false |
|
WeatherDaily
Name |
Type |
Required |
Description |
time |
integer |
false |
|
sunrise |
integer |
false |
|
sunset |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
dewPointC |
number |
false |
|
minTemperatureC |
number |
false |
|
maxTemperatureC |
number |
false |
|
minWindKmH |
number |
false |
|
maxWindKmH |
number |
false |
|
avgPressureKilopascals |
number |
false |
|
avgCloudCoverPercent |
number |
false |
|
avgRelativeHumidityPercent |
number |
false |
|
maxPrecipitationProbabilityPercent |
number |
false |
|
avgTemperatureC |
number |
false |
|
minRelativeHumidityPercent |
number |
false |
|
maxRelativeHumidityPercent |
number |
false |
|
WeatherMonthly
Name |
Type |
Required |
Description |
time |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
minTemperatureC |
number |
false |
|
maxTemperatureC |
number |
false |
|
minWindKmH |
number |
false |
|
maxWindKmH |
number |
false |
|
avgPressureKilopascals |
number |
false |
|
avgCloudCoverPercent |
number |
false |
|
avgRelativeHumidityPercent |
number |
false |
|
maxPrecipitationProbabilityPercent |
number |
false |
|
avgWindKmH |
number |
false |
|
avgTemperatureC |
number |
false |
|
avgDewPointC |
number |
false |
|
minRelativeHumidityPercent |
number |
false |
|
maxRelativeHumidityPercent |
number |
false |
|
Get monthly atmosphere data
Request
curl "https://developer-api.etwater.com/api/v2/atmosphere/extended/monthly?longitude=395.25196021202&latitude=629&startTimestamp=871&endTimestamp=826&keys%5B0%5D=TEMPERATURE" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"cluster": {
"id": "example string value",
"location": {
"type": "example string value",
"coordinates": [
964
]
}
},
"hourly": [
{
"time": 590,
"etMm": 215,
"rainMm": 9.415668905440564,
"ghWm2": 236,
"temperatureC": 867,
"dewPointC": 413.9287813631486,
"windKmH": 253,
"relativeHumidityPercent": 364.69952825675693,
"precipitationProbabilityPercent": 839.1067072931243,
"cloudCoverPercent": 448,
"pressureKilopascals": 457.7387424454739,
"windGustKmH": 759,
"timeEpoch": 4
}
],
"daily": [
{
"time": 652,
"sunrise": 971,
"sunset": 936,
"etMm": 543.4727843587625,
"rainMm": 738.1225385415007,
"ghWm2": 113,
"dewPointC": 378.98002210025675,
"minTemperatureC": 226,
"maxTemperatureC": 997.8556861159651,
"minWindKmH": 977.0302423169046,
"maxWindKmH": 948,
"avgPressureKilopascals": 787,
"avgCloudCoverPercent": 929,
"avgRelativeHumidityPercent": 133.09104886515578,
"maxPrecipitationProbabilityPercent": 371.32618966108475,
"avgTemperatureC": 662,
"minRelativeHumidityPercent": 149,
"maxRelativeHumidityPercent": 218
}
],
"monthly": [
{
"time": 868,
"etMm": 422,
"rainMm": 47.482789981869416,
"ghWm2": 974,
"minTemperatureC": 175,
"maxTemperatureC": 903,
"minWindKmH": 201.81649327362726,
"maxWindKmH": 316,
"avgPressureKilopascals": 756.6691244750605,
"avgCloudCoverPercent": 844,
"avgRelativeHumidityPercent": 791,
"maxPrecipitationProbabilityPercent": 695,
"avgWindKmH": 1,
"avgTemperatureC": 732,
"avgDewPointC": 573,
"minRelativeHumidityPercent": 817,
"maxRelativeHumidityPercent": 571
}
],
"status": 204
}
HTTP Request
GET /api/v2/atmosphere/extended/monthly
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
longitude |
number |
query |
false |
|
latitude |
number |
query |
false |
|
startTimestamp |
integer |
query |
false |
|
endTimestamp |
integer |
query |
false |
|
keys |
array |
query |
false |
names of sections to show |
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
cluster |
WeatherCluster |
true |
|
hourly |
WeatherHourly[ ] |
false |
|
daily |
WeatherDaily[ ] |
false |
|
monthly |
WeatherMonthly[ ] |
false |
|
status |
integer |
true |
|
WeatherCluster
Name |
Type |
Required |
Description |
id |
string |
false |
|
location |
Location |
false |
|
Location
Name |
Type |
Required |
Description |
type |
string |
false |
|
coordinates |
number[ ] |
false |
|
WeatherHourly
Name |
Type |
Required |
Description |
time |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
temperatureC |
number |
false |
|
dewPointC |
number |
false |
|
windKmH |
number |
false |
|
relativeHumidityPercent |
number |
false |
|
precipitationProbabilityPercent |
number |
false |
|
cloudCoverPercent |
number |
false |
|
pressureKilopascals |
number |
false |
|
windGustKmH |
number |
false |
|
timeEpoch |
integer |
false |
|
WeatherDaily
Name |
Type |
Required |
Description |
time |
integer |
false |
|
sunrise |
integer |
false |
|
sunset |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
dewPointC |
number |
false |
|
minTemperatureC |
number |
false |
|
maxTemperatureC |
number |
false |
|
minWindKmH |
number |
false |
|
maxWindKmH |
number |
false |
|
avgPressureKilopascals |
number |
false |
|
avgCloudCoverPercent |
number |
false |
|
avgRelativeHumidityPercent |
number |
false |
|
maxPrecipitationProbabilityPercent |
number |
false |
|
avgTemperatureC |
number |
false |
|
minRelativeHumidityPercent |
number |
false |
|
maxRelativeHumidityPercent |
number |
false |
|
WeatherMonthly
Name |
Type |
Required |
Description |
time |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
minTemperatureC |
number |
false |
|
maxTemperatureC |
number |
false |
|
minWindKmH |
number |
false |
|
maxWindKmH |
number |
false |
|
avgPressureKilopascals |
number |
false |
|
avgCloudCoverPercent |
number |
false |
|
avgRelativeHumidityPercent |
number |
false |
|
maxPrecipitationProbabilityPercent |
number |
false |
|
avgWindKmH |
number |
false |
|
avgTemperatureC |
number |
false |
|
avgDewPointC |
number |
false |
|
minRelativeHumidityPercent |
number |
false |
|
maxRelativeHumidityPercent |
number |
false |
|
Get daily atmosphere data
Request
curl "https://developer-api.etwater.com/api/v2/atmosphere/extended/cluster/{clusterId}/daily?startTimestamp=512&endTimestamp=863&keys%5B0%5D=DEWPOINT" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"cluster": {
"id": "example string value",
"location": {
"type": "example string value",
"coordinates": [
543
]
}
},
"hourly": [
{
"time": 932,
"etMm": 287,
"rainMm": 202,
"ghWm2": 93,
"temperatureC": 999.735358636703,
"dewPointC": 713.3306696607409,
"windKmH": 952,
"relativeHumidityPercent": 529.4972376569626,
"precipitationProbabilityPercent": 159.56928728128284,
"cloudCoverPercent": 635.3521308094971,
"pressureKilopascals": 929.4404480277749,
"windGustKmH": 288.97452041924674,
"timeEpoch": 726
}
],
"daily": [
{
"time": 216,
"sunrise": 889,
"sunset": 943,
"etMm": 863,
"rainMm": 254,
"ghWm2": 277,
"dewPointC": 355,
"minTemperatureC": 522,
"maxTemperatureC": 942.7332775400641,
"minWindKmH": 32,
"maxWindKmH": 279.21851178594795,
"avgPressureKilopascals": 909.8937967372564,
"avgCloudCoverPercent": 253,
"avgRelativeHumidityPercent": 21.33362461874896,
"maxPrecipitationProbabilityPercent": 396.4785562811785,
"avgTemperatureC": 12.200213508773695,
"minRelativeHumidityPercent": 846,
"maxRelativeHumidityPercent": 968.7068848724974
}
],
"monthly": [
{
"time": 468,
"etMm": 69,
"rainMm": 427.1344721443646,
"ghWm2": 849,
"minTemperatureC": 776,
"maxTemperatureC": 597,
"minWindKmH": 252,
"maxWindKmH": 769.0279682953972,
"avgPressureKilopascals": 805,
"avgCloudCoverPercent": 444,
"avgRelativeHumidityPercent": 179.08191642681226,
"maxPrecipitationProbabilityPercent": 673.2287410056352,
"avgWindKmH": 903.5700805967534,
"avgTemperatureC": 369.4547337337652,
"avgDewPointC": 466.8345136879173,
"minRelativeHumidityPercent": 910.8824086891872,
"maxRelativeHumidityPercent": 705
}
],
"status": 14
}
HTTP Request
GET /api/v2/atmosphere/extended/cluster/{clusterId}/daily
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
startTimestamp |
integer |
query |
false |
|
endTimestamp |
integer |
query |
false |
|
keys |
array |
query |
false |
names of sections to show |
clusterId |
string |
path |
true |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
cluster |
WeatherCluster |
true |
|
hourly |
WeatherHourly[ ] |
false |
|
daily |
WeatherDaily[ ] |
false |
|
monthly |
WeatherMonthly[ ] |
false |
|
status |
integer |
true |
|
WeatherCluster
Name |
Type |
Required |
Description |
id |
string |
false |
|
location |
Location |
false |
|
Location
Name |
Type |
Required |
Description |
type |
string |
false |
|
coordinates |
number[ ] |
false |
|
WeatherHourly
Name |
Type |
Required |
Description |
time |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
temperatureC |
number |
false |
|
dewPointC |
number |
false |
|
windKmH |
number |
false |
|
relativeHumidityPercent |
number |
false |
|
precipitationProbabilityPercent |
number |
false |
|
cloudCoverPercent |
number |
false |
|
pressureKilopascals |
number |
false |
|
windGustKmH |
number |
false |
|
timeEpoch |
integer |
false |
|
WeatherDaily
Name |
Type |
Required |
Description |
time |
integer |
false |
|
sunrise |
integer |
false |
|
sunset |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
dewPointC |
number |
false |
|
minTemperatureC |
number |
false |
|
maxTemperatureC |
number |
false |
|
minWindKmH |
number |
false |
|
maxWindKmH |
number |
false |
|
avgPressureKilopascals |
number |
false |
|
avgCloudCoverPercent |
number |
false |
|
avgRelativeHumidityPercent |
number |
false |
|
maxPrecipitationProbabilityPercent |
number |
false |
|
avgTemperatureC |
number |
false |
|
minRelativeHumidityPercent |
number |
false |
|
maxRelativeHumidityPercent |
number |
false |
|
WeatherMonthly
Name |
Type |
Required |
Description |
time |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
minTemperatureC |
number |
false |
|
maxTemperatureC |
number |
false |
|
minWindKmH |
number |
false |
|
maxWindKmH |
number |
false |
|
avgPressureKilopascals |
number |
false |
|
avgCloudCoverPercent |
number |
false |
|
avgRelativeHumidityPercent |
number |
false |
|
maxPrecipitationProbabilityPercent |
number |
false |
|
avgWindKmH |
number |
false |
|
avgTemperatureC |
number |
false |
|
avgDewPointC |
number |
false |
|
minRelativeHumidityPercent |
number |
false |
|
maxRelativeHumidityPercent |
number |
false |
|
Get hourly atmosphere data
Request
curl "https://developer-api.etwater.com/api/v2/atmosphere/extended/cluster/{clusterId}/hourly?startTimestamp=736&endTimestamp=604&keys%5B0%5D=SUNRISE" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"cluster": {
"id": "example string value",
"location": {
"type": "example string value",
"coordinates": [
650
]
}
},
"hourly": [
{
"time": 554,
"etMm": 954.1702279607626,
"rainMm": 466,
"ghWm2": 780,
"temperatureC": 193.53317990597952,
"dewPointC": 784.5770953151291,
"windKmH": 419.43687080379425,
"relativeHumidityPercent": 768,
"precipitationProbabilityPercent": 57.86018867877321,
"cloudCoverPercent": 426.8757158084194,
"pressureKilopascals": 428,
"windGustKmH": 191,
"timeEpoch": 427
}
],
"daily": [
{
"time": 169,
"sunrise": 978,
"sunset": 179,
"etMm": 940,
"rainMm": 553.1997273458167,
"ghWm2": 447,
"dewPointC": 333.8792600361068,
"minTemperatureC": 145.01598577248677,
"maxTemperatureC": 374,
"minWindKmH": 978.2225415009179,
"maxWindKmH": 641,
"avgPressureKilopascals": 570,
"avgCloudCoverPercent": 281,
"avgRelativeHumidityPercent": 985,
"maxPrecipitationProbabilityPercent": 537,
"avgTemperatureC": 478,
"minRelativeHumidityPercent": 336,
"maxRelativeHumidityPercent": 129.92646970317068
}
],
"monthly": [
{
"time": 576,
"etMm": 250,
"rainMm": 474,
"ghWm2": 325,
"minTemperatureC": 387,
"maxTemperatureC": 126,
"minWindKmH": 335,
"maxWindKmH": 733,
"avgPressureKilopascals": 583.5947667172154,
"avgCloudCoverPercent": 116.80504079759356,
"avgRelativeHumidityPercent": 723.5308386029353,
"maxPrecipitationProbabilityPercent": 730.7708853533355,
"avgWindKmH": 814.5400783114787,
"avgTemperatureC": 698.3463343690831,
"avgDewPointC": 595,
"minRelativeHumidityPercent": 596,
"maxRelativeHumidityPercent": 951.5131944564698
}
],
"status": 979
}
HTTP Request
GET /api/v2/atmosphere/extended/cluster/{clusterId}/hourly
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
startTimestamp |
integer |
query |
false |
|
endTimestamp |
integer |
query |
false |
|
keys |
array |
query |
false |
names of sections to show |
clusterId |
string |
path |
true |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
cluster |
WeatherCluster |
true |
|
hourly |
WeatherHourly[ ] |
false |
|
daily |
WeatherDaily[ ] |
false |
|
monthly |
WeatherMonthly[ ] |
false |
|
status |
integer |
true |
|
WeatherCluster
Name |
Type |
Required |
Description |
id |
string |
false |
|
location |
Location |
false |
|
Location
Name |
Type |
Required |
Description |
type |
string |
false |
|
coordinates |
number[ ] |
false |
|
WeatherHourly
Name |
Type |
Required |
Description |
time |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
temperatureC |
number |
false |
|
dewPointC |
number |
false |
|
windKmH |
number |
false |
|
relativeHumidityPercent |
number |
false |
|
precipitationProbabilityPercent |
number |
false |
|
cloudCoverPercent |
number |
false |
|
pressureKilopascals |
number |
false |
|
windGustKmH |
number |
false |
|
timeEpoch |
integer |
false |
|
WeatherDaily
Name |
Type |
Required |
Description |
time |
integer |
false |
|
sunrise |
integer |
false |
|
sunset |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
dewPointC |
number |
false |
|
minTemperatureC |
number |
false |
|
maxTemperatureC |
number |
false |
|
minWindKmH |
number |
false |
|
maxWindKmH |
number |
false |
|
avgPressureKilopascals |
number |
false |
|
avgCloudCoverPercent |
number |
false |
|
avgRelativeHumidityPercent |
number |
false |
|
maxPrecipitationProbabilityPercent |
number |
false |
|
avgTemperatureC |
number |
false |
|
minRelativeHumidityPercent |
number |
false |
|
maxRelativeHumidityPercent |
number |
false |
|
WeatherMonthly
Name |
Type |
Required |
Description |
time |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
minTemperatureC |
number |
false |
|
maxTemperatureC |
number |
false |
|
minWindKmH |
number |
false |
|
maxWindKmH |
number |
false |
|
avgPressureKilopascals |
number |
false |
|
avgCloudCoverPercent |
number |
false |
|
avgRelativeHumidityPercent |
number |
false |
|
maxPrecipitationProbabilityPercent |
number |
false |
|
avgWindKmH |
number |
false |
|
avgTemperatureC |
number |
false |
|
avgDewPointC |
number |
false |
|
minRelativeHumidityPercent |
number |
false |
|
maxRelativeHumidityPercent |
number |
false |
|
Get monthly atmosphere data
Request
curl "https://developer-api.etwater.com/api/v2/atmosphere/extended/cluster/{clusterId}/monthly?startTimestamp=235&endTimestamp=732&keys%5B0%5D=RAIN" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"cluster": {
"id": "example string value",
"location": {
"type": "example string value",
"coordinates": [
298
]
}
},
"hourly": [
{
"time": 942,
"etMm": 725.2131652670042,
"rainMm": 834.4869063396412,
"ghWm2": 291,
"temperatureC": 767.5609960069698,
"dewPointC": 105.07738269170625,
"windKmH": 360.53884418706355,
"relativeHumidityPercent": 690.9924506633507,
"precipitationProbabilityPercent": 286,
"cloudCoverPercent": 656.6424256454419,
"pressureKilopascals": 624,
"windGustKmH": 592,
"timeEpoch": 497
}
],
"daily": [
{
"time": 588,
"sunrise": 61,
"sunset": 12,
"etMm": 756.2213799712347,
"rainMm": 719,
"ghWm2": 156,
"dewPointC": 193,
"minTemperatureC": 266.72148297853835,
"maxTemperatureC": 936.9582049255065,
"minWindKmH": 858.2842926766184,
"maxWindKmH": 293,
"avgPressureKilopascals": 413.8344146375705,
"avgCloudCoverPercent": 515,
"avgRelativeHumidityPercent": 301,
"maxPrecipitationProbabilityPercent": 658.8559321401901,
"avgTemperatureC": 296,
"minRelativeHumidityPercent": 248,
"maxRelativeHumidityPercent": 557.7144085232701
}
],
"monthly": [
{
"time": 211,
"etMm": 751.6657960376077,
"rainMm": 15.056161216951983,
"ghWm2": 794,
"minTemperatureC": 481.1079481062982,
"maxTemperatureC": 594.3433104987924,
"minWindKmH": 602.2633307623971,
"maxWindKmH": 374.4410599462879,
"avgPressureKilopascals": 894.5791781389057,
"avgCloudCoverPercent": 569,
"avgRelativeHumidityPercent": 38,
"maxPrecipitationProbabilityPercent": 884,
"avgWindKmH": 151.43226559806254,
"avgTemperatureC": 84,
"avgDewPointC": 662.7916198516226,
"minRelativeHumidityPercent": 539.9570355843553,
"maxRelativeHumidityPercent": 477.74022886424336
}
],
"status": 539
}
HTTP Request
GET /api/v2/atmosphere/extended/cluster/{clusterId}/monthly
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
startTimestamp |
integer |
query |
false |
|
endTimestamp |
integer |
query |
false |
|
keys |
array |
query |
false |
names of sections to show |
clusterId |
string |
path |
true |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
cluster |
WeatherCluster |
true |
|
hourly |
WeatherHourly[ ] |
false |
|
daily |
WeatherDaily[ ] |
false |
|
monthly |
WeatherMonthly[ ] |
false |
|
status |
integer |
true |
|
WeatherCluster
Name |
Type |
Required |
Description |
id |
string |
false |
|
location |
Location |
false |
|
Location
Name |
Type |
Required |
Description |
type |
string |
false |
|
coordinates |
number[ ] |
false |
|
WeatherHourly
Name |
Type |
Required |
Description |
time |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
temperatureC |
number |
false |
|
dewPointC |
number |
false |
|
windKmH |
number |
false |
|
relativeHumidityPercent |
number |
false |
|
precipitationProbabilityPercent |
number |
false |
|
cloudCoverPercent |
number |
false |
|
pressureKilopascals |
number |
false |
|
windGustKmH |
number |
false |
|
timeEpoch |
integer |
false |
|
WeatherDaily
Name |
Type |
Required |
Description |
time |
integer |
false |
|
sunrise |
integer |
false |
|
sunset |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
dewPointC |
number |
false |
|
minTemperatureC |
number |
false |
|
maxTemperatureC |
number |
false |
|
minWindKmH |
number |
false |
|
maxWindKmH |
number |
false |
|
avgPressureKilopascals |
number |
false |
|
avgCloudCoverPercent |
number |
false |
|
avgRelativeHumidityPercent |
number |
false |
|
maxPrecipitationProbabilityPercent |
number |
false |
|
avgTemperatureC |
number |
false |
|
minRelativeHumidityPercent |
number |
false |
|
maxRelativeHumidityPercent |
number |
false |
|
WeatherMonthly
Name |
Type |
Required |
Description |
time |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
minTemperatureC |
number |
false |
|
maxTemperatureC |
number |
false |
|
minWindKmH |
number |
false |
|
maxWindKmH |
number |
false |
|
avgPressureKilopascals |
number |
false |
|
avgCloudCoverPercent |
number |
false |
|
avgRelativeHumidityPercent |
number |
false |
|
maxPrecipitationProbabilityPercent |
number |
false |
|
avgWindKmH |
number |
false |
|
avgTemperatureC |
number |
false |
|
avgDewPointC |
number |
false |
|
minRelativeHumidityPercent |
number |
false |
|
maxRelativeHumidityPercent |
number |
false |
|
Get atmosphere data bulk
Request
curl "https://developer-api.etwater.com/api/v2/atmosphere/extended/bulk" \
-X POST \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
--data "[
{
\"longitude\": 51,
\"latitude\": 298.92983674021895,
\"startTimestamp\": 343,
\"endTimestamp\": 699,
\"interval\": \"HOUR\",
\"keys\": [
\"PRECIPITATION_PROBABILITY\"
],
\"loadMissingWeather\": \"1\",
\"calcMissingEt\": true
}
]"
On success, the above request returns response like
[
{
"cluster": {
"id": "example string value",
"location": {
"type": "example string value",
"coordinates": [
806.0586279286345
]
}
},
"hourly": [
{
"time": 812,
"etMm": 908,
"rainMm": 747.2563915640378,
"ghWm2": 61,
"temperatureC": 554,
"dewPointC": 972.0847690347977,
"windKmH": 78.53408347747013,
"relativeHumidityPercent": 747.7838326002396,
"precipitationProbabilityPercent": 215.9923921413684,
"cloudCoverPercent": 586.6410539423307,
"pressureKilopascals": 70,
"windGustKmH": 40,
"timeEpoch": 720
}
],
"daily": [
{
"time": 946,
"sunrise": 463,
"sunset": 260,
"etMm": 328,
"rainMm": 573,
"ghWm2": 344,
"dewPointC": 570.4720572430975,
"minTemperatureC": 847.3867438022917,
"maxTemperatureC": 494.5821554840459,
"minWindKmH": 5.154655783043548,
"maxWindKmH": 851.8142294379949,
"avgPressureKilopascals": 524.8075930982864,
"avgCloudCoverPercent": 99.92673858065471,
"avgRelativeHumidityPercent": 803.7254693003956,
"maxPrecipitationProbabilityPercent": 425,
"avgTemperatureC": 525.5255589846175,
"minRelativeHumidityPercent": 207.45810084392227,
"maxRelativeHumidityPercent": 566.7270866999063
}
],
"monthly": [
{
"time": 118,
"etMm": 380,
"rainMm": 96.10195602108816,
"ghWm2": 836,
"minTemperatureC": 884.1146397796063,
"maxTemperatureC": 206,
"minWindKmH": 293,
"maxWindKmH": 467,
"avgPressureKilopascals": 255,
"avgCloudCoverPercent": 750.4641128473282,
"avgRelativeHumidityPercent": 855.3113950674009,
"maxPrecipitationProbabilityPercent": 81,
"avgWindKmH": 724,
"avgTemperatureC": 854.764893117717,
"avgDewPointC": 622.4471184529583,
"minRelativeHumidityPercent": 371,
"maxRelativeHumidityPercent": 741.556111137176
}
],
"status": 119
}
]
HTTP Request
POST /api/v2/atmosphere/extended/bulk
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
GetWeatherByCoordinatesRequest |
GetWeatherByCoordinatesRequest[ ] |
body |
true |
|
GetWeatherByCoordinatesRequest
Name |
Type |
Required |
Description |
longitude |
number |
true |
|
latitude |
number |
true |
|
startTimestamp |
integer |
true |
|
endTimestamp |
integer |
true |
|
interval |
string |
true |
|
keys |
string[ ] |
true |
|
loadMissingWeather |
boolean |
false |
|
calcMissingEt |
boolean |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response is array of
Name |
Type |
Required |
Description |
cluster |
WeatherCluster |
true |
|
hourly |
WeatherHourly[ ] |
false |
|
daily |
WeatherDaily[ ] |
false |
|
monthly |
WeatherMonthly[ ] |
false |
|
status |
integer |
true |
|
WeatherCluster
Name |
Type |
Required |
Description |
id |
string |
false |
|
location |
Location |
false |
|
Location
Name |
Type |
Required |
Description |
type |
string |
false |
|
coordinates |
number[ ] |
false |
|
WeatherHourly
Name |
Type |
Required |
Description |
time |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
temperatureC |
number |
false |
|
dewPointC |
number |
false |
|
windKmH |
number |
false |
|
relativeHumidityPercent |
number |
false |
|
precipitationProbabilityPercent |
number |
false |
|
cloudCoverPercent |
number |
false |
|
pressureKilopascals |
number |
false |
|
windGustKmH |
number |
false |
|
timeEpoch |
integer |
false |
|
WeatherDaily
Name |
Type |
Required |
Description |
time |
integer |
false |
|
sunrise |
integer |
false |
|
sunset |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
dewPointC |
number |
false |
|
minTemperatureC |
number |
false |
|
maxTemperatureC |
number |
false |
|
minWindKmH |
number |
false |
|
maxWindKmH |
number |
false |
|
avgPressureKilopascals |
number |
false |
|
avgCloudCoverPercent |
number |
false |
|
avgRelativeHumidityPercent |
number |
false |
|
maxPrecipitationProbabilityPercent |
number |
false |
|
avgTemperatureC |
number |
false |
|
minRelativeHumidityPercent |
number |
false |
|
maxRelativeHumidityPercent |
number |
false |
|
WeatherMonthly
Name |
Type |
Required |
Description |
time |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
minTemperatureC |
number |
false |
|
maxTemperatureC |
number |
false |
|
minWindKmH |
number |
false |
|
maxWindKmH |
number |
false |
|
avgPressureKilopascals |
number |
false |
|
avgCloudCoverPercent |
number |
false |
|
avgRelativeHumidityPercent |
number |
false |
|
maxPrecipitationProbabilityPercent |
number |
false |
|
avgWindKmH |
number |
false |
|
avgTemperatureC |
number |
false |
|
avgDewPointC |
number |
false |
|
minRelativeHumidityPercent |
number |
false |
|
maxRelativeHumidityPercent |
number |
false |
|
V2 | Atmosphere Weather Cluster API
Get wether cluster by coordinates
Request
curl "https://developer-api.etwater.com/api/v2/atmosphere/weather-clusters/coordinates/{longitude}/{latitude}" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"id": "example string value",
"location": {
"type": "example string value",
"coordinates": [
227.94712112655264
]
}
}
HTTP Request
GET /api/v2/atmosphere/weather-clusters/coordinates/{longitude}/{latitude}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
longitude |
number |
path |
true |
|
latitude |
number |
path |
true |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
false |
|
location |
Location |
false |
|
Location
Name |
Type |
Required |
Description |
type |
string |
false |
|
coordinates |
number[ ] |
false |
|
Get wether cluster by coordinates bulk
Request
curl "https://developer-api.etwater.com/api/v2/atmosphere/weather-clusters/bulk/get-by-coordinates" \
-X POST \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
--data "[
{
\"longitude\": -82,
\"latitude\": 52.08794416956974
}
]"
On success, the above request returns response like
[
{
"id": "example string value",
"location": {
"type": "example string value",
"coordinates": [
735.3479912203494
]
}
}
]
HTTP Request
POST /api/v2/atmosphere/weather-clusters/bulk/get-by-coordinates
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
GetWeatherClusterByCoordinatesRequest |
GetWeatherClusterByCoordinatesRequest[ ] |
body |
true |
|
GetWeatherClusterByCoordinatesRequest
Name |
Type |
Required |
Description |
longitude |
number |
true |
|
latitude |
number |
true |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response is array of
Name |
Type |
Required |
Description |
id |
string |
false |
|
location |
Location |
false |
|
Location
Name |
Type |
Required |
Description |
type |
string |
false |
|
coordinates |
number[ ] |
false |
|
Activate weather cluster bulk
Request
curl "https://developer-api.etwater.com/api/v2/atmosphere/weather-clusters/bulk/activate" \
-X POST \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
--data "[
{
\"clusterId\": \"example string value\"
}
]"
On success, the above request returns response like
HTTP Request
POST /api/v2/atmosphere/weather-clusters/bulk/activate
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
ActivateWeatherClusterRequest |
ActivateWeatherClusterRequest[ ] |
body |
true |
|
ActivateWeatherClusterRequest
Name |
Type |
Required |
Description |
clusterId |
string |
true |
|
HTTP Responses
Code |
Description |
204 |
No Content |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
V2 | Controllers | Time Restrictions
Get Time Restrictions
Request
curl "https://developer-api.etwater.com/api/v2/controllers/time-restrictions?controllerId=example+string+value&siteId=example+string+value&stationGroupId=example+string+value&active=0" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
[
{
"id": "example string value",
"controllerId": "example string value",
"name": "example string value",
"repeatPattern": {
"dailyRepeatPattern": {
"repeatCount": 445,
"applyUntilDateTime": "example string value",
"interval": 746
},
"weeklyRepeatPattern": {
"repeatCount": 663,
"applyUntilDateTime": "example string value",
"interval": 364,
"daysOfWeek": [
"Fri"
]
},
"monthlyRepeatPattern": {
"repeatCount": 180,
"applyUntilDateTime": "example string value",
"interval": 708,
"daysOfMonth": null,
"positionInMonth": 948,
"daysOfWeek": [
"Sat"
]
},
"yearlyRepeatPattern": {
"repeatCount": 610,
"applyUntilDateTime": "example string value",
"interval": 205,
"daysOfWeek": [
"Thu"
],
"daysOfYear": null,
"numbersOfWeek": null
},
"oddDaysRepeatPattern": {
"repeatCount": 535,
"applyUntilDateTime": "example string value"
},
"evenDaysRepeatPattern": {
"repeatCount": 619,
"applyUntilDateTime": "example string value"
},
"weeklySameDayRepeatPattern": {
"repeatCount": 689,
"applyUntilDateTime": "example string value"
},
"weeklyWorkdaysRepeatPattern": {
"repeatCount": 699,
"applyUntilDateTime": "example string value"
},
"monthlySameDateRepeatPattern": {
"repeatCount": 135,
"applyUntilDateTime": "example string value"
},
"monthlySameDayRepeatPattern": {
"repeatCount": 83,
"applyUntilDateTime": "example string value"
},
"annuallySameDateRepeatPattern": {
"repeatCount": 719,
"applyUntilDateTime": "example string value"
}
},
"createdAt": "example string value",
"updatedAt": "example string value",
"startDateTime": "example string value",
"endDateTime": "example string value",
"areaRegionTypeIds": [
649
],
"emitterTypeIds": [
396
],
"stationNumbers": [
57
],
"allStations": true,
"effect": "DENY"
}
]
HTTP Request
GET /api/v2/controllers/time-restrictions
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
controllerId |
string |
query |
false |
|
siteId |
string |
query |
false |
|
stationGroupId |
string |
query |
false |
|
active |
boolean |
query |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response is array of
Name |
Type |
Required |
Description |
id |
string |
true |
|
controllerId |
string |
true |
|
name |
string |
true |
|
repeatPattern |
RepeatPattern |
false |
|
createdAt |
string |
true |
|
updatedAt |
string |
true |
|
startDateTime |
string |
true |
|
endDateTime |
string |
true |
|
areaRegionTypeIds |
integer[ ] |
false |
|
emitterTypeIds |
integer[ ] |
false |
|
stationNumbers |
integer[ ] |
false |
|
allStations |
boolean |
false |
|
effect |
string |
true |
|
RepeatPattern
Name |
Type |
Required |
Description |
dailyRepeatPattern |
DailyRepeatPattern |
false |
|
weeklyRepeatPattern |
WeeklyRepeatPattern |
false |
|
monthlyRepeatPattern |
MonthlyRepeatPattern |
false |
|
yearlyRepeatPattern |
YearlyRepeatPattern |
false |
|
oddDaysRepeatPattern |
OddDaysRepeatPattern |
false |
|
evenDaysRepeatPattern |
EvenDaysRepeatPattern |
false |
|
weeklySameDayRepeatPattern |
WeeklySameDayRepeatPattern |
false |
|
weeklyWorkdaysRepeatPattern |
WeeklyWorkdaysRepeatPattern |
false |
|
monthlySameDateRepeatPattern |
MonthlySameDateRepeatPattern |
false |
|
monthlySameDayRepeatPattern |
MonthlySameDayRepeatPattern |
false |
|
annuallySameDateRepeatPattern |
AnnuallySameDateRepeatPattern |
false |
|
DailyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
WeeklyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
daysOfWeek |
string[ ] |
false |
|
MonthlyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
daysOfMonth |
integer[ ] |
false |
|
positionInMonth |
integer |
false |
|
daysOfWeek |
string[ ] |
false |
|
YearlyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
daysOfWeek |
string[ ] |
false |
|
daysOfYear |
integer[ ] |
false |
|
numbersOfWeek |
integer[ ] |
false |
|
OddDaysRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
EvenDaysRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
WeeklySameDayRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
WeeklyWorkdaysRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
MonthlySameDateRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
MonthlySameDayRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
AnnuallySameDateRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
Get a Time Restriction by id
Request
curl "https://developer-api.etwater.com/api/v2/controllers/time-restrictions/{id}" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"id": "example string value",
"controllerId": "example string value",
"name": "example string value",
"repeatPattern": {
"dailyRepeatPattern": {
"repeatCount": 897,
"applyUntilDateTime": "example string value",
"interval": 888
},
"weeklyRepeatPattern": {
"repeatCount": 688,
"applyUntilDateTime": "example string value",
"interval": 278,
"daysOfWeek": [
"Wed"
]
},
"monthlyRepeatPattern": {
"repeatCount": 410,
"applyUntilDateTime": "example string value",
"interval": 765,
"daysOfMonth": null,
"positionInMonth": 686,
"daysOfWeek": [
"Thu"
]
},
"yearlyRepeatPattern": {
"repeatCount": 226,
"applyUntilDateTime": "example string value",
"interval": 771,
"daysOfWeek": [
"Fri"
],
"daysOfYear": null,
"numbersOfWeek": null
},
"oddDaysRepeatPattern": {
"repeatCount": 797,
"applyUntilDateTime": "example string value"
},
"evenDaysRepeatPattern": {
"repeatCount": 999,
"applyUntilDateTime": "example string value"
},
"weeklySameDayRepeatPattern": {
"repeatCount": 424,
"applyUntilDateTime": "example string value"
},
"weeklyWorkdaysRepeatPattern": {
"repeatCount": 412,
"applyUntilDateTime": "example string value"
},
"monthlySameDateRepeatPattern": {
"repeatCount": 187,
"applyUntilDateTime": "example string value"
},
"monthlySameDayRepeatPattern": {
"repeatCount": 304,
"applyUntilDateTime": "example string value"
},
"annuallySameDateRepeatPattern": {
"repeatCount": 739,
"applyUntilDateTime": "example string value"
}
},
"createdAt": "example string value",
"updatedAt": "example string value",
"startDateTime": "example string value",
"endDateTime": "example string value",
"areaRegionTypeIds": [
619
],
"emitterTypeIds": [
949
],
"stationNumbers": [
210
],
"allStations": false,
"effect": "DENY"
}
HTTP Request
GET /api/v2/controllers/time-restrictions/{id}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
id |
string |
path |
true |
|
HTTP Responses
Code |
Description |
200 |
OK |
404 |
Not Found |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
true |
|
controllerId |
string |
true |
|
name |
string |
true |
|
repeatPattern |
RepeatPattern |
false |
|
createdAt |
string |
true |
|
updatedAt |
string |
true |
|
startDateTime |
string |
true |
|
endDateTime |
string |
true |
|
areaRegionTypeIds |
integer[ ] |
false |
|
emitterTypeIds |
integer[ ] |
false |
|
stationNumbers |
integer[ ] |
false |
|
allStations |
boolean |
false |
|
effect |
string |
true |
|
RepeatPattern
Name |
Type |
Required |
Description |
dailyRepeatPattern |
DailyRepeatPattern |
false |
|
weeklyRepeatPattern |
WeeklyRepeatPattern |
false |
|
monthlyRepeatPattern |
MonthlyRepeatPattern |
false |
|
yearlyRepeatPattern |
YearlyRepeatPattern |
false |
|
oddDaysRepeatPattern |
OddDaysRepeatPattern |
false |
|
evenDaysRepeatPattern |
EvenDaysRepeatPattern |
false |
|
weeklySameDayRepeatPattern |
WeeklySameDayRepeatPattern |
false |
|
weeklyWorkdaysRepeatPattern |
WeeklyWorkdaysRepeatPattern |
false |
|
monthlySameDateRepeatPattern |
MonthlySameDateRepeatPattern |
false |
|
monthlySameDayRepeatPattern |
MonthlySameDayRepeatPattern |
false |
|
annuallySameDateRepeatPattern |
AnnuallySameDateRepeatPattern |
false |
|
DailyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
WeeklyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
daysOfWeek |
string[ ] |
false |
|
MonthlyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
daysOfMonth |
integer[ ] |
false |
|
positionInMonth |
integer |
false |
|
daysOfWeek |
string[ ] |
false |
|
YearlyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
daysOfWeek |
string[ ] |
false |
|
daysOfYear |
integer[ ] |
false |
|
numbersOfWeek |
integer[ ] |
false |
|
OddDaysRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
EvenDaysRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
WeeklySameDayRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
WeeklyWorkdaysRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
MonthlySameDateRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
MonthlySameDayRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
AnnuallySameDateRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
Delete a Time Restriction by id
Request
curl "https://developer-api.etwater.com/api/v2/controllers/time-restrictions/{id}" \
-X DELETE \
-H "Authorization: example string value"
On success, the above request returns response like
HTTP Request
DELETE /api/v2/controllers/time-restrictions/{id}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
id |
string |
path |
true |
|
HTTP Responses
Code |
Description |
204 |
No Content |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Update a Time Restriction by id
Request
curl "https://developer-api.etwater.com/api/v2/controllers/time-restrictions/{id}" \
-X PUT \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
--data "{
\"id\": \"example string value\",
\"controllerId\": \"example string value\",
\"name\": \"example string value\",
\"repeatPattern\": {
\"dailyRepeatPattern\": {
\"repeatCount\": 62,
\"applyUntilDateTime\": \"example string value\",
\"interval\": 702
},
\"weeklyRepeatPattern\": {
\"repeatCount\": 155,
\"applyUntilDateTime\": \"example string value\",
\"interval\": 873,
\"daysOfWeek\": [
\"Thu\"
]
},
\"monthlyRepeatPattern\": {
\"repeatCount\": 904,
\"applyUntilDateTime\": \"example string value\",
\"interval\": 83,
\"daysOfMonth\": null,
\"positionInMonth\": 494,
\"daysOfWeek\": [
\"Sun\"
]
},
\"yearlyRepeatPattern\": {
\"repeatCount\": 102,
\"applyUntilDateTime\": \"example string value\",
\"interval\": 381,
\"daysOfWeek\": [
\"Sat\"
],
\"daysOfYear\": null,
\"numbersOfWeek\": null
},
\"oddDaysRepeatPattern\": {
\"repeatCount\": 887,
\"applyUntilDateTime\": \"example string value\"
},
\"evenDaysRepeatPattern\": {
\"repeatCount\": 386,
\"applyUntilDateTime\": \"example string value\"
},
\"weeklySameDayRepeatPattern\": {
\"repeatCount\": 744,
\"applyUntilDateTime\": \"example string value\"
},
\"weeklyWorkdaysRepeatPattern\": {
\"repeatCount\": 566,
\"applyUntilDateTime\": \"example string value\"
},
\"monthlySameDateRepeatPattern\": {
\"repeatCount\": 82,
\"applyUntilDateTime\": \"example string value\"
},
\"monthlySameDayRepeatPattern\": {
\"repeatCount\": 813,
\"applyUntilDateTime\": \"example string value\"
},
\"annuallySameDateRepeatPattern\": {
\"repeatCount\": 926,
\"applyUntilDateTime\": \"example string value\"
}
},
\"startDateTime\": \"example string value\",
\"endDateTime\": \"example string value\",
\"areaRegionTypeIds\": [
236
],
\"emitterTypeIds\": [
168
],
\"stationNumbers\": [
739
],
\"allStations\": false,
\"effect\": \"DENY\"
}"
On success, the above request returns response like
{
"id": "example string value",
"controllerId": "example string value",
"name": "example string value",
"repeatPattern": {
"dailyRepeatPattern": {
"repeatCount": 468,
"applyUntilDateTime": "example string value",
"interval": 472
},
"weeklyRepeatPattern": {
"repeatCount": 292,
"applyUntilDateTime": "example string value",
"interval": 237,
"daysOfWeek": [
"Thu"
]
},
"monthlyRepeatPattern": {
"repeatCount": 882,
"applyUntilDateTime": "example string value",
"interval": 740,
"daysOfMonth": null,
"positionInMonth": 678,
"daysOfWeek": [
"Sat"
]
},
"yearlyRepeatPattern": {
"repeatCount": 475,
"applyUntilDateTime": "example string value",
"interval": 744,
"daysOfWeek": [
"Fri"
],
"daysOfYear": null,
"numbersOfWeek": null
},
"oddDaysRepeatPattern": {
"repeatCount": 927,
"applyUntilDateTime": "example string value"
},
"evenDaysRepeatPattern": {
"repeatCount": 403,
"applyUntilDateTime": "example string value"
},
"weeklySameDayRepeatPattern": {
"repeatCount": 350,
"applyUntilDateTime": "example string value"
},
"weeklyWorkdaysRepeatPattern": {
"repeatCount": 282,
"applyUntilDateTime": "example string value"
},
"monthlySameDateRepeatPattern": {
"repeatCount": 107,
"applyUntilDateTime": "example string value"
},
"monthlySameDayRepeatPattern": {
"repeatCount": 389,
"applyUntilDateTime": "example string value"
},
"annuallySameDateRepeatPattern": {
"repeatCount": 394,
"applyUntilDateTime": "example string value"
}
},
"createdAt": "example string value",
"updatedAt": "example string value",
"startDateTime": "example string value",
"endDateTime": "example string value",
"areaRegionTypeIds": [
312
],
"emitterTypeIds": [
299
],
"stationNumbers": [
778
],
"allStations": false,
"effect": "ALLOW"
}
HTTP Request
PUT /api/v2/controllers/time-restrictions/{id}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
id |
string |
path |
true |
|
UpdateTimeRestrictionRequest |
UpdateTimeRestrictionRequest |
body |
true |
|
UpdateTimeRestrictionRequest
Name |
Type |
Required |
Description |
id |
string |
true |
|
controllerId |
string |
true |
|
name |
string |
true |
|
repeatPattern |
RepeatPattern |
false |
|
startDateTime |
string |
true |
|
endDateTime |
string |
true |
|
areaRegionTypeIds |
integer[ ] |
false |
|
emitterTypeIds |
integer[ ] |
false |
|
stationNumbers |
integer[ ] |
false |
|
allStations |
boolean |
false |
|
effect |
string |
true |
|
RepeatPattern
Name |
Type |
Required |
Description |
dailyRepeatPattern |
DailyRepeatPattern |
false |
|
weeklyRepeatPattern |
WeeklyRepeatPattern |
false |
|
monthlyRepeatPattern |
MonthlyRepeatPattern |
false |
|
yearlyRepeatPattern |
YearlyRepeatPattern |
false |
|
oddDaysRepeatPattern |
OddDaysRepeatPattern |
false |
|
evenDaysRepeatPattern |
EvenDaysRepeatPattern |
false |
|
weeklySameDayRepeatPattern |
WeeklySameDayRepeatPattern |
false |
|
weeklyWorkdaysRepeatPattern |
WeeklyWorkdaysRepeatPattern |
false |
|
monthlySameDateRepeatPattern |
MonthlySameDateRepeatPattern |
false |
|
monthlySameDayRepeatPattern |
MonthlySameDayRepeatPattern |
false |
|
annuallySameDateRepeatPattern |
AnnuallySameDateRepeatPattern |
false |
|
DailyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
WeeklyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
daysOfWeek |
string[ ] |
false |
|
MonthlyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
daysOfMonth |
integer[ ] |
false |
|
positionInMonth |
integer |
false |
|
daysOfWeek |
string[ ] |
false |
|
YearlyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
daysOfWeek |
string[ ] |
false |
|
daysOfYear |
integer[ ] |
false |
|
numbersOfWeek |
integer[ ] |
false |
|
OddDaysRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
EvenDaysRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
WeeklySameDayRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
WeeklyWorkdaysRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
MonthlySameDateRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
MonthlySameDayRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
AnnuallySameDateRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
404 |
Not Found |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
true |
|
controllerId |
string |
true |
|
name |
string |
true |
|
repeatPattern |
RepeatPattern |
false |
|
createdAt |
string |
true |
|
updatedAt |
string |
true |
|
startDateTime |
string |
true |
|
endDateTime |
string |
true |
|
areaRegionTypeIds |
integer[ ] |
false |
|
emitterTypeIds |
integer[ ] |
false |
|
stationNumbers |
integer[ ] |
false |
|
allStations |
boolean |
false |
|
effect |
string |
true |
|
RepeatPattern
Name |
Type |
Required |
Description |
dailyRepeatPattern |
DailyRepeatPattern |
false |
|
weeklyRepeatPattern |
WeeklyRepeatPattern |
false |
|
monthlyRepeatPattern |
MonthlyRepeatPattern |
false |
|
yearlyRepeatPattern |
YearlyRepeatPattern |
false |
|
oddDaysRepeatPattern |
OddDaysRepeatPattern |
false |
|
evenDaysRepeatPattern |
EvenDaysRepeatPattern |
false |
|
weeklySameDayRepeatPattern |
WeeklySameDayRepeatPattern |
false |
|
weeklyWorkdaysRepeatPattern |
WeeklyWorkdaysRepeatPattern |
false |
|
monthlySameDateRepeatPattern |
MonthlySameDateRepeatPattern |
false |
|
monthlySameDayRepeatPattern |
MonthlySameDayRepeatPattern |
false |
|
annuallySameDateRepeatPattern |
AnnuallySameDateRepeatPattern |
false |
|
DailyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
WeeklyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
daysOfWeek |
string[ ] |
false |
|
MonthlyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
daysOfMonth |
integer[ ] |
false |
|
positionInMonth |
integer |
false |
|
daysOfWeek |
string[ ] |
false |
|
YearlyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
daysOfWeek |
string[ ] |
false |
|
daysOfYear |
integer[ ] |
false |
|
numbersOfWeek |
integer[ ] |
false |
|
OddDaysRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
EvenDaysRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
WeeklySameDayRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
WeeklyWorkdaysRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
MonthlySameDateRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
MonthlySameDayRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
AnnuallySameDateRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
Copy Time Restriction
Request
curl "https://developer-api.etwater.com/api/v2/controllers/time-restrictions/{id}/rpc/copy" \
-X POST \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
--data "{
\"sourceId\": \"example string value\",
\"destinationName\": \"example string value\",
\"destinationControllerId\": \"example string value\"
}"
On success, the above request returns response like
HTTP Request
POST /api/v2/controllers/time-restrictions/{id}/rpc/copy
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
id |
string |
path |
true |
|
TimeRestriction |
CopyTimeRestrictionRequest |
body |
true |
|
CopyTimeRestrictionRequest
Name |
Type |
Required |
Description |
sourceId |
string |
true |
|
destinationName |
string |
false |
|
destinationControllerId |
string |
true |
|
HTTP Responses
Code |
Description |
201 |
Created |
404 |
Not Found |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
true |
|
controllerId |
string |
true |
|
name |
string |
true |
|
repeatPattern |
RepeatPattern |
false |
|
createdAt |
string |
true |
|
updatedAt |
string |
true |
|
startDateTime |
string |
true |
|
endDateTime |
string |
true |
|
areaRegionTypeIds |
integer[ ] |
false |
|
emitterTypeIds |
integer[ ] |
false |
|
stationNumbers |
integer[ ] |
false |
|
allStations |
boolean |
false |
|
effect |
string |
true |
|
RepeatPattern
Name |
Type |
Required |
Description |
dailyRepeatPattern |
DailyRepeatPattern |
false |
|
weeklyRepeatPattern |
WeeklyRepeatPattern |
false |
|
monthlyRepeatPattern |
MonthlyRepeatPattern |
false |
|
yearlyRepeatPattern |
YearlyRepeatPattern |
false |
|
oddDaysRepeatPattern |
OddDaysRepeatPattern |
false |
|
evenDaysRepeatPattern |
EvenDaysRepeatPattern |
false |
|
weeklySameDayRepeatPattern |
WeeklySameDayRepeatPattern |
false |
|
weeklyWorkdaysRepeatPattern |
WeeklyWorkdaysRepeatPattern |
false |
|
monthlySameDateRepeatPattern |
MonthlySameDateRepeatPattern |
false |
|
monthlySameDayRepeatPattern |
MonthlySameDayRepeatPattern |
false |
|
annuallySameDateRepeatPattern |
AnnuallySameDateRepeatPattern |
false |
|
DailyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
WeeklyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
daysOfWeek |
string[ ] |
false |
|
MonthlyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
daysOfMonth |
integer[ ] |
false |
|
positionInMonth |
integer |
false |
|
daysOfWeek |
string[ ] |
false |
|
YearlyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
daysOfWeek |
string[ ] |
false |
|
daysOfYear |
integer[ ] |
false |
|
numbersOfWeek |
integer[ ] |
false |
|
OddDaysRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
EvenDaysRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
WeeklySameDayRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
WeeklyWorkdaysRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
MonthlySameDateRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
MonthlySameDayRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
AnnuallySameDateRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
Get Time Restrictions
Request
curl "https://developer-api.etwater.com/api/v2/controllers/{controllerId}/time-restrictions?active=1" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
[
{
"id": "example string value",
"controllerId": "example string value",
"name": "example string value",
"repeatPattern": {
"dailyRepeatPattern": {
"repeatCount": 484,
"applyUntilDateTime": "example string value",
"interval": 980
},
"weeklyRepeatPattern": {
"repeatCount": 963,
"applyUntilDateTime": "example string value",
"interval": 314,
"daysOfWeek": [
"Tue"
]
},
"monthlyRepeatPattern": {
"repeatCount": 766,
"applyUntilDateTime": "example string value",
"interval": 482,
"daysOfMonth": null,
"positionInMonth": 260,
"daysOfWeek": [
"Sun"
]
},
"yearlyRepeatPattern": {
"repeatCount": 780,
"applyUntilDateTime": "example string value",
"interval": 950,
"daysOfWeek": [
"Thu"
],
"daysOfYear": null,
"numbersOfWeek": null
},
"oddDaysRepeatPattern": {
"repeatCount": 748,
"applyUntilDateTime": "example string value"
},
"evenDaysRepeatPattern": {
"repeatCount": 360,
"applyUntilDateTime": "example string value"
},
"weeklySameDayRepeatPattern": {
"repeatCount": 280,
"applyUntilDateTime": "example string value"
},
"weeklyWorkdaysRepeatPattern": {
"repeatCount": 46,
"applyUntilDateTime": "example string value"
},
"monthlySameDateRepeatPattern": {
"repeatCount": 116,
"applyUntilDateTime": "example string value"
},
"monthlySameDayRepeatPattern": {
"repeatCount": 462,
"applyUntilDateTime": "example string value"
},
"annuallySameDateRepeatPattern": {
"repeatCount": 694,
"applyUntilDateTime": "example string value"
}
},
"createdAt": "example string value",
"updatedAt": "example string value",
"startDateTime": "example string value",
"endDateTime": "example string value",
"areaRegionTypeIds": [
11
],
"emitterTypeIds": [
28
],
"stationNumbers": [
32
],
"allStations": false,
"effect": "ALLOW"
}
]
HTTP Request
GET /api/v2/controllers/{controllerId}/time-restrictions
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
controllerId |
string |
path |
true |
|
active |
boolean |
query |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response is array of
Name |
Type |
Required |
Description |
id |
string |
true |
|
controllerId |
string |
true |
|
name |
string |
true |
|
repeatPattern |
RepeatPattern |
false |
|
createdAt |
string |
true |
|
updatedAt |
string |
true |
|
startDateTime |
string |
true |
|
endDateTime |
string |
true |
|
areaRegionTypeIds |
integer[ ] |
false |
|
emitterTypeIds |
integer[ ] |
false |
|
stationNumbers |
integer[ ] |
false |
|
allStations |
boolean |
false |
|
effect |
string |
true |
|
RepeatPattern
Name |
Type |
Required |
Description |
dailyRepeatPattern |
DailyRepeatPattern |
false |
|
weeklyRepeatPattern |
WeeklyRepeatPattern |
false |
|
monthlyRepeatPattern |
MonthlyRepeatPattern |
false |
|
yearlyRepeatPattern |
YearlyRepeatPattern |
false |
|
oddDaysRepeatPattern |
OddDaysRepeatPattern |
false |
|
evenDaysRepeatPattern |
EvenDaysRepeatPattern |
false |
|
weeklySameDayRepeatPattern |
WeeklySameDayRepeatPattern |
false |
|
weeklyWorkdaysRepeatPattern |
WeeklyWorkdaysRepeatPattern |
false |
|
monthlySameDateRepeatPattern |
MonthlySameDateRepeatPattern |
false |
|
monthlySameDayRepeatPattern |
MonthlySameDayRepeatPattern |
false |
|
annuallySameDateRepeatPattern |
AnnuallySameDateRepeatPattern |
false |
|
DailyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
WeeklyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
daysOfWeek |
string[ ] |
false |
|
MonthlyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
daysOfMonth |
integer[ ] |
false |
|
positionInMonth |
integer |
false |
|
daysOfWeek |
string[ ] |
false |
|
YearlyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
daysOfWeek |
string[ ] |
false |
|
daysOfYear |
integer[ ] |
false |
|
numbersOfWeek |
integer[ ] |
false |
|
OddDaysRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
EvenDaysRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
WeeklySameDayRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
WeeklyWorkdaysRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
MonthlySameDateRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
MonthlySameDayRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
AnnuallySameDateRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
Create a Time Restriction
Request
curl "https://developer-api.etwater.com/api/v2/controllers/{controllerId}/time-restrictions" \
-X POST \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
--data "{
\"controllerId\": \"example string value\",
\"name\": \"example string value\",
\"repeatPattern\": {
\"dailyRepeatPattern\": {
\"repeatCount\": 320,
\"applyUntilDateTime\": \"example string value\",
\"interval\": 875
},
\"weeklyRepeatPattern\": {
\"repeatCount\": 397,
\"applyUntilDateTime\": \"example string value\",
\"interval\": 902,
\"daysOfWeek\": [
\"Tue\"
]
},
\"monthlyRepeatPattern\": {
\"repeatCount\": 41,
\"applyUntilDateTime\": \"example string value\",
\"interval\": 798,
\"daysOfMonth\": null,
\"positionInMonth\": 782,
\"daysOfWeek\": [
\"Fri\"
]
},
\"yearlyRepeatPattern\": {
\"repeatCount\": 424,
\"applyUntilDateTime\": \"example string value\",
\"interval\": 89,
\"daysOfWeek\": [
\"Thu\"
],
\"daysOfYear\": null,
\"numbersOfWeek\": null
},
\"oddDaysRepeatPattern\": {
\"repeatCount\": 855,
\"applyUntilDateTime\": \"example string value\"
},
\"evenDaysRepeatPattern\": {
\"repeatCount\": 590,
\"applyUntilDateTime\": \"example string value\"
},
\"weeklySameDayRepeatPattern\": {
\"repeatCount\": 581,
\"applyUntilDateTime\": \"example string value\"
},
\"weeklyWorkdaysRepeatPattern\": {
\"repeatCount\": 345,
\"applyUntilDateTime\": \"example string value\"
},
\"monthlySameDateRepeatPattern\": {
\"repeatCount\": 422,
\"applyUntilDateTime\": \"example string value\"
},
\"monthlySameDayRepeatPattern\": {
\"repeatCount\": 216,
\"applyUntilDateTime\": \"example string value\"
},
\"annuallySameDateRepeatPattern\": {
\"repeatCount\": 273,
\"applyUntilDateTime\": \"example string value\"
}
},
\"startDateTime\": \"example string value\",
\"endDateTime\": \"example string value\",
\"areaRegionTypeIds\": [
219
],
\"emitterTypeIds\": [
747
],
\"stationNumbers\": [
252
],
\"allStations\": true,
\"effect\": \"ALLOW\"
}"
On success, the above request returns response like
HTTP Request
POST /api/v2/controllers/{controllerId}/time-restrictions
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
controllerId |
string |
path |
true |
|
CreateTimeRestrictionRequest |
CreateTimeRestrictionRequest |
body |
true |
|
CreateTimeRestrictionRequest
Name |
Type |
Required |
Description |
controllerId |
string |
true |
|
name |
string |
true |
|
repeatPattern |
RepeatPattern |
false |
|
startDateTime |
string |
true |
|
endDateTime |
string |
true |
|
areaRegionTypeIds |
integer[ ] |
false |
|
emitterTypeIds |
integer[ ] |
false |
|
stationNumbers |
integer[ ] |
false |
|
allStations |
boolean |
false |
|
effect |
string |
true |
|
RepeatPattern
Name |
Type |
Required |
Description |
dailyRepeatPattern |
DailyRepeatPattern |
false |
|
weeklyRepeatPattern |
WeeklyRepeatPattern |
false |
|
monthlyRepeatPattern |
MonthlyRepeatPattern |
false |
|
yearlyRepeatPattern |
YearlyRepeatPattern |
false |
|
oddDaysRepeatPattern |
OddDaysRepeatPattern |
false |
|
evenDaysRepeatPattern |
EvenDaysRepeatPattern |
false |
|
weeklySameDayRepeatPattern |
WeeklySameDayRepeatPattern |
false |
|
weeklyWorkdaysRepeatPattern |
WeeklyWorkdaysRepeatPattern |
false |
|
monthlySameDateRepeatPattern |
MonthlySameDateRepeatPattern |
false |
|
monthlySameDayRepeatPattern |
MonthlySameDayRepeatPattern |
false |
|
annuallySameDateRepeatPattern |
AnnuallySameDateRepeatPattern |
false |
|
DailyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
WeeklyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
daysOfWeek |
string[ ] |
false |
|
MonthlyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
daysOfMonth |
integer[ ] |
false |
|
positionInMonth |
integer |
false |
|
daysOfWeek |
string[ ] |
false |
|
YearlyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
daysOfWeek |
string[ ] |
false |
|
daysOfYear |
integer[ ] |
false |
|
numbersOfWeek |
integer[ ] |
false |
|
OddDaysRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
EvenDaysRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
WeeklySameDayRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
WeeklyWorkdaysRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
MonthlySameDateRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
MonthlySameDayRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
AnnuallySameDateRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
HTTP Responses
Code |
Description |
201 |
Created |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
id |
string |
true |
|
controllerId |
string |
true |
|
name |
string |
true |
|
repeatPattern |
RepeatPattern |
false |
|
createdAt |
string |
true |
|
updatedAt |
string |
true |
|
startDateTime |
string |
true |
|
endDateTime |
string |
true |
|
areaRegionTypeIds |
integer[ ] |
false |
|
emitterTypeIds |
integer[ ] |
false |
|
stationNumbers |
integer[ ] |
false |
|
allStations |
boolean |
false |
|
effect |
string |
true |
|
RepeatPattern
Name |
Type |
Required |
Description |
dailyRepeatPattern |
DailyRepeatPattern |
false |
|
weeklyRepeatPattern |
WeeklyRepeatPattern |
false |
|
monthlyRepeatPattern |
MonthlyRepeatPattern |
false |
|
yearlyRepeatPattern |
YearlyRepeatPattern |
false |
|
oddDaysRepeatPattern |
OddDaysRepeatPattern |
false |
|
evenDaysRepeatPattern |
EvenDaysRepeatPattern |
false |
|
weeklySameDayRepeatPattern |
WeeklySameDayRepeatPattern |
false |
|
weeklyWorkdaysRepeatPattern |
WeeklyWorkdaysRepeatPattern |
false |
|
monthlySameDateRepeatPattern |
MonthlySameDateRepeatPattern |
false |
|
monthlySameDayRepeatPattern |
MonthlySameDayRepeatPattern |
false |
|
annuallySameDateRepeatPattern |
AnnuallySameDateRepeatPattern |
false |
|
DailyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
WeeklyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
daysOfWeek |
string[ ] |
false |
|
MonthlyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
daysOfMonth |
integer[ ] |
false |
|
positionInMonth |
integer |
false |
|
daysOfWeek |
string[ ] |
false |
|
YearlyRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
interval |
integer |
false |
|
daysOfWeek |
string[ ] |
false |
|
daysOfYear |
integer[ ] |
false |
|
numbersOfWeek |
integer[ ] |
false |
|
OddDaysRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
EvenDaysRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
WeeklySameDayRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
WeeklyWorkdaysRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
MonthlySameDateRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
MonthlySameDayRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
AnnuallySameDateRepeatPattern
Name |
Type |
Required |
Description |
repeatCount |
integer |
false |
|
applyUntilDateTime |
string |
false |
|
V2 | Sites | Manager Invitation
Invite site manager
Request
curl "https://developer-api.etwater.com/api/v2/sites/{siteId}/managers/rpc/invite" \
-X POST \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
--data "{
\"siteManagerInvitationRequest\": {
\"siteId\": \"example string value\",
\"email\": \"example string value\",
\"firstName\": \"example string value\",
\"lastName\": \"example string value\",
\"address1\": \"example string value\",
\"address2\": \"example string value\",
\"city\": \"example string value\",
\"state\": \"example string value\",
\"postcode\": \"example string value\",
\"phone1\": \"example string value\",
\"phone2\": \"example string value\",
\"accessType\": \"example string value\"
},
\"oAuthClientId\": \"example string value\"
}"
On success, the above request returns response like
HTTP Request
POST /api/v2/sites/{siteId}/managers/rpc/invite
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
siteId |
string |
path |
true |
|
SiteManagerInvitationRequestContainer |
SiteManagerInvitationRequestContainer |
body |
true |
|
SiteManagerInvitationRequestContainer
Name |
Type |
Required |
Description |
siteManagerInvitationRequest |
SiteManagerInvitationRequest |
true |
|
oAuthClientId |
string |
true |
|
SiteManagerInvitationRequest
Name |
Type |
Required |
Description |
siteId |
string |
true |
|
email |
string |
true |
|
firstName |
string |
true |
|
lastName |
string |
true |
|
address1 |
string |
true |
|
address2 |
string |
false |
|
city |
string |
true |
|
state |
string |
true |
|
postcode |
string |
true |
|
phone1 |
string |
true |
|
phone2 |
string |
false |
|
accessType |
string |
true |
|
HTTP Responses
Code |
Description |
201 |
Created |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
userId |
string |
true |
|
firstName |
string |
true |
|
lastName |
string |
true |
|
email |
string |
true |
|
accessType |
string |
true |
|
siteId |
string |
true |
|
V2 | Sites | Site Managers
Find site managers
Request
curl "https://developer-api.etwater.com/api/v2/sites/{siteId}/managers?page=1&size=50" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"managers": [
{
"userId": "example string value",
"firstName": "example string value",
"lastName": "example string value",
"email": "example string value",
"accessType": "example string value",
"siteId": "example string value"
}
],
"page": 979,
"size": 118,
"total": 317
}
HTTP Request
GET /api/v2/sites/{siteId}/managers
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
siteId |
string |
path |
true |
|
page |
integer |
query |
false |
|
size |
integer |
query |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
managers |
SiteManager[ ] |
true |
|
page |
integer |
true |
|
size |
integer |
true |
|
total |
integer |
true |
|
SiteManager
Name |
Type |
Required |
Description |
userId |
string |
true |
|
firstName |
string |
true |
|
lastName |
string |
true |
|
email |
string |
true |
|
accessType |
string |
true |
|
siteId |
string |
true |
|
Get site manager
Request
curl "https://developer-api.etwater.com/api/v2/sites/{siteId}/managers/{userId}" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"userId": "example string value",
"firstName": "example string value",
"lastName": "example string value",
"email": "example string value",
"accessType": "example string value",
"siteId": "example string value"
}
HTTP Request
GET /api/v2/sites/{siteId}/managers/{userId}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
siteId |
string |
path |
true |
|
userId |
string |
path |
true |
|
HTTP Responses
Code |
Description |
200 |
OK |
404 |
Not Found |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
userId |
string |
true |
|
firstName |
string |
true |
|
lastName |
string |
true |
|
email |
string |
true |
|
accessType |
string |
true |
|
siteId |
string |
true |
|
Delete manager for site
Request
curl "https://developer-api.etwater.com/api/v2/sites/{siteId}/managers/{userId}" \
-X DELETE \
-H "Authorization: example string value"
On success, the above request returns response like
HTTP Request
DELETE /api/v2/sites/{siteId}/managers/{userId}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
siteId |
string |
path |
true |
|
userId |
string |
path |
true |
|
HTTP Responses
Code |
Description |
204 |
No Content |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Set managerfor site
Request
curl "https://developer-api.etwater.com/api/v2/sites/{siteId}/managers/{userId}" \
-X PUT \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
--data "{
\"accessType\": \"technician\"
}"
On success, the above request returns response like
HTTP Request
PUT /api/v2/sites/{siteId}/managers/{userId}
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
siteId |
string |
path |
true |
|
userId |
string |
path |
true |
|
SetSiteManagerRequest |
SetSiteManagerRequest |
body |
true |
|
SetSiteManagerRequest
Name |
Type |
Required |
Description |
accessType |
string |
false |
|
HTTP Responses
Code |
Description |
201 |
Created |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
userId |
string |
true |
|
firstName |
string |
true |
|
lastName |
string |
true |
|
email |
string |
true |
|
accessType |
string |
true |
|
siteId |
string |
true |
|
V2 | Sites | Water Usage Report
Get water usage report
Request
curl "https://developer-api.etwater.com/api/v2/sites/{siteId}/water-usage/monthly?startDate=example+string+value&endDate=example+string+value" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"siteId": "example string value",
"groupInterval": "DAILY",
"items": [
{
"realWateringMm": 856,
"effectiveWateringMm": 794.4625005100213,
"rainMm": 123.75728326093278,
"effectiveRainMm": 844.1638983060437,
"etcMm": 956,
"etoMm": 6.732976998543823,
"date": "example string value"
}
],
"processingStatus": "UNPROCESSABLE"
}
HTTP Request
GET /api/v2/sites/{siteId}/water-usage/monthly
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
siteId |
string |
path |
true |
|
startDate |
string |
query |
true |
|
endDate |
string |
query |
true |
|
HTTP Responses
Code |
Description |
200 |
OK |
404 |
Not Found |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
siteId |
string |
true |
|
groupInterval |
string |
true |
|
items |
SiteWaterUsageReportItem[ ] |
false |
|
processingStatus |
string |
true |
|
SiteWaterUsageReportItem
Name |
Type |
Required |
Description |
realWateringMm |
number |
true |
|
effectiveWateringMm |
number |
true |
|
rainMm |
number |
true |
|
effectiveRainMm |
number |
true |
|
etcMm |
number |
true |
|
etoMm |
number |
true |
|
date |
string |
true |
|
Get water usage report
Request
curl "https://developer-api.etwater.com/api/v2/sites/{siteId}/water-usage/daily?startDate=example+string+value&endDate=example+string+value" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"siteId": "example string value",
"groupInterval": "MONTHLY",
"items": [
{
"realWateringMm": 664.3977103123431,
"effectiveWateringMm": 152,
"rainMm": 322,
"effectiveRainMm": 529,
"etcMm": 44,
"etoMm": 724.156170023678,
"date": "example string value"
}
],
"processingStatus": "IN_PROGRESS"
}
HTTP Request
GET /api/v2/sites/{siteId}/water-usage/daily
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
siteId |
string |
path |
true |
|
startDate |
string |
query |
true |
|
endDate |
string |
query |
true |
|
HTTP Responses
Code |
Description |
200 |
OK |
404 |
Not Found |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
siteId |
string |
true |
|
groupInterval |
string |
true |
|
items |
SiteWaterUsageReportItem[ ] |
false |
|
processingStatus |
string |
true |
|
SiteWaterUsageReportItem
Name |
Type |
Required |
Description |
realWateringMm |
number |
true |
|
effectiveWateringMm |
number |
true |
|
rainMm |
number |
true |
|
effectiveRainMm |
number |
true |
|
etcMm |
number |
true |
|
etoMm |
number |
true |
|
date |
string |
true |
|
V2 | Users | Notification Preferences
Get all user notification preferences
Request
curl "https://developer-api.etwater.com/api/v2/users/{userId}/notification-preferences?userEditable=0&userVisible=1&platform%5B0%5D=LEGACY&group%5B0%5D=COMMUNICATION_ISSUES" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
[
{
"userId": "example string value",
"notificationKey": "example string value",
"notificationConfig": {
"name": "example string value",
"description": "example string value",
"level": "SITE",
"platform": "LEGACY",
"userEditable": false,
"group": "HARDWARE_FAILURES",
"userVisible": true
},
"notificationConfigParameters": {
"notifyOnceInPeriod": true,
"notificationTimePeriodHours": 63,
"notifyByEmail": false,
"notifyByText": true
}
}
]
HTTP Request
GET /api/v2/users/{userId}/notification-preferences
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
userId |
string |
path |
true |
|
userEditable |
boolean |
query |
false |
|
userVisible |
boolean |
query |
false |
|
platform |
array |
query |
false |
Comma separated status |
group |
array |
query |
false |
Comma separated status |
HTTP Responses
Code |
Description |
200 |
Successful Response |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response is array of
Name |
Type |
Required |
Description |
userId |
string |
true |
|
notificationKey |
string |
true |
|
notificationConfig |
NotificationConfig |
true |
|
notificationConfigParameters |
NotificationConfigParameters |
true |
|
NotificationConfig
Name |
Type |
Required |
Description |
name |
string |
true |
|
description |
string |
true |
|
level |
string |
true |
|
platform |
string |
true |
|
userEditable |
boolean |
true |
|
group |
string |
true |
|
userVisible |
boolean |
true |
|
NotificationConfigParameters
Name |
Type |
Required |
Description |
notifyOnceInPeriod |
boolean |
true |
|
notificationTimePeriodHours |
integer |
true |
|
notifyByEmail |
boolean |
true |
|
notifyByText |
boolean |
false |
|
Set user notification preferences
Request
curl "https://developer-api.etwater.com/api/v2/users/{userId}/notification-preferences" \
-X PUT \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
--data "[
{
\"userId\": \"example string value\",
\"notificationKey\": \"example string value\",
\"notificationConfigParameters\": {
\"notifyOnceInPeriod\": false,
\"notificationTimePeriodHours\": 364,
\"notifyByEmail\": true,
\"notifyByText\": false
}
}
]"
On success, the above request returns response like
[
{
"userId": "example string value",
"notificationKey": "example string value",
"notificationName": "example string value",
"notificationConfigParameters": {
"notifyOnceInPeriod": false,
"notificationTimePeriodHours": 823,
"notifyByEmail": true,
"notifyByText": true
}
}
]
HTTP Request
PUT /api/v2/users/{userId}/notification-preferences
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
userId |
string |
path |
true |
|
SetUserNotificationConfigRequests |
SetUserNotificationConfigRequest[ ] |
body |
true |
|
SetUserNotificationConfigRequest
Name |
Type |
Required |
Description |
userId |
string |
true |
|
notificationKey |
string |
true |
|
notificationConfigParameters |
NotificationConfigParameters |
true |
|
NotificationConfigParameters
Name |
Type |
Required |
Description |
notifyOnceInPeriod |
boolean |
true |
|
notificationTimePeriodHours |
integer |
true |
|
notifyByEmail |
boolean |
true |
|
notifyByText |
boolean |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response is array of
Name |
Type |
Required |
Description |
userId |
string |
true |
|
notificationKey |
string |
true |
|
notificationName |
string |
true |
|
notificationConfigParameters |
NotificationConfigParameters |
true |
|
NotificationConfigParameters
Name |
Type |
Required |
Description |
notifyOnceInPeriod |
boolean |
true |
|
notificationTimePeriodHours |
integer |
true |
|
notifyByEmail |
boolean |
true |
|
notifyByText |
boolean |
false |
|
Get all user site notification preferences
Request
curl "https://developer-api.etwater.com/api/v2/users/{userId}/site/{siteId}/notification-preferences?userEditable=0&userVisible=1&platform%5B0%5D=UNITY&group%5B0%5D=HARDWARE_FAILURES" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
[
{
"userId": "example string value",
"notificationKey": "example string value",
"notificationConfig": {
"name": "example string value",
"description": "example string value",
"level": "STATION",
"platform": "LEGACY",
"userEditable": true,
"group": "FLOW_REPORTS",
"userVisible": false
},
"siteId": "example string value",
"notificationConfigParameters": {
"notifyOnceInPeriod": false,
"notificationTimePeriodHours": 25,
"notifyByEmail": true,
"notifyByText": true
}
}
]
HTTP Request
GET /api/v2/users/{userId}/site/{siteId}/notification-preferences
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
userId |
string |
path |
true |
|
siteId |
string |
path |
true |
|
userEditable |
boolean |
query |
false |
|
userVisible |
boolean |
query |
false |
|
platform |
array |
query |
false |
Comma separated status |
group |
array |
query |
false |
Comma separated status |
HTTP Responses
Code |
Description |
200 |
Successful Response |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response is array of
Name |
Type |
Required |
Description |
userId |
string |
true |
|
notificationKey |
string |
true |
|
notificationConfig |
NotificationConfig |
true |
|
siteId |
string |
true |
|
notificationConfigParameters |
NotificationConfigParameters |
true |
|
NotificationConfig
Name |
Type |
Required |
Description |
name |
string |
true |
|
description |
string |
true |
|
level |
string |
true |
|
platform |
string |
true |
|
userEditable |
boolean |
true |
|
group |
string |
true |
|
userVisible |
boolean |
true |
|
NotificationConfigParameters
Name |
Type |
Required |
Description |
notifyOnceInPeriod |
boolean |
true |
|
notificationTimePeriodHours |
integer |
true |
|
notifyByEmail |
boolean |
true |
|
notifyByText |
boolean |
false |
|
Set user site notification preferences
Request
curl "https://developer-api.etwater.com/api/v2/users/{userId}/site/{siteId}/notification-preferences" \
-X PUT \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
--data "[
{
\"userId\": \"example string value\",
\"notificationKey\": \"example string value\",
\"siteId\": \"example string value\",
\"notificationConfigParameters\": {
\"notifyOnceInPeriod\": false,
\"notificationTimePeriodHours\": 589,
\"notifyByEmail\": false,
\"notifyByText\": true
}
}
]"
On success, the above request returns response like
[
{
"userId": "example string value",
"notificationKey": "example string value",
"notificationName": "example string value",
"siteId": "example string value",
"notificationConfigParameters": {
"notifyOnceInPeriod": true,
"notificationTimePeriodHours": 76,
"notifyByEmail": false,
"notifyByText": false
}
}
]
HTTP Request
PUT /api/v2/users/{userId}/site/{siteId}/notification-preferences
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
userId |
string |
path |
true |
|
siteId |
string |
path |
true |
|
SetUserSiteNotificationConfigRequests |
SetUserSiteNotificationConfigRequest[ ] |
body |
true |
|
SetUserSiteNotificationConfigRequest
Name |
Type |
Required |
Description |
userId |
string |
true |
|
notificationKey |
string |
true |
|
siteId |
string |
true |
|
notificationConfigParameters |
NotificationConfigParameters |
true |
|
NotificationConfigParameters
Name |
Type |
Required |
Description |
notifyOnceInPeriod |
boolean |
true |
|
notificationTimePeriodHours |
integer |
true |
|
notifyByEmail |
boolean |
true |
|
notifyByText |
boolean |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response is array of
Name |
Type |
Required |
Description |
userId |
string |
true |
|
notificationKey |
string |
true |
|
notificationName |
string |
true |
|
siteId |
string |
true |
|
notificationConfigParameters |
NotificationConfigParameters |
true |
|
NotificationConfigParameters
Name |
Type |
Required |
Description |
notifyOnceInPeriod |
boolean |
true |
|
notificationTimePeriodHours |
integer |
true |
|
notifyByEmail |
boolean |
true |
|
notifyByText |
boolean |
false |
|
Get all user controller notification preferences
Request
curl "https://developer-api.etwater.com/api/v2/users/{userId}/controller/{controllerId}/notification-preferences?userEditable=0&userVisible=1&platform%5B0%5D=LEGACY&group%5B0%5D=FLOW_REPORTS" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
[
{
"userId": "example string value",
"notificationKey": "example string value",
"notificationConfig": {
"name": "example string value",
"description": "example string value",
"level": "USER",
"platform": "UNITY",
"userEditable": true,
"group": "HARDWARE_FAILURES",
"userVisible": true
},
"controllerId": "example string value",
"notificationConfigParameters": {
"notifyOnceInPeriod": true,
"notificationTimePeriodHours": 832,
"notifyByEmail": false,
"notifyByText": true
}
}
]
HTTP Request
GET /api/v2/users/{userId}/controller/{controllerId}/notification-preferences
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
userId |
string |
path |
true |
|
controllerId |
string |
path |
true |
|
userEditable |
boolean |
query |
false |
|
userVisible |
boolean |
query |
false |
|
platform |
array |
query |
false |
Comma separated status |
group |
array |
query |
false |
Comma separated status |
HTTP Responses
Code |
Description |
200 |
Successful Response |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response is array of
Name |
Type |
Required |
Description |
userId |
string |
true |
|
notificationKey |
string |
true |
|
notificationConfig |
NotificationConfig |
true |
|
controllerId |
string |
true |
|
notificationConfigParameters |
NotificationConfigParameters |
true |
|
NotificationConfig
Name |
Type |
Required |
Description |
name |
string |
true |
|
description |
string |
true |
|
level |
string |
true |
|
platform |
string |
true |
|
userEditable |
boolean |
true |
|
group |
string |
true |
|
userVisible |
boolean |
true |
|
NotificationConfigParameters
Name |
Type |
Required |
Description |
notifyOnceInPeriod |
boolean |
true |
|
notificationTimePeriodHours |
integer |
true |
|
notifyByEmail |
boolean |
true |
|
notifyByText |
boolean |
false |
|
Set user controller notification preferences
Request
curl "https://developer-api.etwater.com/api/v2/users/{userId}/controller/{controllerId}/notification-preferences" \
-X PUT \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
--data "[
{
\"userId\": \"example string value\",
\"notificationKey\": \"example string value\",
\"controllerId\": \"example string value\",
\"notificationConfigParameters\": {
\"notifyOnceInPeriod\": false,
\"notificationTimePeriodHours\": 83,
\"notifyByEmail\": false,
\"notifyByText\": true
}
}
]"
On success, the above request returns response like
[
{
"userId": "example string value",
"notificationKey": "example string value",
"notificationName": "example string value",
"controllerId": "example string value",
"notificationConfigParameters": {
"notifyOnceInPeriod": false,
"notificationTimePeriodHours": 468,
"notifyByEmail": true,
"notifyByText": true
}
}
]
HTTP Request
PUT /api/v2/users/{userId}/controller/{controllerId}/notification-preferences
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
userId |
string |
path |
true |
|
controllerId |
string |
path |
true |
|
SetUserControllerNotificationConfigRequests |
SetUserControllerNotificationConfigRequest[ ] |
body |
true |
|
SetUserControllerNotificationConfigRequest
Name |
Type |
Required |
Description |
userId |
string |
true |
|
notificationKey |
string |
true |
|
controllerId |
string |
true |
|
notificationConfigParameters |
NotificationConfigParameters |
true |
|
NotificationConfigParameters
Name |
Type |
Required |
Description |
notifyOnceInPeriod |
boolean |
true |
|
notificationTimePeriodHours |
integer |
true |
|
notifyByEmail |
boolean |
true |
|
notifyByText |
boolean |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response is array of
Name |
Type |
Required |
Description |
userId |
string |
true |
|
notificationKey |
string |
true |
|
notificationName |
string |
true |
|
controllerId |
string |
true |
|
notificationConfigParameters |
NotificationConfigParameters |
true |
|
NotificationConfigParameters
Name |
Type |
Required |
Description |
notifyOnceInPeriod |
boolean |
true |
|
notificationTimePeriodHours |
integer |
true |
|
notifyByEmail |
boolean |
true |
|
notifyByText |
boolean |
false |
|
Find user site notification preferences in bulk
Request
curl "https://developer-api.etwater.com/api/v2/users/{userId}/site-notification-preferences/rpc/find-bulk" \
-X POST \
-H "Authorization: example string value" \
-H "Content-Type: example string value" \
--data "[
{
\"userId\": \"example string value\",
\"siteId\": \"example string value\",
\"notificationConfigFilters\": {
\"platforms\": [
\"UNITY\"
],
\"userEditable\": false,
\"groups\": [
\"COMMUNICATION_ISSUES\"
],
\"userVisible\": true
}
}
]"
On success, the above request returns response like
[
{
"userId": "example string value",
"notificationKey": "example string value",
"notificationConfig": {
"name": "example string value",
"description": "example string value",
"level": "USER",
"platform": "LEGACY",
"userEditable": false,
"group": "SCHEDULING_ISSUES",
"userVisible": false
},
"siteId": "example string value",
"notificationConfigParameters": {
"notifyOnceInPeriod": true,
"notificationTimePeriodHours": 184,
"notifyByEmail": true,
"notifyByText": false
}
}
]
HTTP Request
POST /api/v2/users/{userId}/site-notification-preferences/rpc/find-bulk
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
Content-Type |
string |
header |
true |
|
userId |
string |
path |
true |
|
FindUserSiteNotificationConfigsRequest |
FindUserSiteNotificationConfigsRequest[ ] |
body |
true |
|
FindUserSiteNotificationConfigsRequest
Name |
Type |
Required |
Description |
userId |
string |
true |
|
siteId |
string |
true |
|
notificationConfigFilters |
NotificationConfigFilters |
true |
|
NotificationConfigFilters
Name |
Type |
Required |
Description |
platforms |
string[ ] |
false |
|
userEditable |
boolean |
false |
|
groups |
string[ ] |
false |
|
userVisible |
boolean |
false |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response is array of
Name |
Type |
Required |
Description |
userId |
string |
true |
|
notificationKey |
string |
true |
|
notificationConfig |
NotificationConfig |
true |
|
siteId |
string |
true |
|
notificationConfigParameters |
NotificationConfigParameters |
true |
|
NotificationConfig
Name |
Type |
Required |
Description |
name |
string |
true |
|
description |
string |
true |
|
level |
string |
true |
|
platform |
string |
true |
|
userEditable |
boolean |
true |
|
group |
string |
true |
|
userVisible |
boolean |
true |
|
NotificationConfigParameters
Name |
Type |
Required |
Description |
notifyOnceInPeriod |
boolean |
true |
|
notificationTimePeriodHours |
integer |
true |
|
notifyByEmail |
boolean |
true |
|
notifyByText |
boolean |
false |
|
V2 | Weather | Weather
Get daily atmosphere data
Request
curl "https://developer-api.etwater.com/api/v2/atmosphere/daily?longitude=737.82375768657&latitude=343&startTimestamp=720&endTimestamp=341&keys%5B0%5D=ET" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"cluster": {
"id": "example string value",
"location": {
"type": "example string value",
"coordinates": [
680
]
}
},
"hourly": [
{
"time": 639,
"etMm": 850,
"rainMm": 839.2386770989925,
"ghWm2": 374,
"temperatureC": 892,
"dewPointC": 761.2883452145794,
"windKmH": 675.5186173485213,
"relativeHumidityPercent": 208,
"precipitationProbabilityPercent": 230.5510236092615,
"cloudCoverPercent": 294.5086221650748,
"pressureKilopascals": 385,
"windGustKmH": 242.05380922279033
}
],
"daily": [
{
"time": 408,
"sunrise": 345,
"sunset": 594,
"etMm": 530,
"rainMm": 262,
"ghWm2": 93,
"dewPointC": 366.6390014657001,
"minTemperatureC": 781.4435715700703,
"maxTemperatureC": 697.8156798043361,
"minWindKmH": 534,
"maxWindKmH": 165,
"avgPressureKilopascals": 891,
"avgCloudCoverPercent": 14,
"avgRelativeHumidityPercent": 396.9258802928617,
"maxPrecipitationProbabilityPercent": 606,
"avgTemperatureC": 655,
"minRelativeHumidityPercent": 230.6712322079908,
"maxRelativeHumidityPercent": 532
}
],
"monthly": [
{
"time": 176,
"etMm": 868,
"rainMm": 792,
"ghWm2": 966,
"minTemperatureC": 372.03222111427795,
"maxTemperatureC": 604.9690333218169,
"minWindKmH": 862.7497972281416,
"maxWindKmH": 954,
"avgPressureKilopascals": 292,
"avgCloudCoverPercent": 466.9471413208857,
"avgRelativeHumidityPercent": 256.76381599938674,
"maxPrecipitationProbabilityPercent": 364.3972344530734,
"avgWindKmH": 516,
"avgTemperatureC": 74,
"avgDewPointC": 360,
"minRelativeHumidityPercent": 860,
"maxRelativeHumidityPercent": 658
}
],
"status": 241
}
HTTP Request
GET /api/v2/atmosphere/daily
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
longitude |
number |
query |
false |
|
latitude |
number |
query |
false |
|
startTimestamp |
integer |
query |
false |
|
endTimestamp |
integer |
query |
false |
|
keys |
array |
query |
false |
names of sections to show |
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
cluster |
WeatherCluster |
false |
|
hourly |
WeatherHourly[ ] |
false |
|
daily |
WeatherDaily[ ] |
false |
|
monthly |
WeatherMonthly[ ] |
false |
|
status |
integer |
false |
|
WeatherCluster
Name |
Type |
Required |
Description |
id |
string |
false |
|
location |
Location |
false |
|
Location
Name |
Type |
Required |
Description |
type |
string |
false |
|
coordinates |
number[ ] |
false |
|
WeatherHourly
Name |
Type |
Required |
Description |
time |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
temperatureC |
number |
false |
|
dewPointC |
number |
false |
|
windKmH |
number |
false |
|
relativeHumidityPercent |
number |
false |
|
precipitationProbabilityPercent |
number |
false |
|
cloudCoverPercent |
number |
false |
|
pressureKilopascals |
number |
false |
|
windGustKmH |
number |
false |
|
WeatherDaily
Name |
Type |
Required |
Description |
time |
integer |
false |
|
sunrise |
integer |
false |
|
sunset |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
dewPointC |
number |
false |
|
minTemperatureC |
number |
false |
|
maxTemperatureC |
number |
false |
|
minWindKmH |
number |
false |
|
maxWindKmH |
number |
false |
|
avgPressureKilopascals |
number |
false |
|
avgCloudCoverPercent |
number |
false |
|
avgRelativeHumidityPercent |
number |
false |
|
maxPrecipitationProbabilityPercent |
number |
false |
|
avgTemperatureC |
number |
false |
|
minRelativeHumidityPercent |
number |
false |
|
maxRelativeHumidityPercent |
number |
false |
|
WeatherMonthly
Name |
Type |
Required |
Description |
time |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
minTemperatureC |
number |
false |
|
maxTemperatureC |
number |
false |
|
minWindKmH |
number |
false |
|
maxWindKmH |
number |
false |
|
avgPressureKilopascals |
number |
false |
|
avgCloudCoverPercent |
number |
false |
|
avgRelativeHumidityPercent |
number |
false |
|
maxPrecipitationProbabilityPercent |
number |
false |
|
avgWindKmH |
number |
false |
|
avgTemperatureC |
number |
false |
|
avgDewPointC |
number |
false |
|
minRelativeHumidityPercent |
number |
false |
|
maxRelativeHumidityPercent |
number |
false |
|
Get hourly atmosphere data
Request
curl "https://developer-api.etwater.com/api/v2/atmosphere/hourly?longitude=386.03048277368&latitude=765&startTimestamp=401&endTimestamp=688&keys%5B0%5D=ET" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"cluster": {
"id": "example string value",
"location": {
"type": "example string value",
"coordinates": [
256
]
}
},
"hourly": [
{
"time": 680,
"etMm": 409.47744595328226,
"rainMm": 262,
"ghWm2": 679,
"temperatureC": 725.1848563203517,
"dewPointC": 753,
"windKmH": 676,
"relativeHumidityPercent": 483,
"precipitationProbabilityPercent": 369.98454777988815,
"cloudCoverPercent": 607,
"pressureKilopascals": 213.2644677596467,
"windGustKmH": 355.25161696376824
}
],
"daily": [
{
"time": 857,
"sunrise": 319,
"sunset": 640,
"etMm": 509,
"rainMm": 997,
"ghWm2": 152,
"dewPointC": 838.2934228695432,
"minTemperatureC": 695,
"maxTemperatureC": 995.8437932635862,
"minWindKmH": 157.06242674824895,
"maxWindKmH": 416,
"avgPressureKilopascals": 721.2910008250228,
"avgCloudCoverPercent": 831,
"avgRelativeHumidityPercent": 348.58810219382315,
"maxPrecipitationProbabilityPercent": 479.8733710683293,
"avgTemperatureC": 737.1433944148679,
"minRelativeHumidityPercent": 483.9289544541058,
"maxRelativeHumidityPercent": 738
}
],
"monthly": [
{
"time": 255,
"etMm": 400,
"rainMm": 39.563068672811184,
"ghWm2": 522,
"minTemperatureC": 980.4449030107096,
"maxTemperatureC": 785.0108718429742,
"minWindKmH": 348.87738216150433,
"maxWindKmH": 569.4177088185296,
"avgPressureKilopascals": 164.612240234675,
"avgCloudCoverPercent": 8,
"avgRelativeHumidityPercent": 93,
"maxPrecipitationProbabilityPercent": 992.3519478143901,
"avgWindKmH": 998,
"avgTemperatureC": 7,
"avgDewPointC": 391,
"minRelativeHumidityPercent": 677.3542303952175,
"maxRelativeHumidityPercent": 131.24604994954822
}
],
"status": 731
}
HTTP Request
GET /api/v2/atmosphere/hourly
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
longitude |
number |
query |
false |
|
latitude |
number |
query |
false |
|
startTimestamp |
integer |
query |
false |
|
endTimestamp |
integer |
query |
false |
|
keys |
array |
query |
false |
names of sections to show |
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
cluster |
WeatherCluster |
false |
|
hourly |
WeatherHourly[ ] |
false |
|
daily |
WeatherDaily[ ] |
false |
|
monthly |
WeatherMonthly[ ] |
false |
|
status |
integer |
false |
|
WeatherCluster
Name |
Type |
Required |
Description |
id |
string |
false |
|
location |
Location |
false |
|
Location
Name |
Type |
Required |
Description |
type |
string |
false |
|
coordinates |
number[ ] |
false |
|
WeatherHourly
Name |
Type |
Required |
Description |
time |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
temperatureC |
number |
false |
|
dewPointC |
number |
false |
|
windKmH |
number |
false |
|
relativeHumidityPercent |
number |
false |
|
precipitationProbabilityPercent |
number |
false |
|
cloudCoverPercent |
number |
false |
|
pressureKilopascals |
number |
false |
|
windGustKmH |
number |
false |
|
WeatherDaily
Name |
Type |
Required |
Description |
time |
integer |
false |
|
sunrise |
integer |
false |
|
sunset |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
dewPointC |
number |
false |
|
minTemperatureC |
number |
false |
|
maxTemperatureC |
number |
false |
|
minWindKmH |
number |
false |
|
maxWindKmH |
number |
false |
|
avgPressureKilopascals |
number |
false |
|
avgCloudCoverPercent |
number |
false |
|
avgRelativeHumidityPercent |
number |
false |
|
maxPrecipitationProbabilityPercent |
number |
false |
|
avgTemperatureC |
number |
false |
|
minRelativeHumidityPercent |
number |
false |
|
maxRelativeHumidityPercent |
number |
false |
|
WeatherMonthly
Name |
Type |
Required |
Description |
time |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
minTemperatureC |
number |
false |
|
maxTemperatureC |
number |
false |
|
minWindKmH |
number |
false |
|
maxWindKmH |
number |
false |
|
avgPressureKilopascals |
number |
false |
|
avgCloudCoverPercent |
number |
false |
|
avgRelativeHumidityPercent |
number |
false |
|
maxPrecipitationProbabilityPercent |
number |
false |
|
avgWindKmH |
number |
false |
|
avgTemperatureC |
number |
false |
|
avgDewPointC |
number |
false |
|
minRelativeHumidityPercent |
number |
false |
|
maxRelativeHumidityPercent |
number |
false |
|
Get monthly atmosphere data
Request
curl "https://developer-api.etwater.com/api/v2/atmosphere/monthly?longitude=50&latitude=705.29046035618&startTimestamp=955&endTimestamp=44&keys%5B0%5D=TEMPERATURE" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"cluster": {
"id": "example string value",
"location": {
"type": "example string value",
"coordinates": [
113
]
}
},
"hourly": [
{
"time": 670,
"etMm": 136.09639282156547,
"rainMm": 810,
"ghWm2": 479,
"temperatureC": 36.6992559454866,
"dewPointC": 532,
"windKmH": 890,
"relativeHumidityPercent": 513,
"precipitationProbabilityPercent": 63.833156630319145,
"cloudCoverPercent": 664.5737186374952,
"pressureKilopascals": 947.5200990901888,
"windGustKmH": 681.9220849694321
}
],
"daily": [
{
"time": 996,
"sunrise": 722,
"sunset": 34,
"etMm": 163.16984182371286,
"rainMm": 190.20233312165473,
"ghWm2": 420,
"dewPointC": 807.4622563121198,
"minTemperatureC": 42,
"maxTemperatureC": 172,
"minWindKmH": 272.95154764920085,
"maxWindKmH": 808.2432741337657,
"avgPressureKilopascals": 123.18258319198274,
"avgCloudCoverPercent": 532,
"avgRelativeHumidityPercent": 916,
"maxPrecipitationProbabilityPercent": 944,
"avgTemperatureC": 919,
"minRelativeHumidityPercent": 755,
"maxRelativeHumidityPercent": 320.38974730316073
}
],
"monthly": [
{
"time": 354,
"etMm": 390,
"rainMm": 282,
"ghWm2": 504,
"minTemperatureC": 799.3886483830347,
"maxTemperatureC": 914.1351226317395,
"minWindKmH": 246.81016115788842,
"maxWindKmH": 832.245587293173,
"avgPressureKilopascals": 645.773984792537,
"avgCloudCoverPercent": 744,
"avgRelativeHumidityPercent": 547,
"maxPrecipitationProbabilityPercent": 126.06581492631966,
"avgWindKmH": 47,
"avgTemperatureC": 291,
"avgDewPointC": 212,
"minRelativeHumidityPercent": 545.8222532392583,
"maxRelativeHumidityPercent": 823.9363775700035
}
],
"status": 930
}
HTTP Request
GET /api/v2/atmosphere/monthly
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
longitude |
number |
query |
false |
|
latitude |
number |
query |
false |
|
startTimestamp |
integer |
query |
false |
|
endTimestamp |
integer |
query |
false |
|
keys |
array |
query |
false |
names of sections to show |
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
cluster |
WeatherCluster |
false |
|
hourly |
WeatherHourly[ ] |
false |
|
daily |
WeatherDaily[ ] |
false |
|
monthly |
WeatherMonthly[ ] |
false |
|
status |
integer |
false |
|
WeatherCluster
Name |
Type |
Required |
Description |
id |
string |
false |
|
location |
Location |
false |
|
Location
Name |
Type |
Required |
Description |
type |
string |
false |
|
coordinates |
number[ ] |
false |
|
WeatherHourly
Name |
Type |
Required |
Description |
time |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
temperatureC |
number |
false |
|
dewPointC |
number |
false |
|
windKmH |
number |
false |
|
relativeHumidityPercent |
number |
false |
|
precipitationProbabilityPercent |
number |
false |
|
cloudCoverPercent |
number |
false |
|
pressureKilopascals |
number |
false |
|
windGustKmH |
number |
false |
|
WeatherDaily
Name |
Type |
Required |
Description |
time |
integer |
false |
|
sunrise |
integer |
false |
|
sunset |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
dewPointC |
number |
false |
|
minTemperatureC |
number |
false |
|
maxTemperatureC |
number |
false |
|
minWindKmH |
number |
false |
|
maxWindKmH |
number |
false |
|
avgPressureKilopascals |
number |
false |
|
avgCloudCoverPercent |
number |
false |
|
avgRelativeHumidityPercent |
number |
false |
|
maxPrecipitationProbabilityPercent |
number |
false |
|
avgTemperatureC |
number |
false |
|
minRelativeHumidityPercent |
number |
false |
|
maxRelativeHumidityPercent |
number |
false |
|
WeatherMonthly
Name |
Type |
Required |
Description |
time |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
minTemperatureC |
number |
false |
|
maxTemperatureC |
number |
false |
|
minWindKmH |
number |
false |
|
maxWindKmH |
number |
false |
|
avgPressureKilopascals |
number |
false |
|
avgCloudCoverPercent |
number |
false |
|
avgRelativeHumidityPercent |
number |
false |
|
maxPrecipitationProbabilityPercent |
number |
false |
|
avgWindKmH |
number |
false |
|
avgTemperatureC |
number |
false |
|
avgDewPointC |
number |
false |
|
minRelativeHumidityPercent |
number |
false |
|
maxRelativeHumidityPercent |
number |
false |
|
Get daily atmosphere data
Request
curl "https://developer-api.etwater.com/api/v2/atmosphere/cluster/{clusterId}/daily?startTimestamp=732&endTimestamp=434&keys%5B0%5D=PRESSURE" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"cluster": {
"id": "example string value",
"location": {
"type": "example string value",
"coordinates": [
489
]
}
},
"hourly": [
{
"time": 55,
"etMm": 789.725315659179,
"rainMm": 75,
"ghWm2": 411,
"temperatureC": 511.22218487375517,
"dewPointC": 695.0080900895447,
"windKmH": 722,
"relativeHumidityPercent": 252,
"precipitationProbabilityPercent": 454,
"cloudCoverPercent": 640.0373813882644,
"pressureKilopascals": 580.6355511679479,
"windGustKmH": 892.87190087739
}
],
"daily": [
{
"time": 276,
"sunrise": 174,
"sunset": 584,
"etMm": 784.0421319864887,
"rainMm": 386,
"ghWm2": 657,
"dewPointC": 168,
"minTemperatureC": 667,
"maxTemperatureC": 39,
"minWindKmH": 143,
"maxWindKmH": 41.40265381028999,
"avgPressureKilopascals": 317,
"avgCloudCoverPercent": 980.9939162717172,
"avgRelativeHumidityPercent": 976.7717290561515,
"maxPrecipitationProbabilityPercent": 837.3978118586343,
"avgTemperatureC": 546.6833773752131,
"minRelativeHumidityPercent": 894,
"maxRelativeHumidityPercent": 613.5624864201818
}
],
"monthly": [
{
"time": 192,
"etMm": 513.9582983748793,
"rainMm": 877,
"ghWm2": 801,
"minTemperatureC": 817.2553273929541,
"maxTemperatureC": 562,
"minWindKmH": 541.660629930748,
"maxWindKmH": 998,
"avgPressureKilopascals": 324,
"avgCloudCoverPercent": 375.7437716125249,
"avgRelativeHumidityPercent": 58.97571801160262,
"maxPrecipitationProbabilityPercent": 177.37546245445284,
"avgWindKmH": 562.9395183934548,
"avgTemperatureC": 739.7106935920709,
"avgDewPointC": 239,
"minRelativeHumidityPercent": 912.1837312878034,
"maxRelativeHumidityPercent": 84.43424761501804
}
],
"status": 744
}
HTTP Request
GET /api/v2/atmosphere/cluster/{clusterId}/daily
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
startTimestamp |
integer |
query |
false |
|
endTimestamp |
integer |
query |
false |
|
keys |
array |
query |
false |
names of sections to show |
clusterId |
string |
path |
true |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
cluster |
WeatherCluster |
false |
|
hourly |
WeatherHourly[ ] |
false |
|
daily |
WeatherDaily[ ] |
false |
|
monthly |
WeatherMonthly[ ] |
false |
|
status |
integer |
false |
|
WeatherCluster
Name |
Type |
Required |
Description |
id |
string |
false |
|
location |
Location |
false |
|
Location
Name |
Type |
Required |
Description |
type |
string |
false |
|
coordinates |
number[ ] |
false |
|
WeatherHourly
Name |
Type |
Required |
Description |
time |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
temperatureC |
number |
false |
|
dewPointC |
number |
false |
|
windKmH |
number |
false |
|
relativeHumidityPercent |
number |
false |
|
precipitationProbabilityPercent |
number |
false |
|
cloudCoverPercent |
number |
false |
|
pressureKilopascals |
number |
false |
|
windGustKmH |
number |
false |
|
WeatherDaily
Name |
Type |
Required |
Description |
time |
integer |
false |
|
sunrise |
integer |
false |
|
sunset |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
dewPointC |
number |
false |
|
minTemperatureC |
number |
false |
|
maxTemperatureC |
number |
false |
|
minWindKmH |
number |
false |
|
maxWindKmH |
number |
false |
|
avgPressureKilopascals |
number |
false |
|
avgCloudCoverPercent |
number |
false |
|
avgRelativeHumidityPercent |
number |
false |
|
maxPrecipitationProbabilityPercent |
number |
false |
|
avgTemperatureC |
number |
false |
|
minRelativeHumidityPercent |
number |
false |
|
maxRelativeHumidityPercent |
number |
false |
|
WeatherMonthly
Name |
Type |
Required |
Description |
time |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
minTemperatureC |
number |
false |
|
maxTemperatureC |
number |
false |
|
minWindKmH |
number |
false |
|
maxWindKmH |
number |
false |
|
avgPressureKilopascals |
number |
false |
|
avgCloudCoverPercent |
number |
false |
|
avgRelativeHumidityPercent |
number |
false |
|
maxPrecipitationProbabilityPercent |
number |
false |
|
avgWindKmH |
number |
false |
|
avgTemperatureC |
number |
false |
|
avgDewPointC |
number |
false |
|
minRelativeHumidityPercent |
number |
false |
|
maxRelativeHumidityPercent |
number |
false |
|
Get hourly atmosphere data
Request
curl "https://developer-api.etwater.com/api/v2/atmosphere/cluster/{clusterId}/hourly?startTimestamp=338&endTimestamp=787&keys%5B0%5D=SOLAR_IRRADIATION" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"cluster": {
"id": "example string value",
"location": {
"type": "example string value",
"coordinates": [
932
]
}
},
"hourly": [
{
"time": 91,
"etMm": 751.1886482831038,
"rainMm": 438.32958742898404,
"ghWm2": 142,
"temperatureC": 94,
"dewPointC": 216.8083126734981,
"windKmH": 280.17634632074106,
"relativeHumidityPercent": 345.317604181039,
"precipitationProbabilityPercent": 368,
"cloudCoverPercent": 297,
"pressureKilopascals": 481.3838477625436,
"windGustKmH": 393
}
],
"daily": [
{
"time": 839,
"sunrise": 431,
"sunset": 844,
"etMm": 615.7145642748636,
"rainMm": 205,
"ghWm2": 787,
"dewPointC": 728,
"minTemperatureC": 904,
"maxTemperatureC": 102.14799507621117,
"minWindKmH": 168.10066027944006,
"maxWindKmH": 438,
"avgPressureKilopascals": 606,
"avgCloudCoverPercent": 638,
"avgRelativeHumidityPercent": 906.4187379118142,
"maxPrecipitationProbabilityPercent": 160.09296065200724,
"avgTemperatureC": 465,
"minRelativeHumidityPercent": 144.1554274149963,
"maxRelativeHumidityPercent": 579
}
],
"monthly": [
{
"time": 910,
"etMm": 643.26255519095,
"rainMm": 726.2069926253553,
"ghWm2": 237,
"minTemperatureC": 308,
"maxTemperatureC": 532,
"minWindKmH": 325.7815923196178,
"maxWindKmH": 779.3203949831988,
"avgPressureKilopascals": 647.0030931043453,
"avgCloudCoverPercent": 24.923392583114744,
"avgRelativeHumidityPercent": 196,
"maxPrecipitationProbabilityPercent": 620.4486678449663,
"avgWindKmH": 536.9290935513234,
"avgTemperatureC": 472.6534138771954,
"avgDewPointC": 960.7011461447464,
"minRelativeHumidityPercent": 476,
"maxRelativeHumidityPercent": 492.8388840019884
}
],
"status": 681
}
HTTP Request
GET /api/v2/atmosphere/cluster/{clusterId}/hourly
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
startTimestamp |
integer |
query |
false |
|
endTimestamp |
integer |
query |
false |
|
keys |
array |
query |
false |
names of sections to show |
clusterId |
string |
path |
true |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
cluster |
WeatherCluster |
false |
|
hourly |
WeatherHourly[ ] |
false |
|
daily |
WeatherDaily[ ] |
false |
|
monthly |
WeatherMonthly[ ] |
false |
|
status |
integer |
false |
|
WeatherCluster
Name |
Type |
Required |
Description |
id |
string |
false |
|
location |
Location |
false |
|
Location
Name |
Type |
Required |
Description |
type |
string |
false |
|
coordinates |
number[ ] |
false |
|
WeatherHourly
Name |
Type |
Required |
Description |
time |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
temperatureC |
number |
false |
|
dewPointC |
number |
false |
|
windKmH |
number |
false |
|
relativeHumidityPercent |
number |
false |
|
precipitationProbabilityPercent |
number |
false |
|
cloudCoverPercent |
number |
false |
|
pressureKilopascals |
number |
false |
|
windGustKmH |
number |
false |
|
WeatherDaily
Name |
Type |
Required |
Description |
time |
integer |
false |
|
sunrise |
integer |
false |
|
sunset |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
dewPointC |
number |
false |
|
minTemperatureC |
number |
false |
|
maxTemperatureC |
number |
false |
|
minWindKmH |
number |
false |
|
maxWindKmH |
number |
false |
|
avgPressureKilopascals |
number |
false |
|
avgCloudCoverPercent |
number |
false |
|
avgRelativeHumidityPercent |
number |
false |
|
maxPrecipitationProbabilityPercent |
number |
false |
|
avgTemperatureC |
number |
false |
|
minRelativeHumidityPercent |
number |
false |
|
maxRelativeHumidityPercent |
number |
false |
|
WeatherMonthly
Name |
Type |
Required |
Description |
time |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
minTemperatureC |
number |
false |
|
maxTemperatureC |
number |
false |
|
minWindKmH |
number |
false |
|
maxWindKmH |
number |
false |
|
avgPressureKilopascals |
number |
false |
|
avgCloudCoverPercent |
number |
false |
|
avgRelativeHumidityPercent |
number |
false |
|
maxPrecipitationProbabilityPercent |
number |
false |
|
avgWindKmH |
number |
false |
|
avgTemperatureC |
number |
false |
|
avgDewPointC |
number |
false |
|
minRelativeHumidityPercent |
number |
false |
|
maxRelativeHumidityPercent |
number |
false |
|
Get monthly atmosphere data
Request
curl "https://developer-api.etwater.com/api/v2/atmosphere/cluster/{clusterId}/monthly?startTimestamp=564&endTimestamp=829&keys%5B0%5D=SOLAR_IRRADIATION" \
-X GET \
-H "Authorization: example string value"
On success, the above request returns response like
{
"cluster": {
"id": "example string value",
"location": {
"type": "example string value",
"coordinates": [
894
]
}
},
"hourly": [
{
"time": 846,
"etMm": 35,
"rainMm": 674.4925969627186,
"ghWm2": 415,
"temperatureC": 47,
"dewPointC": 430.4212222017447,
"windKmH": 786,
"relativeHumidityPercent": 645.5516450319215,
"precipitationProbabilityPercent": 599.6133804319489,
"cloudCoverPercent": 810.970660211039,
"pressureKilopascals": 233.9572944836492,
"windGustKmH": 613
}
],
"daily": [
{
"time": 217,
"sunrise": 565,
"sunset": 16,
"etMm": 293,
"rainMm": 396,
"ghWm2": 386,
"dewPointC": 269,
"minTemperatureC": 160,
"maxTemperatureC": 913,
"minWindKmH": 871,
"maxWindKmH": 78.03296673951343,
"avgPressureKilopascals": 366.1322362563257,
"avgCloudCoverPercent": 508.15353333398394,
"avgRelativeHumidityPercent": 435.62505088542827,
"maxPrecipitationProbabilityPercent": 585,
"avgTemperatureC": 181.31053176769547,
"minRelativeHumidityPercent": 763.2174714297138,
"maxRelativeHumidityPercent": 708
}
],
"monthly": [
{
"time": 987,
"etMm": 706.2401262606681,
"rainMm": 480.60265252394726,
"ghWm2": 185,
"minTemperatureC": 654,
"maxTemperatureC": 289.78360830330456,
"minWindKmH": 868.5215045085743,
"maxWindKmH": 763,
"avgPressureKilopascals": 608,
"avgCloudCoverPercent": 880,
"avgRelativeHumidityPercent": 159,
"maxPrecipitationProbabilityPercent": 348,
"avgWindKmH": 593.2254291107997,
"avgTemperatureC": 347,
"avgDewPointC": 638.9832192282114,
"minRelativeHumidityPercent": 685.9681479101853,
"maxRelativeHumidityPercent": 728
}
],
"status": 852
}
HTTP Request
GET /api/v2/atmosphere/cluster/{clusterId}/monthly
Request parameters
Name |
Type |
Part |
Required |
Description |
Authorization |
string |
header |
true |
|
startTimestamp |
integer |
query |
false |
|
endTimestamp |
integer |
query |
false |
|
keys |
array |
query |
false |
names of sections to show |
clusterId |
string |
path |
true |
|
HTTP Responses
Code |
Description |
200 |
OK |
400 |
Bad Request |
401 |
Unauthorized |
403 |
Forbidden |
500 |
Internal Server Error |
504 |
Gateway Timeout |
Successful response
Name |
Type |
Required |
Description |
cluster |
WeatherCluster |
false |
|
hourly |
WeatherHourly[ ] |
false |
|
daily |
WeatherDaily[ ] |
false |
|
monthly |
WeatherMonthly[ ] |
false |
|
status |
integer |
false |
|
WeatherCluster
Name |
Type |
Required |
Description |
id |
string |
false |
|
location |
Location |
false |
|
Location
Name |
Type |
Required |
Description |
type |
string |
false |
|
coordinates |
number[ ] |
false |
|
WeatherHourly
Name |
Type |
Required |
Description |
time |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
temperatureC |
number |
false |
|
dewPointC |
number |
false |
|
windKmH |
number |
false |
|
relativeHumidityPercent |
number |
false |
|
precipitationProbabilityPercent |
number |
false |
|
cloudCoverPercent |
number |
false |
|
pressureKilopascals |
number |
false |
|
windGustKmH |
number |
false |
|
WeatherDaily
Name |
Type |
Required |
Description |
time |
integer |
false |
|
sunrise |
integer |
false |
|
sunset |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
dewPointC |
number |
false |
|
minTemperatureC |
number |
false |
|
maxTemperatureC |
number |
false |
|
minWindKmH |
number |
false |
|
maxWindKmH |
number |
false |
|
avgPressureKilopascals |
number |
false |
|
avgCloudCoverPercent |
number |
false |
|
avgRelativeHumidityPercent |
number |
false |
|
maxPrecipitationProbabilityPercent |
number |
false |
|
avgTemperatureC |
number |
false |
|
minRelativeHumidityPercent |
number |
false |
|
maxRelativeHumidityPercent |
number |
false |
|
WeatherMonthly
Name |
Type |
Required |
Description |
time |
integer |
false |
|
etMm |
number |
false |
|
rainMm |
number |
false |
|
ghWm2 |
integer |
false |
|
minTemperatureC |
number |
false |
|
maxTemperatureC |
number |
false |
|
minWindKmH |
number |
false |
|
maxWindKmH |
number |
false |
|
avgPressureKilopascals |
number |
false |
|
avgCloudCoverPercent |
number |
false |
|
avgRelativeHumidityPercent |
number |
false |
|
maxPrecipitationProbabilityPercent |
number |
false |
|
avgWindKmH |
number |
false |
|
avgTemperatureC |
number |
false |
|
avgDewPointC |
number |
false |
|
minRelativeHumidityPercent |
number |
false |
|
maxRelativeHumidityPercent |
number |
false |
|